C语言常用函数详解及示例
2.虚拟产品一经售出概不退款(资源遇到问题,请及时私信上传者)
"C语言函数大全" 在C语言中,函数是编程的重要组成部分,它们用于封装可重用的代码块。以下是一些重要的C语言函数的详细解释: 1. abort() 函数名:abort 功能:这个函数用于异常情况下立即终止进程的执行,通常在无法恢复的错误发生时调用。 用法:`void abort(void);` 示例: ```c #include<stdio.h> #include<stdlib.h> int main(void) { printf("Calling abort()\n"); abort(); return 0; /* This is never reached */ } ``` 2. abs() 函数名:abs 功能:该函数返回一个整数的绝对值,即去掉负号后的值。 用法:`int abs(int i);` 示例: ```c #include<stdio.h> #include<math.h> int main(void) { int number = -1234; printf("number: %d absolute value: %d\n", number, abs(number)); return 0; } ``` 3. absread(), abswrite() 函数名:absread, abswrite 功能:这两个函数分别用于直接读取和写入磁盘扇区的数据,通常在低级别的磁盘操作中使用。 用法: ``` int absread(int drive, int nsects, int sectno, void* buffer); int abswrite(int drive, int nsects, int sectno, void* buffer); ``` 示例(absread示例): ```c /* absread example */ #include<stdio.h> #include<conio.h> #include<process.h> #include<dos.h> int main(void) { int i, strt, ch_out, sector; char buf[512]; printf("Insert a diskette into drive A and press any key\n"); getch(); sector = 0; if (absread(0, 1, sector, &buf) != 0) { perror("Disk problem"); exit(1); } printf("Read OK\n"); strt = 3; for (i = 0; i < 80; i++) { ch_out = buf[strt + i]; putchar(ch_out); } printf("\n"); return(0); } ``` 4. access() 函数名:access 功能:该函数用于检查指定文件或目录的访问权限,如读、写或执行权限。 用法:`int access(const char* filename, int amode);` 示例: ```c #include<stdio.h> #include<unistd.h> int main(void) { if (access("myfile.txt", R_OK) == 0) { printf("File 'myfile.txt' can be read.\n"); } else { printf("File 'myfile.txt' cannot be read.\n"); } return 0; } ``` 这些函数展示了C语言中的基本功能,包括错误处理、数学运算、磁盘I/O以及文件访问。在实际编程中,了解并熟练使用这些函数对于编写高效且可靠的C程序至关重要。C语言函数库还包括大量的其他函数,例如内存管理、字符串操作、时间处理等,这些都是构建复杂程序的基础。通过深入学习和实践,开发者可以充分利用C语言的这些功能来解决各种问题。
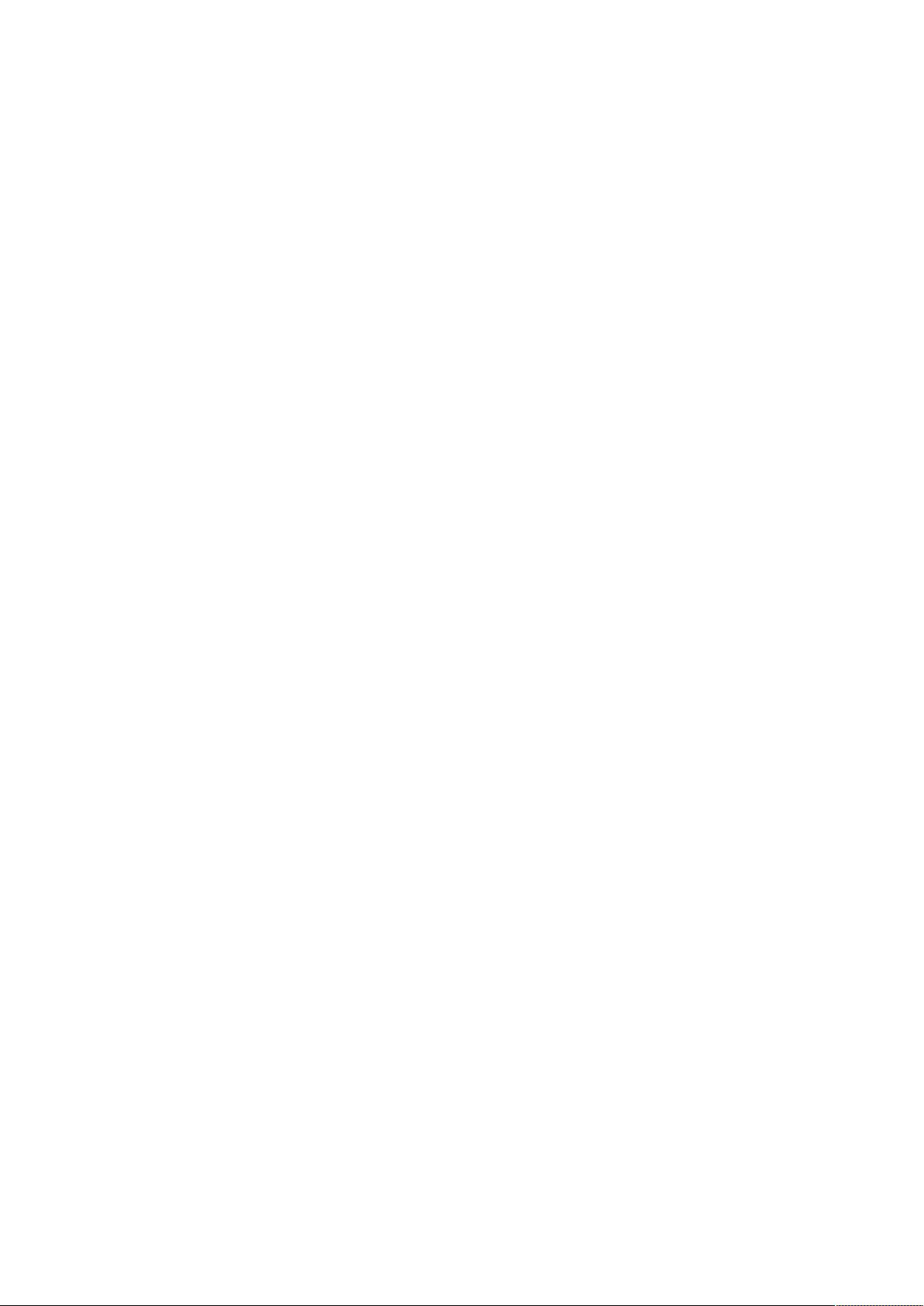
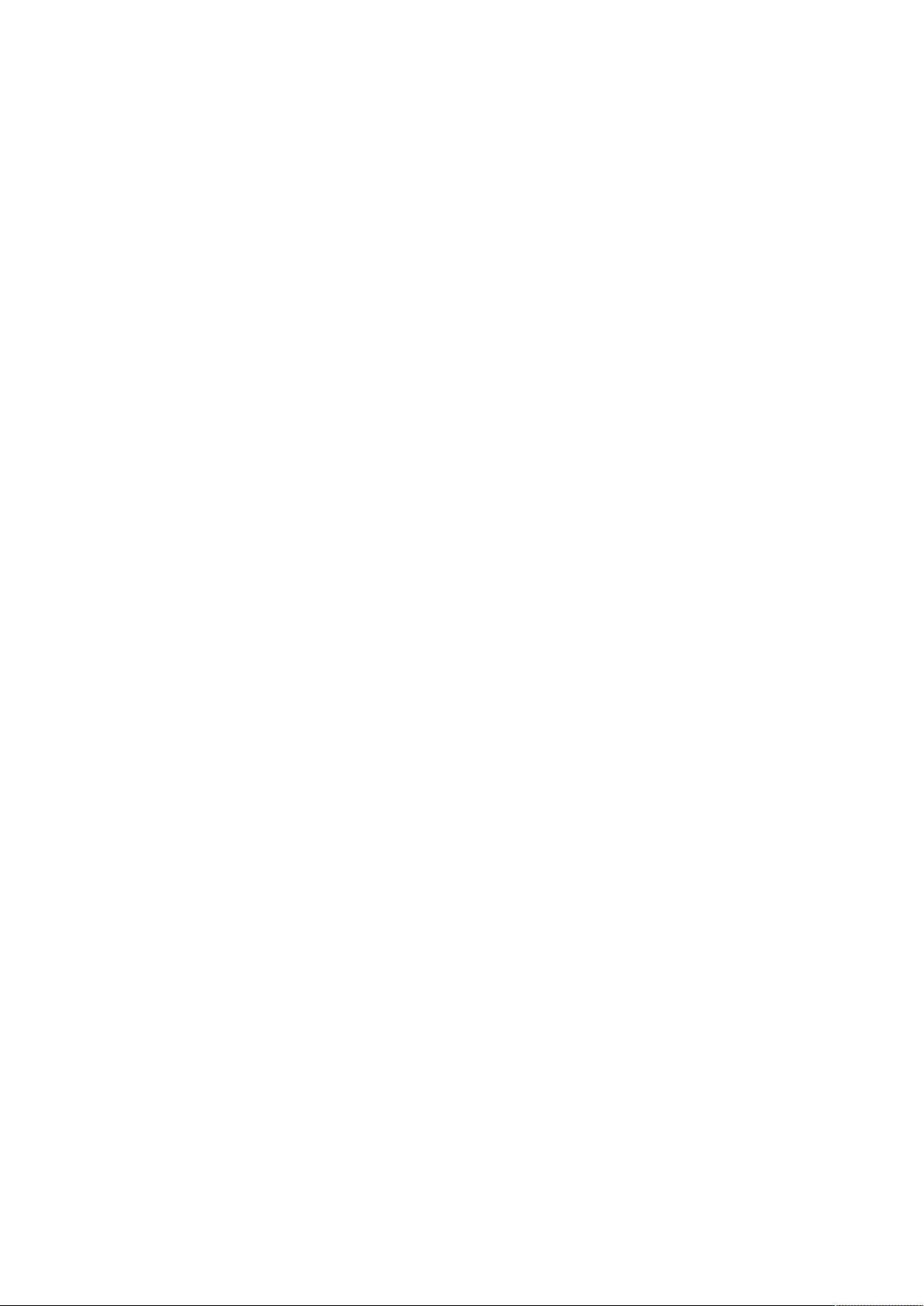
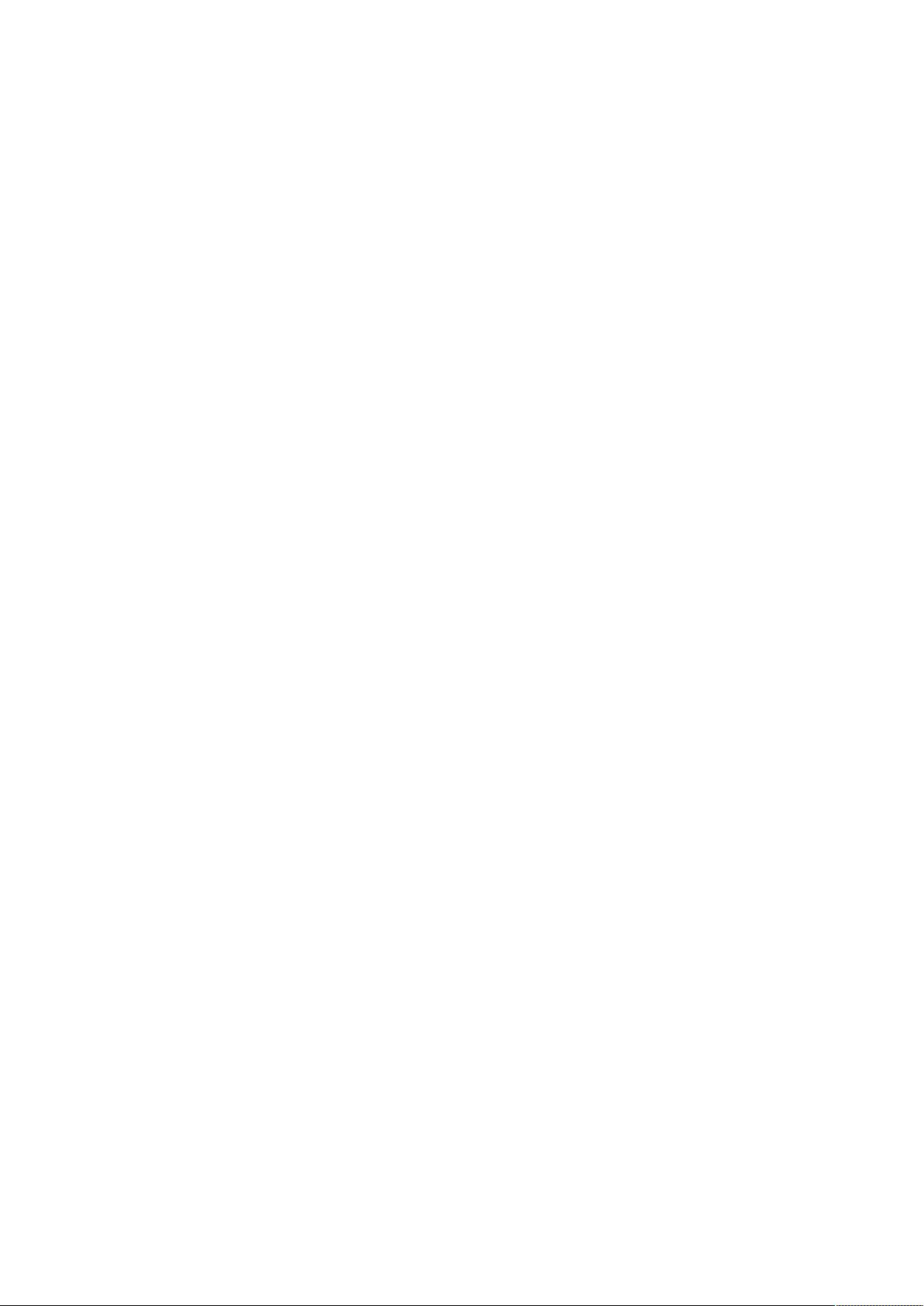
剩余63页未读,继续阅读
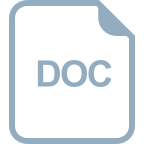

















- 粉丝: 3786
- 资源: 59万+





我的内容管理 展开
我的资源 快来上传第一个资源
我的收益
登录查看自己的收益我的积分 登录查看自己的积分
我的C币 登录后查看C币余额
我的收藏
我的下载
下载帮助

最新资源
- zlib-1.2.12压缩包解析与技术要点
- 微信小程序滑动选项卡源码模版发布
- Unity虚拟人物唇同步插件Oculus Lipsync介绍
- Nginx 1.18.0版本WinSW自动安装与管理指南
- Java Swing和JDBC实现的ATM系统源码解析
- 掌握Spark Streaming与Maven集成的分布式大数据处理
- 深入学习推荐系统:教程、案例与项目实践
- Web开发者必备的取色工具软件介绍
- C语言实现李春葆数据结构实验程序
- 超市管理系统开发:asp+SQL Server 2005实战
- Redis伪集群搭建教程与实践
- 掌握网络活动细节:Wireshark v3.6.3网络嗅探工具详解
- 全面掌握美赛:建模、分析与编程实现教程
- Java图书馆系统完整项目源码及SQL文件解析
- PCtoLCD2002软件:高效图片和字符取模转换
- Java开发的体育赛事在线购票系统源码分析

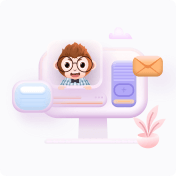
