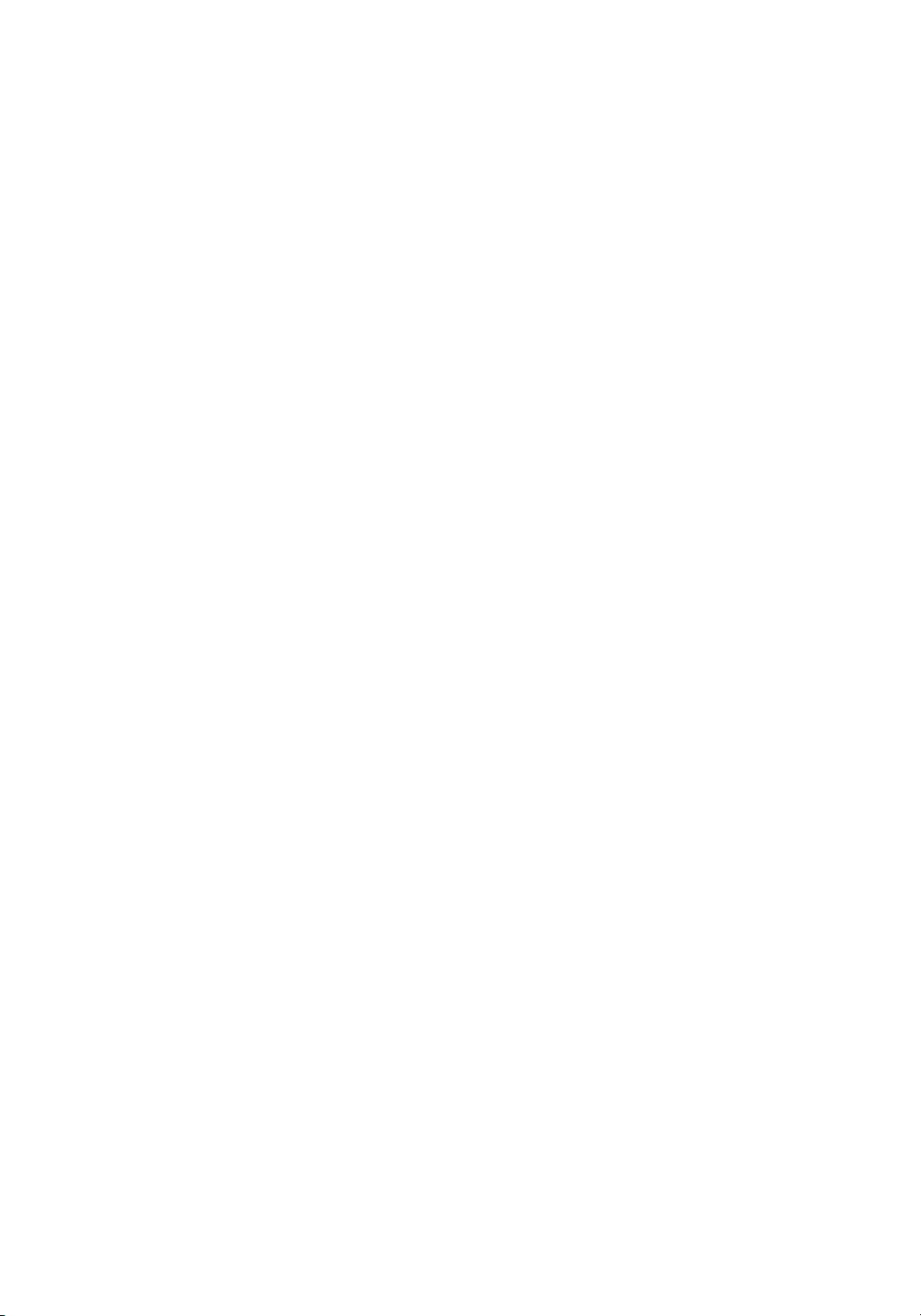
Chapter 1 ■ GoinG inside Java
14
The Java Programming Language and APIs
All that we’ve covered so far happens transparently from the perspective of an application developer. In fact,
you don’t really have to know any details of Java’s internal architecture to program in Java. However, what
you do need to know is how to use Java as a programming language and also how to use the various APIs that
come with the different platforms to communicate with the underlying software and operating system. In
fact, this is essentially what the remainder of the book will be about—how to develop effectively with Java.
The Java Programming Language
Although knowledge of the various APIs is essential to achieving anything with Java, a solid foundation in
the core Java language is also highly desirable to make the most effective use of the APIs. In this book, you’ll
explore the following features of core Java programming:
Method, interface, and class design: Writing the main building blocks of your
applications with Java objects can be simultaneously quite straightforward and very
complex. However, if you take the time to follow some basic guidelines for creating
methods, classes, and libraries, it’s not too difficult to develop classes that not only
provide the required functionality but are also reliable, maintainable, and reusable.
Threading: Java includes built-in support for multithreaded applications, and
you’ll often find it necessary or desirable to take advantage of this. To do so,
you should be familiar with Java’s multithreading capabilities and know how to
implement threads correctly within an application.
The Java APIs
As discussed earlier in the chapter, three major versions of the Java platform exist, and each consists of some
significantly different APIs. In this book, we’ll concentrate on some (although by no means all) of the APIs
that form the Standard Edition. More specifically, we’ll cover the following:
User interface components: We’ll take an in-depth approach to show some
of the more complex user interface components; you’ll also learn how to use a
layout manager to arrange components within an interface.
The data transfer API: Closely related to providing the user interface for your
application is the need to provide cut-and-paste and drag-and-drop capabilities.
The printing API: Another common feature often required is the ability to print,
which you’ll examine through the use of Java’s printing capabilities.
JDBC: All but the most trivial of applications require data to be loaded,
manipulated, and stored in some form or another, and a relational database is
the most common means for storing such data. The Java Database Connectivity
(JDBC) API is provided for that purpose; we’ll discuss it in detail.
Internationalization: Most commercial applications and those developed for
internal use by large organizations are used in more than one country and need to
support more than one language. This requirement is sometimes overlooked and
treated as an implementation detail, but to be done successfully, internationalization
should be considered as part of an application’s design. To create a successful
design that includes internationalization support, you should be familiar with Java’s
capabilities in that area, and we’ll discuss them in detail in this book.