使用Python创作你的电脑游戏
"Invent Your Own Computer Games with Python 2nd" 是一本针对初学者的Python编程入门书籍,作者是Albert Sweigart。该书基于创作共用 Attribution-Noncommercial-Share Alike 3.0 United States License 发布,允许读者自由复制、分发、展示和演绎作品,但必须署名、非商业使用,并在创作新作品时遵循相同或类似的许可条件。 本书主要面向对计算机游戏编程感兴趣的初学者,通过Python语言教授如何创建自己的游戏。Python是一种高级编程语言,因其简洁易读的语法而广受欢迎,特别适合初学者学习。书中的内容可能包括基础编程概念、数据结构、控制流、函数以及面向对象编程等基础知识,同时结合游戏开发的实际案例,帮助读者将所学应用于实践。 在书中,读者可以期待学习到以下核心知识点: 1. **Python基础**:了解Python的基本语法,如变量、数据类型(如整型、浮点型、字符串)、运算符、控制结构(如if语句、for循环、while循环)以及函数定义与调用。 2. **游戏编程基础**:学习如何在Python环境中设置游戏框架,例如使用Python的Turtle库创建简单的图形界面,或者使用Pygame库进行更复杂的游戏开发。 3. **事件驱动编程**:掌握如何处理用户输入和游戏循环,以及如何响应游戏中的各种事件,如键盘输入、鼠标点击等。 4. **数据结构与算法**:理解列表、字典等Python内置数据结构,学习如何有效地存储和操作数据,以及如何使用算法来解决游戏中的问题,如碰撞检测、路径规划等。 5. **面向对象编程**:学习如何定义类和对象,理解封装、继承和多态的概念,以及如何利用这些特性构建可重用的游戏组件。 6. **游戏逻辑与规则**:设计并实现游戏规则,如玩家得分系统、敌人行为、关卡设计等,通过编程将游戏的逻辑清晰地表达出来。 7. **调试与测试**:学习如何调试代码,找出并修复程序中的错误,以及如何编写测试用例以确保游戏功能的正确性。 8. **优化与改进**:了解性能优化技巧,以及如何根据反馈改进游戏体验,如提高游戏性能、调整难度平衡等。 通过阅读和实践这本书中的示例,读者不仅可以掌握Python编程,还能建立起游戏开发的基本技能,为今后进一步深入学习游戏开发打下坚实基础。此外,由于书籍强调动手实践,读者将有机会在实际项目中运用所学,从而加深对编程概念的理解。
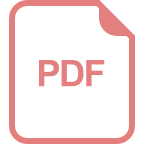
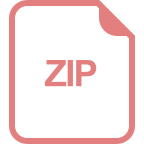
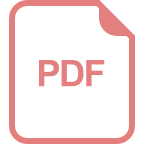












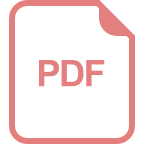
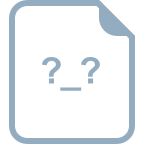
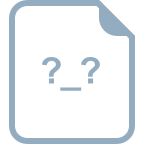
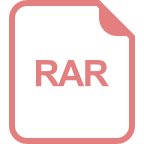

- 粉丝: 1
- 资源: 1
我的内容管理 展开
我的资源 快来上传第一个资源
我的收益
登录查看自己的收益我的积分 登录查看自己的积分
我的C币 登录后查看C币余额
我的收藏
我的下载
下载帮助

最新资源
- OptiX传输试题与SDH基础知识
- C++Builder函数详解与应用
- Linux shell (bash) 文件与字符串比较运算符详解
- Adam Gawne-Cain解读英文版WKT格式与常见投影标准
- dos命令详解:基础操作与网络测试必备
- Windows 蓝屏代码解析与处理指南
- PSoC CY8C24533在电动自行车控制器设计中的应用
- PHP整合FCKeditor网页编辑器教程
- Java Swing计算器源码示例:初学者入门教程
- Eclipse平台上的可视化开发:使用VEP与SWT
- 软件工程CASE工具实践指南
- AIX LVM详解:网络存储架构与管理
- 递归算法解析:文件系统、XML与树图
- 使用Struts2与MySQL构建Web登录验证教程
- PHP5 CLI模式:用PHP编写Shell脚本教程
- MyBatis与Spring完美整合:1.0.0-RC3详解

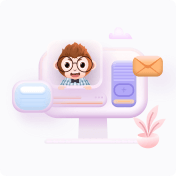
