没有合适的资源?快使用搜索试试~ 我知道了~
首页the-road-to-react-2020.pdf
the-road-to-react-2020.pdf
需积分: 27 29 下载量 153 浏览量
更新于2023-05-13
评论
收藏 760KB PDF 举报
The Road to React teaches the fundamentals of React. You will build a real-world application in plain React without complicated tooling. Everything from project setup to deployment on a server will be explained for you. The book comes with additional referenced reading material and exercises with each chapter. After reading the book, you will be able to build your own applications in React. The material is kept up to date by myself and the community
资源详情
资源评论
资源推荐
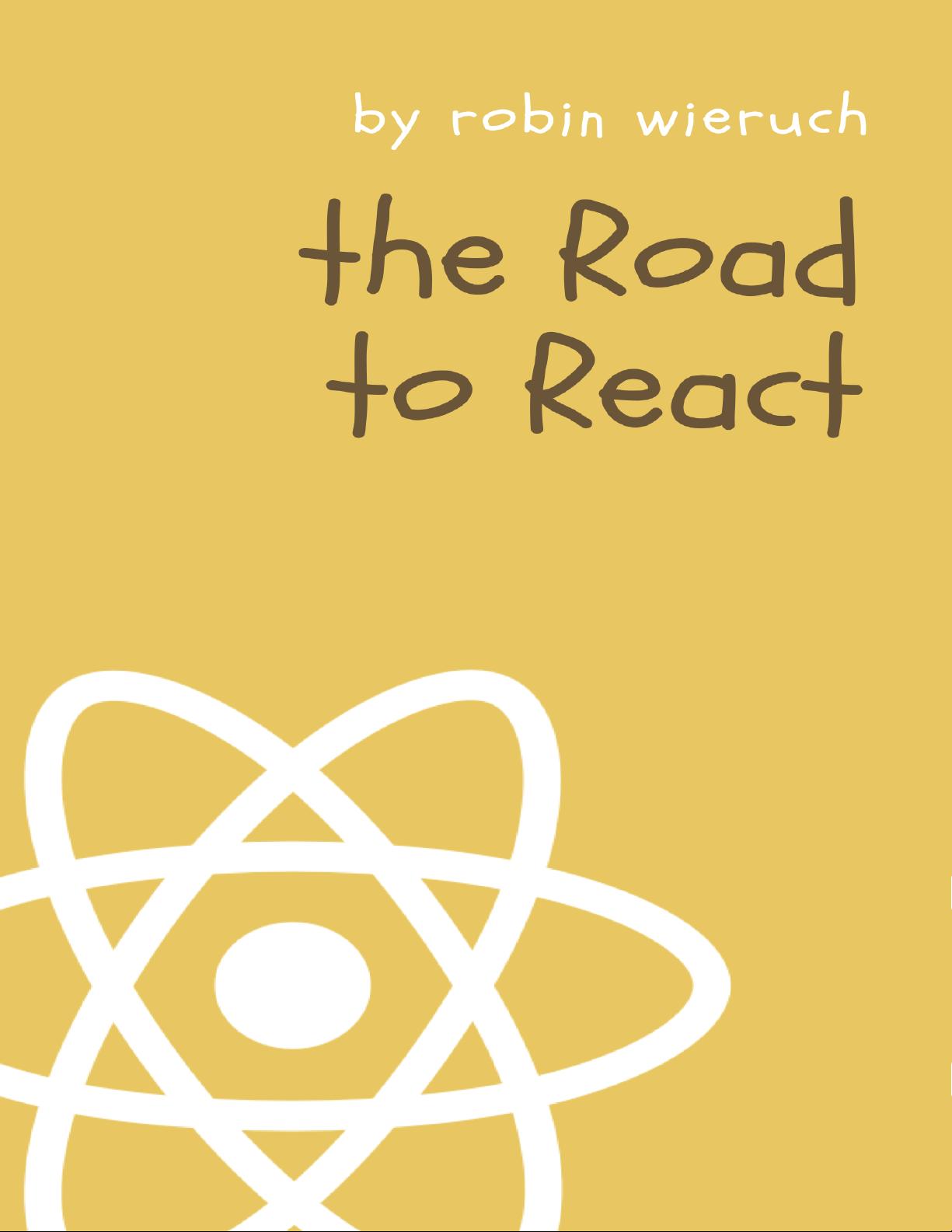
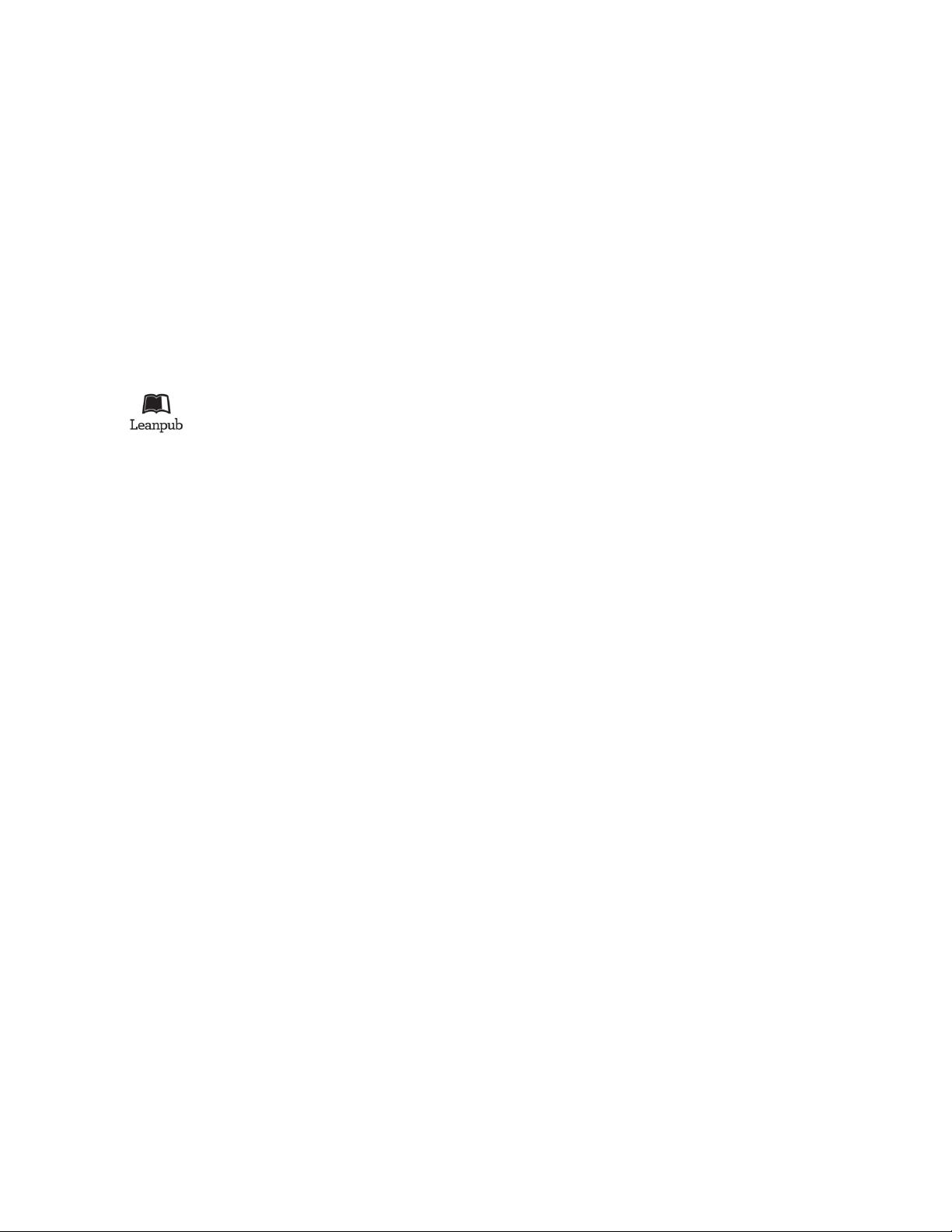
The Road to React
Your journey to master plain yet pragmatic React
Robin Wieruch
This book is for sale at http://leanpub.com/the-road-to-learn-react
This version was published on 2020-04-20
This is a Leanpub book. Leanpub empowers authors and publishers with the Lean Publishing
process. Lean Publishing is the act of publishing an in-progress ebook using lightweight tools and
many iterations to get reader feedback, pivot until you have the right book and build traction once
you do.
© 2016 - 2020 Robin Wieruch
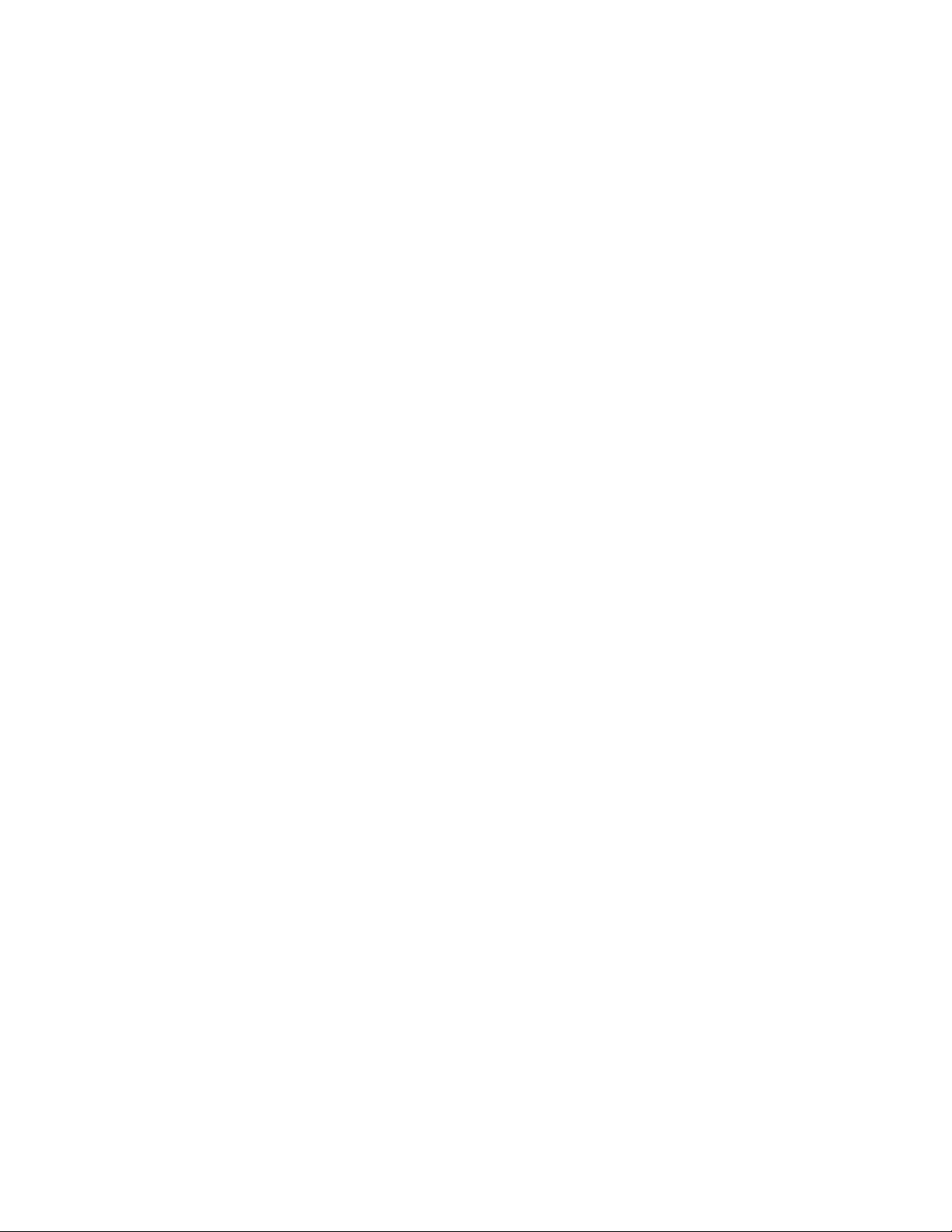
Tweet This Book!
Please help Robin Wieruch by spreading the word about this book on Twitter!
The suggested tweet for this book is:
I am going to learn #ReactJs with The Road to React by @rwieruch Join me on my journey
https://roadtoreact.com
The suggested hashtag for this book is #ReactJs.
Find out what other people are saying about the book by clicking on this link to search for this
hashtag on Twitter:
#ReactJs
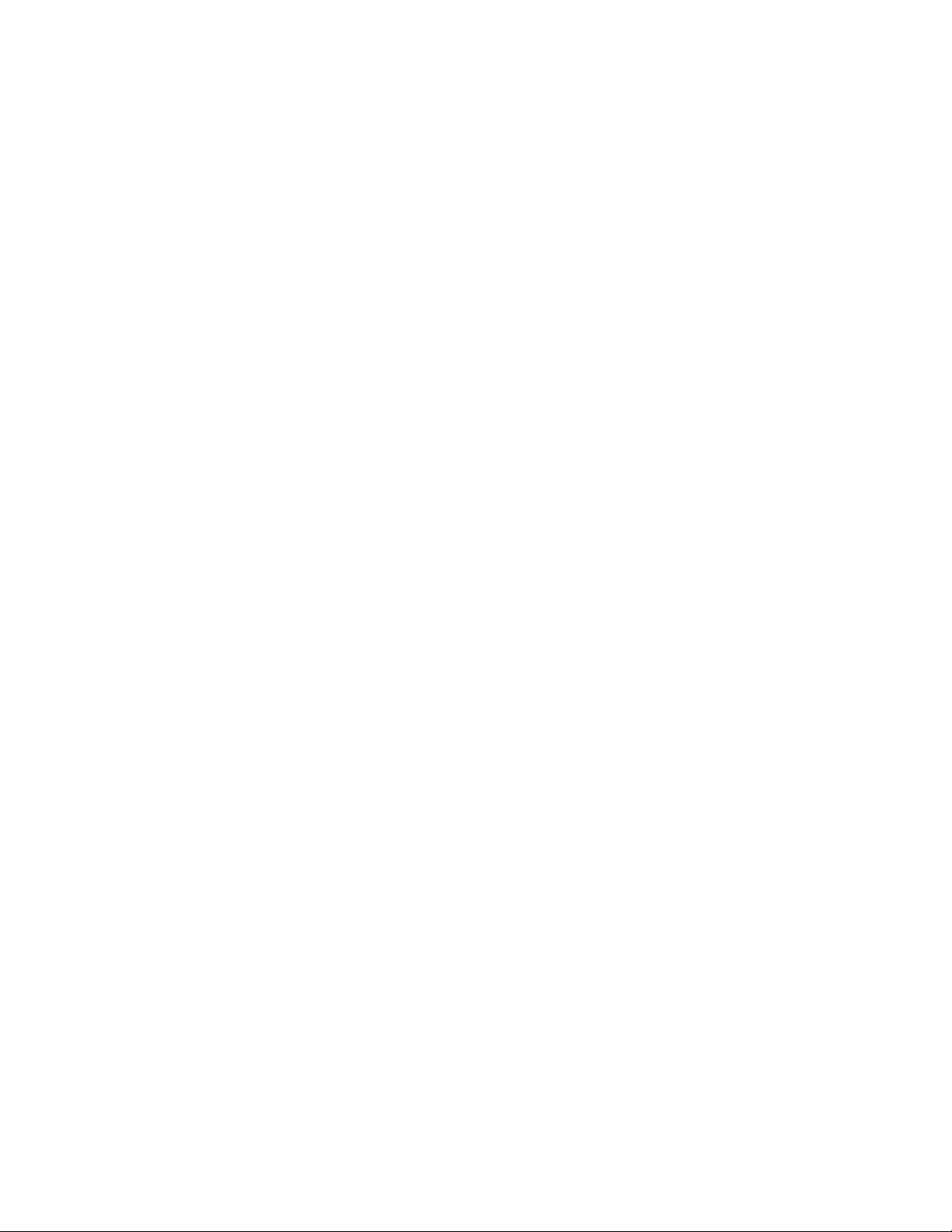
Contents
Foreword . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . i
About the Author . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . ii
FAQ . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . iii
Who is this book for? . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . v
Fundamentals of React . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 1
Hello React . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 2
Requirements . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 4
Setting up a React Project . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 6
Meet the React Component . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 10
React JSX . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 14
Lists in React . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 17
Meet another React Component . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 22
React Component Instantiation . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 24
React DOM . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 26
React Component Definition (Advanced) . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 27
Handler Function in JSX . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 31
React Props . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 34
React State . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 37
Callback Handlers in JSX . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 41
Lifting State in React . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 44
React Controlled Components . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 48
Props Handling (Advanced) . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 51
React Side-Effects . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 61
React Custom Hooks (Advanced) . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 64
React Fragments . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 68
Reusable React Component . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 70
React Component Composition . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 73
Imperative React . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 76
Inline Handler in JSX . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 80
React Asynchronous Data . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 86
React Conditional Rendering . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 88
React Advanced State . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 92
React Impossible States . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 96
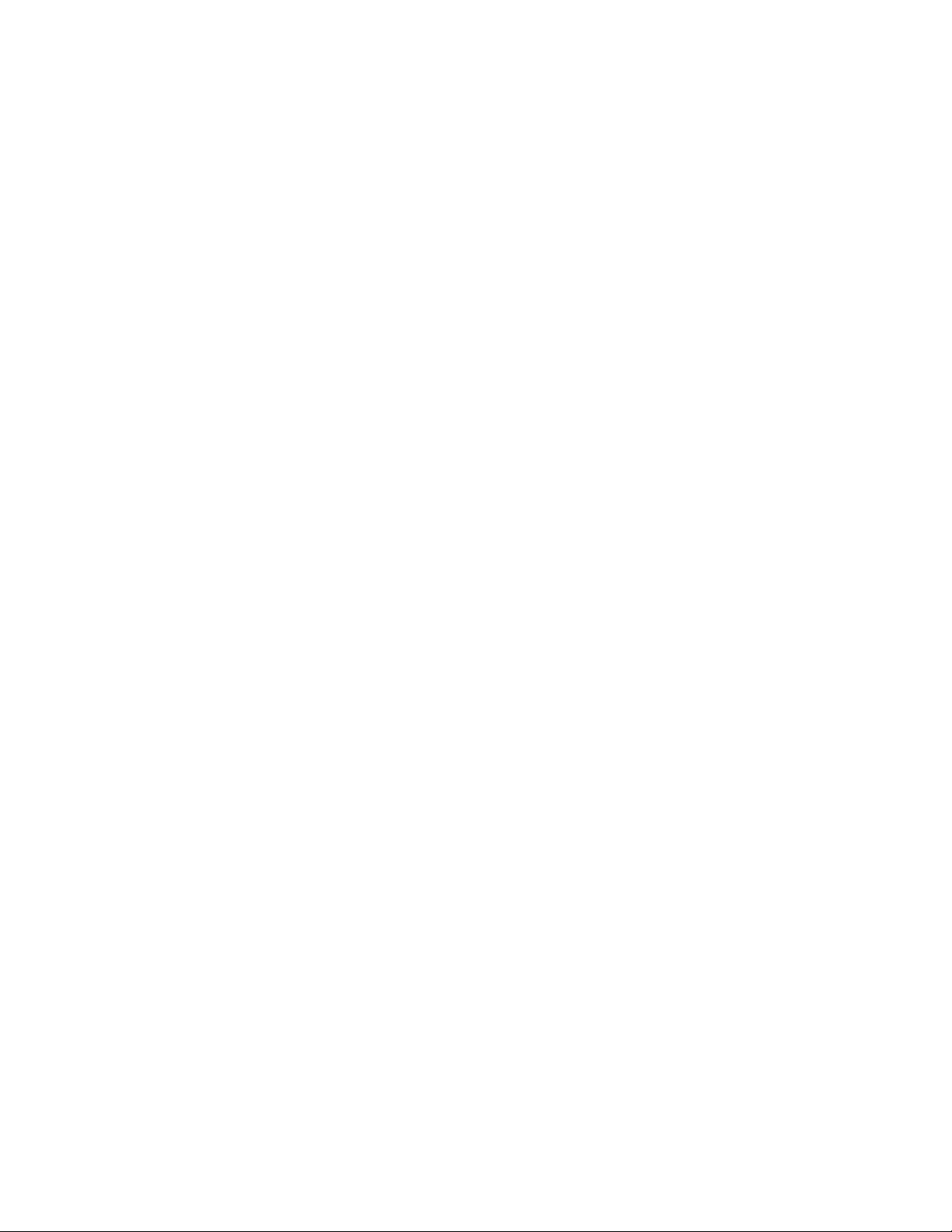
CONTENTS
Data Fetching with React . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 101
Data Re-Fetching in React . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 103
Memoized Handler in React (Advanced) . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 106
Explicit Data Fetching with React . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 108
Third-Party Libraries in React . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 111
Async/Await in React (Advanced) . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 113
Forms in React . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 115
React’s Legacy . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 118
React Class Components . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 119
React Class Components: State . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 121
Imperative React . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 123
Styling in React . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 125
CSS in React . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 126
CSS Modules in React . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 132
Styled Components in React . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 138
SVGs in React . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 144
React Maintenance . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 147
Performance in React (Advanced) . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 148
TypeScript in React . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 156
Unit Testing to Integration Testing . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 166
React Project Structure . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 181
Real World React (Advanced) . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 186
Sorting . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 187
Reverse Sort . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 193
Remember Last Searches . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 196
Paginated Fetch . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 205
Deploying a React Application . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . .
213
Build Process . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 214
Deploy to Firebase . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 215
Outline . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 218
剩余228页未读,继续阅读
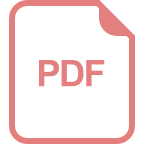
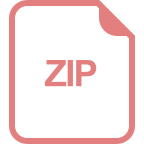

















booknz
- 粉丝: 0
- 资源: 3
上传资源 快速赚钱
我的内容管理 收起
我的资源 快来上传第一个资源
我的收益
登录查看自己的收益我的积分 登录查看自己的积分
我的C币 登录后查看C币余额
我的收藏
我的下载
下载帮助

会员权益专享
最新资源
- zigbee-cluster-library-specification
- JSBSim Reference Manual
- c++校园超市商品信息管理系统课程设计说明书(含源代码) (2).pdf
- 建筑供配电系统相关课件.pptx
- 企业管理规章制度及管理模式.doc
- vb打开摄像头.doc
- 云计算-可信计算中认证协议改进方案.pdf
- [详细完整版]单片机编程4.ppt
- c语言常用算法.pdf
- c++经典程序代码大全.pdf
- 单片机数字时钟资料.doc
- 11项目管理前沿1.0.pptx
- 基于ssm的“魅力”繁峙宣传网站的设计与实现论文.doc
- 智慧交通综合解决方案.pptx
- 建筑防潮设计-PowerPointPresentati.pptx
- SPC统计过程控制程序.pptx
资源上传下载、课程学习等过程中有任何疑问或建议,欢迎提出宝贵意见哦~我们会及时处理!
点击此处反馈


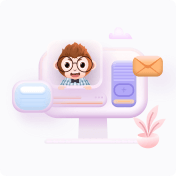
安全验证
文档复制为VIP权益,开通VIP直接复制

评论0