"Android页面中实现可编辑与不可编辑切换的技巧" 在Android应用开发中,经常需要设计一些用户界面,允许用户在查看模式和编辑模式之间自由切换。这种功能通常用于用户输入或修改数据的场景。下面我们将详细介绍如何实现这样一个功能。 首先,我们来看一下提供的代码片段。这个页面包含了一个`LinearLayout`作为根布局,它有两个主要组件:一个`Button`和一个`RadioGroup`。`Button`用于触发编辑模式,`RadioGroup`则展示了两个选项供用户选择,例如性别。 ```xml <LinearLayout xmlns:android="http://schemas.android.com/apk/res/android" xmlns:tools="http://schemas.android.com/tools" android:id="@+id/all_views" android:layout_width="match_parent" android:layout_height="match_parent" android:orientation="vertical" tools:context=".MainActivity"> <Button android:id="@+id/edit" android:layout_width="match_parent" android:layout_height="wrap_content" android:text="编辑" android:textSize="25sp"/> <RadioGroup android:layout_width="match_parent" android:layout_height="wrap_content" android:orientation="horizontal"> <RadioButton android:id="@+id/boy" android:layout_width="wrap_content" android:layout_height="wrap_content" android:text="男" android:textSize="25sp"/> <RadioButton android:id="@+id/girl" android:layout_width="wrap_content" android:layout_height="wrap_content" android:text="女" android:textSize="25sp"/> </RadioGroup> </LinearLayout> ``` 实现编辑切换的关键步骤: 1. 监听按钮点击事件:我们需要给`Button`设置一个点击监听器,当用户点击"编辑"按钮时,触发切换操作。可以使用`setOnClickListener`方法来实现。 ```java Button editButton = findViewById(R.id.edit); editButton.setOnClickListener(new View.OnClickListener() { @Override public void onClick(View v) { // 在这里实现切换逻辑 } }); ``` 2. 禁用/启用组件:在点击事件处理函数中,我们可以根据当前状态启用或禁用`RadioGroup`及其内部的`RadioButton`。`setEnabled(false)`将使组件变为灰色,不可点击;而`setEnabled(true)`则恢复其可交互状态。 ```java RadioGroup radioGroup = findViewById(R.id.radio_group); radioGroup.setEnabled(!radioGroup.isEnabled()); ``` 3. 更改UI提示:在切换编辑模式时,可以更新按钮的文本,例如从"编辑"变为"完成",让用户清楚当前所处的状态。 ```java editButton.setText(editButton.getText().equals("编辑") ? "完成" : "编辑"); ``` 4. 保存和提交数据:在进入编辑模式后,用户对数据的修改应当被记录。当用户完成编辑并点击"完成"按钮时,可以收集修改的数据,并进行必要的验证和存储。 ```java RadioButton selectedButton = radioGroup.getCheckedRadioButtonId() == R.id.boy ? findViewById(R.id.boy) : findViewById(R.id.girl); String gender = selectedButton.getText().toString(); // 保存gender到数据库或其他存储方式 ``` 5. 动画效果:为了提供更好的用户体验,可以添加一些过渡动画,如淡入淡出、缩放等,以平滑地展示编辑模式的切换。 通过以上步骤,你可以创建一个能够实现编辑与查看模式切换的Android页面。这只是一个基本的实现,实际项目中可能还需要考虑其他因素,比如数据的持久化、错误处理和用户反馈等。同时,对于更复杂的界面,你可能需要处理更多类型的组件,如`EditText`、`Spinner`等,方法类似,只是组件类型不同,处理方式也会有所差异。
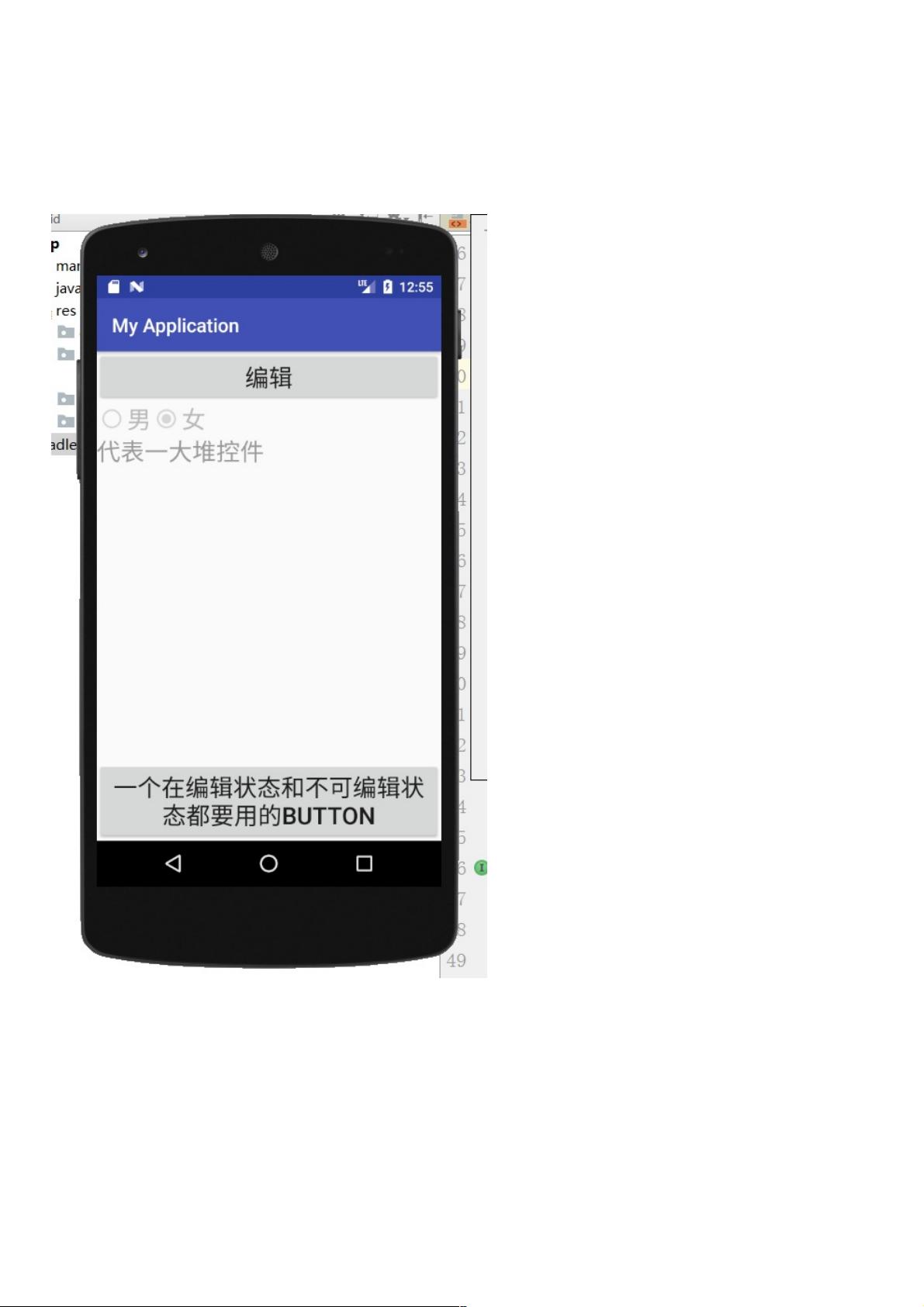
下载后可阅读完整内容,剩余5页未读,立即下载
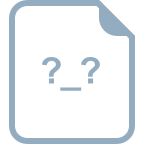
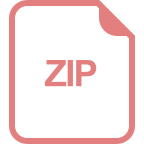


















- 粉丝: 2
- 资源: 948
我的内容管理 展开
我的资源 快来上传第一个资源
我的收益
登录查看自己的收益我的积分 登录查看自己的积分
我的C币 登录后查看C币余额
我的收藏
我的下载
下载帮助

最新资源
- 十种常见电感线圈电感量计算公式详解
- 军用车辆:CAN总线的集成与优势
- CAN总线在汽车智能换档系统中的作用与实现
- CAN总线数据超载问题及解决策略
- 汽车车身系统CAN总线设计与应用
- SAP企业需求深度剖析:财务会计与供应链的关键流程与改进策略
- CAN总线在发动机电控系统中的通信设计实践
- Spring与iBATIS整合:快速开发与比较分析
- CAN总线驱动的整车管理系统硬件设计详解
- CAN总线通讯智能节点设计与实现
- DSP实现电动汽车CAN总线通讯技术
- CAN协议网关设计:自动位速率检测与互连
- Xcode免证书调试iPad程序开发指南
- 分布式数据库查询优化算法探讨
- Win7安装VC++6.0完全指南:解决兼容性与Office冲突
- MFC实现学生信息管理系统:登录与数据库操作

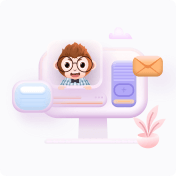
