没有合适的资源?快使用搜索试试~ 我知道了~
首页Clean Python.pdf
资源详情
资源评论
资源推荐
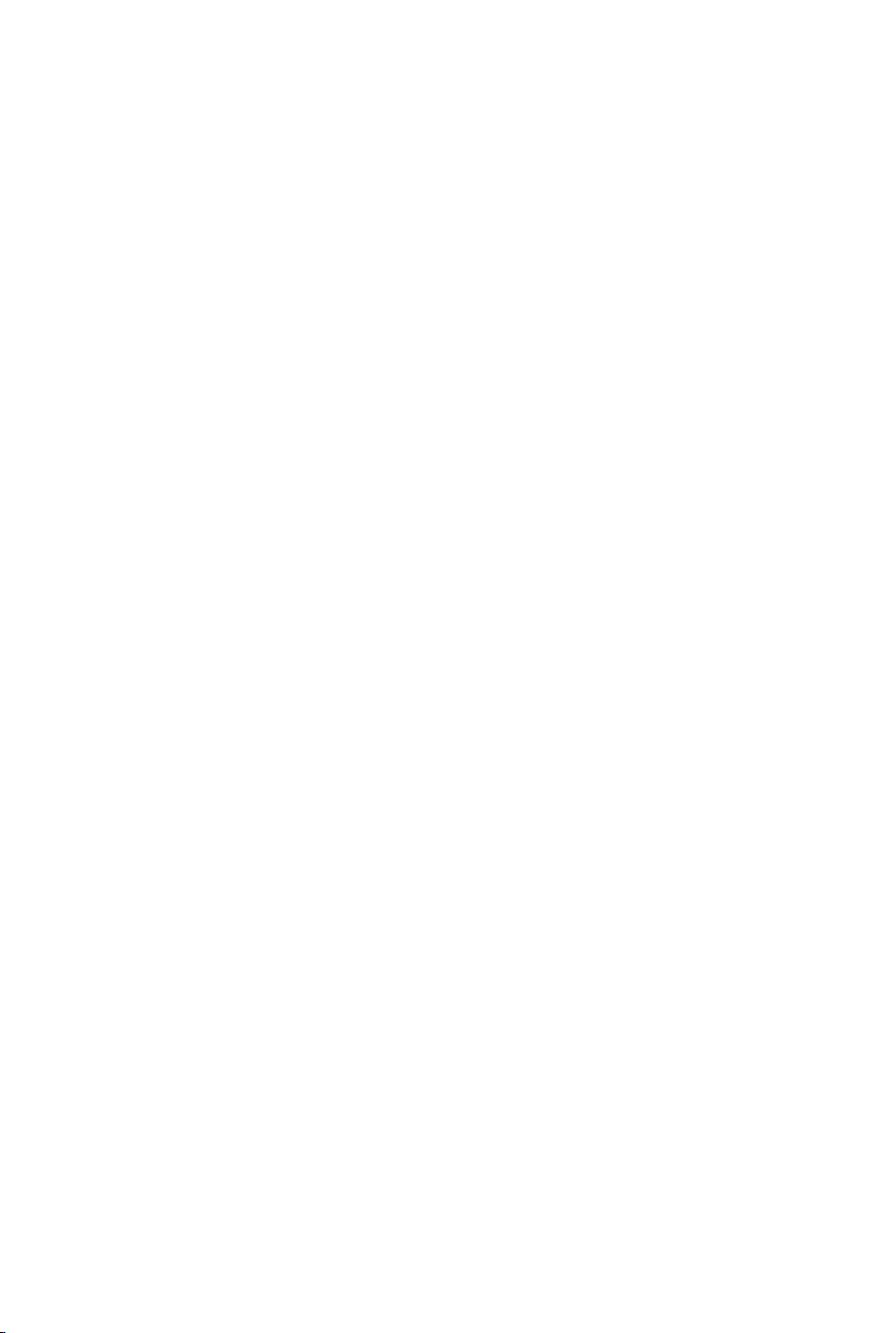
Clean Python: Elegant Coding in Python
ISBN-13 (pbk): 978-1-4842-4877-5 ISBN-13 (electronic): 978-1-4842-4878-2
https://doi.org/10.1007/978-1-4842-4878-2
Copyright © 2019 by Sunil Kapil
is work is subject to copyright. All rights are reserved by the Publisher, whether the whole or
part of the material is concerned, specically the rights of translation, reprinting, reuse of
illustrations, recitation, broadcasting, reproduction on microlms or in any other physical way,
and transmission or information storage and retrieval, electronic adaptation, computer software,
or by similar or dissimilar methodology now known or hereafter developed.
Trademarked names, logos, and images may appear in this book. Rather than use a trademark
symbol with every occurrence of a trademarked name, logo, or image we use the names, logos,
and images only in an editorial fashion and to the benet of the trademark owner, with no
intention of infringement of the trademark.
e use in this publication of trade names, trademarks, service marks, and similar terms, even if
they are not identied as such, is not to be taken as an expression of opinion as to whether or not
they are subject to proprietary rights.
While the advice and information in this book are believed to be true and accurate at the date of
publication, neither the authors nor the editors nor the publisher can accept any legal
responsibility for any errors or omissions that may be made. e publisher makes no warranty,
express or implied, with respect to the material contained herein.
Managing Director, Apress Media LLC: Welmoed Spahr
Acquisitions Editor: Nikhil Karkal
Development Editor: Rita Fernando
Coordinating Editor: Divya Modi
Cover designed by eStudioCalamar
Cover image designed by Freepik (www.freepik.com)
Distributed to the book trade worldwide by Springer Science+Business Media NewYork, 233
Spring Street, 6th Floor, NewYork, NY 10013. Phone 1-800-SPRINGER, fax (201) 348-4505, e-mail
orders-ny@springer-sbm.com, or visit www.springeronline.com. Apress Media, LLC is a
California LLC and the sole member (owner) is Springer Science + Business Media Finance Inc
(SSBM Finance Inc). SSBM Finance Inc is a Delaware corporation.
For information on translations, please e-mail rights@apress.com, or visit www.apress.com/
rights-permissions.
Apress titles may be purchased in bulk for academic, corporate, or promotional use. eBook
versions and licenses are also available for most titles. For more information, reference our Print
and eBook Bulk Sales web page at www.apress.com/bulk-sales.
Any source code or other supplementary material referenced by the author in this book is
available to readers on GitHub via the book’s product page, located at www.apress.
com/9781484248775. For more detailed information, please visit www.apress.com/source-code.
Printed on acid-free paper
SunilKapil
Sunnyvale, CA, USA
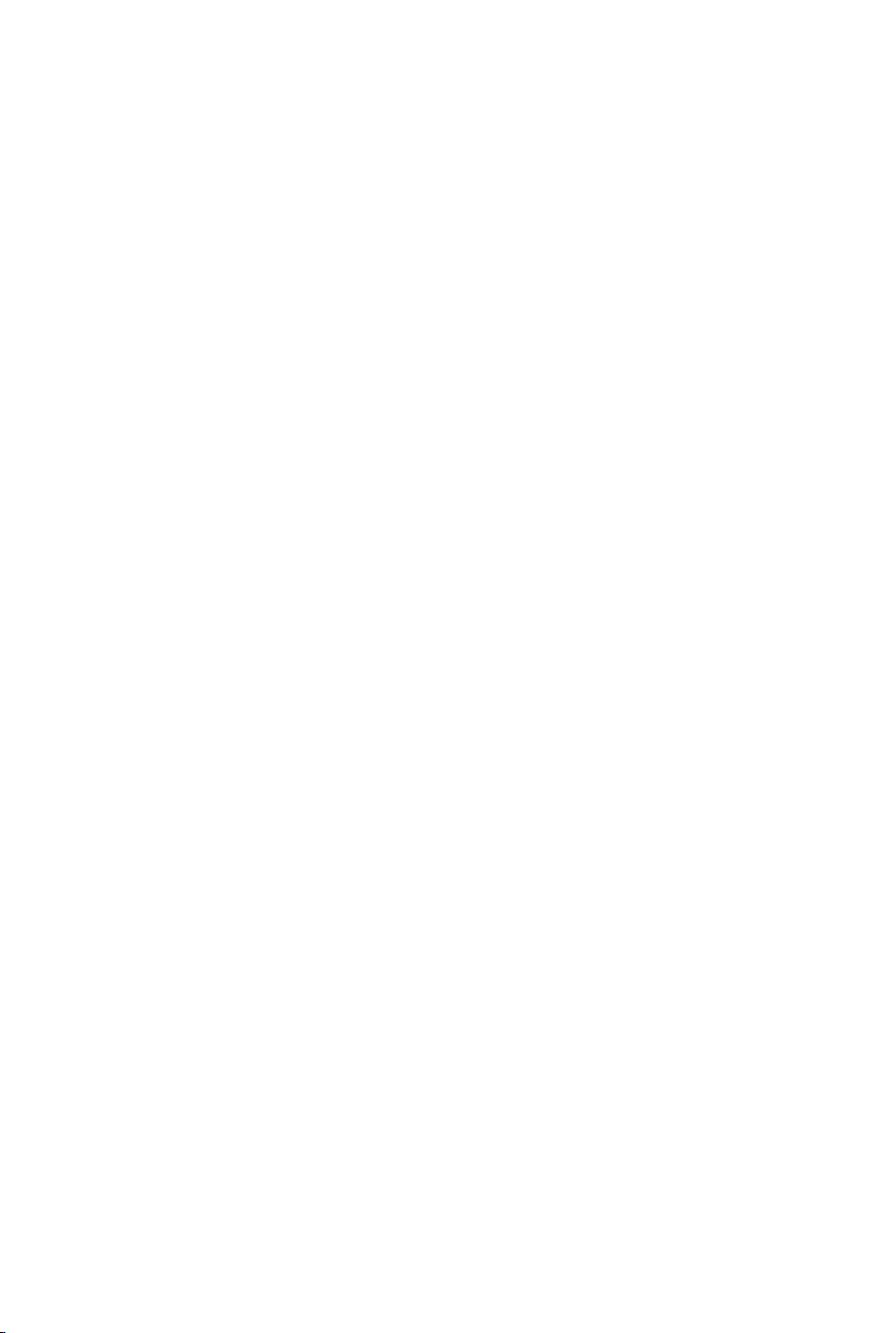
iii
Table of Contents
Chapter 1: Pythonic Thinking ���������������������������������������������������������������1
Write Pythonic Code ����������������������������������������������������������������������������������������������2
Naming ������������������������������������������������������������������������������������������������������������2
Expressions and Statements in Your Code ������������������������������������������������������6
Embrace the Pythonic Way to Write Code ��������������������������������������������������������9
Using Docstrings ������������������������������������������������������������������������������������������������� 18
Module-Level Docstrings �������������������������������������������������������������������������������21
Make the Class Docstring Descriptive ����������������������������������������������������������� 23
Function Docstrings ���������������������������������������������������������������������������������������24
Some Useful Docstring Tools �������������������������������������������������������������������������25
Write Pythonic Control Structures �����������������������������������������������������������������������26
Use List Comprehensions ������������������������������������������������������������������������������26
Don’t Make Complex List Comprehension ����������������������������������������������������� 28
Should You Use a Lambda? ���������������������������������������������������������������������������30
When to Use Generators vs� List Comprehension ������������������������������������������31
Why Not to Use else with Loops ��������������������������������������������������������������������32
Why range Is Better in Python 3 ��������������������������������������������������������������������35
About the Author ���������������������������������������������������������������������������������ix
About the Technical Reviewer �������������������������������������������������������������xi
Acknowledgments �����������������������������������������������������������������������������xiii
Introduction ����������������������������������������������������������������������������������������xv
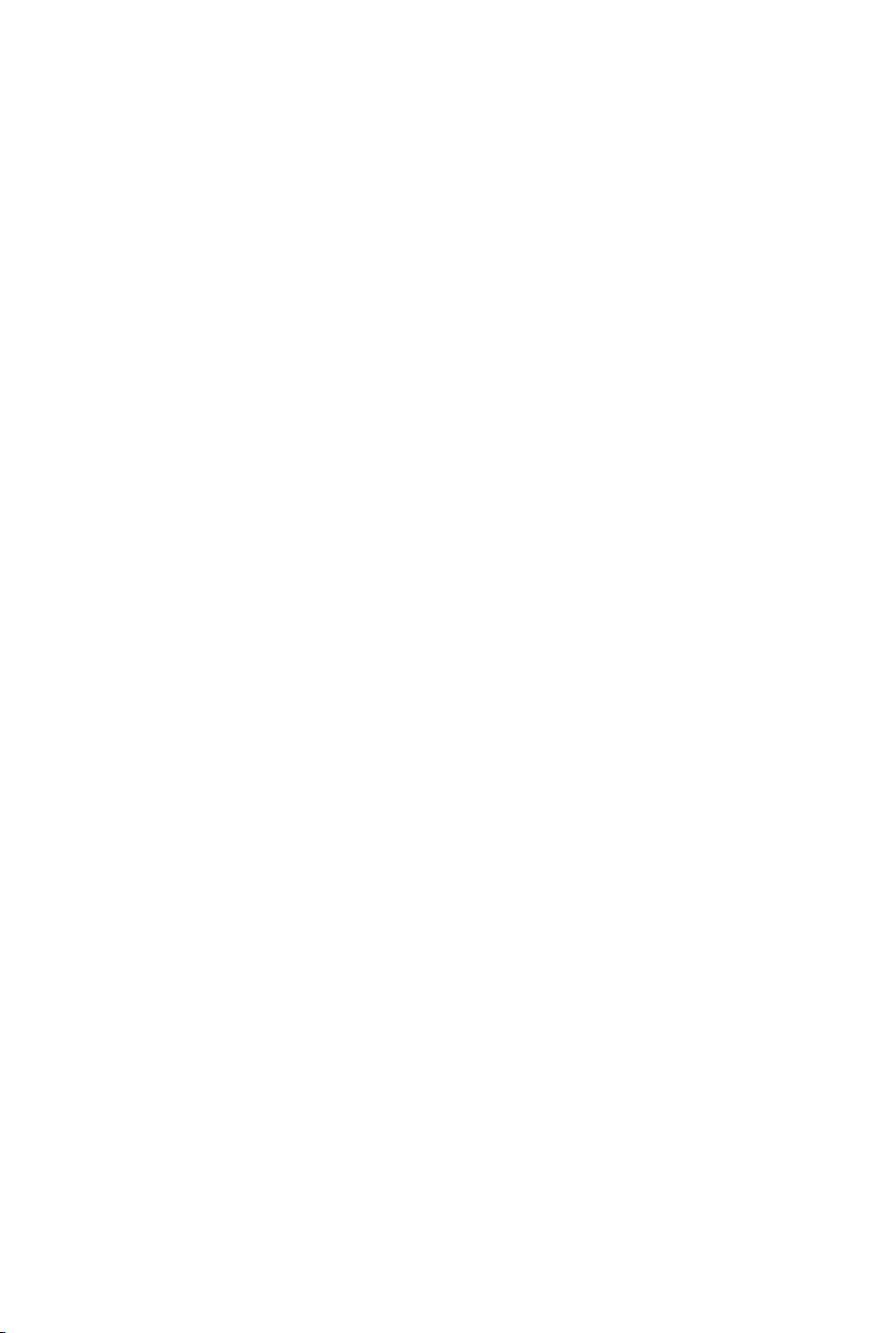
iv
Raising Exceptions ���������������������������������������������������������������������������������������������� 37
Frequently Raised Exceptions �����������������������������������������������������������������������37
Leverage nally to Handle Exceptions �����������������������������������������������������������39
Create Your Own Exception Class ������������������������������������������������������������������41
Handle Only Specic Exceptions �������������������������������������������������������������������43
Watch Out for Third-Party Exceptions ������������������������������������������������������������45
Prefer to Have Minimum Code Under try ������������������������������������������������������� 46
Summary�������������������������������������������������������������������������������������������������������������48
Chapter 2: Data Structures �����������������������������������������������������������������49
Common Data Structures ������������������������������������������������������������������������������������ 49
Use Sets for Speed ����������������������������������������������������������������������������������������50
Use namedtuple for Returning and Accessing Data ��������������������������������������52
Understanding str, Unicode, and byte ������������������������������������������������������������55
Use Lists Carefully and Prefer Generators ����������������������������������������������������� 57
Use zip to Process a List �������������������������������������������������������������������������������60
Take Advantage of Python’s Built-in Functions ���������������������������������������������62
Take Advantage of Dictionary ������������������������������������������������������������������������������65
When to Use a Dictionary vs� Other Data Structures ������������������������������������� 65
collections �����������������������������������������������������������������������������������������������������66
Ordered Dictionary vs� Default Dictionary vs� Normal Dictionary ������������������ 70
switch Statement Using Dictionary ���������������������������������������������������������������72
Ways to Merge Two Dictionaries��������������������������������������������������������������������73
Pretty Printing a Dictionary����������������������������������������������������������������������������74
Summary�������������������������������������������������������������������������������������������������������������75
Chapter 3: Writing Better Functions and Classes �������������������������������77
Functions ������������������������������������������������������������������������������������������������������������77
Create Small Functions ���������������������������������������������������������������������������������78
Return Generators �����������������������������������������������������������������������������������������80
Table of ConTenTsTable of ConTenTs
剩余273页未读,继续阅读
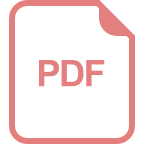
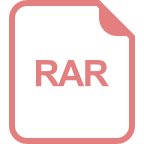

















weixin_44038680
- 粉丝: 0
- 资源: 3
上传资源 快速赚钱
我的内容管理 收起
我的资源 快来上传第一个资源
我的收益
登录查看自己的收益我的积分 登录查看自己的积分
我的C币 登录后查看C币余额
我的收藏
我的下载
下载帮助

会员权益专享
最新资源
- RTL8188FU-Linux-v5.7.4.2-36687.20200602.tar(20765).gz
- c++校园超市商品信息管理系统课程设计说明书(含源代码) (2).pdf
- 建筑供配电系统相关课件.pptx
- 企业管理规章制度及管理模式.doc
- vb打开摄像头.doc
- 云计算-可信计算中认证协议改进方案.pdf
- [详细完整版]单片机编程4.ppt
- c语言常用算法.pdf
- c++经典程序代码大全.pdf
- 单片机数字时钟资料.doc
- 11项目管理前沿1.0.pptx
- 基于ssm的“魅力”繁峙宣传网站的设计与实现论文.doc
- 智慧交通综合解决方案.pptx
- 建筑防潮设计-PowerPointPresentati.pptx
- SPC统计过程控制程序.pptx
- SPC统计方法基础知识.pptx
资源上传下载、课程学习等过程中有任何疑问或建议,欢迎提出宝贵意见哦~我们会及时处理!
点击此处反馈


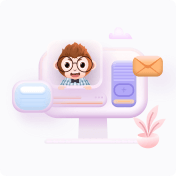
安全验证
文档复制为VIP权益,开通VIP直接复制

评论0