没有合适的资源?快使用搜索试试~ 我知道了~
首页OpenGL 1.1官方指南:视觉效果编程详解
OpenGL 1.1官方指南:视觉效果编程详解
需积分: 9 0 下载量 75 浏览量
更新于2024-07-24
收藏 7.84MB PDF 举报
"OpenGL红书英文版"是一本由Addison-Wesley Publishing Company出版的第二版专业指南,专为学习OpenGL 1.1而设计。该书是OpenGL图形系统的核心教程,OpenGL作为图形硬件软件接口(GL),为开发者提供了创建交互式程序的强大工具,这些程序能够生成动态的三维图像,实现从现实主义到创新想象的视觉效果。
本书详细介绍了如何利用OpenGL进行编程,帮助读者掌握如何控制计算机图形技术来创造出各种视觉体验。它覆盖了OpenGL的基本概念、核心函数、渲染管线、几何变换、纹理映射、光照和阴影等多个关键领域。通过阅读,读者不仅可以了解到OpenGL的底层原理,还能学习如何实际应用这些技术来构建复杂的3D场景和游戏。
书中特别提到了一些重要的商标声明,如Silicon Graphics、IRIS Graphics Library、XWindow System以及Display PostScript,这些都是相关公司或技术的注册商标。版权方面,本书由Silicon Graphics, Inc.于1997年版权所有,并强调了所有权利保留,禁止未经许可的任何形式复制、存储或传输。
针对那些对计算机图形学感兴趣并希望进一步深入学习OpenGL的开发者而言,这是一本不可或缺的参考书籍。它不仅适合初学者作为入门教材,也适合有一定经验的程序员提升其在图形处理领域的技能。通过阅读《OpenGL Programming Guide》第二版,读者将掌握一个强大且灵活的工具,用于创造现代数字世界中的视觉艺术。"
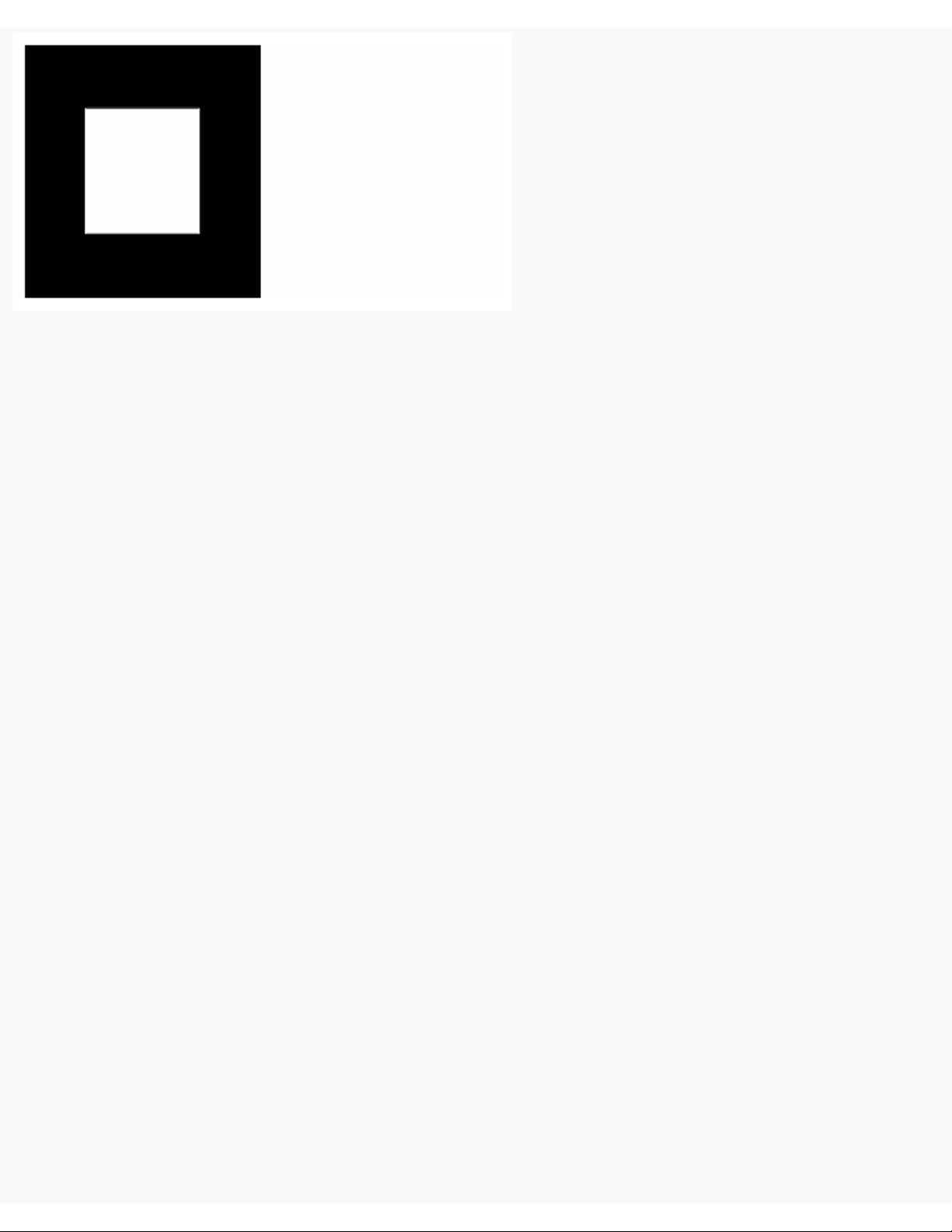
Figure 1-1 : White Rectangle on a Black Background
Example 1-1 : Chunk of OpenGL Code
#include <whateverYouNeed.h>
main() {
InitializeAWindowPlease();
glClearColor (0.0, 0.0, 0.0, 0.0);
glClear (GL_COLOR_BUFFER_BIT);
glColor3f (1.0, 1.0, 1.0);
glOrtho(0.0, 1.0, 0.0, 1.0, -1.0, 1.0);
glBegin(GL_POLYGON);
glVertex3f (0.25, 0.25, 0.0);
glVertex3f (0.75, 0.25, 0.0);
glVertex3f (0.75, 0.75, 0.0);
glVertex3f (0.25, 0.75, 0.0);
glEnd();
glFlush();
UpdateTheWindowAndCheckForEvents();
}
The first line of the main() routine initializes a window on the screen: The InitializeAWindowPlease() routine is meant as a
placeholder for window system-specific routines, which are generally not OpenGL calls. The next two lines are OpenGL
commands that clear the window to black: glClearColor() establishes what color the window will be cleared to, and glClear()
actually clears the window. Once the clearing color is set, the window is cleared to that color whenever glClear() is called. This
clearing color can be changed with another call to glClearColor(). Similarly, the glColor3f() command establishes what color to
use for drawing objects - in this case, the color is white. All objects drawn after this point use this color, until it's changed with
another call to set the color.
The next OpenGL command used in the program, glOrtho(), specifies the coordinate system OpenGL assumes as it draws the
final image and how the image gets mapped to the screen. The next calls, which are bracketed by glBegin() and glEnd(), define
the object to be drawn - in this example, a polygon with four vertices. The polygon's "corners" are defined by the glVertex3f()
commands. As you might be able to guess from the arguments, which are (x, y, z) coordinates, the polygon is a rectangle on the
z=0 plane.
Finally, glFlush() ensures that the drawing commands are actually executed rather than stored in a buffer awaiting additional
OpenGL commands. The UpdateTheWindowAndCheckForEvents() placeholder routine manages the contents of the window
and begins event processing.
OpenGL Programming Guide (Addison-Wesley Publishing Company)
http://heron.cc.ukans.edu/ebt-bin/nph-dweb/dynaw...PG/@Generic__BookTextView/622;cs=fullhtml;pt=532 (4 of 16) [4/28/2000 9:44:15 PM]
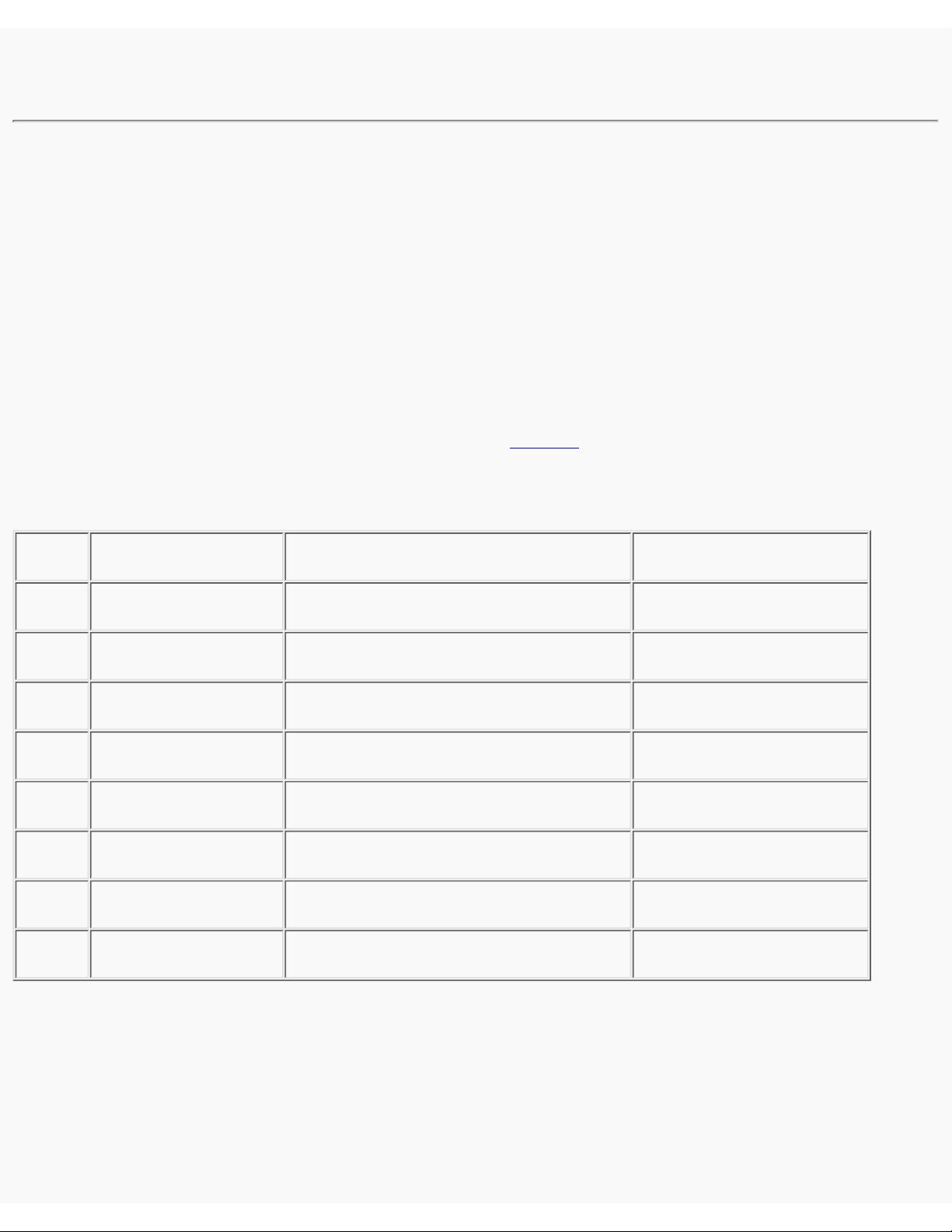
Actually, this piece of OpenGL code isn't well structured. You may be asking, "What happens if I try to move or resize the
window?" Or, "Do I need to reset the coordinate system each time I draw the rectangle?" Later in this chapter, you will see
replacements for both InitializeAWindowPlease() and UpdateTheWindowAndCheckForEvents() that actually work but will
require restructuring the code to make it efficient.
OpenGL Command Syntax
As you might have observed from the simple program in the previous section, OpenGL commands use the prefix gl and initial
capital letters for each word making up the command name (recall glClearColor(), for example). Similarly, OpenGL defined
constants begin with GL_, use all capital letters, and use underscores to separate words (like GL_COLOR_BUFFER_BIT).
You might also have noticed some seemingly extraneous letters appended to some command names (for example, the 3f in
glColor3f() and glVertex3f()). It's true that the Color part of the command name glColor3f() is enough to define the command
as one that sets the current color. However, more than one such command has been defined so that you can use different types of
arguments. In particular, the 3 part of the suffix indicates that three arguments are given; another version of the Color command
takes four arguments. The f part of the suffix indicates that the arguments are floating-point numbers. Having different formats
allows OpenGL to accept the user's data in his or her own data format.
Some OpenGL commands accept as many as 8 different data types for their arguments. The letters used as suffixes to specify
these data types for ISO C implementations of OpenGL are shown in Table 1-1, along with the corresponding OpenGL type
definitions. The particular implementation of OpenGL that you're using might not follow this scheme exactly; an implementation
in C++ or Ada, for example, wouldn't need to.
Table 1-1 : Command Suffixes and Argument Data Types
Suffix Data Type Typical Corresponding C-Language Type OpenGL Type Definition
b 8-bit integer signed char GLbyte
s 16-bit integer short GLshort
i 32-bit integer int or long GLint, GLsizei
f 32-bit floating-point float GLfloat, GLclampf
d 64-bit floating-point double GLdouble, GLclampd
ub 8-bit unsigned integer unsigned char GLubyte, GLboolean
us 16-bit unsigned integer unsigned short GLushort
ui 32-bit unsigned integer unsigned int or unsigned long GLuint, GLenum, GLbitfield
Thus, the two commands
glVertex2i(1, 3);
glVertex2f(1.0, 3.0);
are equivalent, except that the first specifies the vertex's coordinates as 32-bit integers, and the second specifies them as
single-precision floating-point numbers.
OpenGL Programming Guide (Addison-Wesley Publishing Company)
http://heron.cc.ukans.edu/ebt-bin/nph-dweb/dynaw...PG/@Generic__BookTextView/622;cs=fullhtml;pt=532 (5 of 16) [4/28/2000 9:44:15 PM]
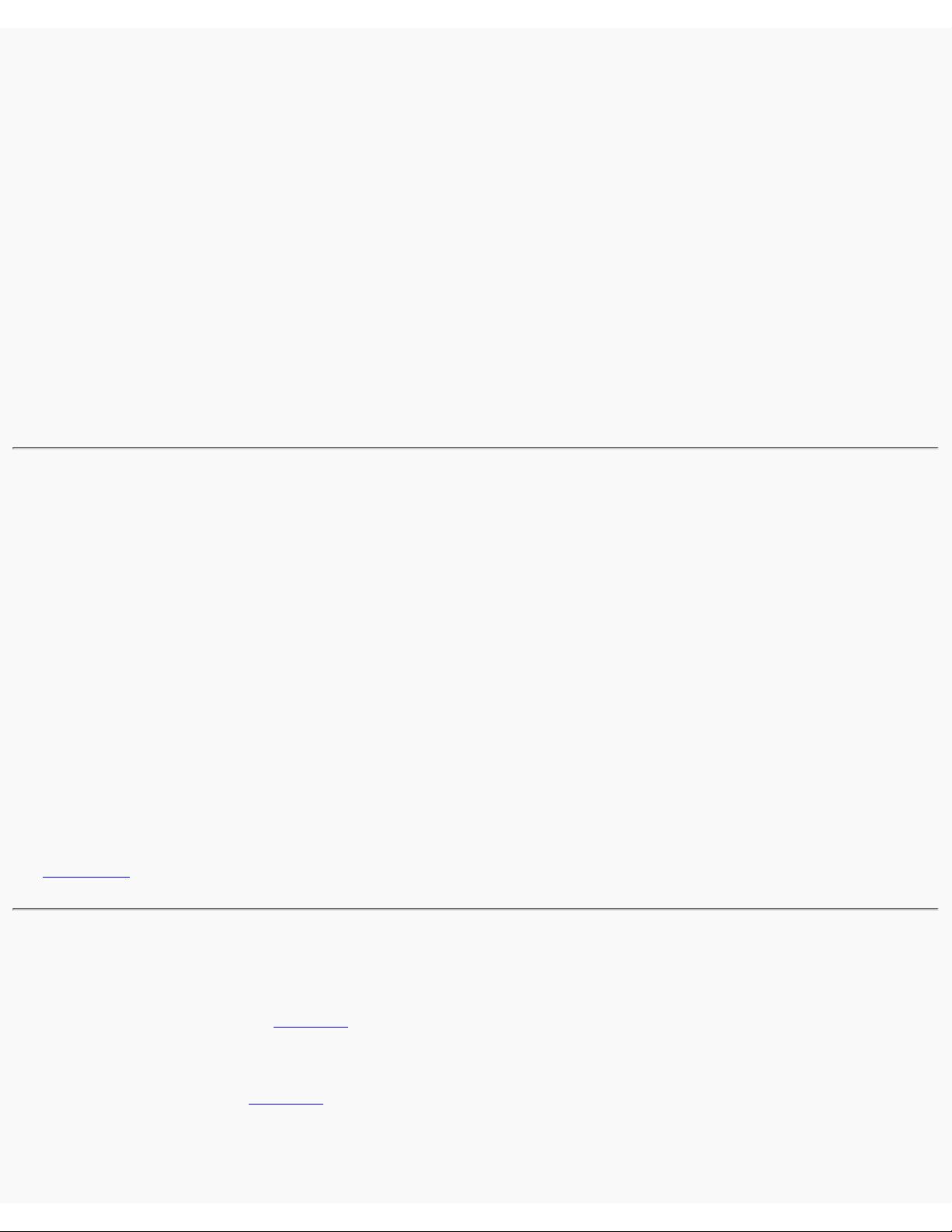
Note: Implementations of OpenGL have leeway in selecting which C data type to use to represent OpenGL data types. If you
resolutely use the OpenGL defined data types throughout your application, you will avoid mismatched types when porting your
code between different implementations.
Some OpenGL commands can take a final letter v, which indicates that the command takes a pointer to a vector (or array) of
values rather than a series of individual arguments. Many commands have both vector and nonvector versions, but some
commands accept only individual arguments and others require that at least some of the arguments be specified as a vector. The
following lines show how you might use a vector and a nonvector version of the command that sets the current color:
glColor3f(1.0, 0.0, 0.0);
GLfloat color_array[] = {1.0, 0.0, 0.0};
glColor3fv(color_array);
Finally, OpenGL defines the typedef GLvoid. This is most often used for OpenGL commands that accept pointers to arrays of
values.
In the rest of this guide (except in actual code examples), OpenGL commands are referred to by their base names only, and an
asterisk is included to indicate that there may be more to the command name. For example, glColor*() stands for all variations of
the command you use to set the current color. If we want to make a specific point about one version of a particular command, we
include the suffix necessary to define that version. For example, glVertex*v() refers to all the vector versions of the command
you use to specify vertices.
OpenGL as a State Machine
OpenGL is a state machine. You put it into various states (or modes) that then remain in effect until you change them. As you've
already seen, the current color is a state variable. You can set the current color to white, red, or any other color, and thereafter
every object is drawn with that color until you set the current color to something else. The current color is only one of many state
variables that OpenGL maintains. Others control such things as the current viewing and projection transformations, line and
polygon stipple patterns, polygon drawing modes, pixel-packing conventions, positions and characteristics of lights, and material
properties of the objects being drawn. Many state variables refer to modes that are enabled or disabled with the command
glEnable() or glDisable().
Each state variable or mode has a default value, and at any point you can query the system for each variable's current value.
Typically, you use one of the six following commands to do this: glGetBooleanv(), glGetDoublev(), glGetFloatv(),
glGetIntegerv(), glGetPointerv(), or glIsEnabled(). Which of these commands you select depends on what data type you want
the answer to be given in. Some state variables have a more specific query command (such as glGetLight*(), glGetError(), or
glGetPolygonStipple()). In addition, you can save a collection of state variables on an attribute stack with glPushAttrib() or
glPushClientAttrib(), temporarily modify them, and later restore the values with glPopAttrib() or glPopClientAttrib(). For
temporary state changes, you should use these commands rather than any of the query commands, since they're likely to be more
efficient.
See Appendix B for the complete list of state variables you can query. For each variable, the appendix also lists a suggested
glGet*() command that returns the variable's value, the attribute class to which it belongs, and the variable's default value.
OpenGL Rendering Pipeline
Most implementations of OpenGL have a similar order of operations, a series of processing stages called the OpenGL rendering
pipeline. This ordering, as shown in Figure 1-2, is not a strict rule of how OpenGL is implemented but provides a reliable guide
for predicting what OpenGL will do.
If you are new to three-dimensional graphics, the upcoming description may seem like drinking water out of a fire hose. You can
skim this now, but come back to Figure 1-2 as you go through each chapter in this book.
The following diagram shows the Henry Ford assembly line approach, which OpenGL takes to processing data. Geometric data
(vertices, lines, and polygons) follow the path through the row of boxes that includes evaluators and per-vertex operations, while
OpenGL Programming Guide (Addison-Wesley Publishing Company)
http://heron.cc.ukans.edu/ebt-bin/nph-dweb/dynaw...PG/@Generic__BookTextView/622;cs=fullhtml;pt=532 (6 of 16) [4/28/2000 9:44:16 PM]
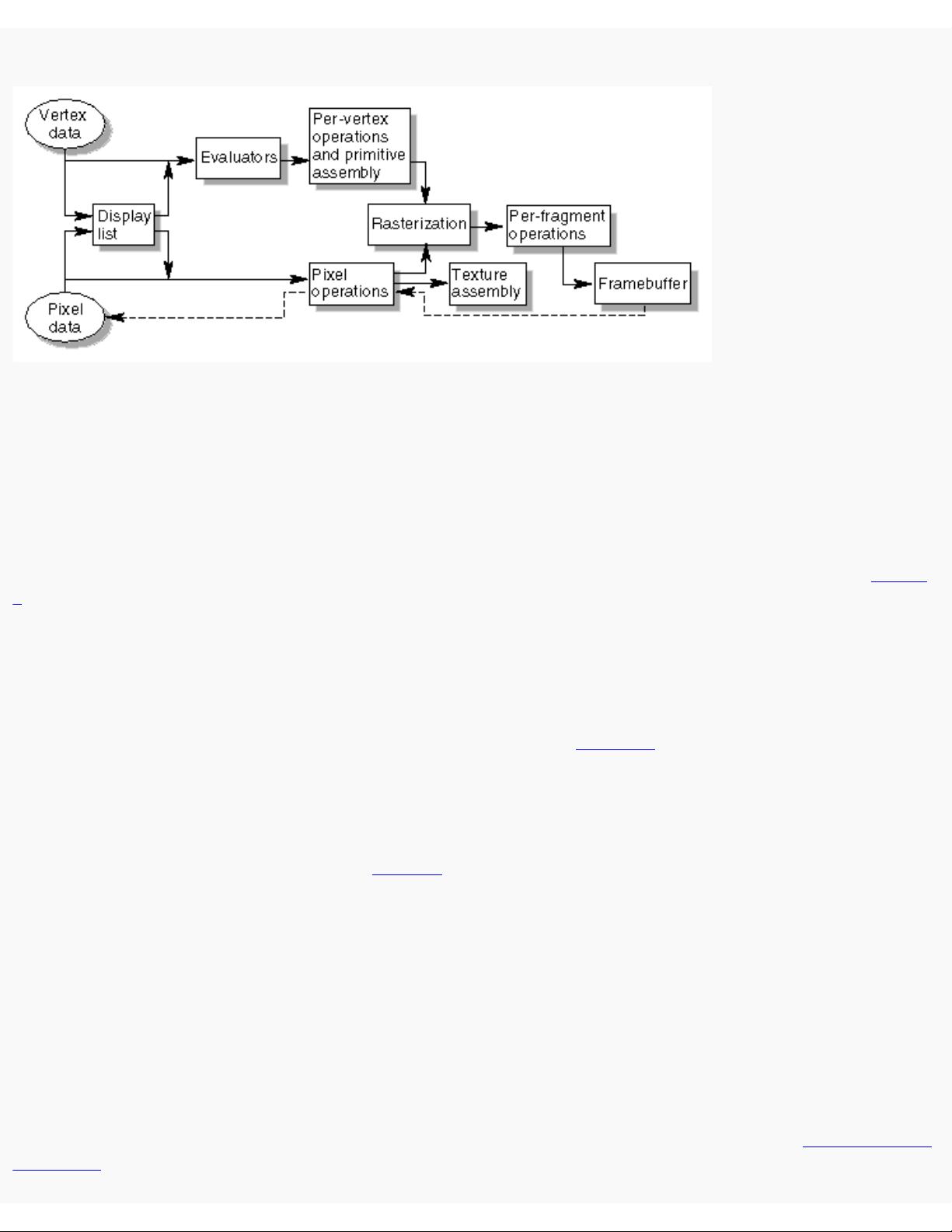
pixel data (pixels, images, and bitmaps) are treated differently for part of the process. Both types of data undergo the same final
steps (rasterization and per-fragment operations) before the final pixel data is written into the framebuffer.
Figure 1-2 : Order of Operations
Now you'll see more detail about the key stages in the OpenGL rendering pipeline.
Display Lists
All data, whether it describes geometry or pixels, can be saved in a display list for current or later use. (The alternative to
retaining data in a display list is processing the data immediately - also known as immediate mode.) When a display list is
executed, the retained data is sent from the display list just as if it were sent by the application in immediate mode. (See Chapter
7 for more information about display lists.)
Evaluators
All geometric primitives are eventually described by vertices. Parametric curves and surfaces may be initially described by
control points and polynomial functions called basis functions. Evaluators provide a method to derive the vertices used to
represent the surface from the control points. The method is a polynomial mapping, which can produce surface normal, texture
coordinates, colors, and spatial coordinate values from the control points. (See Chapter 12 to learn more about evaluators.)
Per-Vertex Operations
For vertex data, next is the "per-vertex operations" stage, which converts the vertices into primitives. Some vertex data (for
example, spatial coordinates) are transformed by 4 x 4 floating-point matrices. Spatial coordinates are projected from a position
in the 3D world to a position on your screen. (See Chapter 3 for details about the transformation matrices.)
If advanced features are enabled, this stage is even busier. If texturing is used, texture coordinates may be generated and
transformed here. If lighting is enabled, the lighting calculations are performed using the transformed vertex, surface normal,
light source position, material properties, and other lighting information to produce a color value.
Primitive Assembly
Clipping, a major part of primitive assembly, is the elimination of portions of geometry which fall outside a half-space, defined
by a plane. Point clipping simply passes or rejects vertices; line or polygon clipping can add additional vertices depending upon
how the line or polygon is clipped.
In some cases, this is followed by perspective division, which makes distant geometric objects appear smaller than closer objects.
Then viewport and depth (z coordinate) operations are applied. If culling is enabled and the primitive is a polygon, it then may be
rejected by a culling test. Depending upon the polygon mode, a polygon may be drawn as points or lines. (See "Polygon Details"
in Chapter 2.)
OpenGL Programming Guide (Addison-Wesley Publishing Company)
http://heron.cc.ukans.edu/ebt-bin/nph-dweb/dynaw...PG/@Generic__BookTextView/622;cs=fullhtml;pt=532 (7 of 16) [4/28/2000 9:44:16 PM]
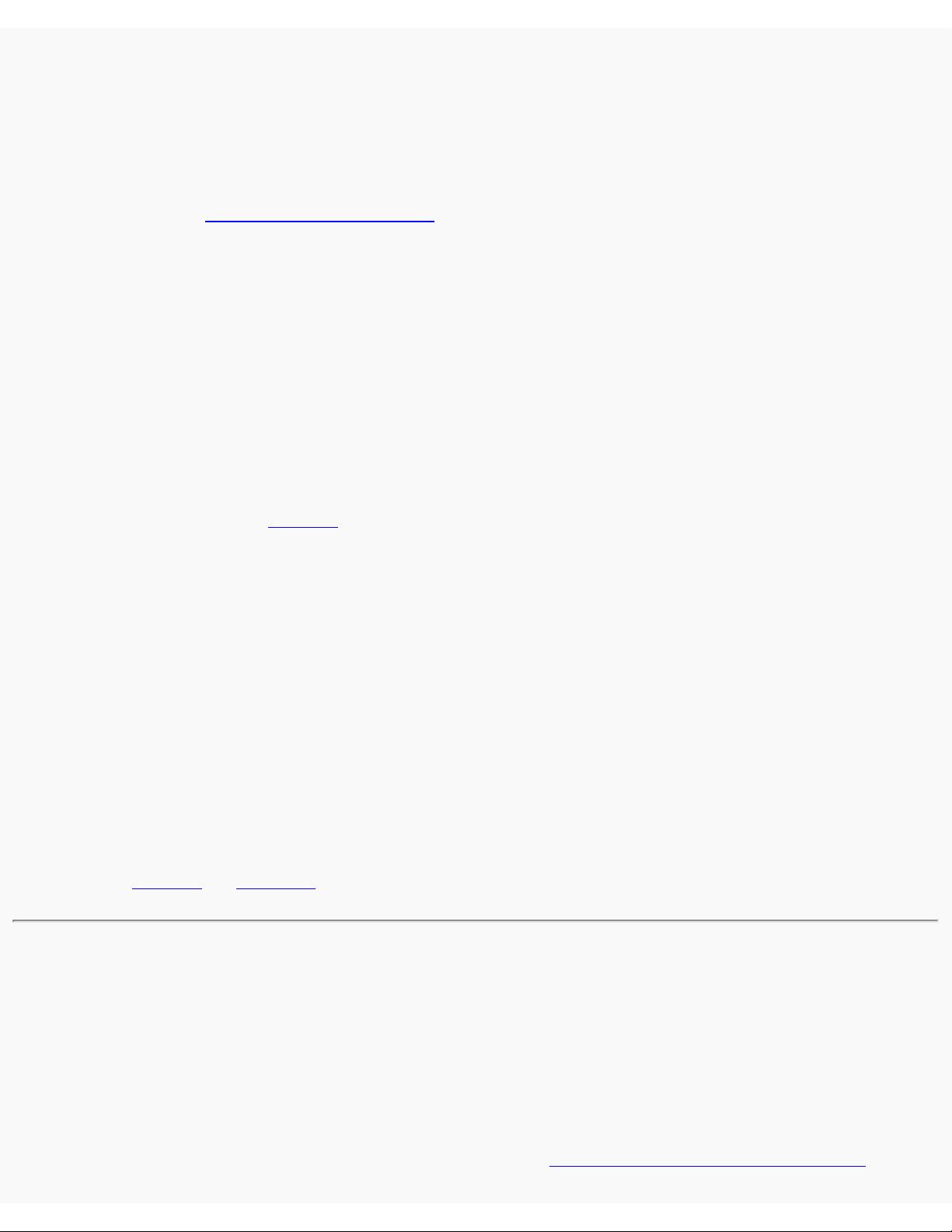
The results of this stage are complete geometric primitives, which are the transformed and clipped vertices with related color,
depth, and sometimes texture-coordinate values and guidelines for the rasterization step.
Pixel Operations
While geometric data takes one path through the OpenGL rendering pipeline, pixel data takes a different route. Pixels from an
array in system memory are first unpacked from one of a variety of formats into the proper number of components. Next the data
is scaled, biased, and processed by a pixel map. The results are clamped and then either written into texture memory or sent to
the rasterization step. (See "Imaging Pipeline" in Chapter 8.)
If pixel data is read from the frame buffer, pixel-transfer operations (scale, bias, mapping, and clamping) are performed. Then
these results are packed into an appropriate format and returned to an array in system memory.
There are special pixel copy operations to copy data in the framebuffer to other parts of the framebuffer or to the texture memory.
A single pass is made through the pixel transfer operations before the data is written to the texture memory or back to the
framebuffer.
Texture Assembly
An OpenGL application may wish to apply texture images onto geometric objects to make them look more realistic. If several
texture images are used, it's wise to put them into texture objects so that you can easily switch among them.
Some OpenGL implementations may have special resources to accelerate texture performance. There may be specialized,
high-performance texture memory. If this memory is available, the texture objects may be prioritized to control the use of this
limited and valuable resource. (See Chapter 9.)
Rasterization
Rasterization is the conversion of both geometric and pixel data into fragments. Each fragment square corresponds to a pixel in
the framebuffer. Line and polygon stipples, line width, point size, shading model, and coverage calculations to support
antialiasing are taken into consideration as vertices are connected into lines or the interior pixels are calculated for a filled
polygon. Color and depth values are assigned for each fragment square.
Fragment Operations
Before values are actually stored into the framebuffer, a series of operations are performed that may alter or even throw out
fragments. All these operations can be enabled or disabled.
The first operation which may be encountered is texturing, where a texel (texture element) is generated from texture memory for
each fragment and applied to the fragment. Then fog calculations may be applied, followed by the scissor test, the alpha test, the
stencil test, and the depth-buffer test (the depth buffer is for hidden-surface removal). Failing an enabled test may end the
continued processing of a fragment's square. Then, blending, dithering, logical operation, and masking by a bitmask may be
performed. (See Chapter 6 and Chapter 10) Finally, the thoroughly processedfragment is drawn into the appropriate buffer,
where it has finally advanced to be a pixel and achieved its final resting place.
OpenGL-Related Libraries
OpenGL provides a powerful but primitive set of rendering commands, and all higher-level drawing must be done in terms of
these commands. Also, OpenGL programs have to use the underlying mechanisms of the windowing system. A number of
libraries exist to allow you to simplify your programming tasks, including the following:
The OpenGL Utility Library (GLU) contains several routines that use lower-level OpenGL commands to perform such
tasks as setting up matrices for specific viewing orientations and projections, performing polygon tessellation, and
rendering surfaces. This library is provided as part of every OpenGL implementation. Portions of the GLU are described in
the OpenGL Reference Manual. The more useful GLU routines are described in this guide, where they're relevant to the
topic being discussed, such as in all of Chapter 11 and in the section "The GLU NURBS Interface" in Chapter 12. GLU
●
OpenGL Programming Guide (Addison-Wesley Publishing Company)
http://heron.cc.ukans.edu/ebt-bin/nph-dweb/dynaw...PG/@Generic__BookTextView/622;cs=fullhtml;pt=532 (8 of 16) [4/28/2000 9:44:16 PM]
剩余615页未读,继续阅读
2022-01-16 上传
2010-07-29 上传
2024-08-30 上传
2021-03-22 上传

wchyumo2009
- 粉丝: 30
- 资源: 3
上传资源 快速赚钱
我的内容管理 展开
我的资源 快来上传第一个资源
我的收益
登录查看自己的收益我的积分 登录查看自己的积分
我的C币 登录后查看C币余额
我的收藏
我的下载
下载帮助

最新资源
- myilportfolio
- GH1.25连接器封装PCB文件3D封装AD库
- Network-Canvas-Web:网络画布的主要网站
- 基于机器学习和LDA主题模型的缺陷报告分派方法的Python实现。原论文为:Accurate developer r.zip
- ReactBlogProject:Blog项目,测试模块,React函数和后端集成
- prefuse-caffe-layout-visualization:杂项 BVLC Caffe .prototxt 实用程序
- thresholding_operator:每个单元基于阈值的标志值
- 基于深度学习的计算机视觉(python+tensorflow))文件学习.zip
- app-sistemaweb:sistema web de citas medicasRuby在轨道上
- 记录书籍学习的笔记,顺便分享一些学习的项目笔记。包括了Python和SAS内容,也包括了Tableau、SPSS数据.zip
- bpm-validator:Bizagi BPM 验证器
- DocBook ToolKit-开源
- file_renamer:通过文本编辑器轻松重命名文件和文件夹
- log4j-to-slf4j-2.10.0-API文档-中文版.zip
- django-advanced-forms:Django高级脆皮形式用法示例
- android-sispur
安全验证
文档复制为VIP权益,开通VIP直接复制
