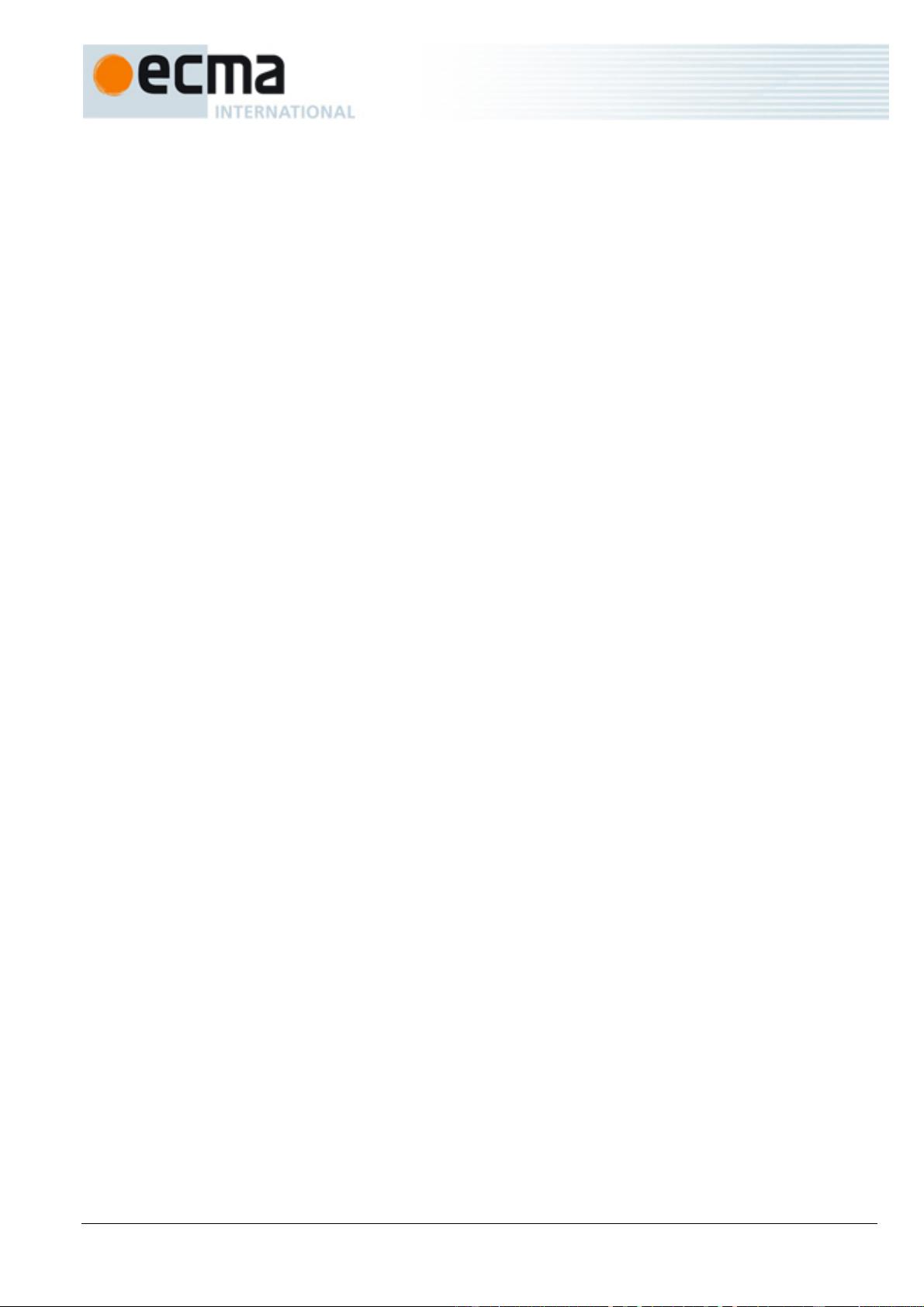
1. Let total = 0
2. For each product in groceryList
a. If product.price > maxPrice, throw an exception
b. Let total = total + product.price
In this example, steps 2.a and 2.b are repeated once for each member of the collection groceryList or until an
exception is thrown in line 2.a. The variable product is bound to the value of a different member of groceryList
before each repetition of these steps.
The second iteration convention defined by this specification is for expressing a sequence of steps that shall be
repeated once for each integer in a specified range of integers. It is expressed using the following for notation:
For variable = first to last steps
This for notation is equivalent to computing first and last, which will evaluate to integers i and j respectively, and
performing the given steps repeatedly with the variable variable bound to each member of the sequence i, i+1 … j
in numerical order. The values of first and last are computed once prior to performing steps and do not change
while performing steps. The repetition ends after variable has been bound to each item of this sequence or when
the algorithm exits via a return or a thrown exception. If i is greater than j, the steps are not performed. The steps
may be specified on the same line following a comma or on the following lines using the indentation style described
above. For example,
1. For i =
0 to priceList.length-1, call ToString(priceList[i])
In this example, ToString is called once for each item in priceList in sequential order.
A modified version of the for notation exists for iterating through a range of integers in reverse sequential order. It
is expressed using the following notation:
For variable = first downto last steps
The modified for notation works exactly as described above except the variable variable is bound to each member
of the sequence i, i-1, .. j in reverse numerical order. If i is less than j, the steps are not performed.
7.1.4 Conditional Repetition
This specification extends the notation used in the ECMAScript Edition 3 specification by defining a convention for
expressing conditional repetition of a set of steps. This convention is defined by the following notation:
While ( expression ) steps
The while notation is equivalent to computing the expression, which will evaluate to either true or false and if it is
true, taking the given steps and repeating this process until the expression evaluates to false or the algorithm exits
via a return or a thrown exception. The steps may be specified on the same line following a comma or on the
following lines using the indentation style described above. For example,
1. Let log2 = 0
2. While (n > 1)
a. Let n = n / 2
b. Let log2 = log2 +
1
In this example, steps 2.a and 2.b are repeated until the expression n > 1 evaluates to false.
7.1.5 Method Invocation
This specification extends the notation used in the ECMAScript Edition 3 specification by defining a method
invocation convention. The method invocation convention is used in this specification for calling a method of a
given object passing a given set of arguments and returning the result. This convention is defined by the following
notation:
object . methodName ( arguments )
- 7-