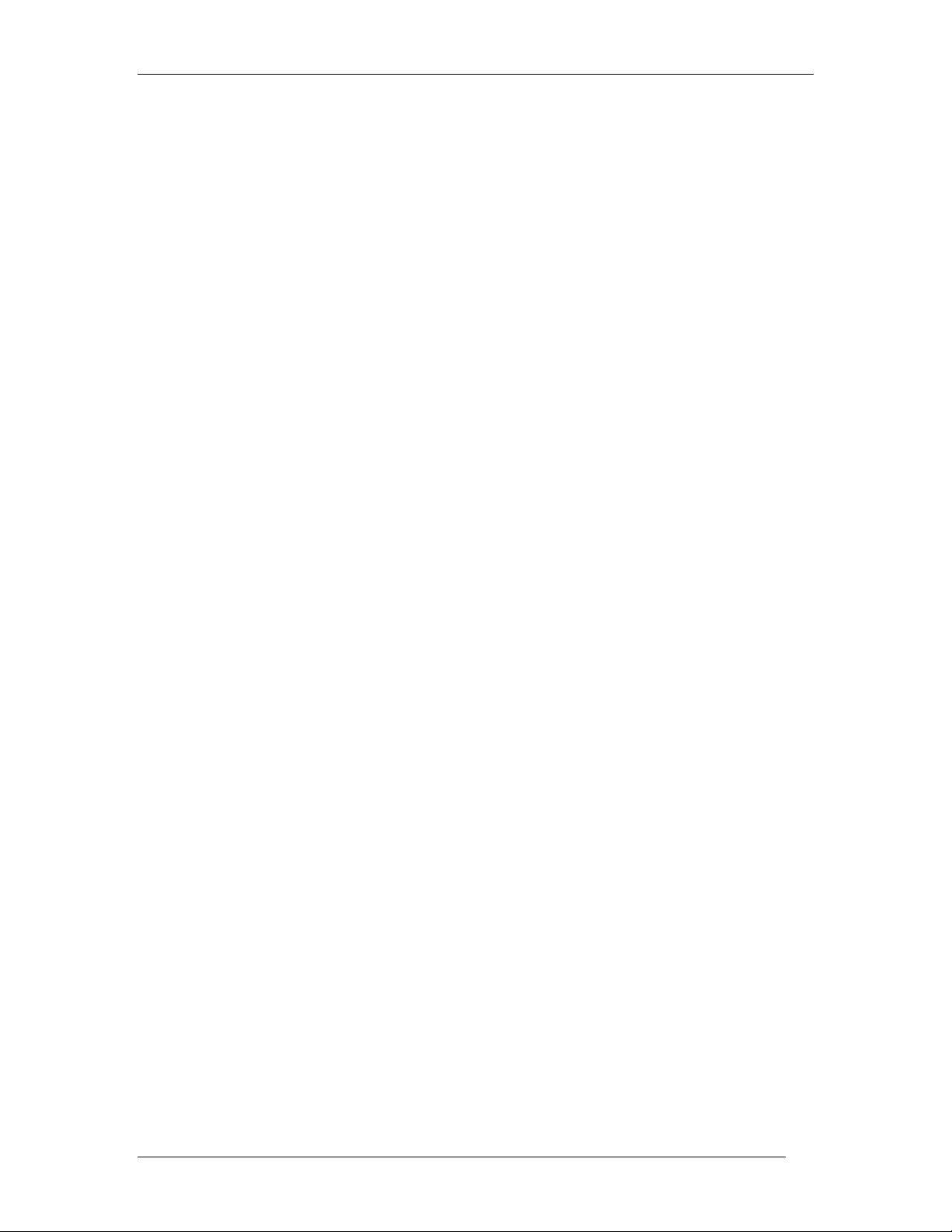
The C++ Standard Library
dyne-book
15
Templates are functions or classes that are written for one or more types not yet specified. When
you use a template, you pass the types as arguments, explicitly or implicitly. The following is a
typical example — a function that returns the maximum of two values:
template <class T>
inline const T& max (const T& a, const T& b)
{
// if a <b then use b else use a
return a < b ? b : a;
}
Here, the first line defines T as an arbitrary data type that is specified by the caller when the caller
calls the function. You can use any identifier as a parameter name, but using T is very common, if
not a de facto convention. The type is classified by class, although it does not have to be a
class. You can use any data type as long as it provides the operations that the template uses.
[3]
[3]
class was used here to avoid the introduction of a new keyword when templates were introduced.
However, now there is a new keyword, typename, that you can also use here (see page 11).
Following the same principle, you can "parameterize" classes on arbitrary types. This is useful for
container classes. You can implement the container operations for an arbitrary element type. The
C++ standard library provides many template container classes (for example, see Chapter 6 or
Chapter 10). It also uses template classes for many other reasons. For example, the string
classes are parameterized on the type of the characters and the properties of the character set
(see Chapter 11).
A template is not compiled once to generate code usable for any type; instead, it is compiled for
each type or combination of types for which it is used. This leads to an important problem in the
handling of templates in practice: You must have the implementation of a template function
available when you call it, so that you can compile the function for your specific type. Therefore,
the only portable way of using templates at the moment is to implement them in header files by
using inline functions.
[4]
[4]
To avoid the problem of templates having to be present in header files, the standard introduced a
template compilation model with the keyword export. However, I have not seen it implemented yet.
The full functionality of the C++ standard library requires not only the support of templates in
general, but also many new standardized template features, including those discussed in the
following paragraphs.
Nontype Template Parameters
In addition to type parameters, it is also possible to use nontype parameters. A nontype
parameter is then considered as part of the type. For example, for the standard class bitset<>
(class bitset<> is introduced in Section 10.4,) you can pass the number of bits as the
template argument. The following statements define two bitfields, one with 32 bits and one with
50 bits:
bitset<32> fIags32; // bitset with 32 bits
bitset<50> flags50; // bitset with 50 bits
These bitsets have different types because they use different template arguments. Thus, you
can't assign or compare them (except if a corresponding type conversion is provided).
Default Template Parameters