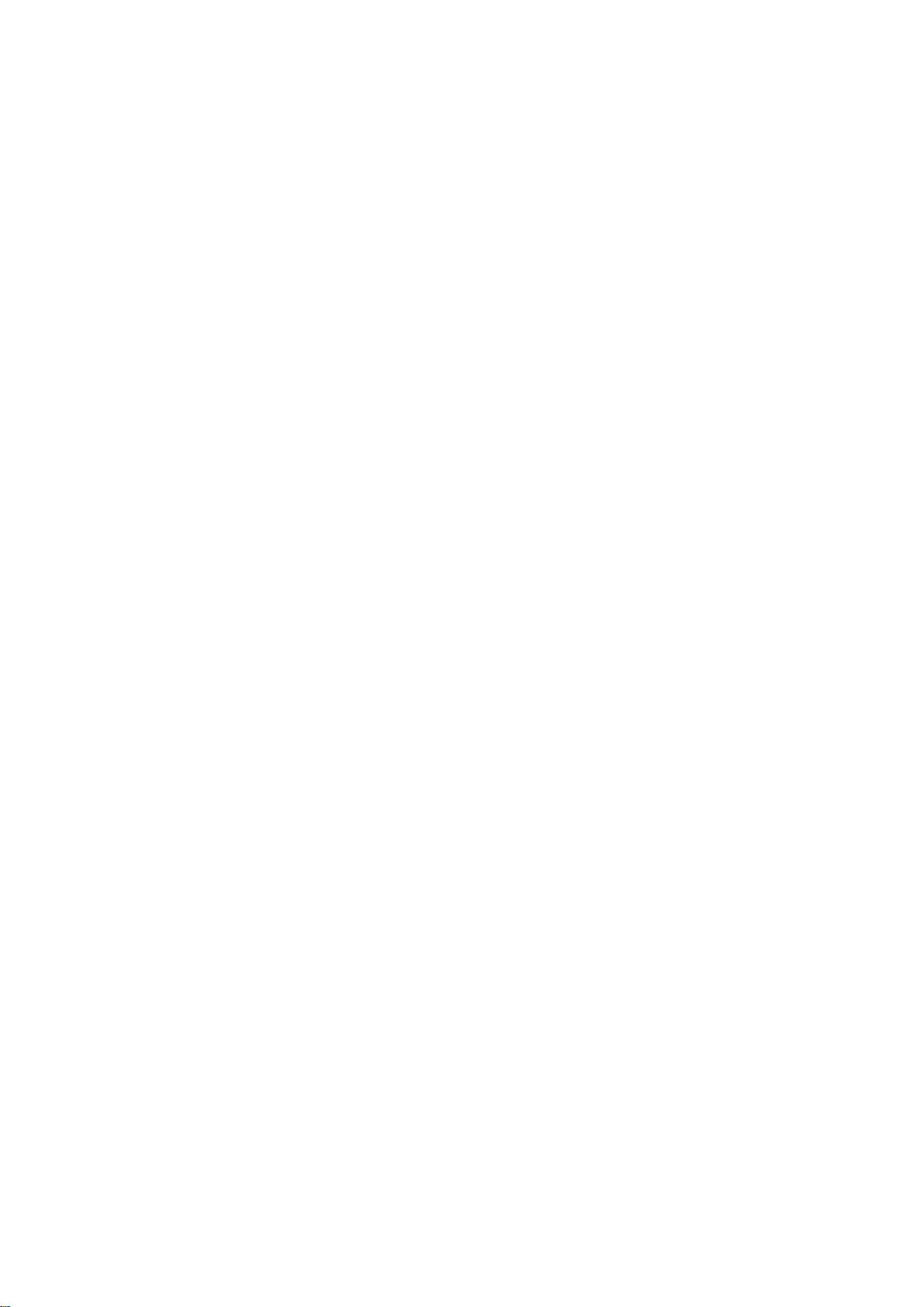
3.26
float Sqrt_recursive(float A,float p,float e)//求平方根的递归算法
{
if(abs(p^2-A)<=e) return p;
else return sqrt_recurve(A,(p+A/p)/2,e);
}//Sqrt_recurve
float Sqrt_nonrecursive(float A,float p,float e)//求平方根的非递归算法
{
while(abs(p^2-A)>=e)
p=(p+A/p)/2;
return p;
}//Sqrt_nonrecursive
3.27
这一题的所有算法以及栈的变化过程请参见《数据结构(pascal 版)》,作者不再详细写出.
3.28
void InitCiQueue(CiQueue &Q)//初始化循环链表表示的队列 Q
{
Q=(CiLNode*)malloc(sizeof(CiLNode));
Q->next=Q;
}//InitCiQueue
void EnCiQueue(CiQueue &Q,int x)//把元素 x插入循环链表表示的队列 Q,Q 指向队尾元
素,Q->next 指向头结点,Q->next->next 指向队头元素
{
p=(CiLNode*)malloc(sizeof(CiLNode));
p->data=x;
p->next=Q->next; //直接把 p 加在 Q 的后面
Q->next=p;
Q=p; //修改尾指针
}
Status DeCiQueue(CiQueue &Q,int x)//从循环链表表示的队列 Q 头部删除元素 x
{
if(Q==Q->next) return INFEASIBLE; //队列已空
p=Q->next->next;
x=p->data;
Q->next->next=p->next;
free(p);
return OK;
}//DeCiQueue
3.29
Status EnCyQueue(CyQueue &Q,int x)//带 tag 域的循环队列入队算法
{
if(Q.front==Q.rear&&Q.tag==1) //tag 域的值为 0 表示"空",1 表示"满"
return OVERFLOW;
Q.base[Q.rear]=x;
Q.rear=(Q.rear+1)%MAXSIZE;
if(Q.front==Q.rear) Q.tag=1; //队列满
}//EnCyQueue
Status DeCyQueue(CyQueue &Q,int &x)//带 tag 域的循环队列出队算法
{
if(Q.front==Q.rear&&Q.tag==0) return INFEASIBLE;
Q.front=(Q.front+1)%MAXSIZE;
x=Q.base[Q.front];
if(Q.front==Q.rear) Q.tag=1; //队列空
return OK;
}//DeCyQueue
分析:当循环队列容量较小而队列中每个元素占的空间较多时,此种表示方法可以节约较多
的存储空间,较有价值.
3.30
Status EnCyQueue(CyQueue &Q,int x)//带 length 域的循环队列入队算法
{
if(Q.length==MAXSIZE) return OVERFLOW;
Q.rear=(Q.rear+1)%MAXSIZE;
Q.base[Q.rear]=x;
Q.length++;
return OK;
}//EnCyQueue
Status DeCyQueue(CyQueue &Q,int &x)//带 length 域的循环队列出队算法
{
if(Q.length==0) return INFEASIBLE;
head=(Q.rear-Q.length+1)%MAXSIZE; //详见书后注释
x=Q.base[head];
Q.length--;
}//DeCyQueue
3.31
int Palindrome_Test()//判别输入的字符串是否回文序列,是则返回 1,否则返回 0
{
InitStack(S);InitQueue(Q);
while((c=getchar()!='@')
{
Push(S,c);EnQueue(Q,c); //同时使用栈和队列两种结构
}
while(!StackEmpty(S))
{