C语言权威指南:《C Programming Language》第二版中文版
"《C Programming Language》第二版中文版,由C语言的设计者Brian W. Kernighan和Dennis M. Ritchie合著,是一部详细介绍标准C语言和程序设计的经典著作。书中涵盖C语言的基本概念、类型和表达式、控制流、函数、指针与数组、结构、输入与输出、UNIX系统接口以及标准库等内容。" C语言是计算机编程的基础,由Brian W. Kernighan和Dennis M. Ritchie共同开发并由他们编写的这本书,被广泛认为是学习C语言的权威指南。书中深入浅出的解释和丰富的示例使得复杂的编程概念变得易于理解,适合初学者和有经验的程序员。 1. **基本概念**:C语言的基础知识,包括变量、常量、数据类型(如整型、浮点型、字符型等)、运算符和表达式,以及声明和初始化。 2. **类型和表达式**:详细阐述了C语言中的不同类型,如基本类型、枚举、结构体和联合体,以及表达式的计算规则和类型转换。 3. **控制流**:讲解了条件语句(如if-else)、循环(如for、while、do-while)以及跳转语句(如break、continue)等控制程序执行流程的结构。 4. **函数**:介绍了函数的定义、调用、参数传递和返回值,以及函数指针,展示了如何组织和重用代码。 5. **指针与数组**:深入讨论了指针的概念,包括指针的声明、赋值、解引用和指针运算,以及指针与数组的关系,如何通过指针操作数组。 6. **结构**:讲解了如何定义和使用结构体,将不同类型的数据组合成一个复合类型,以及结构体指针的应用。 7. **输入与输出**:介绍了标准输入输出函数(如printf和scanf),以及文件I/O操作,如何读写文件。 8. **UNIX系统接口**:由于C语言在UNIX系统中起着核心作用,本书还涉及了UNIX系统调用,如系统内存管理、进程控制和文件系统操作。 9. **标准库**:涵盖了C标准库中的常用函数,如数学函数、字符串处理函数、内存管理函数等,并讨论了如何利用这些库来扩展程序功能。 这本书不仅是C语言学习者的必备参考资料,也是软件开发者的重要工具书。通过阅读和实践书中给出的示例,读者可以掌握C语言的核心概念和编程技巧,为进一步的系统级编程和软件开发打下坚实基础。
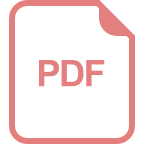
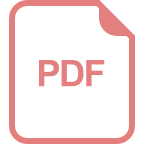

















- 粉丝: 1
- 资源: 17
我的内容管理 展开
我的资源 快来上传第一个资源
我的收益
登录查看自己的收益我的积分 登录查看自己的积分
我的C币 登录后查看C币余额
我的收藏
我的下载
下载帮助

最新资源
- Vue实现iOS原生Picker组件:详细解析与实现思路
- Arduino蓝牙小车:参数调试与功能控制
- 百度Java面试精华:200页精选资源涵盖核心知识点
- Swift使用CoreData填坑指南:CoreData在Swift 3.0的变化
- 微距离无线充电器创新设计及其实验探索
- MTK Android平台开发全攻略:44步详解流程
- RecyclerView全面解析:替代ListView的新选择
- Android开发:自动适配中英文键盘解决方案
- Android调用WebService接口教程
- Android开发:BitmapUtil图片处理全解析与实例
- Android多线程断点续传实现详解
- PCA算法在人脸识别会议签到系统中的应用
- EventBus 3.0:Android事件总线详解与实战应用
- Android FileUtil:全面解析文件操作实用技巧与实例
- RecyclerView添加头部和尾部实战教程
- Android实现微博滑动固定顶部栏实战与优化

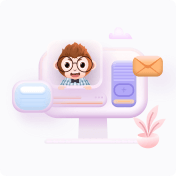
