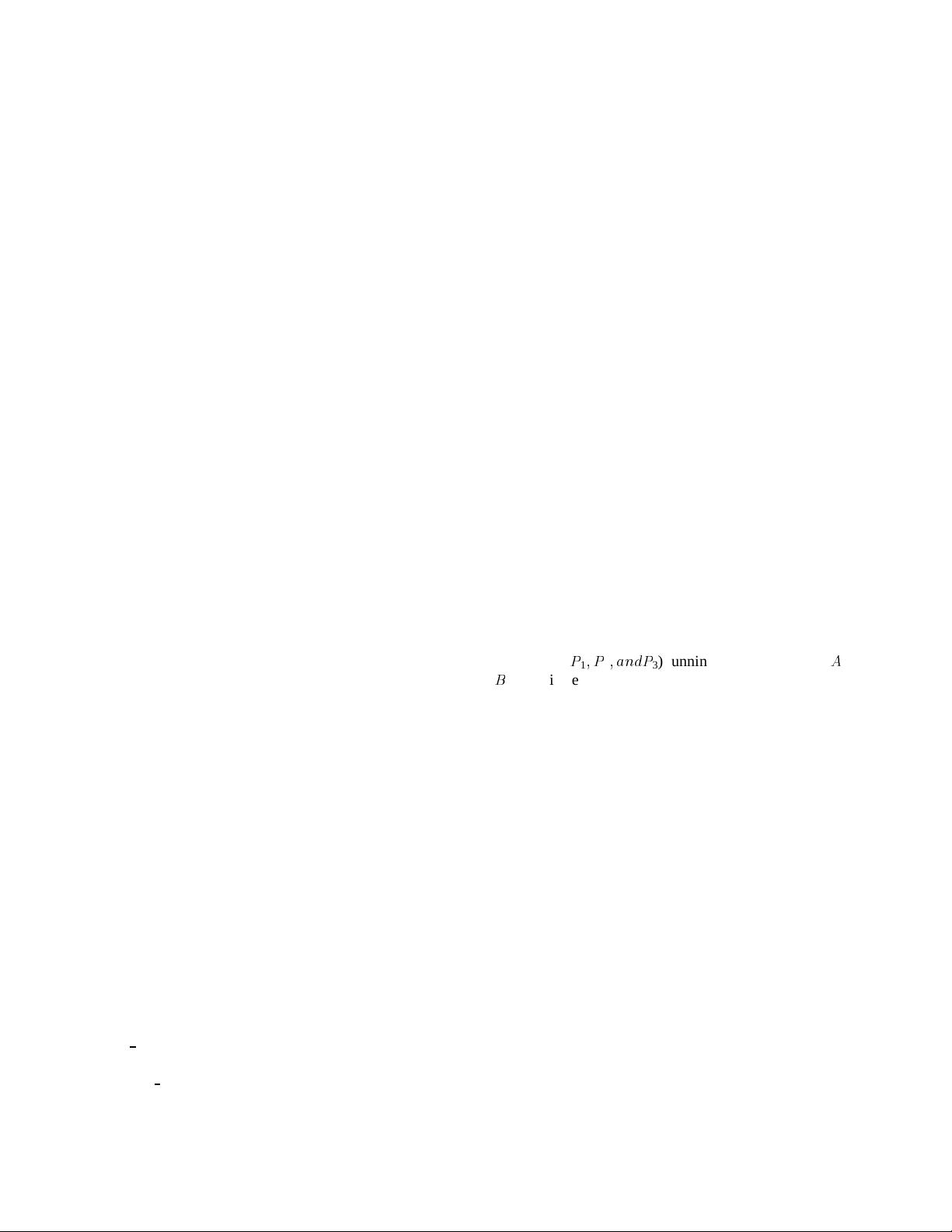
Systems Programming with C++ Wrappers
Encapsulating Interprocess Communication Mechanisms
with Object-Oriented Interfaces
Douglas C. Schmidt
schmidt@ics.uci.edu
Department of Information and Computer Science
University of California, Irvine, CA 92717, (714) 856-4105
An earlier version of this paper appeared in the Septem-
ber/October 1992 issue of the C++ Report.
1 Introduction
This article is the first in a series that describes techniques
for encapsulating operating system (OS) interprocess com-
munication (IPC) mechanisms within object-oriented (OO)
C++ wrappers. These OS mechanisms include support for
both local-host IPC (such as semaphores; message queues;
shared memory; memory-mapped files; named, unnamed,
and STREAM pipes; and BSD UNIX-domain sockets) and
network IPC (such as remote procedure calls (RPC); BSD
Internet-domain sockets; and the System V Transport Layer
Interface (TLI)). The primary motivations for using C++
wrappers are (1) to simplifythedevelopment of correct, con-
cise, portable, and efficient applications and (2) to facilitate
the introduction of object-oriented design (OOD) and C++
into software development organizations. In addition to de-
scribing C++ wrappers, this series of articles also clarifies
the semantics of several advanced UNIX and Windows NT
IPC mechanisms.
This introductory article motivates the concept of C++
wrappers, describes the limitations of existing OS interfaces
that wrappers help to overcome, outlines the topics that will
appear in subsequent articles, defines relevant networking
and IPC terms (see Table 1), and summarizes the advantages
and disadvantages of using C++ as a systems programming
language for applications that utilize IPC mechanisms. Sub-
sequent articles examine several IPC mechanisms that ben-
efit from object-oriented encapsulation. For instance, the
next article in this series describes the object-oriented de-
sign and implementation of a network IPC interface known
as IPC SAP, which encapsulates the local and remote IPC
mechanisms available in the BSD socket and System V TLI
APIs. IPC SAP is currently being used in the ADAPTIVE
system, which is a flexible development and evaluation en-
vironment for producing customized lightweight transport-
layer communication protocols [1].
2 Systems Programming and IPC
Systems software programs (such as databases, window-
ing systems, network file servers, compilers, linkers, ed-
itors, and device drivers) typically access and manipulate
operating system resources such as I/O controllers and in-
formation located in data structures residing within an OS
kernel. As distributedcomputing become more prevalent, an
increasingly important class of OS system mechanisms in-
volveinterprocess communication (IPC) withina singlehost,
as well as across networks and internetworks. IPC mecha-
nisms exchange different types of bytestream-oriented and
message-oriented data between processes that are executing
in different address spaces on the same and/or different host
machines. For example, Figure 1 illustrates a distributed
applicationthat utilizes both local and remote IPC in a multi-
level client/server manner. In this scenario, application pro-
cesses (e.g.,
P
1
;P
2
; andP
3
) runningon the client hosts
A
and
B
send discrete messages to a local client daemon via named
pipes. In turn, each client daemon forwards these messages
to the remote daemon on a designated server host across the
network via TCP stream connections. The server receives the
messages and displays them on one or more output devices
(such as a printer, persistent storage device, or monitoring
console). Subsequent articles will address this client/server
architecture in greater detail using the Reactor I/O-based
and timer-based service multiplexing facility [2, 3].
2.1 Limitations with Existing Operating Sys-
tem Interfaces
Developingcommunicationsystemsoftwareis difficultsince
it requires detailed knowledge of many concepts such as (1)
networkaddressingandremote serviceidentification,(2)cre-
ation, synchronization, and communication mechanisms for
processes and threads, (3) system call Application Program-
matic Interfaces (API)s for local and remote IPC, and (4)
presentation layer conversion techniques. Moreover, appli-
cations are often required to be efficient, functional, correct,
and portable across heterogeneous operating environments.
In addition, popular operating systems like UNIX, VMS,
and MVS were developed well before the advent of object-
oriented design and programming. This becomes evident
1