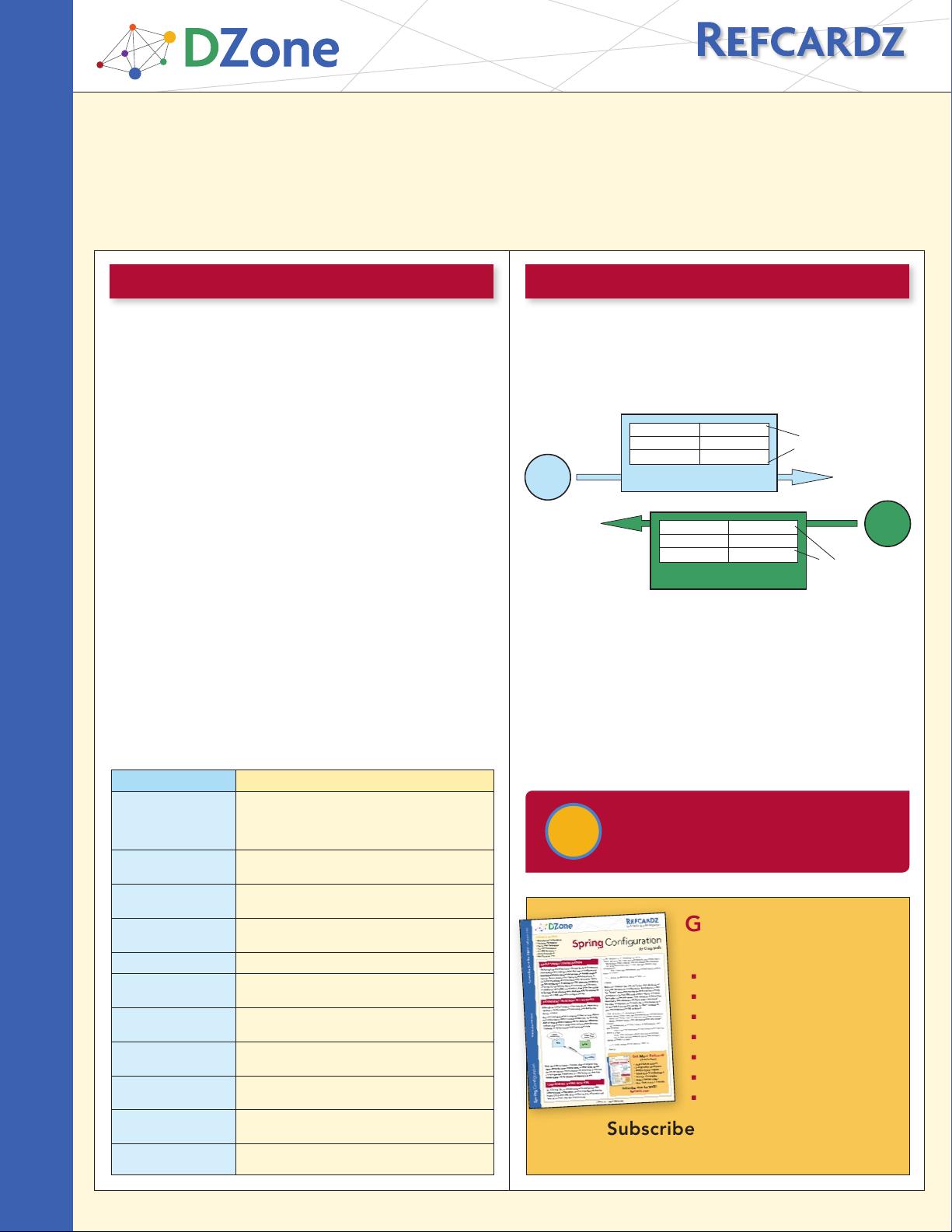
Getting Started with Ajax
By Dave Crane
CONTENTS INCLUDE:
n
Getting to Know HTTP
n
Tips for Using XHR
n
Ajax and Architecture
n
Ajax Toolkits
n
Ajax User Interfaces
n
Hot Tips and more...
n
Authoritative content
n
Designed for developers
n
Written by top experts
n
Latest tools & technologies
n
Hot tips & examples
n
Bonus content online
n
New issue every 1-2 weeks
Subscribe Now for FREE!
Refcardz.com
Get More Refcardz
(
They’re free!
)
DZone, Inc.
|
www.dzone.com
gETTINg STarTED
The standard way to do Ajax is to use the XMLHttpRequest object,
known as XHR by its friends. Use XHR directly, or via one of the
helpful Ajax libraries such as Prototype or jQuery. How do we use
XHR “by hand”? To start with, we need to get a reference to it:
We can then open a connection to a URL:
Specify a callback function to receive the response:
and then send the request:
The server may be busy, or the network may be slow. We don’t want
to sit around doing nothing until the response arrives, and because
we’ve assigned the callback function, we don’t have to. That’s the
five-minute guide for the impatient. For those who like to know
the details, we’ve listed the fuller details of the XHR object below.
if (window.XMLHttpRequest) {
xhr = new XMLHttpRequest();
} else if (window.ActiveXObject) {
xhr = new ActiveXObject(“Microsoft.XMLHTTP”);
}
xhr.onreadystatechange = function(){
processReqChange(req);
}
xhr.send(null);
xhr.open(
“GET”,
“my-dynamic-content.jsp?id=”
+encodeURI(myId),
true
);
Method Name Parameters and Descriptions
open(method, url, async) open a connection to a URL
method = HTTP verb (GET, POST, etc.)
url = url to open, may include querystring
async = whether to make asynchronous request
onreadystatechange assign a function object as callback (similar to onclick,
onload, etc. in browser event model)
setRequestHeader
(namevalue)
add a header to the HTTP request
send(body) send the request
body = string to be used as request body
abort() stop the XHR from listening for the response
readyState stage in lifecycle of response (only populated after send()
is called)
httpStatus The HTTP return code (integer, only populated after
response reaches the loaded state)
responseText body of response as a JavaScript string (only set after
response reaches the interactive readyState)
responseXML body of the response as a XML document object (only
set after response reaches the interactive readyState)
getResponseHeader
(name)
read a response header by name
getAllResponseHeaders() Get an array of all response header names
Getting Started with Ajax www.dzone.com Subscribe Now for FREE! refcardz.com
To make use of the XHR to its fullest, we recommend you
become familiar with the workings of the HTTP protocol. Using
Ajax, you have much more control over HTTP than with classic
web app development.
HTTP is a stateless request-response protocol.
n
Both request and response contain headers and an optional
body, which is free text.
n
Only a POST request contains a body.
n
A request defines a verb or method.
n
The Mime type of request and response can be set by the
header Content-type
gETTINg TO KNOw HTTP
body
request
headers
browser
body
response
headers
server
Hot
Tip
Not all Microsoft browsers rely on ActiveX.
IE7 provides a native JavaScript XHR, so we
check for that first.
tech facts at your fingertips