没有合适的资源?快使用搜索试试~ 我知道了~
首页Operating Systems - William Stalling 6th edition
资源详情
资源评论
资源推荐
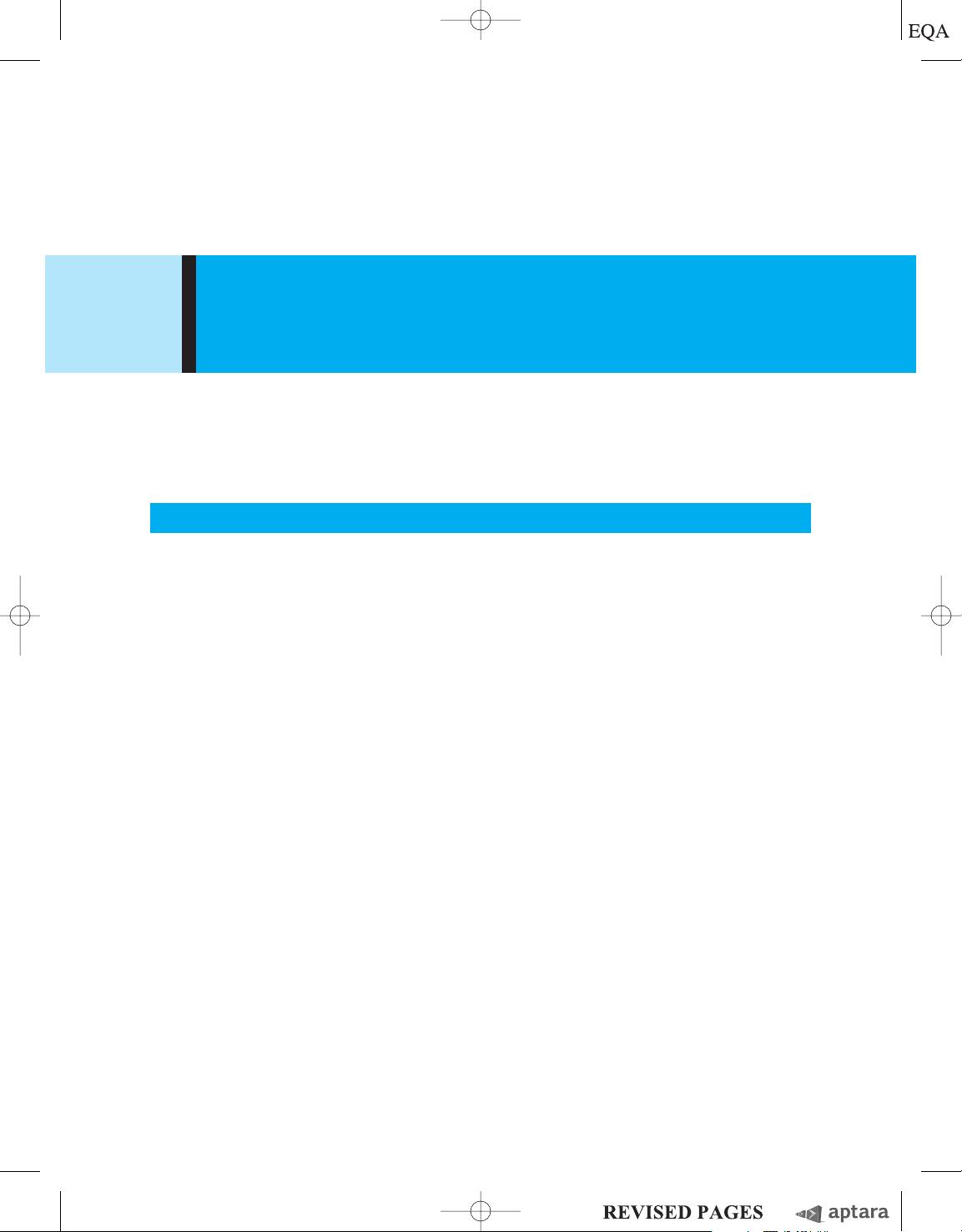
PART ONE
P
art One provides a background and context for the remainder of this book.
This part presents the fundamental concepts of computer architecture and
operating system internals.
ROAD MAP FOR PART ONE
Chapter 1 Computer System Overview
An operating system mediates among application programs, utilities, and users, on
the one hand, and the computer system hardware on the other. To appreciate the
functionality of the operating system and the design issues involved, one must have
some appreciation for computer organization and architecture. Chapter 1 provides
a brief survey of the processor, memory, and Input/Output (I/O) elements of a com-
puter system.
Chapter 2 Operating System Overview
The topic of operating system (OS) design covers a huge territory, and it is easy to
get lost in the details and lose the context of a discussion of a particular issue.
Chapter 2 provides an overview to which the reader can return at any point in the
book for context. We begin with a statement of the objectives and functions of an
operating system. Then some historically important systems and OS functions are
described. This discussion allows us to present some fundamental OS design princi-
ples in a simple environment so that the relationship among various OS functions is
clear.The chapter next highlights important characteristics of modern operating sys-
tems. Throughout the book, as various topics are discussed, it is necessary to talk
about both fundamental, well-established principles as well as more recent innova-
tions in OS design. The discussion in this chapter alerts the reader to this blend of
established and recent design approaches that must be addressed. Finally, we pre-
sent an overview of Windows, UNIX, and Linux; this discussion establishes the gen-
eral architecture of these systems, providing context for the detailed discussions to
follow.
Background
6
M01_STAL6329_06_SE_C01.QXD 2/13/08 1:48 PM Page 6
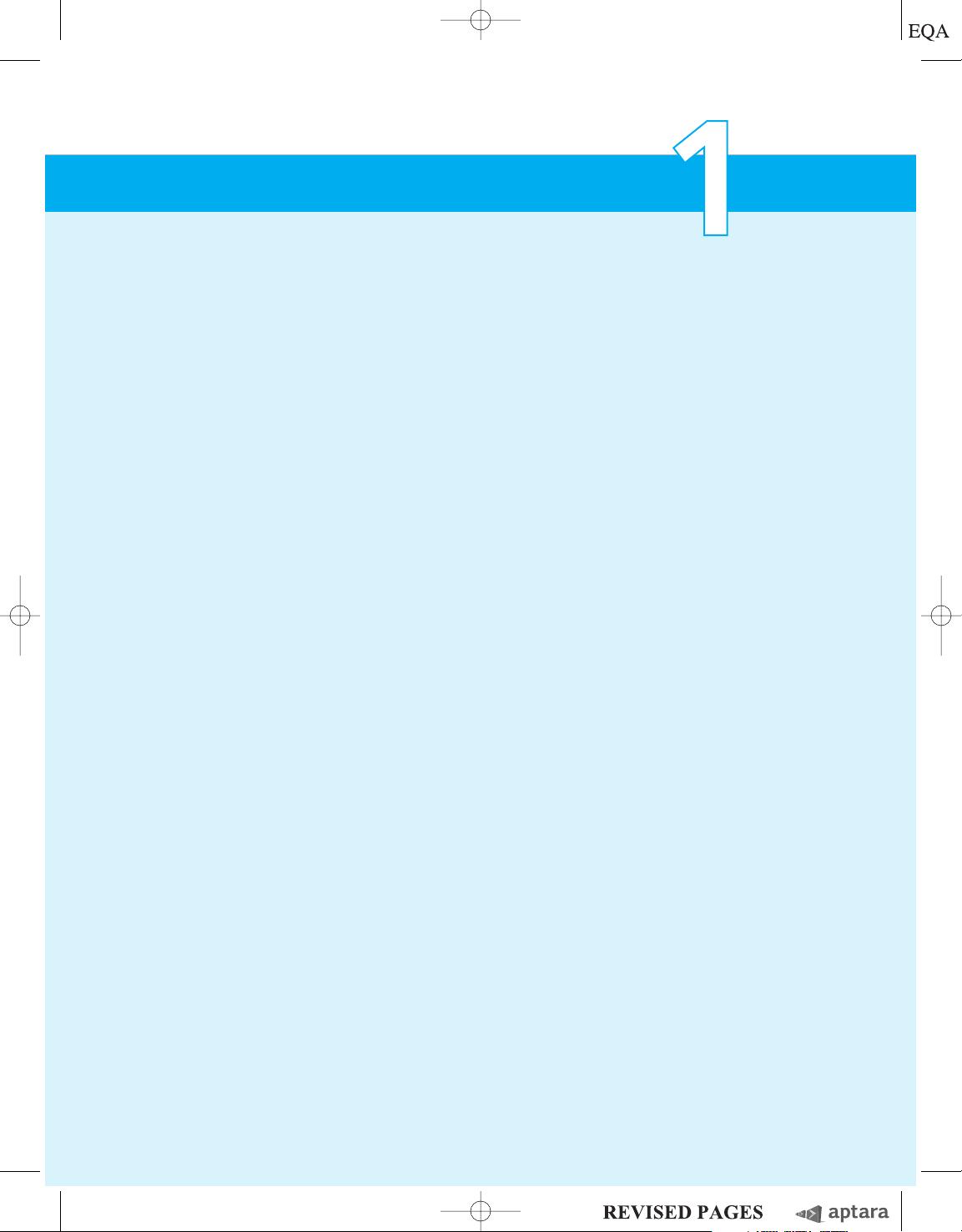
COMPUTER SYSTEM OVERVIEW
1.1 Basic Elements
1.2 Processor Registers
User-Visible Registers
Control and Status Registers
1.3 Instruction Execution
Instruction Fetch and Execute
I/O Function
1.4 Interrupts
Interrupts and the Instruction Cycle
Interrupt Processing
Multiple Interrupts
Multiprogramming
1.5 The Memory Hierarchy
1.6 Cache Memory
Motivation
Cache Principles
Cache Design
1.7 I/O Communication Techniques
Programmed I/O
Interrupt-Driven I/O
Direct Memory Access
1.8 Recommended Reading and Web Sites
1.9 Key Terms, Review Questions, and Problems
APPENDIX 1A Performance Characteristicd of Two-Level Memories
Locality
Operation of Two-Level Memory
Performance
APPENDIX 1B Procedure Control
Stack Implementation
Procedure Calls and Returns
Reentrant Procedures
7
CHAPTER
M01_STAL6329_06_SE_C01.QXD 2/13/08 1:48 PM Page 7
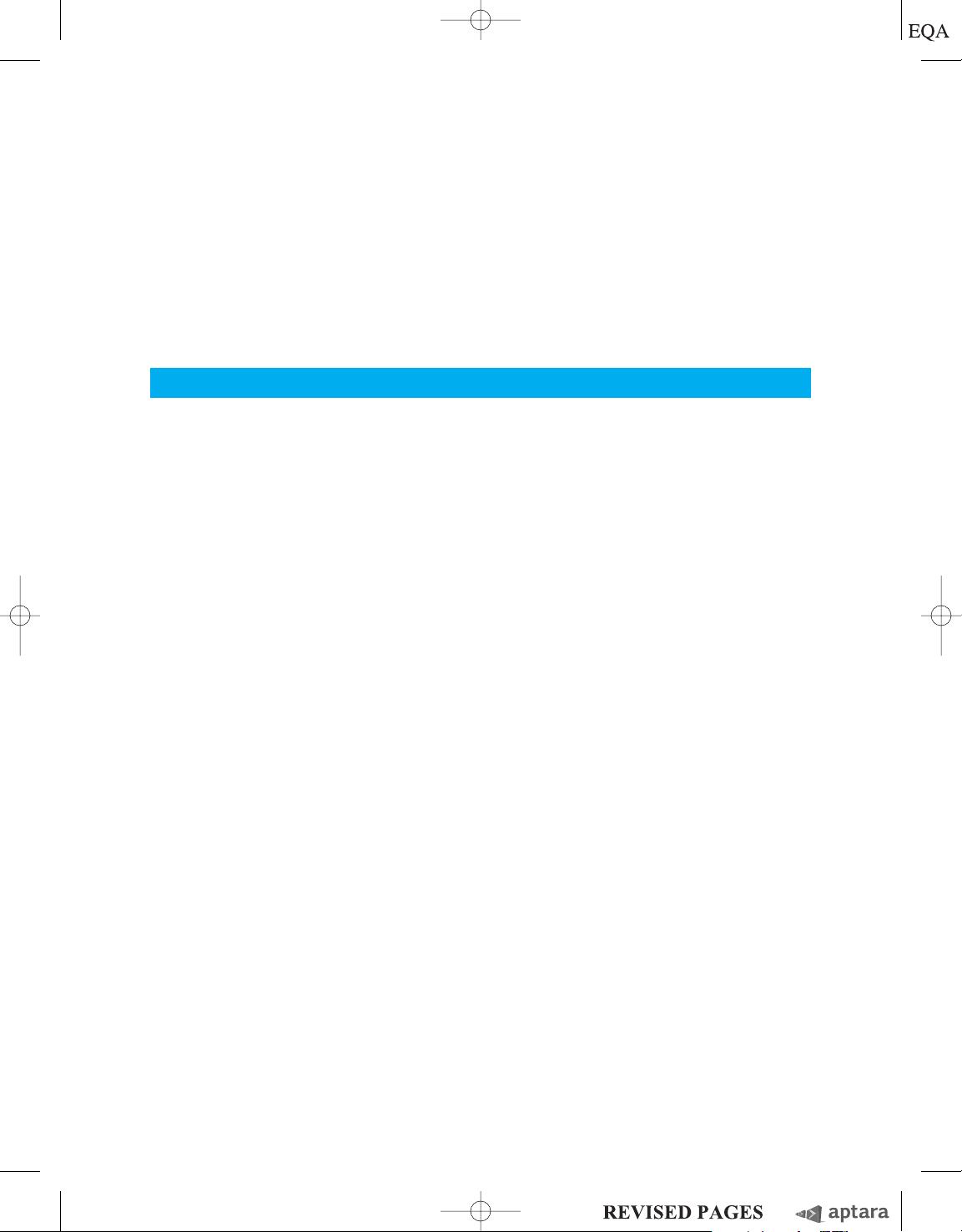
8 CHAPTER 1 / COMPUTER SYSTEM OVERVIEW
An operating system (OS) exploits the hardware resources of one or more processors
to provide a set of services to system users. The OS also manages secondary memory
and I/O (input/output) devices on behalf of its users. Accordingly, it is important to
have some understanding of the underlying computer system hardware before we begin
our examination of operating systems.
This chapter provides an overview of computer system hardware. In most areas,
the survey is brief, as it is assumed that the reader is familiar with this subject. However,
several areas are covered in some detail because of their importance to topics covered
later in the book.
1.1 BASIC ELEMENTS
At a top level, a computer consists of processor, memory, and I/O components, with
one or more modules of each type. These components are interconnected in some
fashion to achieve the main function of the computer, which is to execute programs.
Thus, there are four main structural elements:
• Processor: Controls the operation of the computer and performs its data pro-
cessing functions. When there is only one processor, it is often referred to as
the central processing unit (CPU).
• Main memory: Stores data and programs. This memory is typically volatile;
that is, when the computer is shut down, the contents of the memory are lost.
In contrast, the contents of disk memory are retained even when the computer
system is shut down. Main memory is also referred to as real memory or primary
memory.
• I/O modules: Move data between the computer and its external environ-
ment. The external environment consists of a variety of devices, including
secondary memory devices (e. g., disks), communications equipment, and
terminals.
• System bus: Provides for communication among processors, main memory,
and I/O modules.
Figure 1.1 depicts these top-level components. One of the processor’s func-
tions is to exchange data with memory. For this purpose, it typically makes use of
two internal (to the processor) registers: a memory address register (MAR), which
specifies the address in memory for the next read or write; and a memory buffer reg-
ister (MBR), which contains the data to be written into memory or which receives
the data read from memory. Similarly, an I/O address register (I/OAR) specifies a
particular I/O device. An I/O buffer register (I/OBR) is used for the exchange of
data between an I/O module and the processor.
A memory module consists of a set of locations, defined by sequentially num-
bered addresses. Each location contains a bit pattern that can be interpreted as ei-
ther an instruction or data. An I/O module transfers data from external devices to
processor and memory, and vice versa. It contains internal buffers for temporarily
holding data until they can be sent on.
M01_STAL6329_06_SE_C01.QXD 2/13/08 1:48 PM Page 8
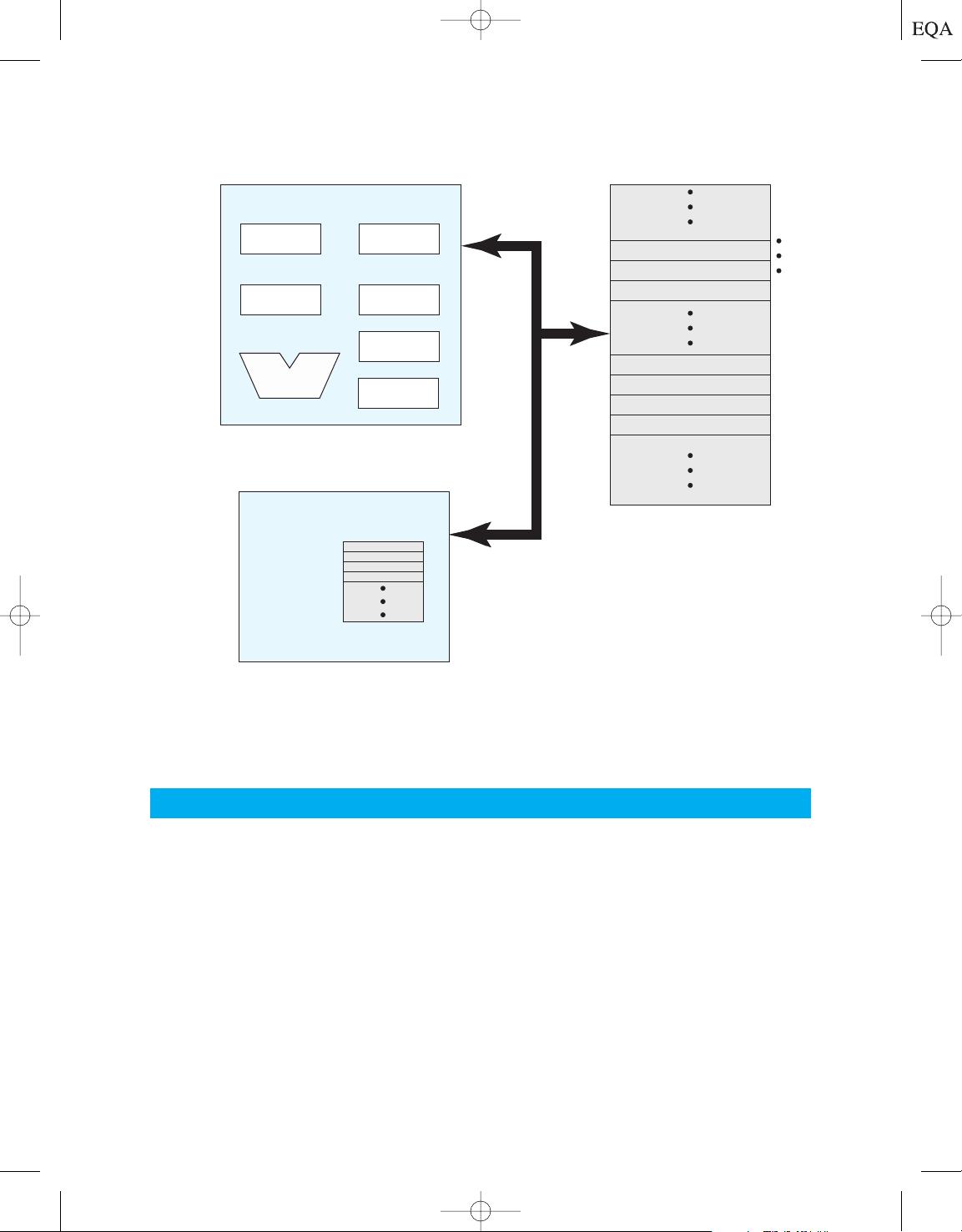
1.2 / PROCESSOR REGISTERS 9
1.2 PROCESSOR REGISTERS
A processor includes a set of registers that provide memory that is faster and smaller
than main memory. Processor registers serve two functions:
• User-visible registers: Enable the machine or assembly language programmer
to minimize main memory references by optimizing register use. For high-
level languages, an optimizing compiler will attempt to make intelligent
choices of which variables to assign to registers and which to main memory
locations. Some high-level languages, such as C, allow the programmer to sug-
gest to the compiler which variables should be held in registers.
• Control and status registers: Used by the processor to control the operation
of the processor and by privileged OS routines to control the execution of
programs.
Figure 1.1 Computer Components: Top-Level View
CPU
Main memory
System
bus
I/O module
Buffers
Instruction
n⫺2
n⫺1
Data
Data
Data
Data
Instruction
Instruction
PC ⫽ Program counter
IR ⫽ Instruction register
MAR ⫽ Memory address register
MBR ⫽ Memory buffer register
I/O AR ⫽ Input/output address register
I/O BR ⫽ Input/output buffer register
0
1
2
PC
MAR
IR MBR
I/O AR
I/O BR
Execution
unit
M01_STAL6329_06_SE_C01.QXD 2/28/08 3:42 AM Page 9
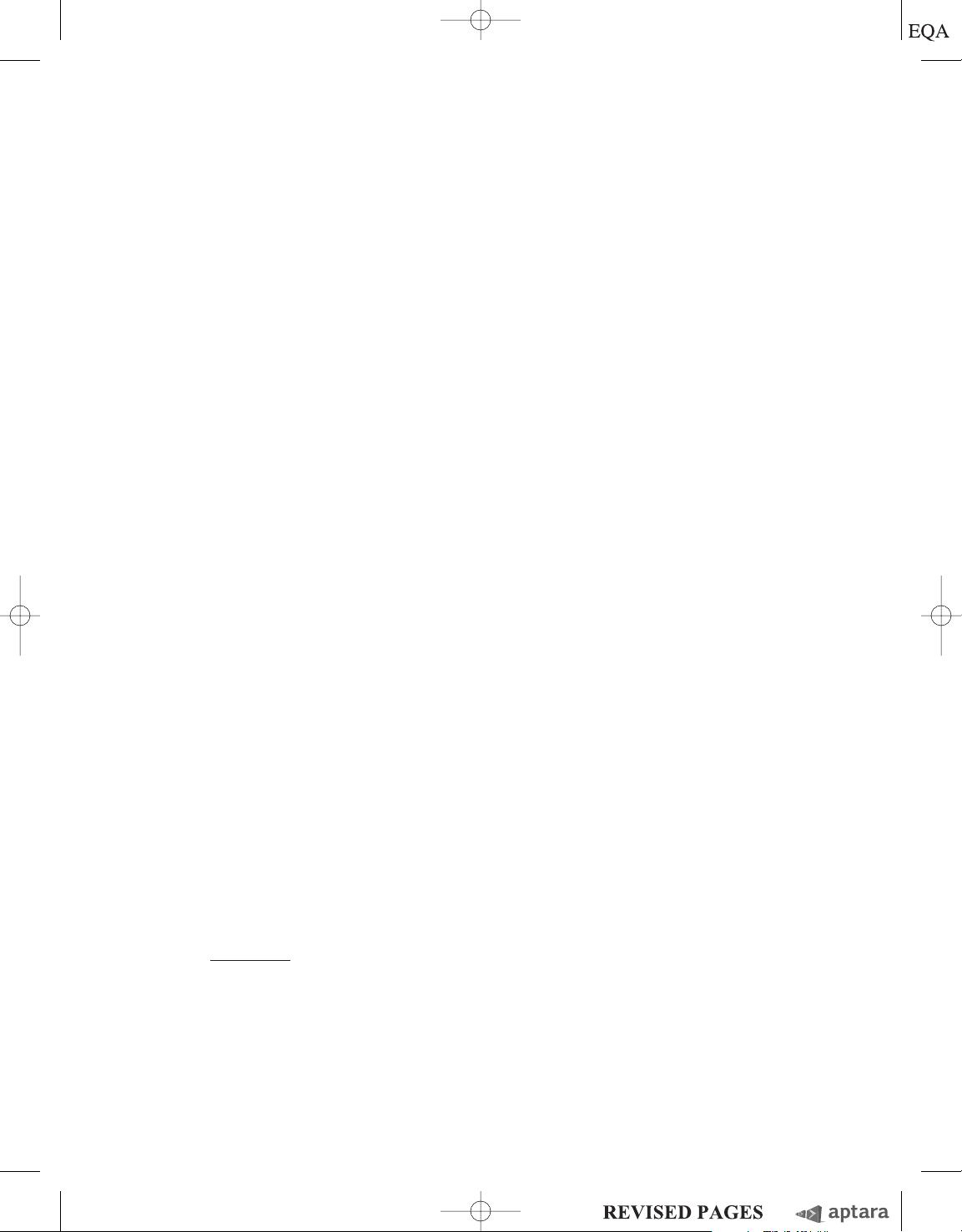
10 CHAPTER 1 / COMPUTER SYSTEM OVERVIEW
There is not a clean separation of registers into these two categories. For
example, on some processors, the program counter is user visible, but on many it
is not. For purposes of the following discussion, however,it is convenient to use these
categories.
User-Visible Registers
A user-visible register may be referenced by means of the machine language that the
processor executes and is generally available to all programs, including application
programs as well as system programs. Types of registers that are typically available
are data, address, and condition code registers.
Data registers can be assigned to a variety of functions by the programmer. In
some cases, they are general purpose in nature and can be used with any machine in-
struction that performs operations on data. Often, however, there are restrictions.
For example, there may be dedicated registers for floating-point operations and oth-
ers for integer operations.
Address registers contain main memory addresses of data and instructions, or
they contain a portion of the address that is used in the calculation of the complete
or effective address. These registers may themselves be general purpose, or may be
devoted to a particular way, or mode, of addressing memory. Examples include the
following:
• Index register: Indexed addressing is a common mode of addressing that in-
volves adding an index to a base value to get the effective address.
• Segment pointer: With segmented addressing, memory is divided into segments,
which are variable-length blocks of words.
1
A memory reference consists of a
reference to a particular segment and an offset within the segment; this mode of
addressing is important in our discussion of memory management in Chapter 7.
In this mode of addressing, a register is used to hold the base address (starting
location) of the segment. There may be multiple registers; for example, one for
the OS (i.e., when OS code is executing on the processor) and one for the cur-
rently executing application.
• Stack pointer: If there is user-visible stack
2
addressing, then there is a dedi-
cated register that points to the top of the stack.This allows the use of instruc-
tions that contain no address field, such as push and pop.
For some processors, a procedure call will result in automatic saving of all user-
visible registers, to be restored on return. Saving and restoring is performed by the
processor as part of the execution of the call and return instructions.This allows each
1
There is no universal definition of the term word. In general, a word is an ordered set of bytes or bits that
is the normal unit in which information may be stored, transmitted, or operated on within a given com-
puter. Typically, if a processor has a fixed-length instruction set, then the instruction length equals the
word length.
2
A stack is located in main memory and is a sequential set of locations that are referenced similarly to a
physical stack of papers, by putting on and taking away from the top. See Appendix 1B for a discussion of
stack processing.
M01_STAL6329_06_SE_C01.QXD 2/13/08 1:48 PM Page 10
剩余798页未读,继续阅读
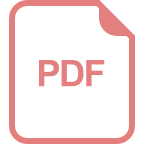
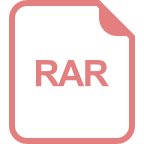



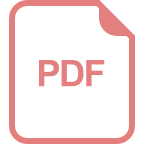
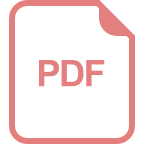
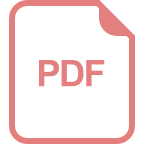
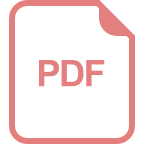
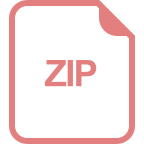
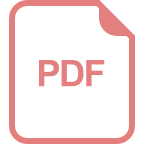
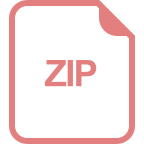

hezhen522112
- 粉丝: 1
- 资源: 1
上传资源 快速赚钱
我的内容管理 收起
我的资源 快来上传第一个资源
我的收益
登录查看自己的收益我的积分 登录查看自己的积分
我的C币 登录后查看C币余额
我的收藏
我的下载
下载帮助

会员权益专享
最新资源
- RTL8188FU-Linux-v5.7.4.2-36687.20200602.tar(20765).gz
- c++校园超市商品信息管理系统课程设计说明书(含源代码) (2).pdf
- 建筑供配电系统相关课件.pptx
- 企业管理规章制度及管理模式.doc
- vb打开摄像头.doc
- 云计算-可信计算中认证协议改进方案.pdf
- [详细完整版]单片机编程4.ppt
- c语言常用算法.pdf
- c++经典程序代码大全.pdf
- 单片机数字时钟资料.doc
- 11项目管理前沿1.0.pptx
- 基于ssm的“魅力”繁峙宣传网站的设计与实现论文.doc
- 智慧交通综合解决方案.pptx
- 建筑防潮设计-PowerPointPresentati.pptx
- SPC统计过程控制程序.pptx
- SPC统计方法基础知识.pptx
资源上传下载、课程学习等过程中有任何疑问或建议,欢迎提出宝贵意见哦~我们会及时处理!
点击此处反馈


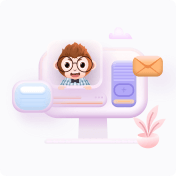
安全验证
文档复制为VIP权益,开通VIP直接复制

评论1