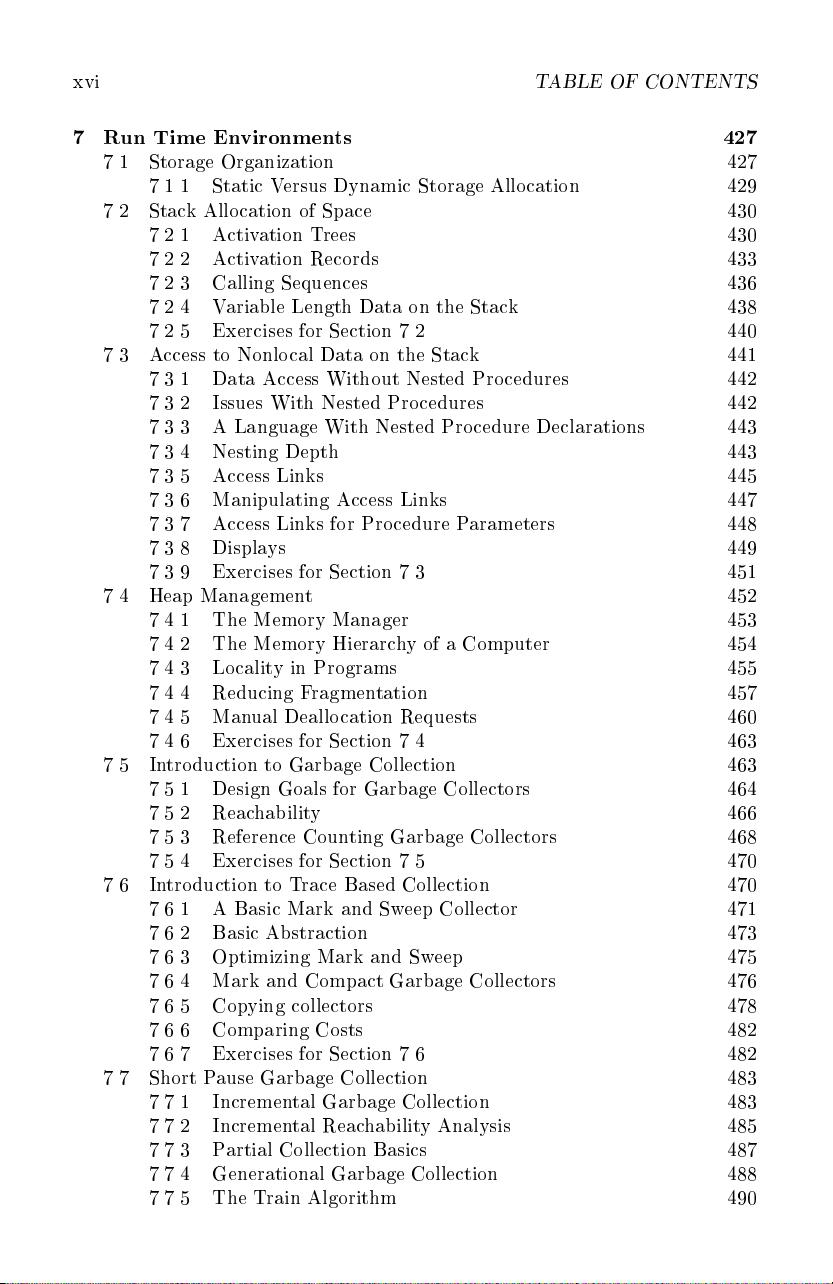
xvi
TABLE OF CONTENTS
7 Run-Time Environments 427
7.1 Storage Organization . . . . . . . . . . . . . . . . . . . . . . . . . 427
7.1.1 Static Versus Dynamic Storage Allo cation . . . . . . . . . 429
7.2 Stack Allo cation of Space . . . . . . . . . . . . . . . . . . . . . . 430
7.2.1 Activation Trees . . . . . . . . . . . . . . . . . . . . . . . 430
7.2.2 Activation Records . . . . . . . . . . . . . . . . . . . . . . 433
7.2.3 Calling Sequences . . . . . . . . . . . . . . . . . . . . . . 436
7.2.4 Variable-Length Data on the Stack . . . . . . . . . . . . . 438
7.2.5 Exercises for Section 7.2 . . . . . . . . . . . . . . . . . . . 440
7.3 Access to Nonlo cal Data on the Stack . . . . . . . . . . . . . . . 441
7.3.1 Data Access Without Nested Procedures . . . . . . . . . . 442
7.3.2 Issues With Nested Pro cedures . . . . . . . . . . . . . . . 442
7.3.3 A Language With Nested Pro cedure Declarations . . . . . 443
7.3.4 Nesting Depth . . . . . . . . . . . . . . . . . . . . . . . . 443
7.3.5 Access Links . . . . . . . . . . . . . . . . . . . . . . . . . 445
7.3.6 Manipulating Access Links . . . . . . . . . . . . . . . . . 447
7.3.7 Access Links for Pro cedure Parameters . . . . . . . . . . 448
7.3.8 Displays . . . . . . . . . . . . . . . . . . . . . . . . . . . . 449
7.3.9 Exercises for Section 7.3 . . . . . . . . . . . . . . . . . . . 451
7.4 Heap Management . . . . . . . . . . . . . . . . . . . . . . . . . . 452
7.4.1 The Memory Manager . . . . . . . . . . . . . . . . . . . . 453
7.4.2 The Memory Hierarchy of a Computer . . . . . . . . . . . 454
7.4.3 Locality in Programs . . . . . . . . . . . . . . . . . . . . . 455
7.4.4 Reducing Fragmentation . . . . . . . . . . . . . . . . . . . 457
7.4.5 Manual Deallo cation Requests . . . . . . . . . . . . . . . 460
7.4.6 Exercises for Section 7.4 . . . . . . . . . . . . . . . . . . . 463
7.5 Introduction to Garbage Collection . . . . . . . . . . . . . . . . . 463
7.5.1 Design Goals for Garbage Collectors . . . . . . . . . . . . 464
7.5.2 Reachability. . . . . . . . . . . . . . . . . . . . . . . . . . 466
7.5.3 Reference Counting Garbage Collectors . . . . . . . . . . 468
7.5.4 Exercises for Section 7.5 . . . . . . . . . . . . . . . . . . . 470
7.6 Introduction to Trace-Based Collection . . . . . . . . . . . . . . . 470
7.6.1 A Basic Mark-and-Sweep Collector . . . . . . . . . . . . . 471
7.6.2 Basic Abstraction . . . . . . . . . . . . . . . . . . . . . . 473
7.6.3 Optimizing Mark-and-Sweep . . . . . . . . . . . . . . . . 475
7.6.4 Mark-and-Compact Garbage Collectors . . . . . . . . . . 476
7.6.5 Copying collectors . . . . . . . . . . . . . . . . . . . . . . 478
7.6.6 Comparing Costs . . . . . . . . . . . . . . . . . . . . . . . 482
7.6.7 Exercises for Section 7.6 . . . . . . . . . . . . . . . . . . . 482
7.7 Short-Pause Garbage Collection . . . . . . . . . . . . . . . . . . . 483
7.7.1 Incremental Garbage Collection . . . . . . . . . . . . . . . 483
7.7.2 Incremental Reachability Analysis . . . . . . . . . . . . . 485
7.7.3 Partial-Collection Basics . . . . . . . . . . . . . . . . . . . 487
7.7.4 Generational Garbage Collection . . . . . . . . . . . . . . 488
7.7.5 The Train Algorithm . . . . . . . . . . . . . . . . . . . . . 490