C语言实现凯撒密码的加密与解密
版权申诉
192 浏览量
更新于2024-10-16
收藏 166KB RAR 举报
资源摘要信息:"凯撒密码C语言实现文档"
凯撒密码是一种最简单和最广为人知的替换加密技术,它通过将字母在字母表中向前或向后移动固定数目来进行加密和解密。在计算机科学和信息科技领域中,凯撒密码常常作为基础加密算法的教学案例,它被用来向初学者展示加密算法的基本概念,包括加密、解密、密钥等核心概念。
凯撒密码的核心原理是字母替换。它涉及以下知识点:
1. 密码学基础:凯撒密码是密码学的一个分支,它研究如何隐藏信息,使未授权者无法读取。凯撒密码就是其中最简单的加密方法之一。
2. 加密与解密:在凯撒密码中,加密是将明文中的每个字母根据密钥(移动的位数)向前移动相应的字母表位置;解密则是相反的过程,根据密钥将加密后的字母向后移动相应的字母表位置。
3. 密钥概念:密钥是加密和解密过程中的关键参数。在凯撒密码中,密钥就是字母移动的数目。例如,若密钥为3,那么字母“A”将被替换为“D”,“B”将被替换为“E”,以此类推。
4. C语言编程:凯撒密码的C语言实现涉及到基本的编程概念,如变量、循环、条件判断和数组等。通过C语言实现凯撒密码可以让学习者了解如何使用编程语言处理文本信息和实现基本的算法。
5. 字符处理:在C语言中,字符通常使用ASCII编码表示。凯撒密码C语言实现时需要正确处理字符的移动,这包括大写字母和小写字母,以及处理字母Z或z到达字母表末尾的情况。
6. 模数运算:为了能够循环地进行字符替换,凯撒密码算法中需要使用模数运算。例如,如果密钥为3,在到达字母“Z”后,下一步应该跳到“C”,而不是直接跳到“D”。这可以通过对26(英文字母的总数)取模来实现。
7. 用户交互:在实现凯撒密码时,通常需要与用户进行交互,允许用户输入要加密或解密的文本,以及密钥的数值。这涉及到标准输入输出函数,如printf和scanf等。
8. 函数封装:为了代码的模块化和重用,可以将加密和解密的过程封装成独立的函数。这样可以提高代码的清晰度和可维护性。
9. 安全性分析:虽然凯撒密码在现代已经不再安全,但研究它可以帮助理解加密算法的弱点。比如,凯撒密码仅通过简单的替换操作,没有复杂的加密逻辑,因此很容易被破解,尤其是当使用频率分析时。
在文件"ma.rar_凯撒密码c"中,我们可以预期到包含上述知识点的详细解释和C语言代码示例。这个资源可能以教程的形式出现,详细说明如何用C语言编写凯撒密码的加密和解密函数,可能包括代码解释、用户交互部分的实现方法,以及如何处理特殊情况(比如非字母字符的输入)。此外,该文件可能还会讨论凯撒密码的局限性,并简要介绍密码学中更为复杂的概念,为学习者提供进一步学习的路径。
2022-09-21 上传
2022-09-21 上传
2022-09-20 上传
2022-09-23 上传
2022-09-21 上传
2022-07-15 上传
2022-09-14 上传
2022-09-14 上传
2022-09-24 上传
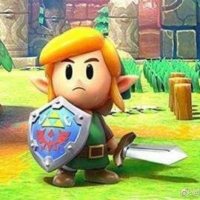
御道御小黑
- 粉丝: 74
- 资源: 1万+
最新资源
- 深入浅出:自定义 Grunt 任务的实践指南
- 网络物理突变工具的多点路径规划实现与分析
- multifeed: 实现多作者间的超核心共享与同步技术
- C++商品交易系统实习项目详细要求
- macOS系统Python模块whl包安装教程
- 掌握fullstackJS:构建React框架与快速开发应用
- React-Purify: 实现React组件纯净方法的工具介绍
- deck.js:构建现代HTML演示的JavaScript库
- nunn:现代C++17实现的机器学习库开源项目
- Python安装包 Acquisition-4.12-cp35-cp35m-win_amd64.whl.zip 使用说明
- Amaranthus-tuberculatus基因组分析脚本集
- Ubuntu 12.04下Realtek RTL8821AE驱动的向后移植指南
- 掌握Jest环境下的最新jsdom功能
- CAGI Toolkit:开源Asterisk PBX的AGI应用开发
- MyDropDemo: 体验QGraphicsView的拖放功能
- 远程FPGA平台上的Quartus II17.1 LCD色块闪烁现象解析