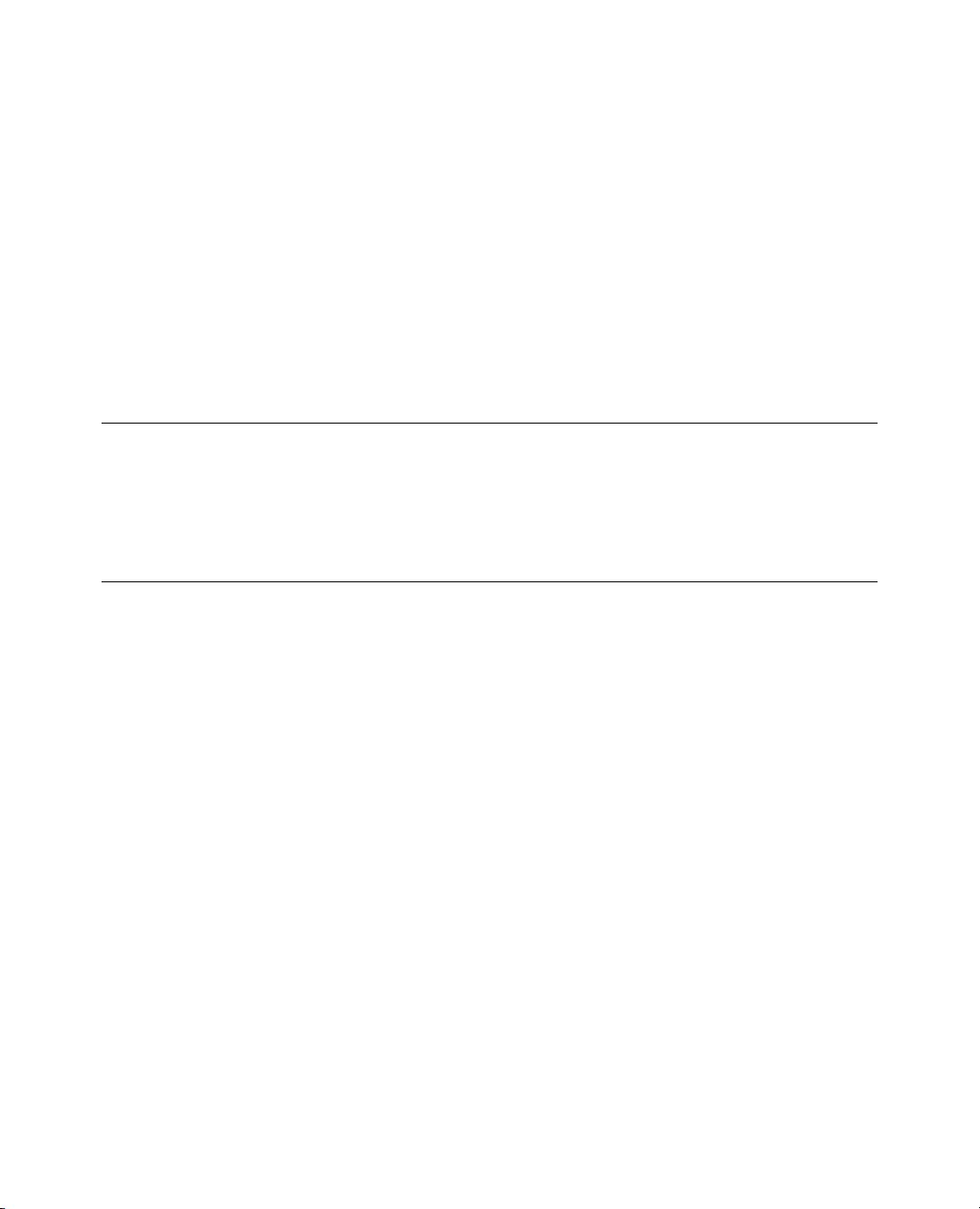
CHAPTER 1 JAVAFX FUNDAMENTALS
10
|projects
|helloworld
|HelloWorldMain.java
| helloworldmain
|HelloWorldMain.class
With the preceding directory structure in mind, the following commands will compile and run our
JavaFX Hello World application:
cd /projects/helloworld
javac –d . HelloWorldMain.java
java helloworldmain.HelloWorldMain
Note There are many ways to package and deploy JavaFX applications. To learn more, please see “Learning
how to deploy and package JavaFX applications” at
http://blogs.oracle.com/thejavatutorials/entry/javafx_2_0_beta_packager. For in-depth JavaFX
deployment strategies, see Oracle’s “Deploying JavaFX Applications” at
http://download.oracle.com/javafx/2.0/deployment/deployment_toolkit.htm.
In both solutions you’ll notice in the source code that JavaFX applications extend the
javafx.application.Application class. The Application class provides application life cycle functions
such as launching and stopping during runtime. This also provides a mechanism for Java applications to
launch JavaFX GUI components in a threadsafe manner. Keep in mind that synonymous to Java Swing’s
event dispatch thread, JavaFX will have its own JavaFX application thread.
In our main() method’s entry point we launch the JavaFX application by simply passing in the
command line arguments to the Application.launch() method. Once the application is in a ready state,
the framework internals will invoke the start() method to begin. When the start() method is invoked,
a JavaFX javafx.stage.Stage object is available for the developer to use and manipulate.
You’ll notice that some objects are oddly named, such as Stage or Scene. The designers of the API
have modeled things similar to a theater or a play in which actors perform in front of an audience. With
this same analogy, in order to show a play, there are basically one-to-many scenes that actors perform
in. And, of course, all scenes are performed on a stage. In JavaFX the Stage is equivalent to an application
window similar to Java Swing API JFrame or JDialog. You may think of a Scene object as a content pane
capable of holding zero-to-many Node objects. A Node is a fundamental base class for all scene graph
nodes to be rendered. Commonly used nodes are UI controls and Shape objects. Similar to a tree data
structure, a scene graph will contain children nodes by using a container class Group. We’ll learn more
about the Group class later when we look at the ObservableList, but for now we can think of them as Java
Lists or Collections that are capable of holding Nodes.
Once the child nodes have been added, we set the primaryStage’s (Stage) scene and call the show()
method on the Stage object to show the JavaFX window.
One last thing: in this chapter most of the example applications will be structured the same as this
example in which recipe code solutions will reside inside the start() method. Having said this, most of
the recipes in this chapter will follow the same pattern. In other words, for the sake of brevity, much of
www.it-ebooks.info