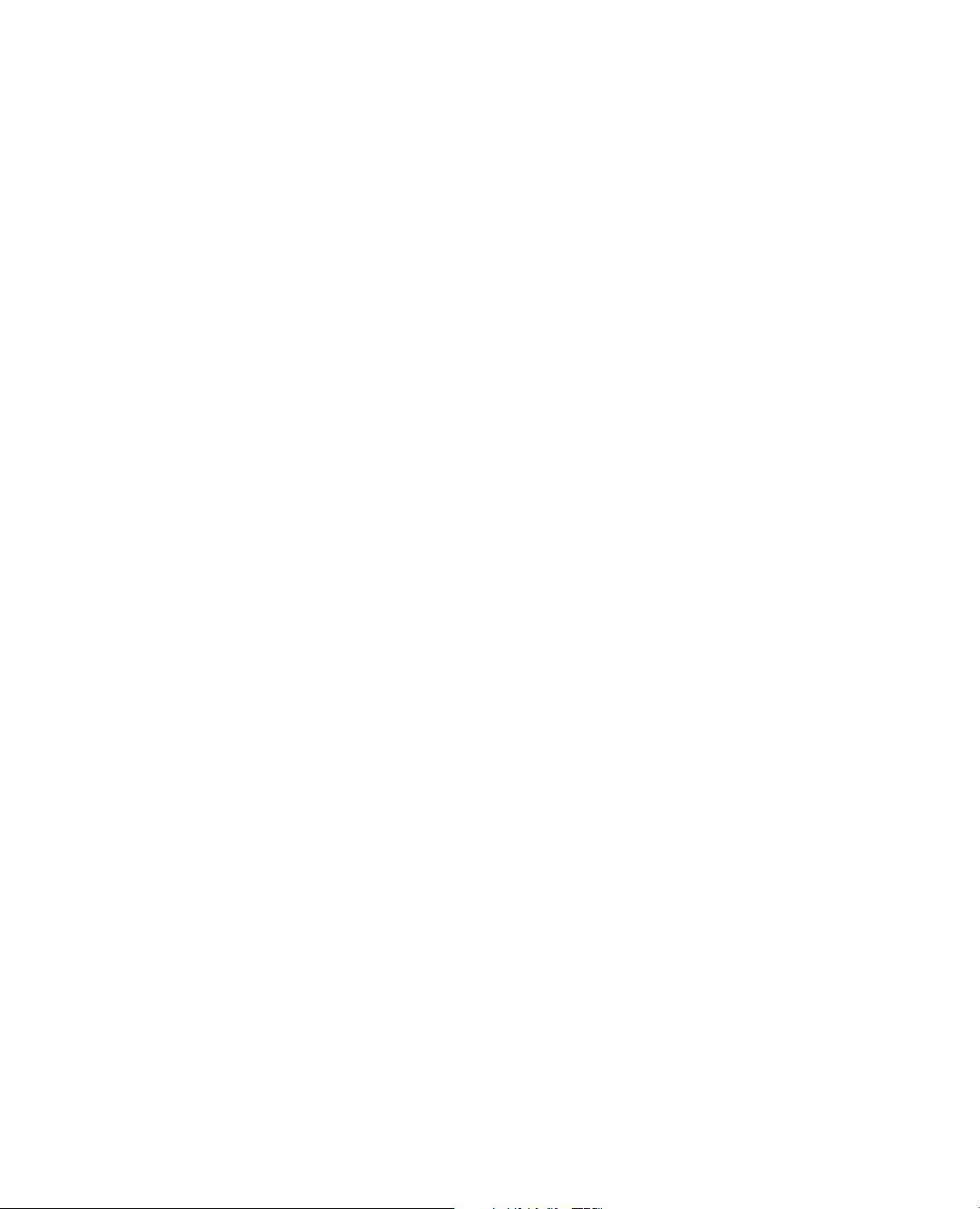
for the name n to represent an array of numbers. The arrangement would be made by stating n to be an array
in a declarative statement (a TDS) at the start of the program. Thus, the meaning and effect of an executable
statement may depend profoundly on the attributes that items of data have been declared to have.
Likewise, most of Fortran’s intrinsic mathematical functions will work for arrays: an expression like
SQRT(x), if x is the name of an array, will calculate the square roots of all the numbers in the array x.
Data items may be of a number of different “types”. For example, integer numbers and complex numbers
are different types. The word “type” is not used loosely: it is a technical term that refers to the nature, and
not the particular value, of a single data item. Most programming languages allow for a few different types
of data, but Fortran 90 allows, in principle, for an arbitrary variety of types. This is because of a feature
known as “user-defined types” or “derived types”, by which the programmer may construct a definition of a
fresh type of data relevant to the problem in hand. It is easy to draw examples from advanced mathematics:
for example, a programmer interested in four-dimensional geometry could define four-component vectors
and 4×4 transformation matrices, and could also define what would be meant by the sums and products of
such entities. As a more down-to-earth example, the latitude and longitude of a geographical point together
comprise a special sort of data item and could be set up as a user-defined type obeying its own rules
according to the programmer’s wishes. User-defined types can be more flexible than arrays: an array is a set
of items all of which must be of the same type, but a user-defined type can be a complicated structure
incorporating data of quite different basic types, e.g. mixing numbers and words. So, a single Fortran
“name”, like x, could in this way refer to a mixed package of data such as a person’s name, address,
telephone number and age.
Another interesting feature in Fortran is that of “pointer” data. The concept of a pointer is not easy to
appreciate at first, but one way to illustrate the idea is to take the example at the end of the previous
paragraph, of a set of data consisting of a person’s name, address, telephone number and age. Imagine that
we have a large list of information of this sort. Suppose that we want the set of data for each person to
include also a cross-reference to the corresponding information for the person’s spouse. There are various
ways in which this might be achieved. One way might be to give an index number to each person listed, and
to include the spouse’s index number in the data set for each person who is married. However, the simplest
method in Fortran is to include a “pointer” to a spouse’s details. The pointer would have the appearance of
an additional data item encapsulating all the spouse’s personal details; but in reality it would simply “point”
to the other area of memory where the spouse’s details are already listed in his/her own right. Using
pointers, there is no need to take up unnecessary memory space by repeating chunks of data, nor to set up
special codes for cross-referencing between data.
Modules are another very valuable feature of Fortran, and were mentioned above in Section 1.3. Like
pointers, modules are difficult to appreciate in the abstract. You have to use them, in real programs, to see
how effective they can be. Modules are useful in the architecture of large programs or systems of programs,
where they can be used to organize plug-in sets of procedures for particular purposes. In smaller programs,
modules are most useful for memory access, i.e. for containing data that is to be used by more than one
procedure.
1.7
Writing Fortran
In practice, any Fortran program takes the form of text divided into lines. Each line may be up to 132
characters in length. Usually there is one Fortran “statement” per line. Within the line, the statement does
not have to start at the first character or end at the end of the line: there may be blank spaces leading or
PROGRAMS AND PROGRAMMING 7