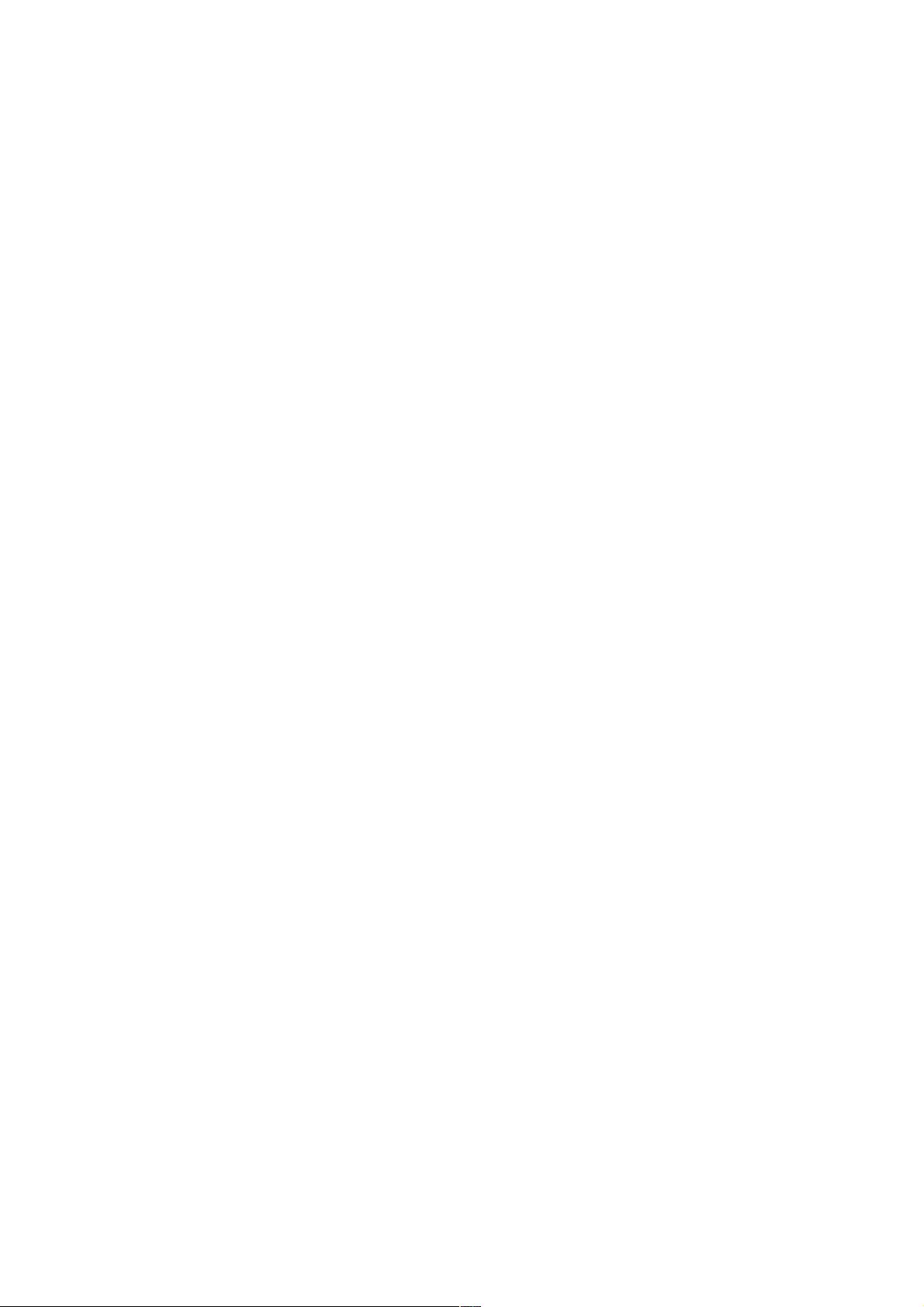
Listing I - Automatic management of Instance life-cycle.
3.1.3. Explicit Life-Cycle Management
Topic-instances life-cycle can also be managed explicitly via the API defined on
the DataWriter. In this case the application programmer has the control on
when instances are registered, unregistered and disposed. Topic-instances
registration is a good practice to follow whenever an applications writes an
instance very often and requires the lowest latency write. In essence the act of
explicitly registering an instance allows the middleware to reserve resources as
well as optimize the instance lookup. Topic-instance unregistration provides a
mean for telling DDS that an application is done with writing a specific topic-
instance, thus all the resources locally associated with is can be safely released.
Finally, disposing topic-instances gives a way of communicating DDS that the
instance is no more relevant for the distributed system, thus whenever possible
resources allocated with the specific instances should be released both locally
and remotely.
Listing 2 shows and example of how the DataWriter API can be used to
register, unregister and dispose topic-instances. In order to show you
the full life-cycle management I’ve changed the default DataWriter behavior
to avoid that instances are automatically disposed when unregistered. In
addition, to maintain the code compact I take advantage of the new C++0x
auto feature which leaves it to the the compiler to infer the left hand side
types from the right hand side return-type. Listing 2 shows an application that
writes four samples belonging to four different topic-instances, respectively
those with id=0,1,2,3. The instances with id=1,2,3 are explicitly registered by
calling the DataWriter::register_instance method, while the instance
with id=0 is automatically registered as result of the write on the DataWriter.
To show the different possible state transitions, the topic-instance with id=1 is
explicitly unregistered thus causing it to transition to the
NOT_ALIVE_NO_WRITER state; the topic-instance with id=2 is explicitly
disposed thus causing it to transition to the NOT_ALIVE_DISPOSED state.
Finally, the topic-instance with id=0,3 will be automatically unregistered, as a
result of the destruction of the objects dw and dwi3 respectively, thus
transitioning to the state NOT_ALIVE_NO_WRITER. Once again, as mentioned
above, in this example the writer has been configured to ensure that topic-
instances are not automatically disposed upon unregistration.
3.1.4. Keyless Topics
Most of the discussion above has focused on keyed topics, but what about
keyless topics? As explained in the last installment of the series, keyless topics
are like singletons, in the sense that there is only one instance. As a result for
keyless topics the the state transitions are tied to the lifecycle of the data-writer.
int main(int, char**) {
dds::Topic<TempSensorType> tsTopic(“TempSensorTopic”);
dds::DataWriter<TempSensorType> dw(tsTopic);
TempSensorType ts;
//[NOTE #1]: Instances implicitly registered as part
// of the write.
// {id, temp hum scale};
ts = {1, 25.0F, 65.0F, CELSIUS};
dw.write(ts);
ts = {2, 26.0F, 70.0F, CELSIUS};
dw.write(ts);
ts = {3, 27.0F, 75.0F, CELSIUS};
dw.write(ts);
sleep(10);
//[NOTE #2]: Instances automatically unregistered and
// disposed as result of the destruction of the dw object
return 0;
}