没有合适的资源?快使用搜索试试~ 我知道了~
首页WebGL入门指南:浏览器内的3D图形革命
WebGL编程指南是一份全面的教程,专为那些想要在网页浏览器中实现高级3D图形交互的开发者而设计。它突破了传统上对3D图形技术的限制,这些技术以往主要局限于高端计算机和游戏机,需要复杂的编程技能。随着个人电脑和特别是Web浏览器性能的提升,WebGL将OpenGL ES和HTML5与JavaScript相结合,使得开发人员能够轻松创建并展示三维图形,进而推动了下一代用户界面和网络内容的易用性和直观性。
该指南深入讲解了如何利用WebGL进行JavaScript编程、OpenGLES(OpenGL的轻量级版本)以及基本的图形技术,为开发者提供了一个全面的入门教程。随着HTML5的发展,Web成为了一个支持丰富、吸引人且互动性强的应用平台,跨多种系统的兼容性使其愈发重要。WebGL作为HTML5的核心组成部分,使得网络程序员能够访问最新3D图形加速技术的全部力量和功能,实现了安全运行在任何具备网络能力的系统上。
WebGL的出现预示着一个创新的浪潮,开发者们可以利用这一技术在从标准PC到消费电子、智能手机和平板电脑等多种设备上开发出具有桌面应用体验的在线应用。《WebGL编程指南》因其详尽的内容和前瞻性,被业内专家如戴夫·施雷纳尔,OpenGL编程指南第八版的合著者以及OpenGL库系列编辑,高度评价,认为它引领了创建这种未来趋势应用的潮流,使读者能够站在技术发展的前沿。
通过学习这本书,开发者不仅能掌握WebGL的基本原理和实践,还能了解到如何利用这项技术来构建高度沉浸式和交互式的网络应用,从而在这个快速发展的领域保持竞争力。随着WebGL的广泛应用,我们期待看到更多创新的3D Web内容和应用程序的诞生,进一步丰富和扩展了互联网的功能。
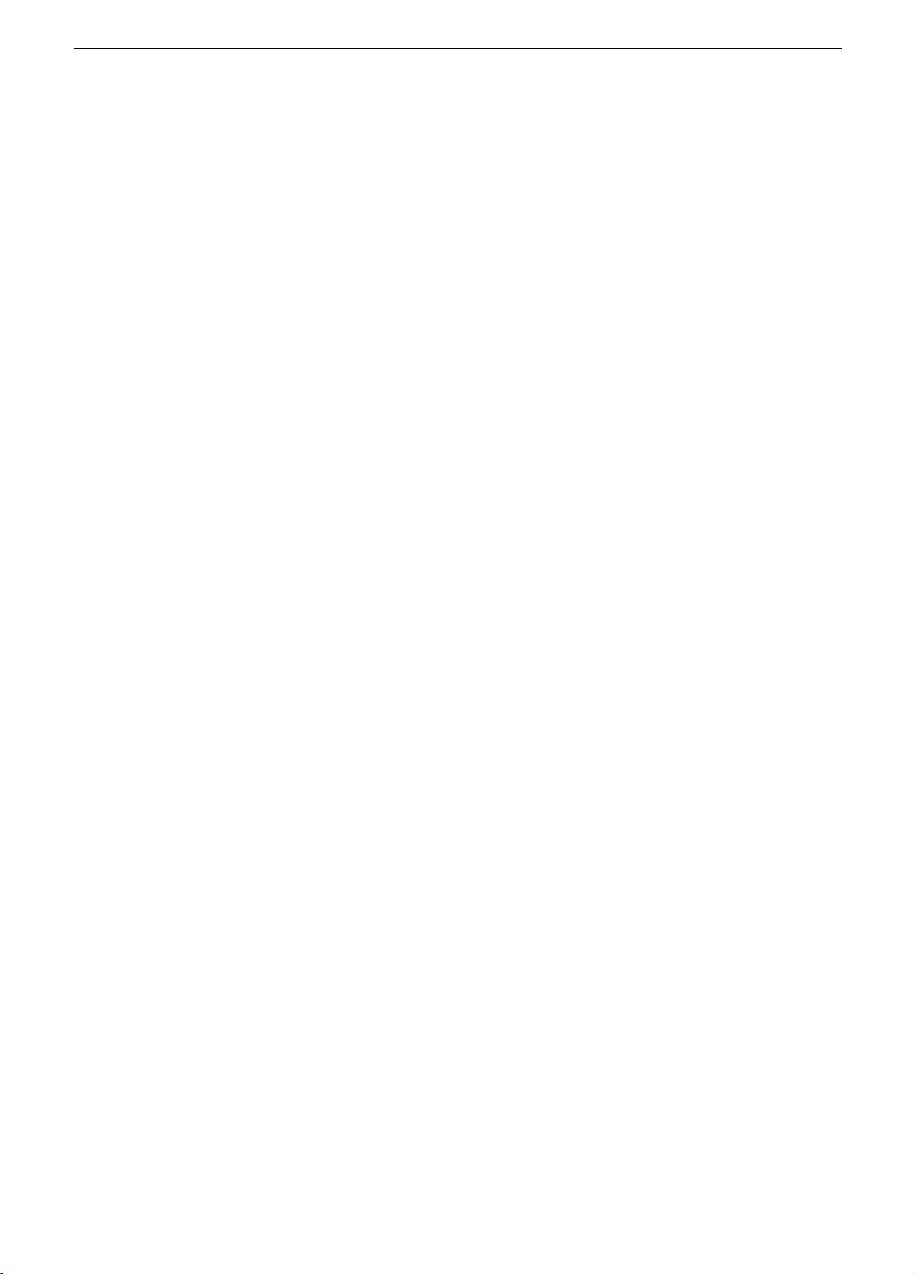
ptg11539634
Contents
xv
Switching Shaders ....................................................................................................... 386
How to Implement Switching Shaders ..................................................................387
Sample Program (ProgramObject.js) ......................................................................387
Use What You’ve Drawn as a Texture Image .............................................................392
Framebuffer Object and Renderbuffer Object .......................................................392
How to Implement Using a Drawn Object as a Texture .......................................394
Sample Program (FramebufferObjectj.js) ...............................................................395
Create Frame Buffer Object (gl.createFramebuffer()) .............................................397
Create Texture Object and Set Its Size and Parameters .........................................397
Create Renderbuffer Object (gl.createRenderbuffer()) ...........................................398
Bind Renderbuffer Object to Target and Set Size (gl.bindRenderbuffer(),
gl.renderbufferStorage()) ........................................................................................399
Set Texture Object to Framebuffer Object (gl.bindFramebuffer(),
gl.framebufferTexture2D()) ....................................................................................400
Set Renderbuffer Object to Framebuffer Object
(gl.framebufferRenderbuffer()) ...............................................................................401
Check Configuration of Framebuffer Object (gl.checkFramebufferStatus()) ........402
Draw Using the Framebuffer Object ......................................................................403
Display Shadows .........................................................................................................405
How to Implement Shadows ................................................................................. 405
Sample Program (Shadow.js) ..................................................................................406
Increasing Precision ...............................................................................................412
Sample Program (Shadow_highp.js) ......................................................................413
Load and Display 3D Models .....................................................................................414
The OBJ File Format ...............................................................................................417
The MTL File Format ..............................................................................................418
Sample Program (OBJViewer.js) .............................................................................419
User-Defined Object ...............................................................................................422
Sample Program (Parser Code in OBJViewer.js) ....................................................423
Handling Lost Context ............................................................................................... 430
How to Implement Handling Lost Context ..........................................................431
Sample Program (RotatingTriangle_contextLost.js) ..............................................432
Summary ....................................................................................................................434
A. No Need to Swap Buffers in WebGL 437
B. Built-in Functions of GLSL ES 1.0 441
Angle and Trigonometry Functions ...........................................................................441
Exponential Functions ................................................................................................443
Common Functions ....................................................................................................444
Geometric Functions ..................................................................................................447
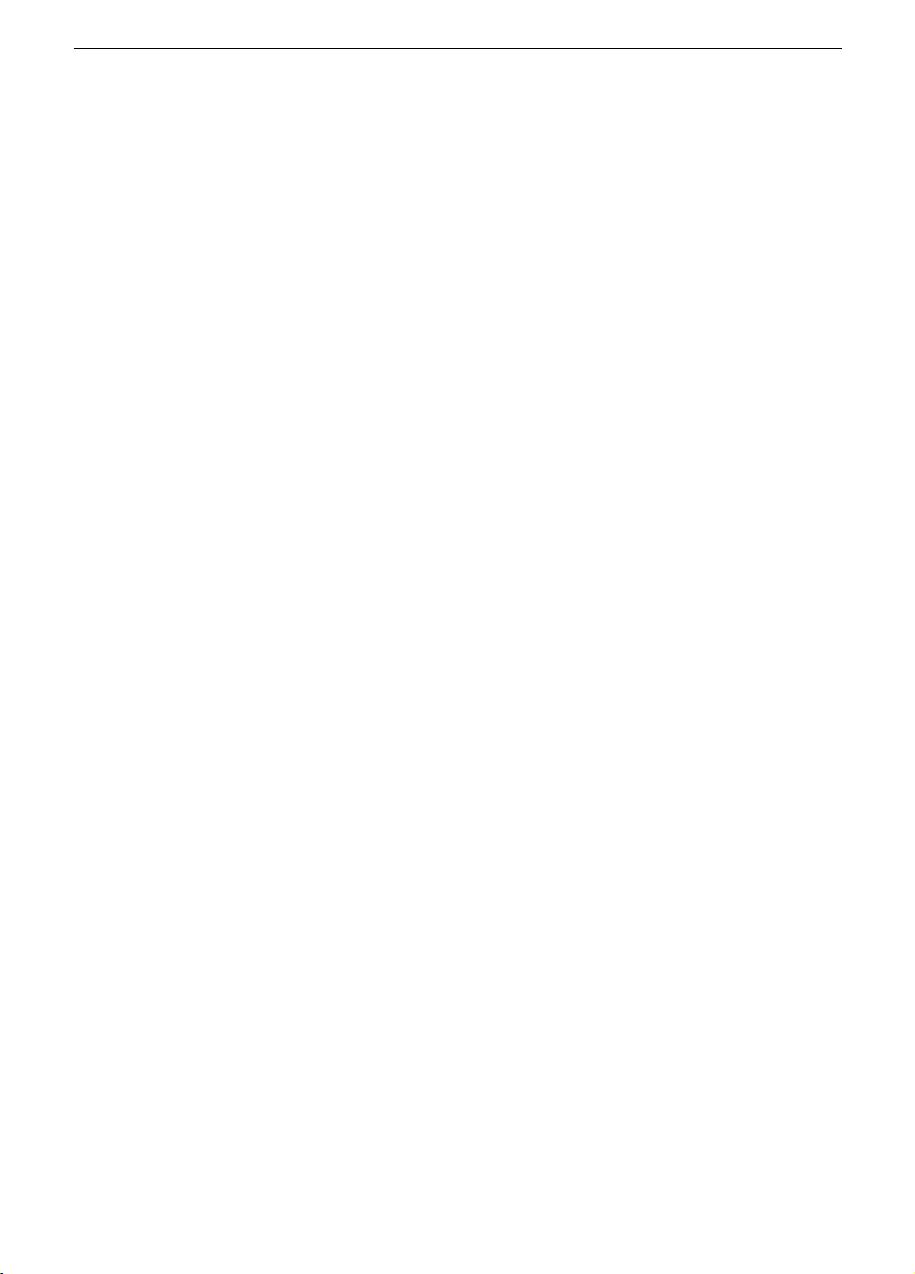
ptg11539634
WebGL Programming Guide
xvi
Matrix Functions .........................................................................................................448
Vector Functions .........................................................................................................449
Texture Lookup Functions ..........................................................................................451
C. Projection Matrices 453
Orthogonal Projection Matrix ....................................................................................453
Perspective Projection Matrix ..................................................................................... 453
D. WebGL/OpenGL: Left or Right Handed? 455
Sample Program CoordinateSystem.js ........................................................................456
Hidden Surface Removal and the Clip Coordinate System .......................................459
The Clip Coordinate System and the Viewing Volume.............................................460
What Is Correct? .........................................................................................................462
Summary ....................................................................................................................464
E. The Inverse Transpose Matrix 465
F. Load Shader Programs from Files 471
G. World Coordinate System Versus Local Coordinate System 473
The Local Coordinate System .....................................................................................474
The World Coordinate System ...................................................................................475
Transformations and the Coordinate Systems ...........................................................477
H. Web Browser Settings for WebGL 479
Glossary 481
References 485
Index 487
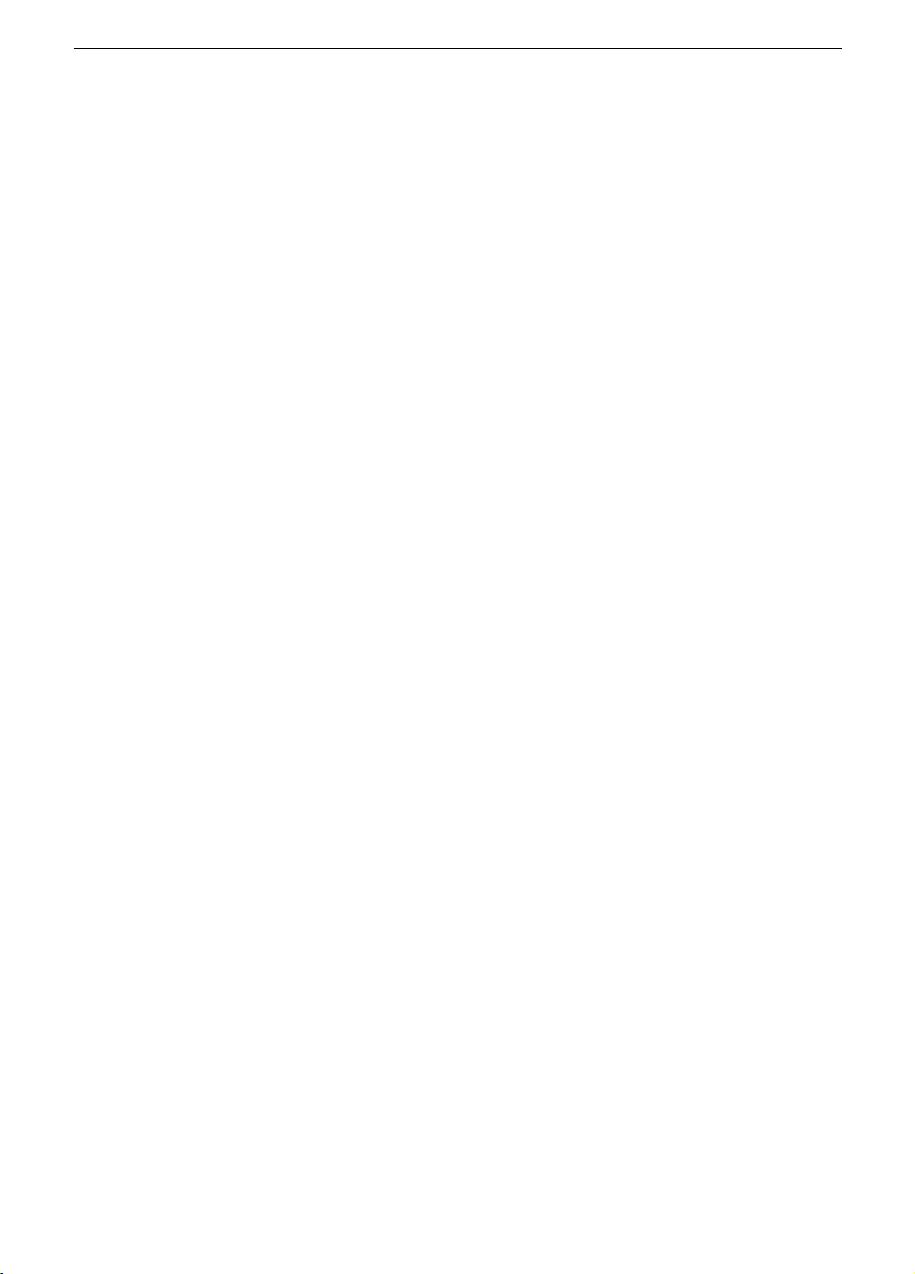
ptg11539634
xvii
Preface
Preface
WebGL is a technology that enables drawing, displaying, and interacting with sophis-
ticated interactive three-dimensional computer graphics (“3D graphics”) from within
web browsers. Traditionally, 3D graphics has been restricted to high-end computers or
dedicated game consoles and required complex programming. However, as both personal
computers and, more importantly, web browsers have become more sophisticated, it has
become possible to create and display 3D graphics using accessible and well-known web
technologies. This book provides a comprehensive overview of WebGL and takes the
reader, step by step, through the basics of creating WebGL applications. Unlike other
3D graphics technologies such as OpenGL and Direct3D, WebGL applications can be
constructed as web pages so they can be directly executed in the browsers without install-
ing any special plug-ins or libraries. Therefore, you can quickly develop and try out a
sample program with a standard PC environment; because everything is web based, you
can easily publish the programs you have constructed on the web. One of the promises
of WebGL is that, because WebGL applications are constructed as web pages, the same
program can be run across a range of devices, such as smart phones, tablets, and game
consoles, through the browser. This powerful model means that WebGL will have a signif-
icant impact on the developer community and will become one of the preferred tools for
graphics programming.
Who the Book Is For
We had two main audiences in mind when we wrote this book: web developers looking
to add 3D graphics to their web pages and applications, and 3D graphics programmers
wishing to understand how to apply their knowledge to the web environment. For web
developers who are familiar with standard web technologies such as HTML and JavaScript
and who are looking to incorporate 3D graphics into their web pages or web applica-
tions, WebGL offers a simple yet powerful solution. It can be used to add 3D graphics to
enhance web pages, to improve the user interface (UI) for a web application by using a 3D
interface, and even to develop more complex 3D applications and games that run in web
browsers.
The second target audience is programmers who have worked with one of the main 3D
application programming interfaces (APIs), such as Direct3D or OpenGL, and who are
interested in understanding how to apply their knowledge to the web environment. We
would expect these programmers to be interested in the more complex 3D applications
that can be developed in modern web browsers.
However, the book has been designed to be accessible to a wide audience using a step-by-
step approach to introduce features of WebGL, and it assumes no background in 2D or 3D
graphics. As such, we expect it also to be of interest to the following:
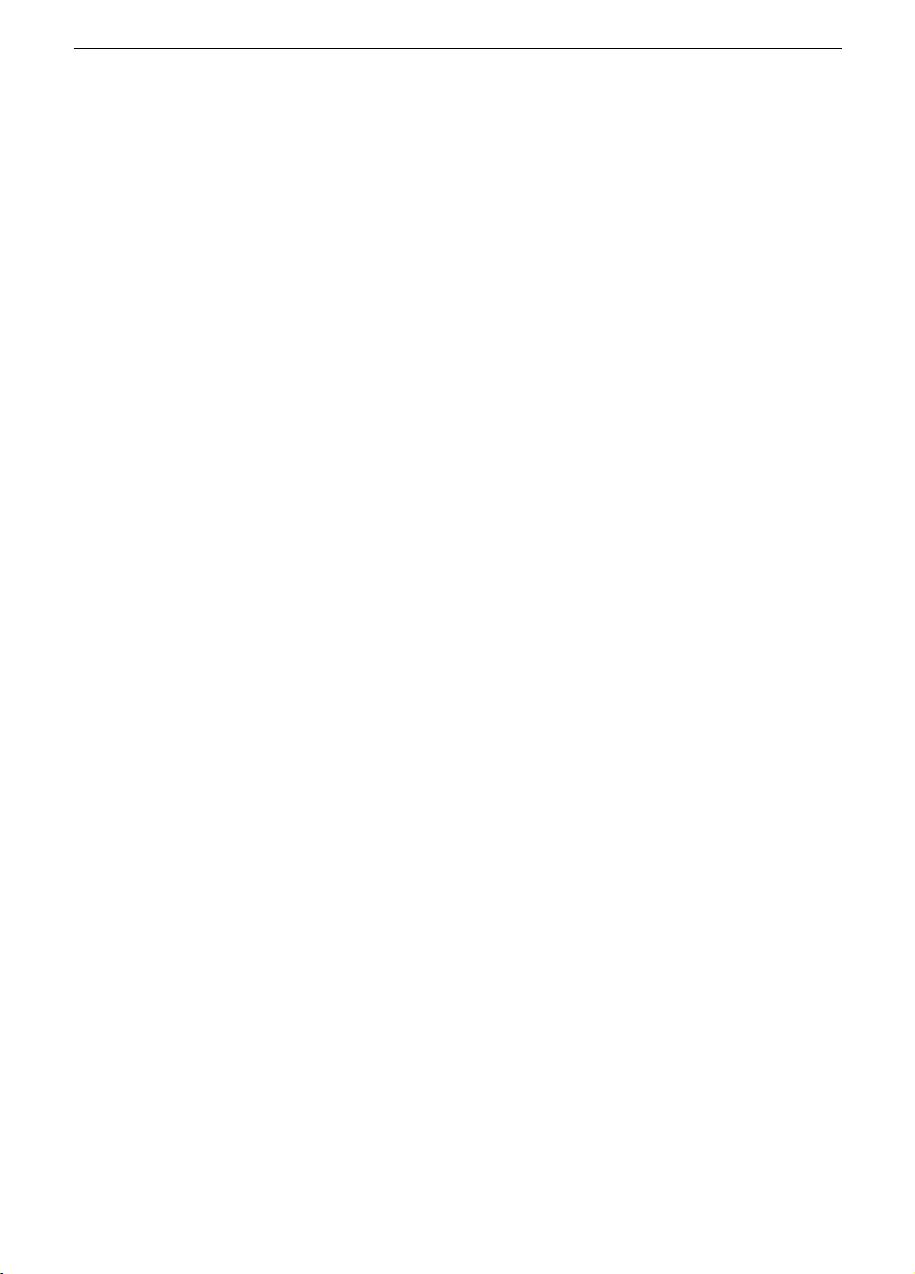
ptg11539634
WebGL Programming Guide
xviii
• General programmers seeking an understanding of how web technologies are evolv-
ing in the graphics area
• Students studying 2D and 3D graphics because it offers a simple way to begin to ex-
periment with graphics via a web browser rather than setting up a full programming
environment
• Web developers exploring the “bleeding edge” of what is possible on mobile devices
such as Android or iPhone using the latest mobile web browsers
What the Book Covers
This book covers the WebGL 1.0 API along with all related JavaScript functions. You will
learn how HTML, JavaScript, and WebGL are related, how to set up and run WebGL appli-
cations, and how to incorporate sophisticated 3D program “shaders” under the control
of JavaScript. The book details how to write vertex and fragment shaders, how to imple-
ment advanced rendering techniques such as per-pixel lighting and shadowing, and basic
interaction techniques such as selecting 3D objects. Each chapter develops a number of
working, fully functional WebGL applications and explains key WebGL features through
these examples. After finishing the book, you will be ready to write WebGL applications
that fully harness the programmable power of web browsers and the underlying graphics
hardware.
How the Book Is Structured
This book is organized to cover the API and related web APIs in a step-by-step fashion,
building up your knowledge of WebGL as you go.
Chapter 1—Overview of WebGL
This chapter briefly introduces you to WebGL, outlines some of the key features and
advantages of WebGL, and discusses its origins. It finishes by explaining the relationship
of WebGL to HTML5 and JavaScript and which web browsers you can use to get started
with your exploration of WebGL.
Chapter 2—Your First Step with WebGL
This chapter explains the <canvas> element and the core functions of WebGL by taking
you, step-by-step, through the construction of several example programs. Each example
is written in JavaScript and uses WebGL to display and interact with a simple shape on
a web page. The example WebGL programs will highlight some key points, including:
(1) how WebGL uses the
<canvas> element object and how to draw on it; (2) the linkage
between HTML and WebGL using JavaScript; (3) simple WebGL drawing functions; and
(4) the role of shader programs within WebGL.
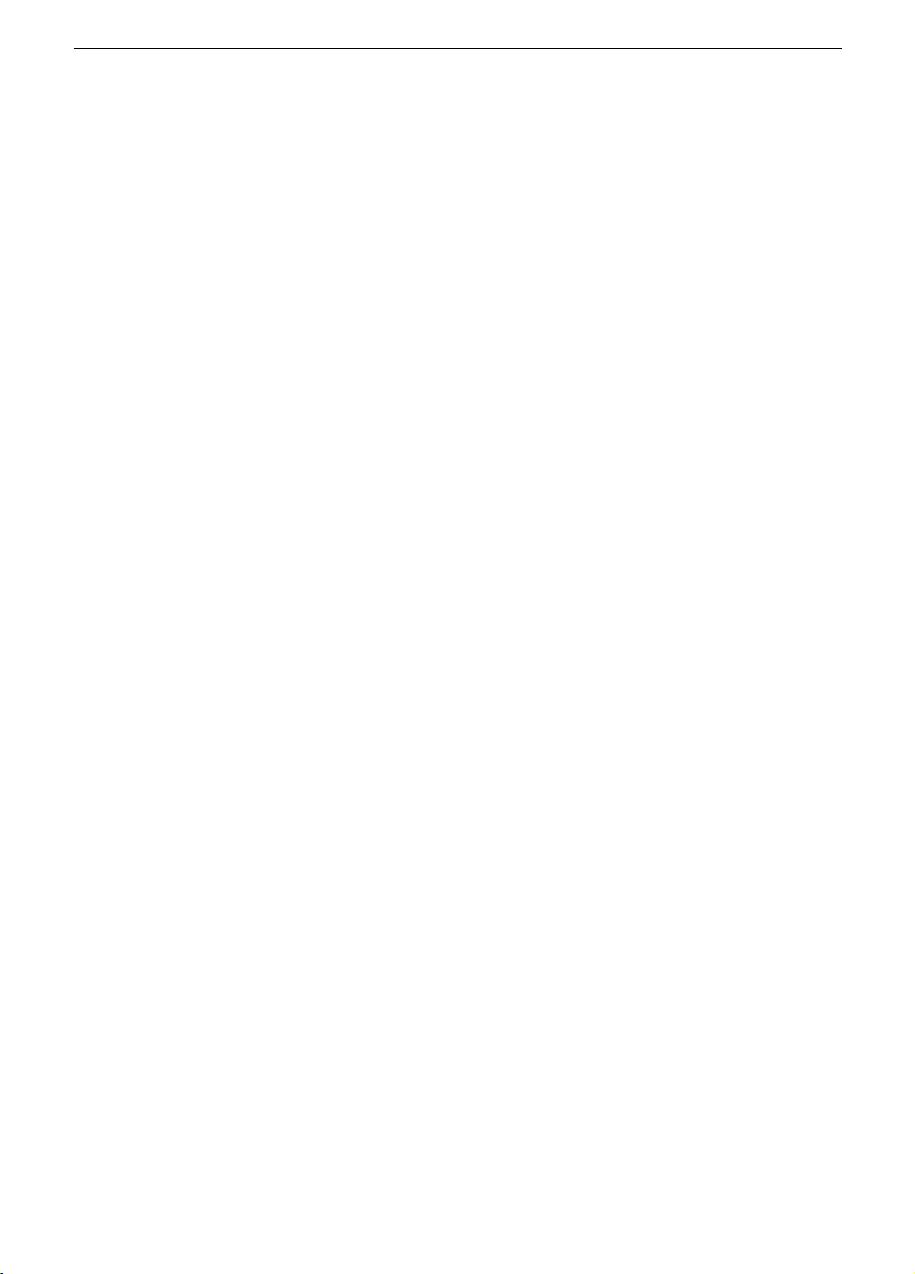
ptg11539634
xix
Preface
Chapter 3—Drawing and Transforming Triangles
This chapter builds on those basics by exploring how to draw more complex shapes and
how to manipulate those shapes in 3D space. This chapter looks at: (1) the critical role of
triangles in 3D graphics and WebGL’s support for drawing triangles; (2) using multiple
triangles to draw other basic shapes; (3) basic transformations that move, rotate, and
scale triangles using simple equations; and (4) how matrix operations make transforma-
tions simple.
Chapter 4—More Transformations and Basic Animation
In this chapter, you explore further transformations and begin to combine transformations
into animations. You: (1) are introduced to a matrix transformation library that hides the
mathematical details of matrix operations; (2) use the library to quickly and easily combine
multiple transformations; and (3) explore animation and how the library helps you
animate simple shapes. These techniques provide the basics to construct quite complex
WebGL programs and will be used in the sample programs in the following chapters.
Chapter 5—Using Colors and Texture Images
Building on the basics described in previous chapters, you now delve a little further into
WebGL by exploring the following three subjects: (1) besides passing vertex coordinates,
how to pass other data such as color information to the vertex shader; (2) the conver-
sion from a shape to fragments that takes place between the vertex shader and the frag-
ment shader, which is known as the rasterization process; and (3) how to map images (or
textures) onto the surfaces of a shape or object. This chapter is the final chapter focusing
on the key functionalities of WebGL.
Chapter 6—The OpenGL ES Shading Language (GLSL ES)
This chapter takes a break from examining WebGL sample programs and explains the core
features of the OpenGL ES Shading Language (GLSL ES) in detail. You will cover: (1) data,
variables, and variable types; (2) vector, matrix, structure, array, and sampler; (3) opera-
tors, control flow, and functions; (4) attributes, uniforms, and varyings; (5) precision
qualifier; and (6) preprocessor and directives. By the end of this chapter you will have a
good understanding of GLSL ES and how it can be used to write a variety of shaders.
Chapter 7—Toward the 3D World
This chapter takes the first step into the 3D world and explores the implications of
moving from 2D to 3D. In particular, you will explore: (1) representing the user’s view
into the 3D world; (2) how to control the volume of 3D space that is viewed; (3) clipping;
(4) foreground and background objects; and (5) drawing a 3D object—a cube. All these
issues have a significant impact on how the 3D scene is drawn and presented to viewers.
A mastery of them is critical to building compelling 3D scenes.
www.allitebooks.com
剩余543页未读,继续阅读

虾球xz
- 粉丝: 1199
- 资源: 104
上传资源 快速赚钱
我的内容管理 展开
我的资源 快来上传第一个资源
我的收益
登录查看自己的收益我的积分 登录查看自己的积分
我的C币 登录后查看C币余额
我的收藏
我的下载
下载帮助

最新资源
- PortafolioAdsi:工业生物技术中心 ADSI 案例研究项目 - Palmira。 软件开发的整个过程将展示实施 Scrum 框架,以同样的方式利用 JAVA、JPA、Mysql、Html5、CSS 等技术
- ISO15118是欧洲的电动汽车充电协议标准,这是第一部分,通用信息及用例定义
- 测试
- teamtool-spring:团队工具(Spring MVC)
- Learners-Academy
- 为桌面和Web应用程序配置Log4Net
- be-kanBAO:后端做看报
- react-redux-flask-mongodb:带有Mongodb的Flask JWT后端和带有Material UI的ReactRedux前端的入门应用程序
- 新的多站点DLL或如何在根目录中开发.NET项目
- fakhrusy.com:我的个人网站
- image-mosaic
- pyg_lib-0.3.0+pt20-cp310-cp310-macosx_11_0_x86_64whl.zip
- N10SG开发教学视频.zip
- Toolint-tests-Empty-TC-Add-Tools-2021-04-07T15-40-16.889Z:为工具链创建
- 122页中国移动互联网2019半年大报告-QuestMobile-2019.7.rar
- practice:练习
安全验证
文档复制为VIP权益,开通VIP直接复制
