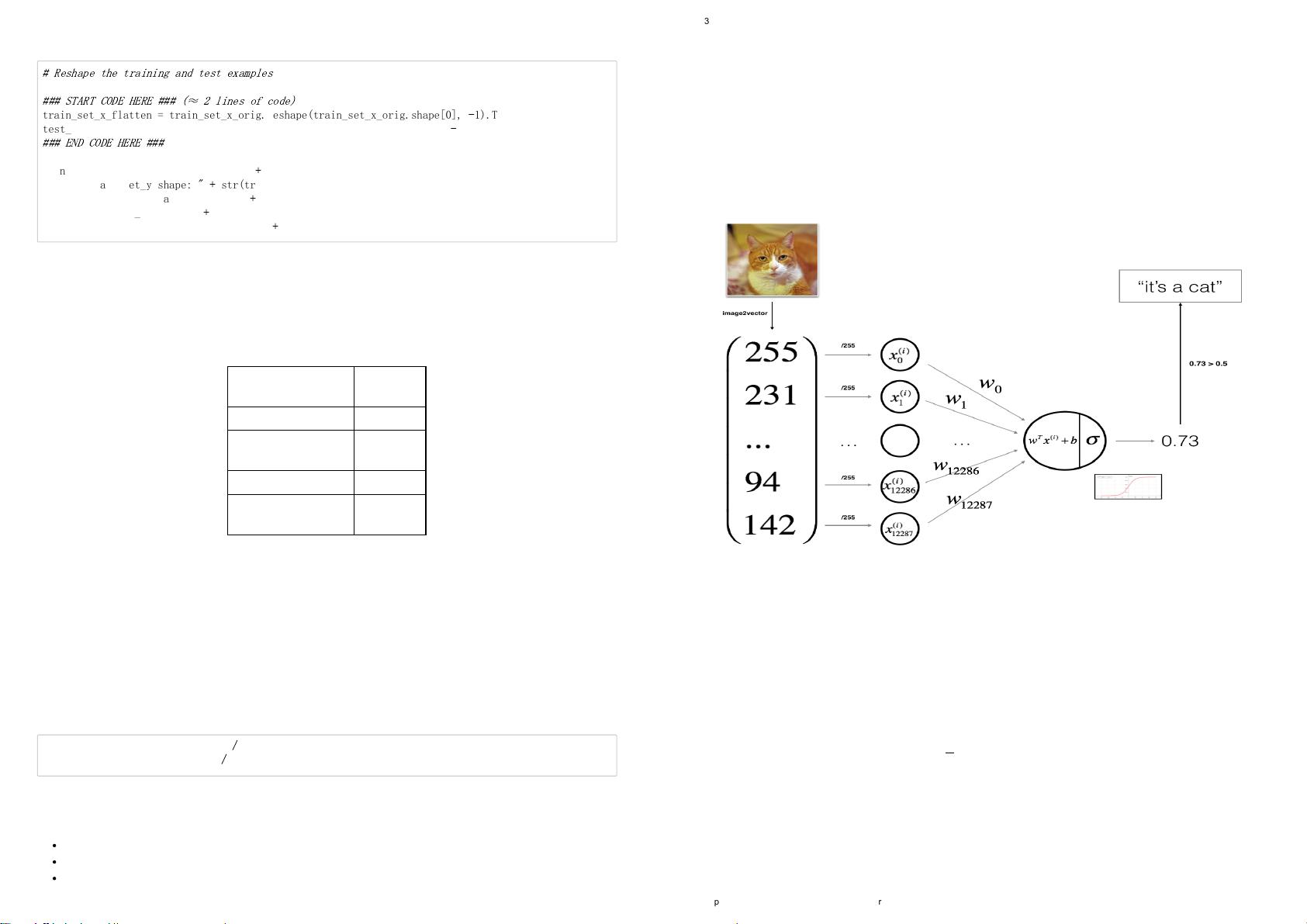
2019/3/29 Logistic Regression with a Neural Network mindset v4
file:///E:/python/Andrew Ng_DL/DeepLearning/Course1/Logistic Regression with a Neural Network mindset v4(week-2).htm 5/24
In[49]:
Expected Output:
train_set_x_flatten
shape
(12288,
209)
train_set_y shape (1, 209)
test_set_x_flatten
shape
(12288, 50)
test_set_y shape (1, 50)
sanity check after
reshaping
[17 31 56 22
33]
To represent color images, the red, green and blue channels (RGB) must be specified for each pixel, and so the
pixel value is actually a vector of three numbers ranging from 0 to 255.
One common preprocessing step in machine learning is to center and standardize your dataset, meaning that
you substract the mean of the whole numpy array from each example, and then divide each example by the
standard deviation of the whole numpy array. But for picture datasets, it is simpler and more convenient and
works almost as well to just divide every row of the dataset by 255 (the maximum value of a pixel channel).
Let's standardize our dataset.
In[50]:
What you need to remember:
Common steps for pre-processing a new dataset are:
Figure out the dimensions and shapes of the problem (m_train, m_test, num_px, ...)
Reshape the datasets such that each example is now a vector of size (num_px * num_px * 3, 1)
"Standardize" the data
train_set_x_flatten shape: (12288, 209)
train_set_y shape: (1, 209)
test_set_x_flatten shape: (12288, 50)
test_set_y shape: (1, 50)
sanity check after reshaping: [17 31 56 22 33]
# Reshape the training and test examples
### START CODE HERE ### (
≈
2 lines of code)
train_set_x_flatten = train_set_x_orig.reshape(train_set_x_orig.shape[0],
-
1).T
test_set_x_flatten = test_set_x_orig.reshape(test_set_x_orig.shape[0],
-
1).T
### END CODE HERE ###
print ("train_set_x_flatten shape: "
+
str(train_set_x_flatten.shape))
print ("train_set_y shape: "
+
str(train_set_y.shape))
print ("test_set_x_flatten shape: "
+
str(test_set_x_flatten.shape))
print ("test_set_y shape: "
+
str(test_set_y.shape))
print ("sanity check after reshaping: "
+
str(train_set_x_flatten[0:5,0]))
train_set_x = train_set_x_flatten
/
255.
test_set_x = test_set_x_flatten
/
255.
2019/3/29 Logistic Regression with a Neural Network mindset v4
file:///E:/python/Andrew Ng_DL/DeepLearning/Course1/Logistic Regression with a Neural Network mindset v4(week-2).htm 6/24
3 - General Architecture of the learning algorithm
It's time to design a simple algorithm to distinguish cat images from non-cat images.
You will build a Logistic Regression, using a Neural Network mindset. The following Figure explains why
Logistic Regression is actually a very simple Neural Network!
Mathematical expression of the algorithm:
For one example x
( i )
:
z
( i )
= w
T
x
( i )
+ b
ˆ
y
( i )
= a
( i )
= sigmoid(z
( i )
)
L(a
( i )
, y
( i )
) = − y
( i )
log(a
( i )
) − (1 − y
( i )
)log(1 − a
( i )
)
The cost is then computed by summing over all training examples:
J =
1
m
m
∑
i = 1
L(a
( i )
, y
( i )
)
Key steps: In this exercise, you will carry out the following steps:
- Initialize the parameters of the model
- Learn the parameters for the model by minimizing the cost
- Use the learned parameters to make predictions (on the test set)
- Analyse the results and conclude