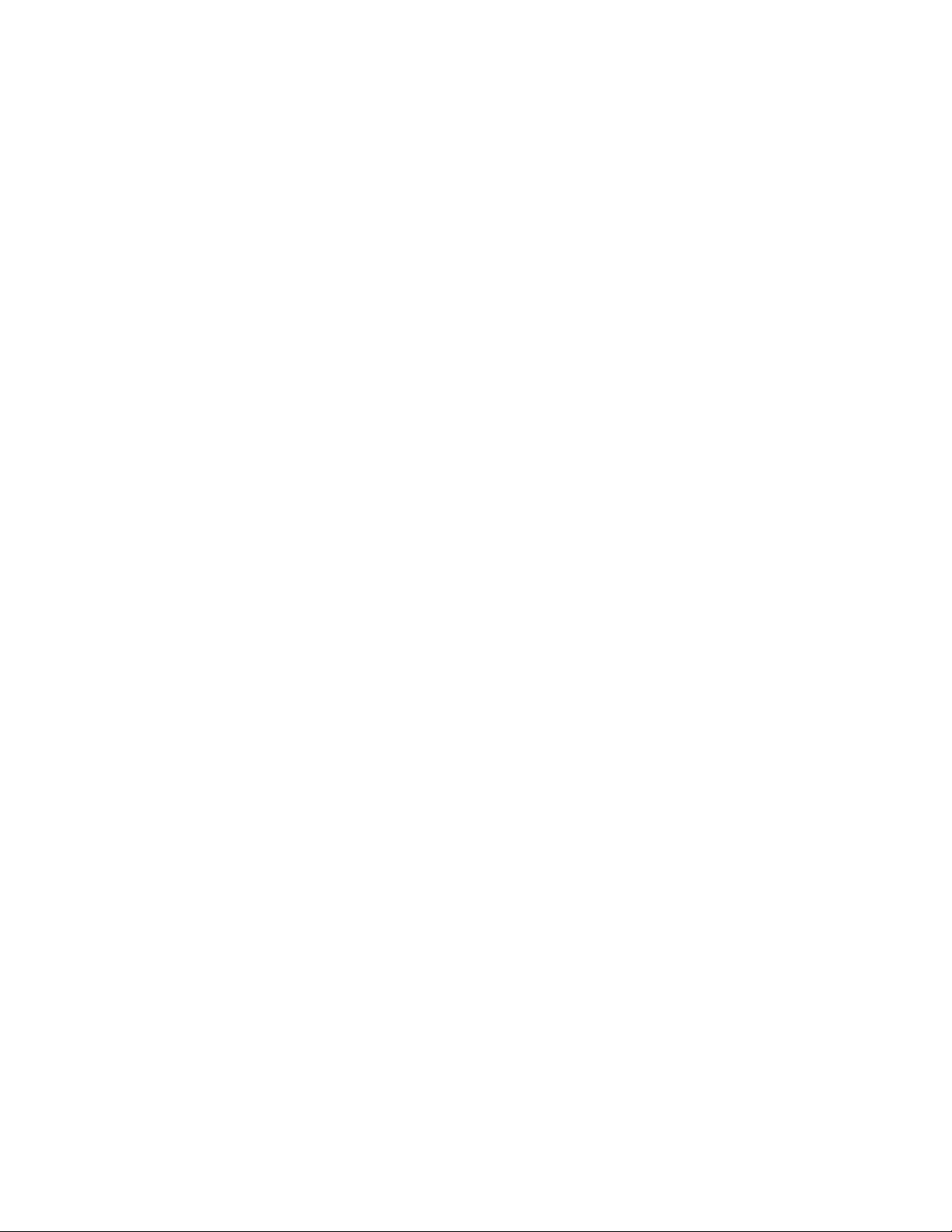
x GAWK: Effective AWK Programming
15 Namespaces in gawk . . . . . . . . . . . . . . . . . . . . . . . . . 359
15.1 Standard awk’s Single Namespace. . . . . . . . . . . . . . . . . . . . . . . . . . . . 359
15.2 Qualified Names. . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 359
15.3 The Default Namespace . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 359
15.4 Changing The Namespace . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 360
15.5 Namespace and Component Naming Rules. . . . . . . . . . . . . . . . . . . 360
15.6 Internal Name Management . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 361
15.7 Namespace Example . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 362
15.8 Namespaces and Other gawk Features . . . . . . . . . . . . . . . . . . . . . . . 363
15.9 Summary . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 364
16 Arithmetic and Arbitrary-Precision
Arithmetic with gawk . . . . . . . . . . . . . . . . . . . . . . . . . . 365
16.1 A General Description of Computer Arithmetic . . . . . . . . . . . . . . 365
16.2 Other Stuff to Know . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 366
16.3 Arbitrary-Precision Arithmetic Features in gawk . . . . . . . . . . . . . 368
16.4 Floating-Point Arithmetic: Caveat Emptor! . . . . . . . . . . . . . . . . . . 368
16.4.1 Floating-Point Arithmetic Is Not Exact . . . . . . . . . . . . . . . . . 369
16.4.1.1 Many Numbers Cannot Be Represented Exactly. . . . 369
16.4.1.2 Be Careful Comparing Values . . . . . . . . . . . . . . . . . . . . . . 369
16.4.1.3 Errors Accumulate . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 370
16.4.2 Getting the Accuracy You Need . . . . . . . . . . . . . . . . . . . . . . . . 371
16.4.3 Try a Few Extra Bits of Precision and Rounding . . . . . . . . 371
16.4.4 Setting the Precision . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 372
16.4.5 Setting the Rounding Mode . . . . . . . . . . . . . . . . . . . . . . . . . . . . 373
16.5 Arbitrary-Precision Integer Arithmetic with gawk . . . . . . . . . . . . 375
16.6 How To Check If MPFR Is Available . . . . . . . . . . . . . . . . . . . . . . . . 376
16.7 Standards Versus Existing Practice . . . . . . . . . . . . . . . . . . . . . . . . . . 376
16.8 Summary . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 378
17 Writing Extensions for gawk. . . . . . . . . . . . . . . . . 379
17.1 Introduction . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 379
17.2 Extension Licensing . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 379
17.3 How It Works at a High Level . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 379
17.4 API Description . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 381
17.4.1 Introduction. . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 381
17.4.2 General-Purpose Data Types . . . . . . . . . . . . . . . . . . . . . . . . . . . 383
17.4.3 Memory Allocation Functions and Convenience Macros . . 387
17.4.4 Constructor Functions . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 388
17.4.5 Registration Functions. . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 390
17.4.5.1 Registering An Extension Function . . . . . . . . . . . . . . . . 390
17.4.5.2 Registering An Exit Callback Function. . . . . . . . . . . . . 392
17.4.5.3 Registering An Extension Version String . . . . . . . . . . . 392
17.4.5.4 Customized Input Parsers . . . . . . . . . . . . . . . . . . . . . . . . . 392
17.4.5.5 Customized Output Wrappers . . . . . . . . . . . . . . . . . . . . . 397
17.4.5.6 Customized Two-way Processors . . . . . . . . . . . . . . . . . . . 399
17.4.6 Printing Messages . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 399