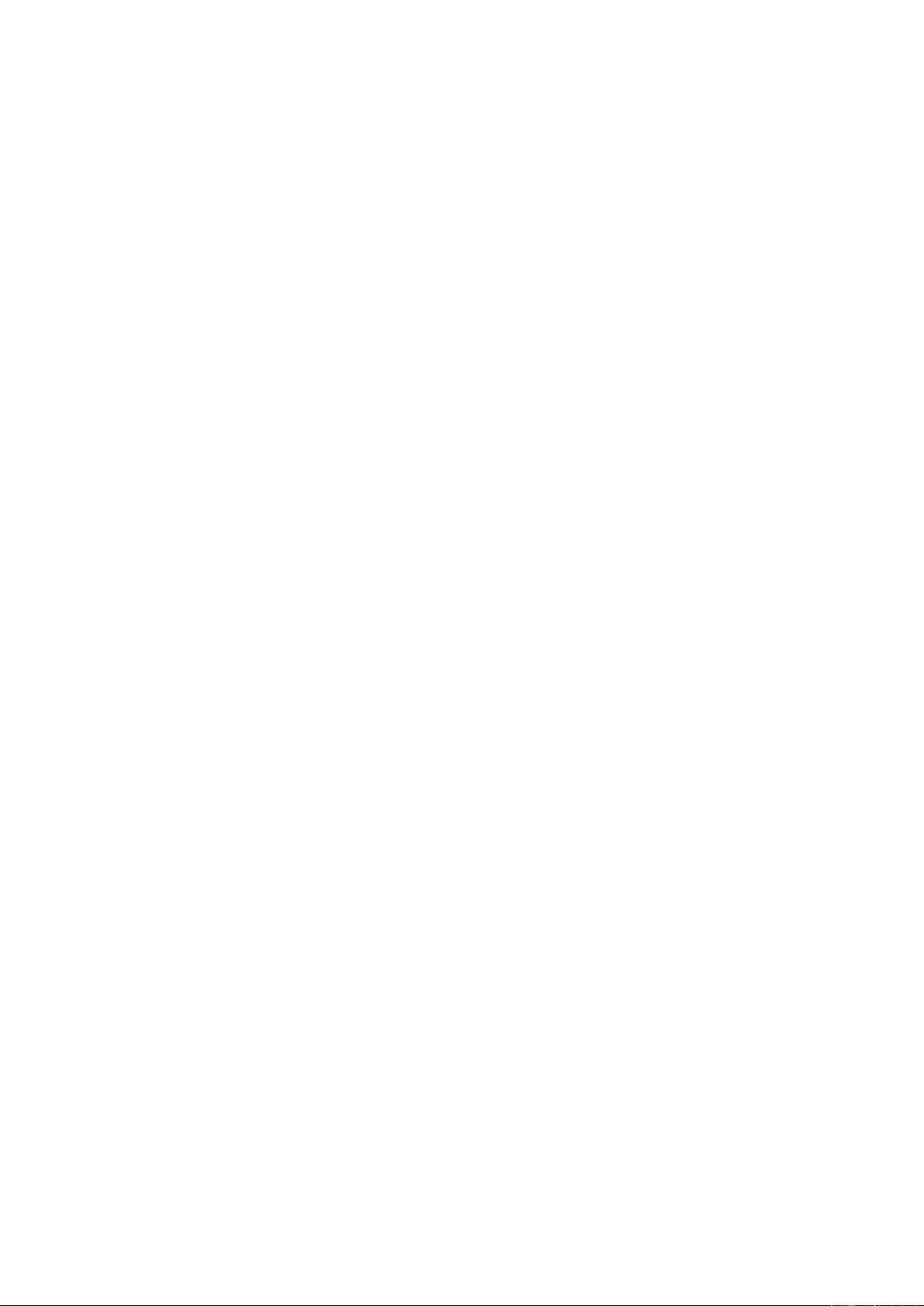
Organizing classes into hierarchies is a form of compression, taking the superclass and including it textually in all the subclasses.
As with all compression techniques, it leaves the code more difficult to read. You have to understand the context of the superclass
to be able to understand the subclass.
Using inheritance judiciously is another aspect of programming effectively with objects. Creating a subclass says, "I'm like that
superclass, only different." (Isn't it strange that we speak of overriding a method in a subclass? How much better programmers
would we be if we had thoughtfully selected our metaphors?)
Classes are relatively expensive design elements in programs built from objects. A class should do something significant.
Reducing the number of classes in a system is an improvement, as long as the remaining classes do not become bloated.
The patterns that follow explain how to communicate by declaring classes.
Simple Superclass Name
Finding just the right name is one of the most satisfying moments in programming. You're struggling with an idea. Oftentimes, the
code has gotten complicated and it doesn't seem like it needs to be. Then, often in conversation, someone says, "Oh, I see! This is
really a Scheduler." Everyone sits back and lets out a breath. The right name results in a cascade of further simplifications and
improvements.
Some of the most important names to choose well are those of classes. Classes are the central anchoring concept in the design.
Once classes have been named, the names of operations follow. It is rare for the reverse to be true, except if the class was poorly
named in the first place.
In naming classes there is a tension between brevity and expressiveness. You'll be using class names in conversation: "Did you
remember to rotate the Figure before translating it?" The names should be short and punchy. However, to make the names precise
sometimes seems to require several words.
A way out of this dilemma is picking a strong metaphor for the computation. With a metaphor in mind, even single words bring
with them a rich web of associations, connections, and implications. For example, in the HotDraw drawing framework, my first
name for an object in a drawing was DrawingObject. Ward Cunningham came along with the typography metaphor: a drawing is
like a printed, laid-out page. Graphical items on a page are figures, so the class became Figure. In the context of the metaphor,
Figure is simultaneously shorter, richer, and more precise than DrawingObject.
Sometimes, good names take time to find. You may have code "done" and working for weeks, months, or (in one notable case for
me) years when you discover a better name for a class. Sometimes you need to push a little harder to find a name: pull out a
thesaurus, write down a list of the least-suitable names you can think of, take a walk. Sometimes you need to forge ahead with new
functionality, trusting time, frustration, and your subconscious to supply a better name.
Conversation is a tool that consistently helps me find better names. Explaining the purpose of an object to another person leads me
to look for rich and evocative images to describe it. These images can lead in turn to new names.
Look for one-word names for important classes.
Qualified Subclass Name
The names of subclasses have two jobs. They need to communicate what class they are like and how they are different. Again, the
balance to be struck is between length and expressiveness. Unlike the names at the roots of hierarchies, subclass names aren't used
nearly as often in conversation, so they can be expressive at the cost of being concise. Prepend one or more modifiers to the
superclass name to form a subclass name.
One exception to this rule is when subclassing is used strictly as an implementation sharing mechanism and the subclass is an
important concept in its own right. Give subclasses that serve as the roots of hierarchies their own simple names. For example,
HotDraw has a class Handle which presents figure-editing operations when a figure is selected. It is called, simply, Handle in
spite of extending Figure. There is a whole family of handles and they most appropriately have names like StretchyHandle and
TransparencyHandle. Because Handle is the root of its own hierarchy, it deserves a simple superclass name more than a
qualified subclass name.
Another wrinkle in subclass naming is multiple-level hierarchies. Multi-level hierarchies are usually delegation waiting to happen,
but while they exist they need good names. Rather than blindly prepend the modifiers to the immediate superclass, think about the