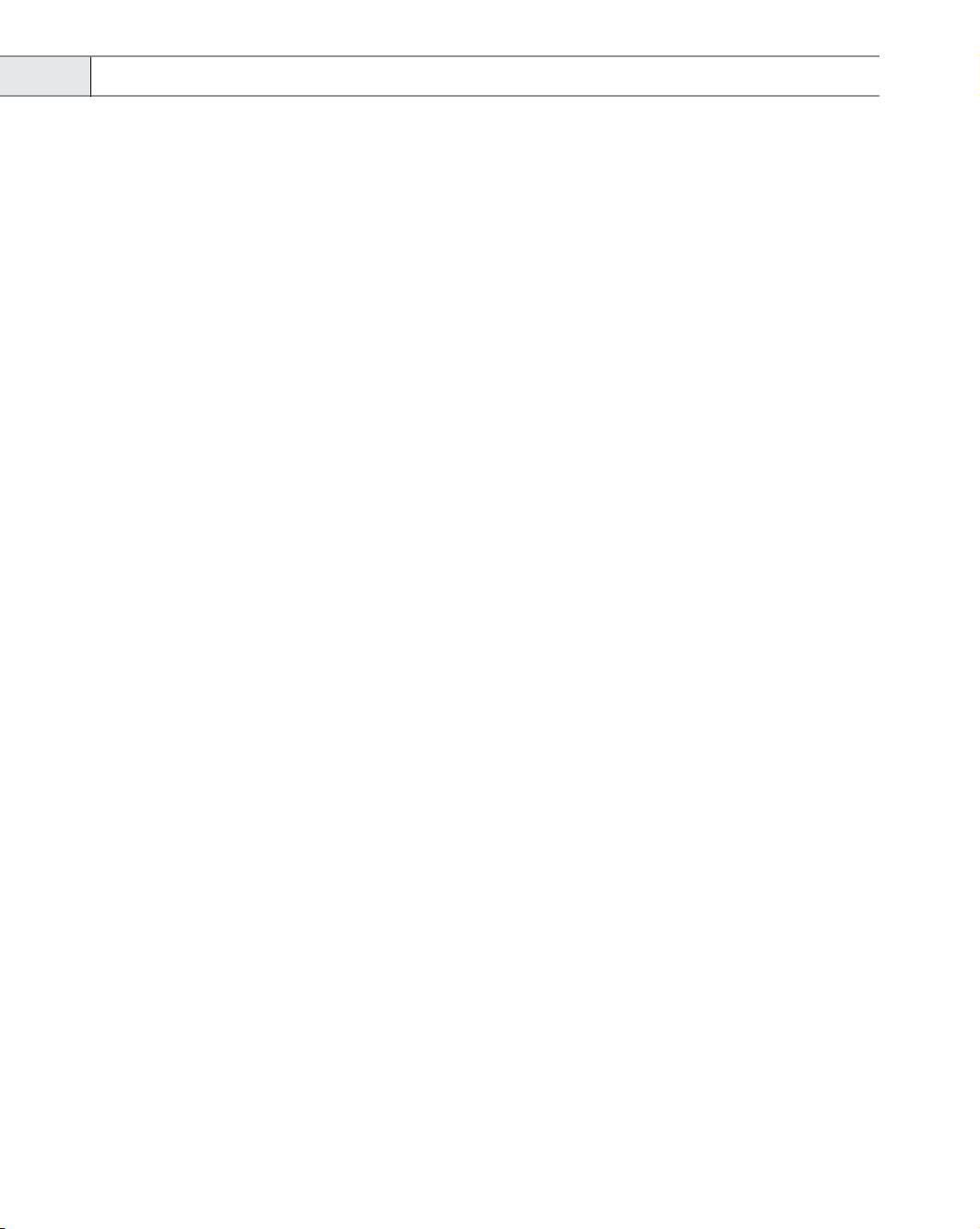
xiv Contents
RESTfulEngine Architecture (iHotelApp Sample Code) ..........................192
NSURLConnection Versus Third-Party Frameworks . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 193
Creating the RESTfulEngine . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 193
Adding Authentication to the RESTfulEngine ........................................ 194
Adding Blocks to the RESTfulEngine ................................................. 195
Authenticating Your API Calls with Access Tokens . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 196
Overriding Methods to Add Custom Authentication
Headers in RESTfulEngine.m . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 197
Canceling Requests . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 197
Request Responses . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 198
Key Value Coding JSONs . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 198
List Versus Detail JSON Objects . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 201
Nested JSON Objects . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 202
Less Is More . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 203
Error Handling . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 203
Localization . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 205
Handling Additional Formats Using Categories . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 206
Tips to Improve Performance on iOS . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 207
Caching. . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 207
Reasons for Going Oine .....................................................207
Strategies for Caching .........................................................208
Storing the Cache . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 209
Implementing Data Model Caching ................................................ 209
Core Data ........................................................................... 210
Using Core Data for On-Demand Caching .......................................... 210
Raw SQLite .......................................................................... 211
Which Caching Technique Should You Use? ........................................ 211
Data Model Cache Versus URL Cache ............................................... 211
Cache Versioning and Invalidation . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 211
Creating a Data Model Cache ..................................................212
Refactoring ......................................................................... 214
Cache Versioning .............................................................215
Invalidating the Cache . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 216
Creating an In-Memory Cache .................................................217
Designing the In-Memory Cache for AppCache . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 218
Handling Memory Warnings . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 219
Handling Termination and Entering Background Notications . . . . . . . . . . . . . . . . . . . 220
Creating a URL Cache .........................................................221
Expiration Model . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 221
Validation Model . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 221