没有合适的资源?快使用搜索试试~ 我知道了~
首页Java数据库编程深入解析
Java数据库编程深入解析
需积分: 10 5 下载量 152 浏览量
更新于2024-08-02
收藏 1.6MB PDF 举报
"《java 数据库编程》是一本深度探讨Java与数据库交互的教材,全面讲解了如何在Java环境中进行数据库操作。本书适合学习、编码和设计数据库应用的读者使用,书中涵盖了各种软件版本和Java数据库连接(JDBC)的相关知识,同时也介绍了企业级开发中的Java应用和关系型数据库的基本概念。"
在Java世界中,数据库编程是一个至关重要的领域,特别是在企业级应用开发中。本书首先介绍了企业在信息技术时代所扮演的角色,强调了Java作为企业级开发工具的优势。数据库,尤其是关系型数据库,是存储和管理大量数据的核心,因此理解数据库的基础知识至关重要。书中通过第2章详细阐述了什么是关系型数据库以及SQL语言的基本概念,帮助读者建立对数据库管理和查询语言的基础理解。
进入JDBC(Java Database Connectivity)的学习,这是Java与各种数据库交互的标准化接口。第3章介绍了JDBC的基础,包括JDBC是什么,如何连接数据库,处理连接问题,执行基本的数据库访问操作,以及Java数据类型与SQL数据类型的映射。此外,还讨论了滚动结果集和JDBC支持类的作用,最后展示了如何在Servlet中实现数据库操作。
第4章深入JDBC,探讨了预编译SQL语句(PreparedStatement)的使用,分析在不同场景下选择合适的Statement类型,介绍了批处理功能,使读者能够高效地处理多条数据库操作。此外,还涉及了可更新的结果集和处理复杂数据类型的技巧。
第5章则将焦点转向JDBC可选包,特别是数据源和连接池的概念,这些在大型企业应用中用于优化性能和资源管理。数据源提供了一种更有效、更安全的方式来获取数据库连接,而连接池则允许重复使用已建立的连接,减少创建和关闭连接的开销。
《java 数据库编程》是学习Java数据库编程的宝贵资源,它不仅讲解了基础理论,也涵盖了高级特性,适合从初学者到经验丰富的开发者阅读,帮助他们在实际项目中更好地运用Java进行数据库操作。
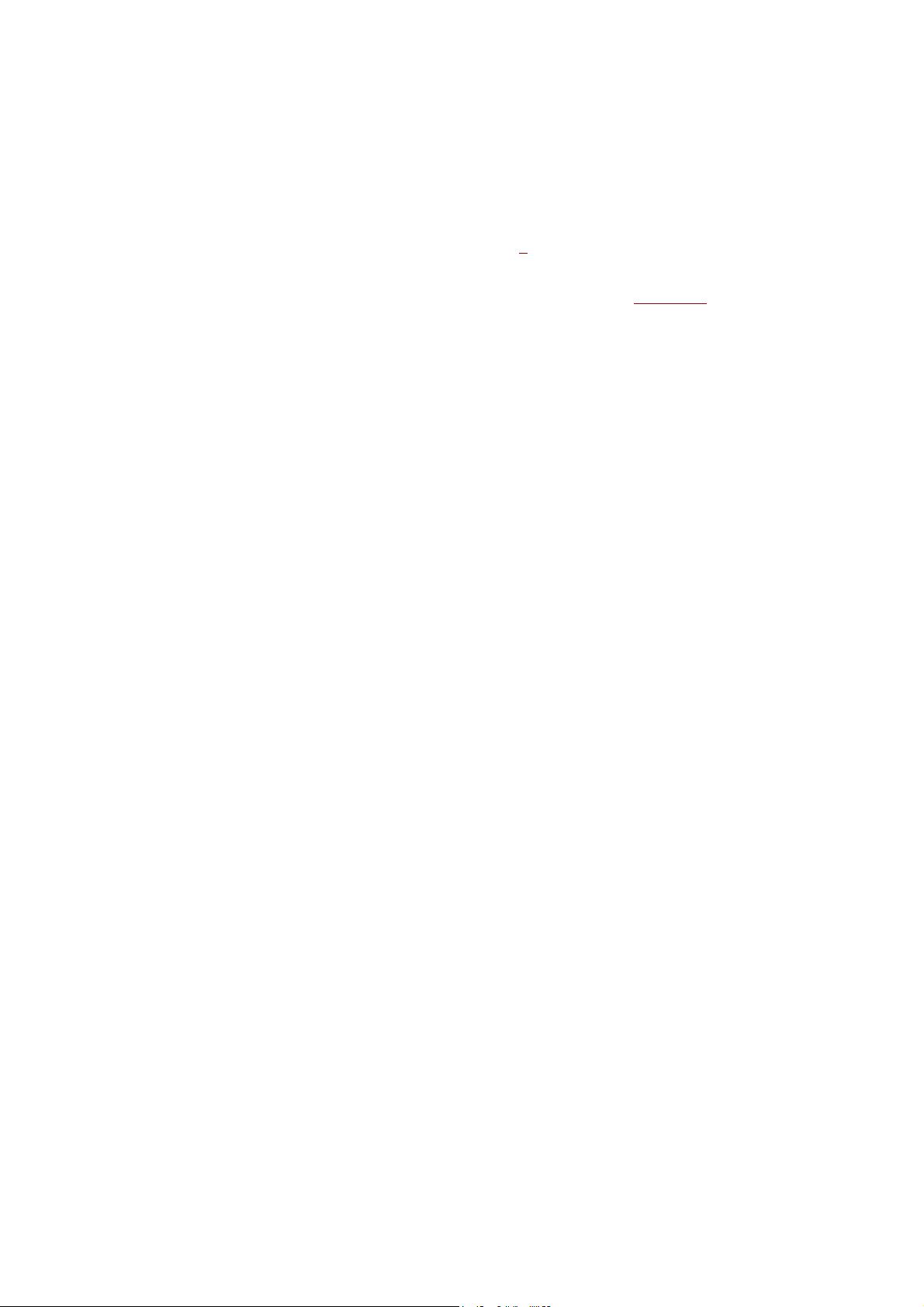
JDBC and Java 2
nd
edition
p
age 13
transaction management. Building on this foundation, we will discuss JDBC and how it can be used
to execute SQL against any potential database engine.
1.3.1.1 SQL
The Java database API, JDBC, requires that the database being used support ANSI SQL2 as the
query language. The SQL language itself is worthy of a tiny mini-industry within the publishing
field, so covering it is well beyond the scope of this book.
[1]
The SQL in this book, however, stays
away from the more complex areas of the language and instead sticks with basic DELETE, INSERT,
SELECT, and UPDATE statements. For a short overview of SQL, check out Chapter 2.
[1]
O'Reilly is publishing a SQL reference guide, SQL in a Nutshell, by Kevin Kline with Daniel Kline.
The only additional level of complexity I use consists of stored procedures in the later chapters.
Stored procedures are precompiled SQL stored on the database server and executed by naming the
procedure and passing parameters to it. In other words, a stored procedure is much like a database
server function. Stored procedures provide an easy mechanism for separating Java programmers
from database issues and improving database performance.
1.3.1.2 JDBC
JDBC is in a SQL-level API that allows you to embed SQL statements as arguments to methods in
JDBC interfaces. To enable you to do this in a database-independent fashion, JDBC requires
database vendors (such as those mentioned earlier in this chapter) to furnish a runtime
implementation of its interfaces. These implementations route your SQL calls to the database in the
proprietary fashion it recognizes. As the programmer, though, you do not ever have to worry about
how it is routing SQL statements. The façade provided by JDBC gives you complete freedom from
any issues related to particular database issues; you can run the same code no matter what database
is present.
1.3.1.3 Transaction management
Transaction management involves packaging related database transactions into a single unit and
handling any error conditions that result. To get through this book, you need only to understand
basic transaction management in the form of beginning a transaction and either committing it on
success or aborting it on failure. JDBC provides you with the ability to auto-commit any transaction
on the atomic level (that is, statement by statement) or wait until a series of statements have
succeeded (or failed) and call the appropriate commit (or rollback) method.
1.3.2 Database Technologies
A Java application can use one of three major database architectures:
• Relational database
• Object database
• Object-relational database
The overwhelming majority of today's database applications use relational databases. The JDBC
API is thus heavily biased toward relational databases and their standard query language, SQL.
Relational databases find and provide relationships between data, so they collide head-on with
object solutions such as Java, since object-oriented philosophy dictates that an object's behavior is
inseparable from its data. In choosing the object-oriented reality of Java, you need to create a
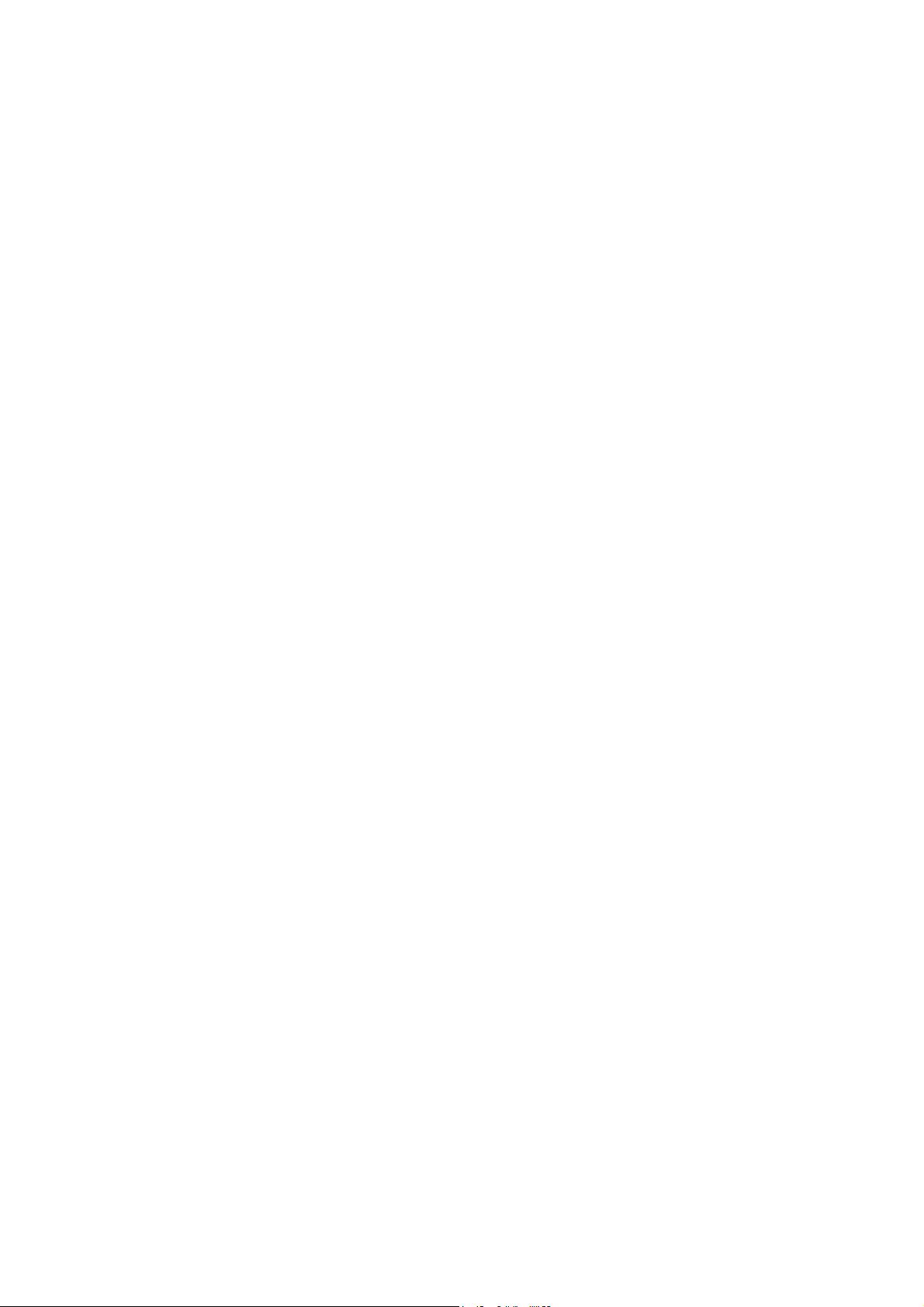
JDBC and Java 2
nd
edition
p
age 14
translation layer that maps the relational world into your object world. While JDBC provides you
with access to relational databases, it leaves the issue of object-to-relational mapping up to you.
Object databases, on the other hand, do not attempt to separate object data from behavior. The best
way to think of an object database is as a permanent store of objects with which your applications
can interface. This object-oriented encapsulation of data, however, makes it difficult to relate data
as well as relational databases do. Additionally, with JDBC so tightly bound to SQL, it is difficult
to create JDBC drivers to run against an object database. As of the writing of this book, Sun, in
cooperation with the Object Database Management Group (ODMG), is working on a specification
for a Java object database API.
Object-relational databases enjoy a "best of both worlds" advantage by providing both object and
relational means of accessing data. Until recently, object relational databases have relied almost
entirely on C++ objects to act as their object store. With all of the excitement around Java, however,
object-relational vendors are starting to enable their systems to support database objects written and
extended in Java. In this realm, your Java objects do not need to map relational data into business
objects. For the sake of easy, ad hoc querying, however, an object-relational database also provides
complex relational queries; sometimes these queries can even be done in an ANSI SQL superset
language.
1.4 Database Programming with Java
While the marriage of Java and database programming is beneficial to Java programmers, Java also
helps database programmers. Specifically, Java provides database programmers with the following
features they have traditionally lacked:
• Easy object to relational mapping
• Database independence
• Distributed computing
If you are interested in taking a pure object approach to systems development, you may have run
into the cold reality that most of the world runs on relational databases into which companies have
often placed hefty investments. This leaves you trying to map C++ and Smalltalk objects to
relational entities. Java provides an alternative to these two tools that frees you from the proprietary
interfaces associated with database programming. With the "write once, compile once, run
anywhere" power that JDBC offers you, Java's database connectivity allows you to worry about the
translation of relational data into objects instead of worrying about how you are getting that data.
A Java database application does not care what its database engine is. No matter how many times
the database engine changes, the application itself need never change. In addition, a company can
build a class library that maps its business objects to database entities in such a way that
applications do not even know whether or not their objects are being stored in a database. Later in
the book I discuss building a class library that allows you to map the data you retrieve through the
JDBC API into Java objects.
Java affects the way you distribute and maintain an application. A traditional client/server
application requires an administrator responsible for the deployment of the client program on users'
desktops. That administrator takes great pains to assure that each desktop provides a similar
operating environment so that the application may run as it was intended to run. When a change is
made to the application, the administrator makes the rounds and installs the upgrade.
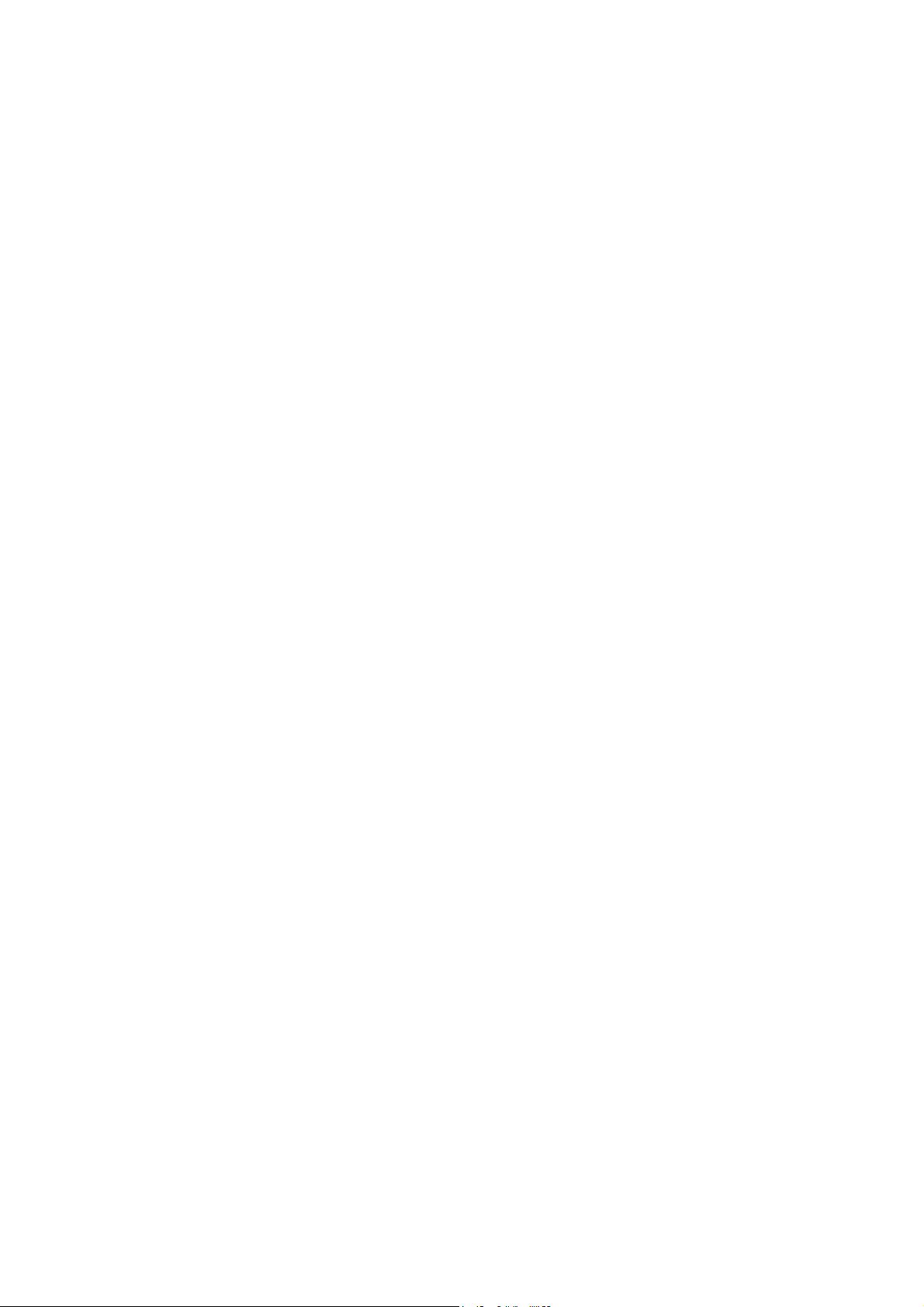
JDBC and Java 2
nd
edition
p
age 15
The Java language employs the idea of the zero-install client. The object code for the entire
application, client and server, resides on the network. Since the JVM provides an application with a
guaranteed runtime environment, no administration is needed for the configuration of that
environment for individual applications. The users simply use a virtual machine interface such as
HotJava to locate the desired application. By clicking on the application icon, a user can run it
without even realizing the application was never stored on their local machine.
The traditional application makes a clear distinction between the locations where processing occurs.
In traditional applications, database access occurs on the server, and GUI processing occurs on the
client; the objects on the client machine talk to the database through a specialized database API. In
other situations, the client might talk to the server through a set of TCP/IP or UDP/IP socket APIs.
Either way, a wall of complex protocols divides the objects found on the client from those on the
server. Java helps tear down this wall between client and server through another piece of its
Enterprise platform, RMI.
RMI allows applications to call methods in objects on remote machines as if those objects were
located on the same machine. Calling a method in another object in Java is of course as simple as
the syntax object.method(arg). If you want to call that method from a remote machine without
RMI, however, you would have to write code that allows you to send an object handle, a method
name, and arguments through a TCP/IP socket, translate it into an object.method(arg) call on the
remote end, perform the method call, pass the return value back across the socket, and then write a
bunch of code to handle network failures. That is a lot of work for a simple method call, and I did
not even get into the issues you would have to deal with, such as passing object references as
arguments and handling garbage collection on each end. Finally, since you have written this
complex protocol to handle method calls across the network, you have serious rewriting to do if you
decide that a given object needs to exist on the client instead of the server (or vice versa).
With RMI, any method call, whether on the same machine or across the network, uses the same
Java method call syntax. This freedom to distribute objects across the network is called a distributed
object architecture. Other languages use much more complex protocols like Common Object
Request Broker Architecture (CORBA) and the Distributed Computing Environment (DCE). RMI,
however, is a Java-specific API for enabling a distributed architecture. As such, it removes many of
the complexities of those two solutions.
For a client/server database application, a distributed architecture allows the various parts of the
application to refer to the same physical objects when referring to particular objects in the data
model. For example, take an airline ticketing system that allows customers on the Internet to book
flights. Current web applications would have a user download a bunch of flight information as an
HTML page. If I book the last seat on a flight that you are viewing at the same time, you will not
see my booking of that last seat. This is because on each client screen you simply see copies of data
from the database.
If you reconstruct this web application so that it uses RMI to retrieve data from a single flight object
on the server, you can allow any number of different customers to view the exact same plane
objects at the same time. In this way, you can be certain that all viewers see any change made to the
plane object simultaneously. When I book the last seat on that flight, the flight object makes an
RMI call to all clients viewing it to let them know another seat was booked.
1.4.1 Putting It All Together
The pieces of the story are now in place. You will be using JDBC for your database access and RMI
to distribute the objects that make up your application. This book covers the JDBC API in complete
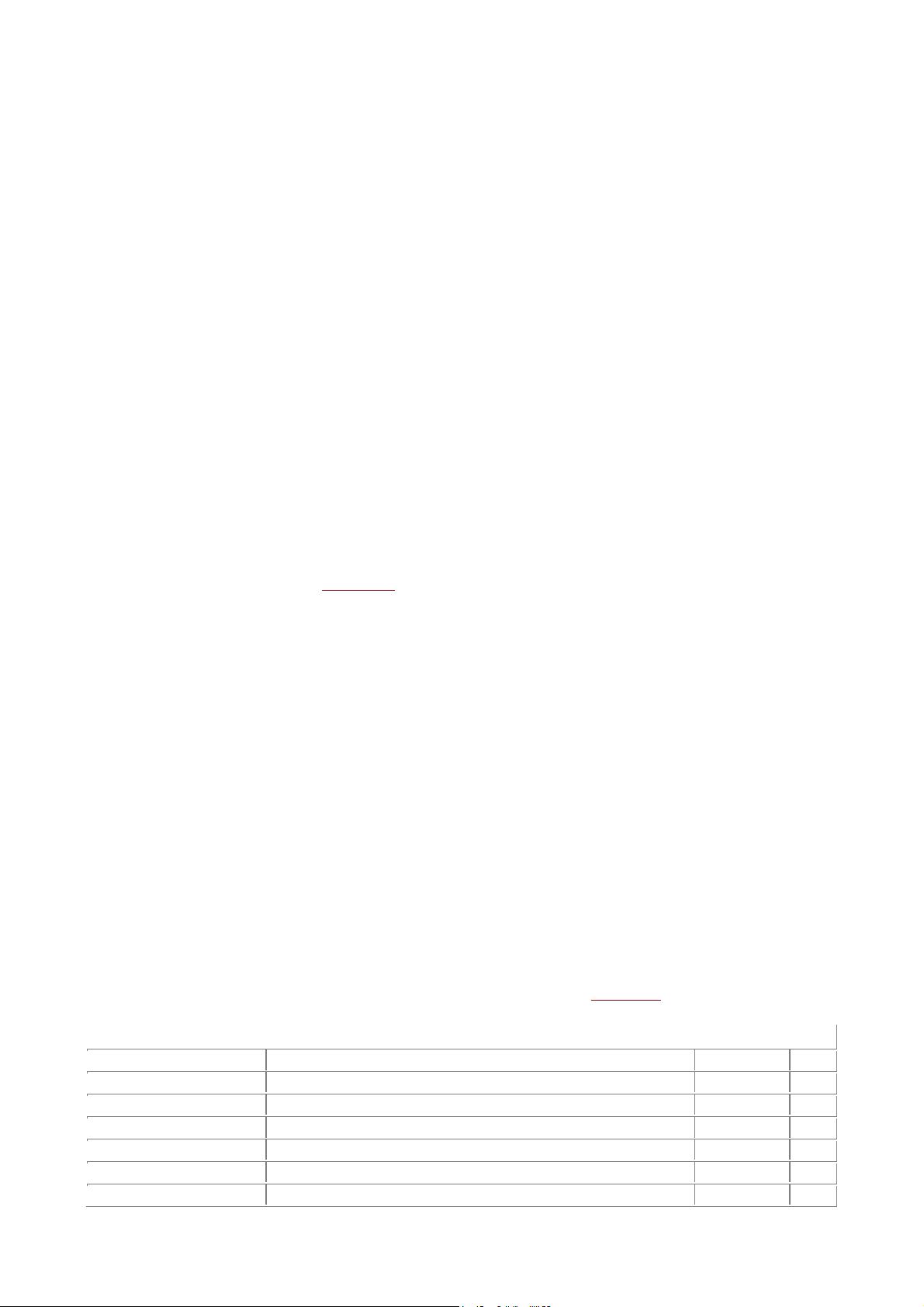
JDBC and Java 2
nd
edition
p
age 1
6
detail and discusses RMI as it pertains to the creation of distributed three-tier database applications.
To better use these APIs once you have gone beyond this book, I strongly recommend further
reading on these topics: object-oriented design methodologies, patterns in software development,
and general database programming.
Chapter 2. Relational Databases and SQL
Good sense is the most evenly shared thing in the world, for each of us thinks he is so well endowed
with it that even those who are the hardest to please in all other respects are not in the habit of
wanting more than they have. It is unlikely that everyone is mistaken in this. It indicates rather that
the capacity to judge correctly and to distinguish true from false, which is properly what one calls
common sense or reason, is naturally equal in all men, and consequently the diversity in our
opinions does not spring from some of us being more able to reason than others, but only from our
conducting our thoughts along different lines and not examining the same things.
— René Descartes, Discourse on the Method
Before you dive into the details of database programming in Java, I would like to take a chapter to
provide a basic discussion of relational databases for those of you who might have little or no
experience in this area. The subject of relational databases, however, is a huge topic that cannot
possibly be covered fully in this chapter. It is only designed to provide you with the most basic
introduction. Experienced database developers will find nothing new in this chapter; you will
probably want to skip ahead to Chapter 3.
2.1 What Is a Relational Database?
Programming is all about data processing; data is central to everything you do with a computer.
Databases—like filesystems—are nothing more than specialized tools for data storage. Filesystems
are good for storing and retrieving a single volume of information associated with a single virtual
location. In other words, when you want to save a WordPerfect document, a filesystem allows you
to associate it with a location in a directory tree for easy retrieval later.
Databases provide applications with a more powerful data storage and retrieval system based on
mathematical theories about data devised by Dr. E. F. Codd. Conceptually, a relational database can
be pictured as a set of spreadsheets in which rows from one spreadsheet can be related to rows from
another; in reality, however, the theory behind databases is much more complex. Each spreadsheet
in a database is called a table. As with a spreadsheet, a table is made up of rows and columns.
A simple way to illustrate the structure of a relational database is through a CD catalog. Let's say
that you have decided to create a database to keep track of your music collection. Not only do you
want to be able to store a list of your albums, but you also want to use this data later to help you
select music for parties. Your collection might look something like Table 2.1.
Table 2.1, A List of CDs from a Sample Music Collection
Artist Title Category Year
The Cure Pornography Alternative 1983
Garbage Garbage Alternative 1996
Hole Live Through This Alternative 1994
Nine Inch Nails The Downward Spiral Industrial 1994
Public Image Limited Compact Disc Alternative 1985
The Sex Pistols Never Mind the Bollocks, Here Come the Sex Pistols Punk 1977
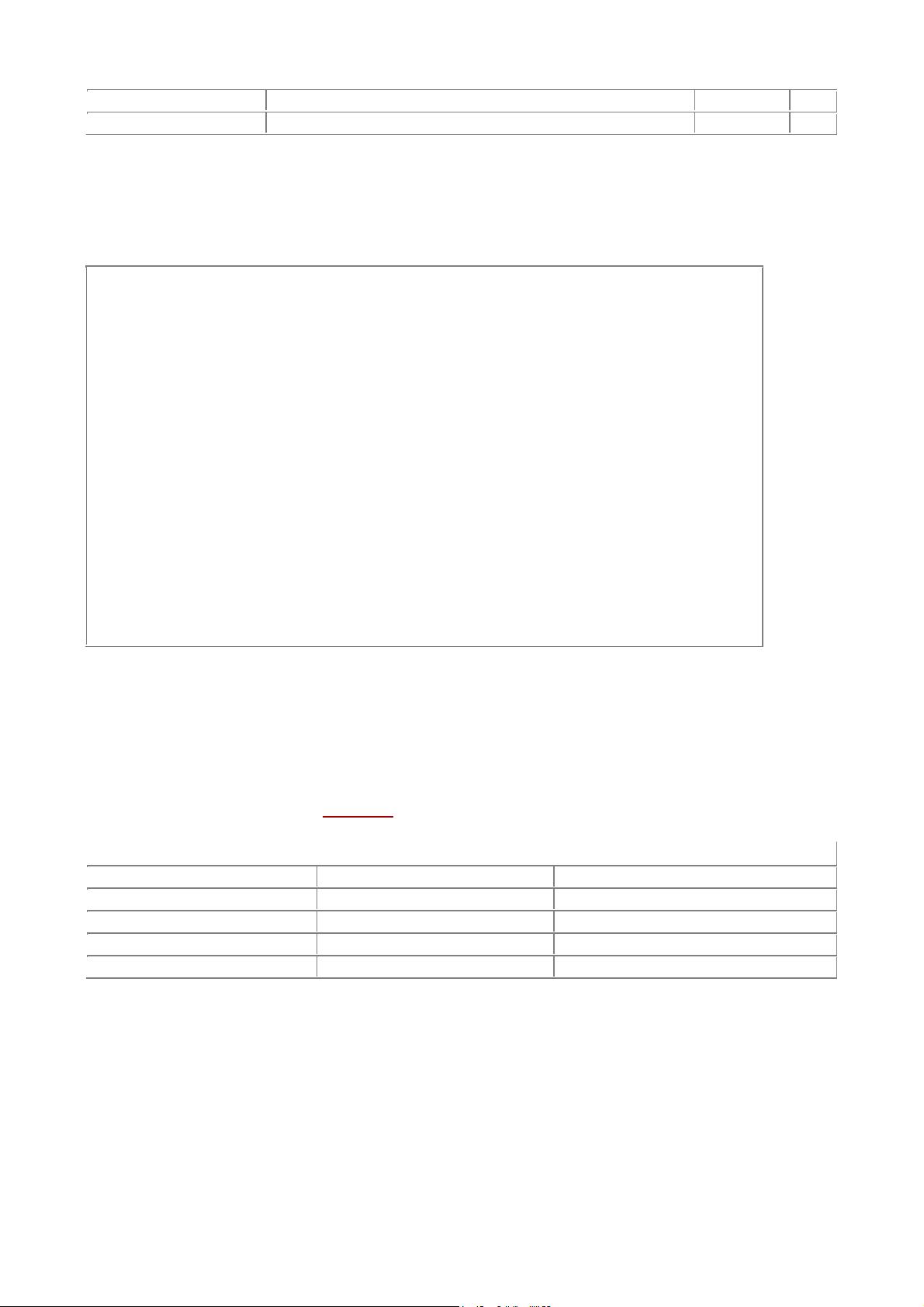
JDBC and Java 2
nd
edition
p
age 1
7
Skinny Puppy Last Rights Industrial 1992
Wire A Bell Is a Cup Until It Is Struck Alternative 1989
Of course, you could simply keep this list in a spreadsheet. But what if you wanted to have Johnny
Rotten night? Nothing in this list tells you which music in your catalog features him. You might
have another spreadsheet that lists musicians and the bands to which they belong, but there is
nothing about such a spreadsheet that can provide an easy programmatic answer to your question.
Databases and Database Engines
Developers new to database programming often run into problems understanding just
what a database is. In some contexts, it represents a collection of common data like the
music database you are looking at in this chapter. In other contexts, however, it may mean
the software used to support that collection of data, a process instance of that software, or
even the server machine on which the process is running.
Technically speaking, a database really is the collection of related data and the
relationships supporting the data. The database software is the software—such as Oracle,
Sybase, MySQL, and UDB—that is used to access that data. A database engine, in turn, is
a process instance of the software accessing your database. Finally, the database server is
the computer on which the database engine is running.
I will continue to use the term database interchangeably to refer to any of these
definitions. It is important, however, to database programming to understand this
breakdown.
With a database, you could easily ask the question "Can you give me all compact discs in my
collection with which Johnny Rotten was involved?" We will formally ask that question in a
minute. To make asking that question easier, however, you have to design your database to store the
information you need so that you can relate compact discs to individual musicians. You might
create another table called musicians that stores a list of musicians. For your purposes, you will
store only last names, first names, and nicknames in this list. However, you could store more
information, such as birthdays. Table 2.2
shows a part of your list.
Table 2.2, The Data in the Musicians Table
Last Name First Name Nickname
Jourgenson Al
Lydon John Johnny Rotten
Reznor Trent
Smith Robert
Nothing in these two lists relates musicians to bands, much less musicians to compact discs.
Another problem you can see in this list is that Robert Smith is a very common name, and there are
likely multiple artists who have that name. How do you know which Robert Smith should be related
to which compact disc? Database tables generally have one or more columns called keys that
uniquely identify each row. The key of the albums table could be the CD title; it is not uncommon,
however, for the same title to be used for different albums by different bands. The simplest thing to
do is to add another column to serve as the key column—let's call it an album ID. This column will
just be a sequential list of numbers. As you add new discs to the collection, increment the album ID
by one and insert that information. Thus album 1 is The Cure's Pornography, album 2 is Garbage's
剩余252页未读,继续阅读
241 浏览量
473 浏览量
989 浏览量

zhongjishengke
- 粉丝: 2
上传资源 快速赚钱
我的内容管理 展开
我的资源 快来上传第一个资源
我的收益
登录查看自己的收益我的积分 登录查看自己的积分
我的C币 登录后查看C币余额
我的收藏
我的下载
下载帮助

最新资源
- SmaartLive声场测试软件规范操作指南
- 详解PHP multipartform-data 远程DOS漏洞及其验证方法
- AI技术突破:8拼图解谜算法研究
- TouchIDPass:简化iOS用户认证的开源库
- 初学者无线点餐系统软件安装全教程
- 酒店网上预订HTML模板下载
- C#编程实现CPU使用率正弦波动效果
- Lucene5源码解读与拼音检索分词器应用教程
- Metricark仪表板:Java基本指标展示与安装
- 探索iOS开发的MVVM框架及其维护优势
- SSM框架整合:SpringMVC与MyBatis集成应用
- 节省时间的Chrome插件Did you mean?-自动更正拼写错误
- 黄维通《VC++面向对象与可视化程序设计(第三版)》课后练习
- Java 7并发编程食谱:实例教程与代码解析
- 免费下载酒店HTML5官网模板
- IEC61850 SCL文件编辑器:深度优化与中英语言支持
安全验证
文档复制为VIP权益,开通VIP直接复制
