C++程序设计-有参函数解析
需积分: 32 56 浏览量
更新于2024-08-19
收藏 8.81MB PPT 举报
"有参函数是C++编程中一个重要的概念,它允许主调函数向被调函数传递数据,同时被调函数也能将结果返回给主调函数。这种数据传递方式是程序设计中实现功能模块化和代码复用的关键。在C++中,函数的声明通常包括类型说明、函数名和形式参数列表。形式参数列表是在函数调用时用来传递实际参数的位置,它们在函数定义中只起到占位符的作用。
C++的发展历程是这样的:从最初的BCPL和B语言,经过Dennis Ritchie和Brian Kernighan的改进,诞生了C语言。C语言因其结构化特性、高效执行和良好的可移植性而广受欢迎。C++是在C语言的基础上添加了面向对象的特性,如类、对象、继承、多态等,使得程序设计更加灵活和强大。
C++语言的特点包括:
1. 结构化编程:C++支持结构化编程,允许程序员将复杂的问题分解成独立的函数,提高了代码的可读性和维护性。
2. 高级语言和汇编语言特性结合:C++拥有丰富的运算符,包括算术逻辑运算和位运算,既能进行复杂的逻辑控制,又能直接操作硬件。
3. 可移植性:C++编写的程序可以在不同的计算机平台之间轻松移植,无需或只需少量修改。
4. 自由度高的语法:C++的语法较为宽松,为熟练的程序员提供了设计高质量、通用程序的灵活性,但也增加了初学者的学习难度。
在学习有参函数时,需要注意以下几点:
- 参数类型:函数的形式参数和实际参数需要匹配,类型必须一致。
- 参数传递:C++支持值传递和引用传递。值传递会复制参数值,而引用传递则是传址,直接操作原始变量。
- 返回值:函数可以通过return语句返回结果,返回值类型需要在函数声明时指定。
- 函数重载:C++允许同一函数名但参数列表不同的函数存在,这是实现多态性的一种方式。
调试C++程序时,虽然相对困难,但理解语法规则、利用调试工具可以帮助找出程序错误。熟练掌握有参函数的概念和用法对于进行C++编程至关重要,尤其在游戏开发等需要高效计算和模块化设计的领域。"
2012-02-21 上传
2011-01-04 上传
2011-03-01 上传
2023-05-25 上传
2023-05-19 上传
2023-09-07 上传
2023-05-19 上传
2023-06-05 上传
2023-05-22 上传
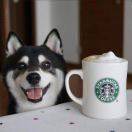
琳琅破碎
- 粉丝: 17
- 资源: 2万+
最新资源
- OptiX传输试题与SDH基础知识
- C++Builder函数详解与应用
- Linux shell (bash) 文件与字符串比较运算符详解
- Adam Gawne-Cain解读英文版WKT格式与常见投影标准
- dos命令详解:基础操作与网络测试必备
- Windows 蓝屏代码解析与处理指南
- PSoC CY8C24533在电动自行车控制器设计中的应用
- PHP整合FCKeditor网页编辑器教程
- Java Swing计算器源码示例:初学者入门教程
- Eclipse平台上的可视化开发:使用VEP与SWT
- 软件工程CASE工具实践指南
- AIX LVM详解:网络存储架构与管理
- 递归算法解析:文件系统、XML与树图
- 使用Struts2与MySQL构建Web登录验证教程
- PHP5 CLI模式:用PHP编写Shell脚本教程
- MyBatis与Spring完美整合:1.0.0-RC3详解