Objective-C中银行卡归属银行的判断方法
需积分: 9 188 浏览量
更新于2024-11-08
收藏 11KB ZIP 举报
资源摘要信息:"在Objective-C编程语言中,创建一个能够判断银行卡属于哪个银行的系统需要理解并实现以下几个关键组件:BankModel、BankShareManager以及bank.plist文件。
首先,BankModel可以看作是一个银行卡实体类,它可能包含银行卡的基本属性,比如卡号、发卡行名称、卡类型等。在Objective-C中,一个典型的BankModel类可能包含各种属性(以属性的形式定义)和方法(以实例方法的形式定义)。属性可能包括NSString类型或NSNumber类型的卡号、发卡行名称等,而方法可能包括初始化方法(init)、获取和设置属性值的方法等。使用BankModel类可以方便地在程序中创建和管理银行卡对象。
接下来,BankShareManager可以被设计为一个单例(Singleton)类,用于管理对银行卡的验证操作。在Objective-C中,实现单例模式需要确保BankShareManager有一个类方法来获取单例对象,并且在外部不能创建类的多个实例。单例类中可能会包含一个方法,比如validateBankCard:,该方法接收一个BankModel对象作为参数,通过某种逻辑(可能是查找bank.plist文件)来判断输入的银行卡实体属于哪个具体的银行。这个方法会返回一个表示银行的字符串或者枚举值。
最后,bank.plist文件是一个属性列表文件,它在Objective-C项目中常被用来存储静态数据,例如银行的详细信息。bank.plist文件可以存储一个字典或数组的结构,其中包含多个键值对,每个键对应一个银行的特定标识(例如银行的短代码),而值可能包含该银行的详细信息,如银行名称、相应的卡类型、正则表达式等用于校验卡号的规则。在验证银行卡时,BankShareManager单例会读取这个文件,根据银行卡的特定标识(如卡号的前几位)来确定银行卡所属的银行,并返回相应的信息。
以上组件一起工作,就能构建出一个完整的系统,用于识别用户输入的银行卡属于哪个银行。这种系统在金融类应用程序中非常常见,用于在用户注册或支付时验证银行卡信息。
在Objective-C项目中实现这样的系统,开发者需要熟悉类的创建和继承、单例模式的设计原则、文件的操作(尤其是plist文件的读写)、字符串处理以及可能的正则表达式的应用。这些知识点是编写Objective-C程序的基础,对于构建任何需要银行卡信息验证的应用程序都是至关重要的。"
2021-05-04 上传
2022-09-21 上传
2017-08-10 上传
2023-05-12 上传
2023-07-09 上传
2023-05-24 上传
2023-05-30 上传
2023-06-06 上传
2023-06-07 上传
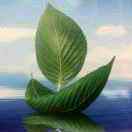
孙洋Sonya
- 粉丝: 27
- 资源: 4633
最新资源
- 黑板风格计算机毕业答辩PPT模板下载
- CodeSandbox实现ListView快速创建指南
- Node.js脚本实现WXR文件到Postgres数据库帖子导入
- 清新简约创意三角毕业论文答辩PPT模板
- DISCORD-JS-CRUD:提升 Discord 机器人开发体验
- Node.js v4.3.2版本Linux ARM64平台运行时环境发布
- SQLight:C++11编写的轻量级MySQL客户端
- 计算机专业毕业论文答辩PPT模板
- Wireshark网络抓包工具的使用与数据包解析
- Wild Match Map: JavaScript中实现通配符映射与事件绑定
- 毕业答辩利器:蝶恋花毕业设计PPT模板
- Node.js深度解析:高性能Web服务器与实时应用构建
- 掌握深度图技术:游戏开发中的绚丽应用案例
- Dart语言的HTTP扩展包功能详解
- MoonMaker: 投资组合加固神器,助力$GME投资者登月
- 计算机毕业设计答辩PPT模板下载