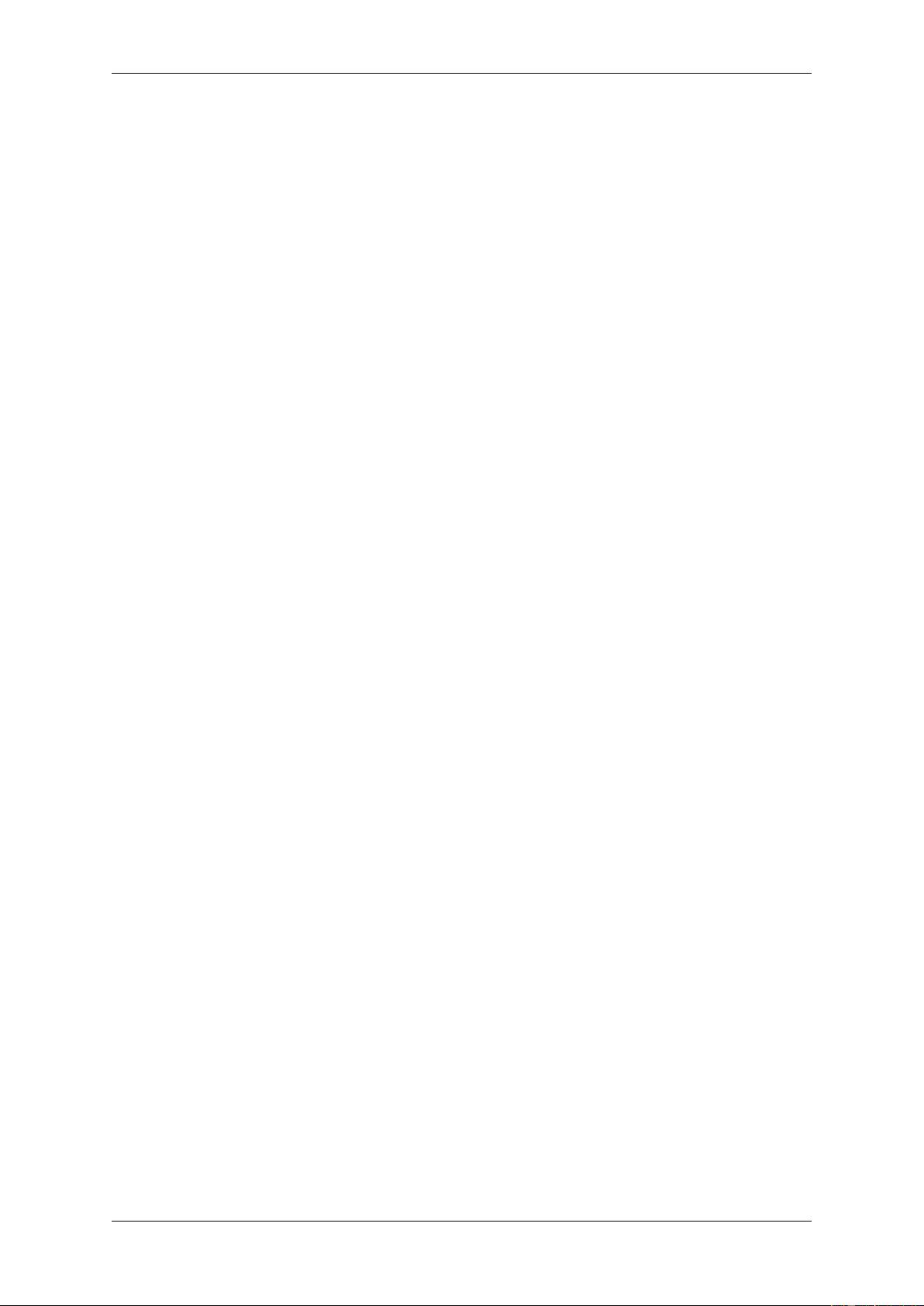
The Python Language Reference, Release 2.6.2
numbers.Real (float) These represent machine-level double precision floating point numbers. You
are at the mercy of the underlying machine architecture (and C or Java implementation) for the ac-
cepted range and handling of overflow. Python does not support single-precision floating point num-
bers; the savings in processor and memory usage that are usually the reason for using these is dwarfed
by the overhead of using objects in Python, so there is no reason to complicate the language with two
kinds of floating point numbers.
numbers.Complex These represent complex numbers as a pair of machine-level double precision float-
ing point numbers. The same caveats apply as for floating point numbers. The real and imaginary
parts of a complex number z can be retrieved through the read-only attributes z.real and z.imag.
Sequences These represent finite ordered sets indexed by non-negative numbers. The built-in function len()
returns the number of items of a sequence. When the length of a sequence is n, the index set contains the
numbers 0, 1, ..., n-1. Item i of sequence a is selected by a[i]. Sequences also support slicing: a[i:j]
selects all items with index k such that i <= k < j. When used as an expression, a slice is a sequence of the
same type. This implies that the index set is renumbered so that it starts at 0. Some sequences also support
“extended slicing” with a third “step” parameter: a[i:j:k] selects all items of a with index x where x =
i + n*k, n >= 0 and i <= x < j.
Sequences are distinguished according to their mutability:
Immutable sequences An object of an immutable sequence type cannot change once it is created. (If the
object contains references to other objects, these other objects may be mutable and may be changed;
however, the collection of objects directly referenced by an immutable object cannot change.)
The following types are immutable sequences:
Strings The items of a string are characters. There is no separate character type; a character is repre-
sented by a string of one item. Characters represent (at least) 8-bit bytes. The built-in functions
chr() and ord() convert between characters and nonnegative integers representing the byte
values. Bytes with the values 0-127 usually represent the corresponding ASCII values, but the
interpretation of values is up to the program. The string data type is also used to represent ar-
rays of bytes, e.g., to hold data read from a file. (On systems whose native character set is not
ASCII, strings may use EBCDIC in their internal representation, provided the functions chr()
and ord() implement a mapping between ASCII and EBCDIC, and string comparison preserves
the ASCII order. Or perhaps someone can propose a better rule?)
Unicode The items of a Unicode object are Unicode code units. A Unicode code unit is represented by
a Unicode object of one item and can hold either a 16-bit or 32-bit value representing a Unicode
ordinal (the maximum value for the ordinal is given in sys.maxunicode, and depends on how
Python is configured at compile time). Surrogate pairs may be present in the Unicode object, and
will be reported as two separate items. The built-in functions unichr() and ord() convert
between code units and nonnegative integers representing the Unicode ordinals as defined in the
Unicode Standard 3.0. Conversion from and to other encodings are possible through the Unicode
method encode() and the built-in function unicode().
Tuples The items of a tuple are arbitrary Python objects. Tuples of two or more items are formed by
comma-separated lists of expressions. A tuple of one item (a ‘singleton’) can be formed by affix-
ing a comma to an expression (an expression by itself does not create a tuple, since parentheses
must be usable for grouping of expressions). An empty tuple can be formed by an empty pair of
parentheses.
Mutable sequences Mutable sequences can be changed after they are created. The subscription and slicing
notations can be used as the target of assignment and del (delete) statements.
There is currently a single intrinsic mutable sequence type:
Lists The items of a list are arbitrary Python objects. Lists are formed by placing a comma-separated
list of expressions in square brackets. (Note that there are no special cases needed to form lists of
length 0 or 1.)
The extension module array provides an additional example of a mutable sequence type.
Set types These represent unordered, finite sets of unique, immutable objects. As such, they cannot be indexed
by any subscript. However, they can be iterated over, and the built-in function len() returns the number
3.2. The standard type hierarchy 15