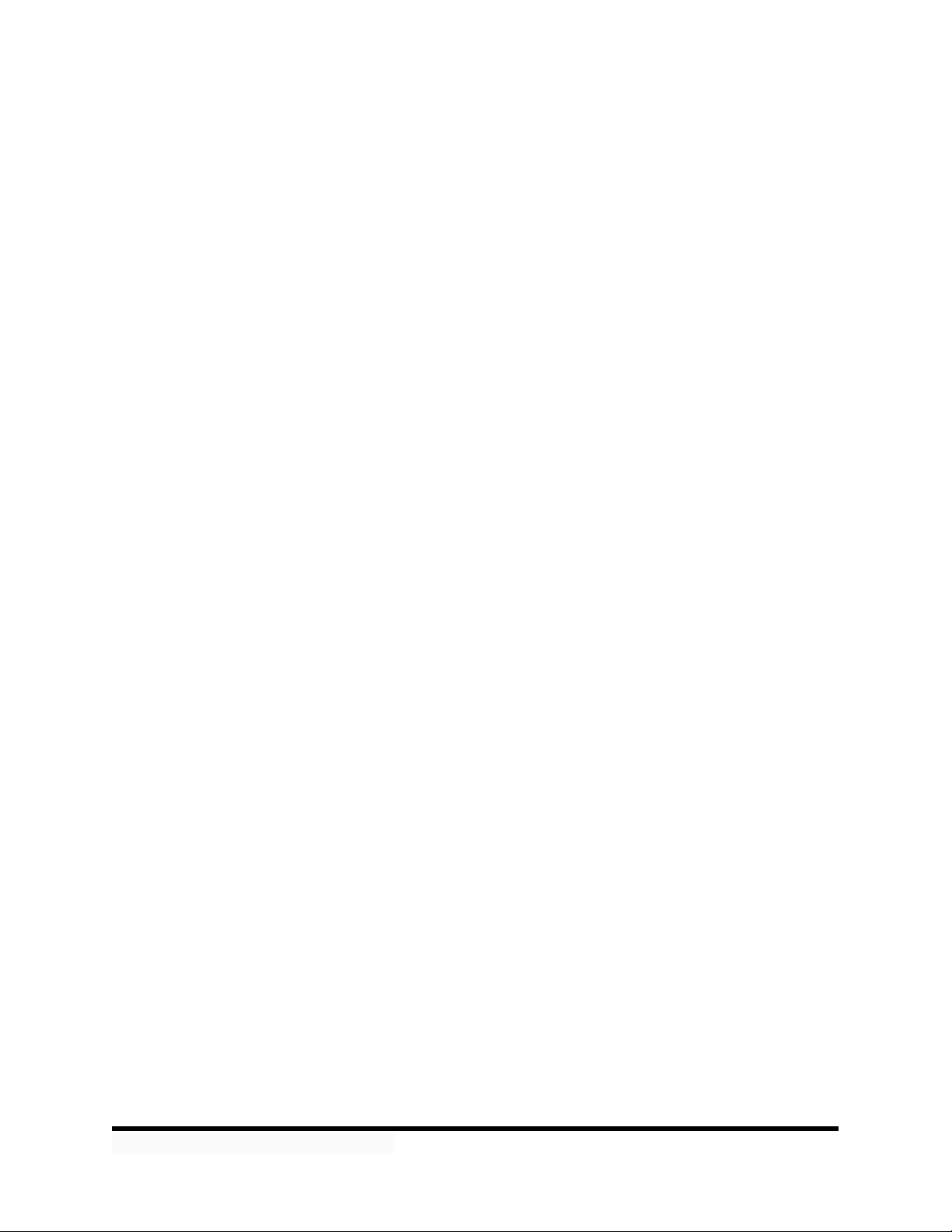
© Alex Allain (www.cprogramming.com)
Terminology
Throughout the book, I’ll be defining new terms, but let’s get started with some very basic concepts that
you’ll need to get started.
Programming
Programming is the act of writing instructions in a way that allows a computer to understand and
execute those instructions. The instructions themselves are called source code. That's what you'll be
writing. We'll see some source code for the very first time in a few pages.
Executable
The end result of programming is that you have an executable file. An executable is a file that your
computer can run—if you’re on Windows, you’ll know these files as EXEs. A computer program like
Microsoft Word is an executable. Some programs have additional files (graphics files, music files, etc.)
but every program requires an executable file. To make an executable, you need a compiler, which is a
program that turns source code into an executable. Without a compiler, you won’t be able to do
anything except look at your source code. Since that gets boring quickly, the very next thing we will do is
set you up with a compiler.
Editing and compiling source files
The rest of this chapter is devoted to getting you set up with a simple, easy-to-use development
environment. I'll get you set up with two specific tools, a compiler and an editor. You've already learned
why you need a compiler—to make the program do stuff. The editor is less obvious, but equally
important: an editor makes it possible for you to create source code in the right format.
Source code must be written in a plain text format. Plain text files contain nothing but the text of the
file; there is no additional information about how to format or display the content. In contrast, a file you
produce using Microsoft Word (or similar products) is not a plain text file because it contains
information about the fonts used, the size of the text, and how you’ve formatted the text. You don’t see
this information when you open the file in Word, but it’s all there. Plain text files have just the raw text,
and you can create them using the tools we're about to discuss.
The editor will also give you two other nice features, syntax highlighting and auto-indentation. Syntax
highlighting just means it adds color coding so that you can easily tell apart different elements of a
program. Auto-indentation means that it will help you format your code in a readable way.
If you're using Windows or a Mac, I'll get you set you up with a sophisticated editor, known as an
integrated development environment (IDE) that combines an editor with a compiler. If you're using
Linux, we'll use an easy-to-use editor known as nano. I'll explain everything you need in order to get set
up and working!
A note about sample source code
This book includes extensive sample source code, all of which is made available for you to use, without
restriction but also without warranty, for your own programs. The sample code is included in
sample_code.zip, which came with this book. All sample source code files are stored in a separate
folder named after the chapter in which that source file appears (e.g. files from this chapter appear in
the folder ch1). Each source code listing in this book that has an associated file has the name (but not
the chapter) of the file as a caption.