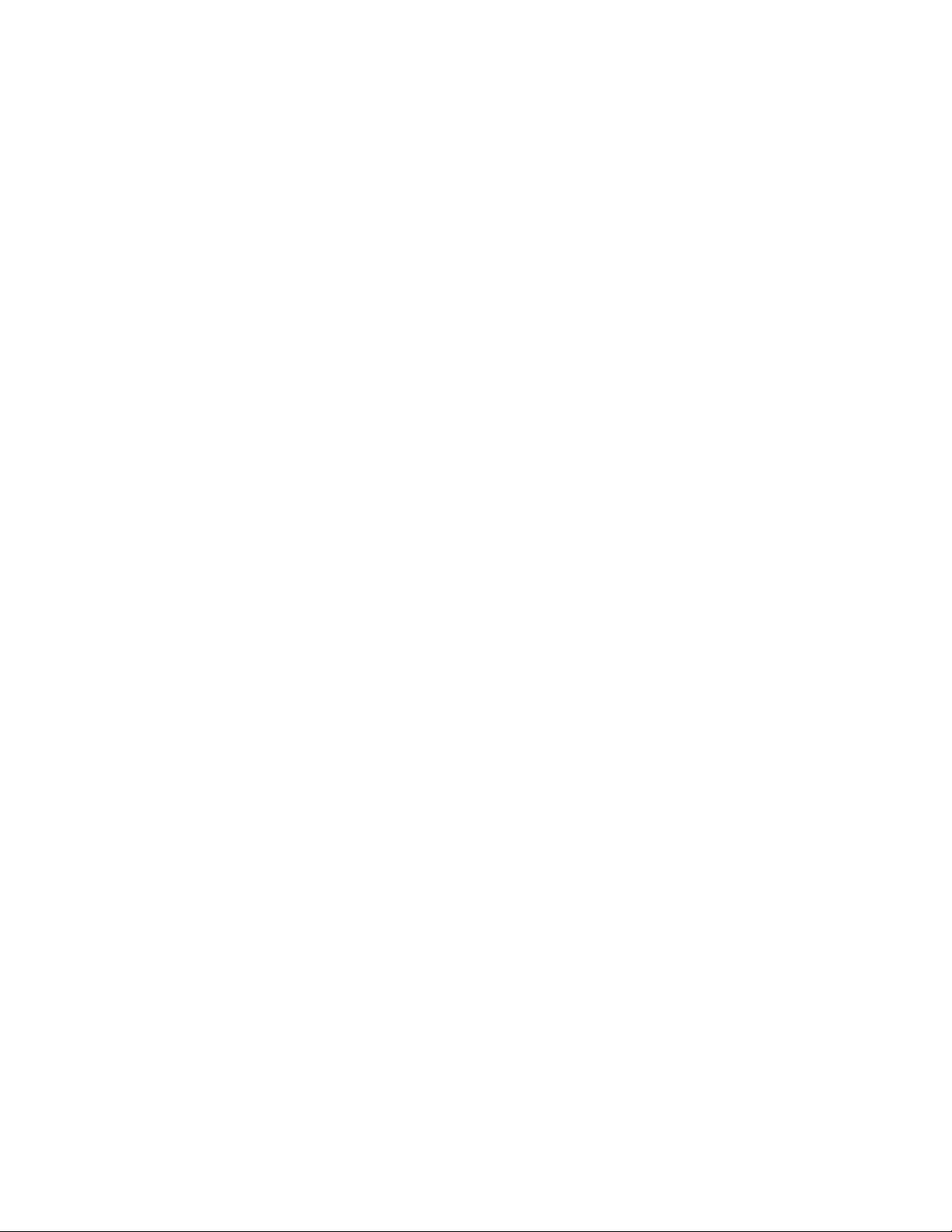
xiv
24 Signal Handling . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 654
24.1 Basic Concepts of Signals . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 654
24.1.1 Some Kinds of Signals . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 654
24.1.2 Concepts of Signal Generation . . . . . . . . . . . . . . . . . . . . . . . . . 654
24.1.3 How Signals Are Delivered . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 655
24.2 Standard Signals. . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 656
24.2.1 Program Error Signals . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 656
24.2.2 Termination Signals . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 659
24.2.3 Alarm Signals. . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 660
24.2.4 Asynchronous I/O Signals . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 660
24.2.5 Job Control Signals . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 661
24.2.6 Operation Error Signals . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 662
24.2.7 Miscellaneous Signals . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 663
24.2.8 Signal Messages . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 664
24.3 Specifying Signal Actions . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 664
24.3.1 Basic Signal Handling . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 665
24.3.2 Advanced Signal Handling . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 667
24.3.3 Interaction of signal and sigaction . . . . . . . . . . . . . . . . . . . 668
24.3.4 sigaction Function Example . . . . . . . . . . . . . . . . . . . . . . . . . . 668
24.3.5 Flags for sigaction . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 669
24.3.6 Initial Signal Actions. . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 670
24.4 Defining Signal Handlers . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 671
24.4.1 Signal Handlers that Return . . . . . . . . . . . . . . . . . . . . . . . . . . . 671
24.4.2 Handlers That Terminate the Process . . . . . . . . . . . . . . . . . . 672
24.4.3 Nonlocal Control Transfer in Handlers . . . . . . . . . . . . . . . . . . 673
24.4.4 Signals Arriving While a Handler Runs . . . . . . . . . . . . . . . . . 674
24.4.5 Signals Close Together Merge into One . . . . . . . . . . . . . . . . . 674
24.4.6 Signal Handling and Nonreentrant Functions . . . . . . . . . . . 677
24.4.7 Atomic Data Access and Signal Handling . . . . . . . . . . . . . . . 678
24.4.7.1 Problems with Non-Atomic Access . . . . . . . . . . . . . . . . 679
24.4.7.2 Atomic Types . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 679
24.4.7.3 Atomic Usage Patterns . . . . . . . . . . . . . . . . . . . . . . . . . . . . 680
24.5 Primitives Interrupted by Signals . . . . . . . . . . . . . . . . . . . . . . . . . . . 680
24.6 Generating Signals . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 681
24.6.1 Signaling Yourself . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 681
24.6.2 Signaling Another Process. . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 682
24.6.3 Permission for using kill . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 684
24.6.4 Using kill for Communication . . . . . . . . . . . . . . . . . . . . . . . . . 684
24.7 Blocking Signals . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 685
24.7.1 Why Blocking Signals is Useful . . . . . . . . . . . . . . . . . . . . . . . . . 686
24.7.2 Signal Sets . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 686
24.7.3 Process Signal Mask . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 687
24.7.4 Blocking to Test for Delivery of a Signal . . . . . . . . . . . . . . . . 688
24.7.5 Blocking Signals for a Handler . . . . . . . . . . . . . . . . . . . . . . . . . 689
24.7.6 Checking for Pending Signals . . . . . . . . . . . . . . . . . . . . . . . . . . . 690
24.7.7 Remembering a Signal to Act On Later . . . . . . . . . . . . . . . . 691
24.8 Waiting for a Signal . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 692
24.8.1 Using pause . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 692