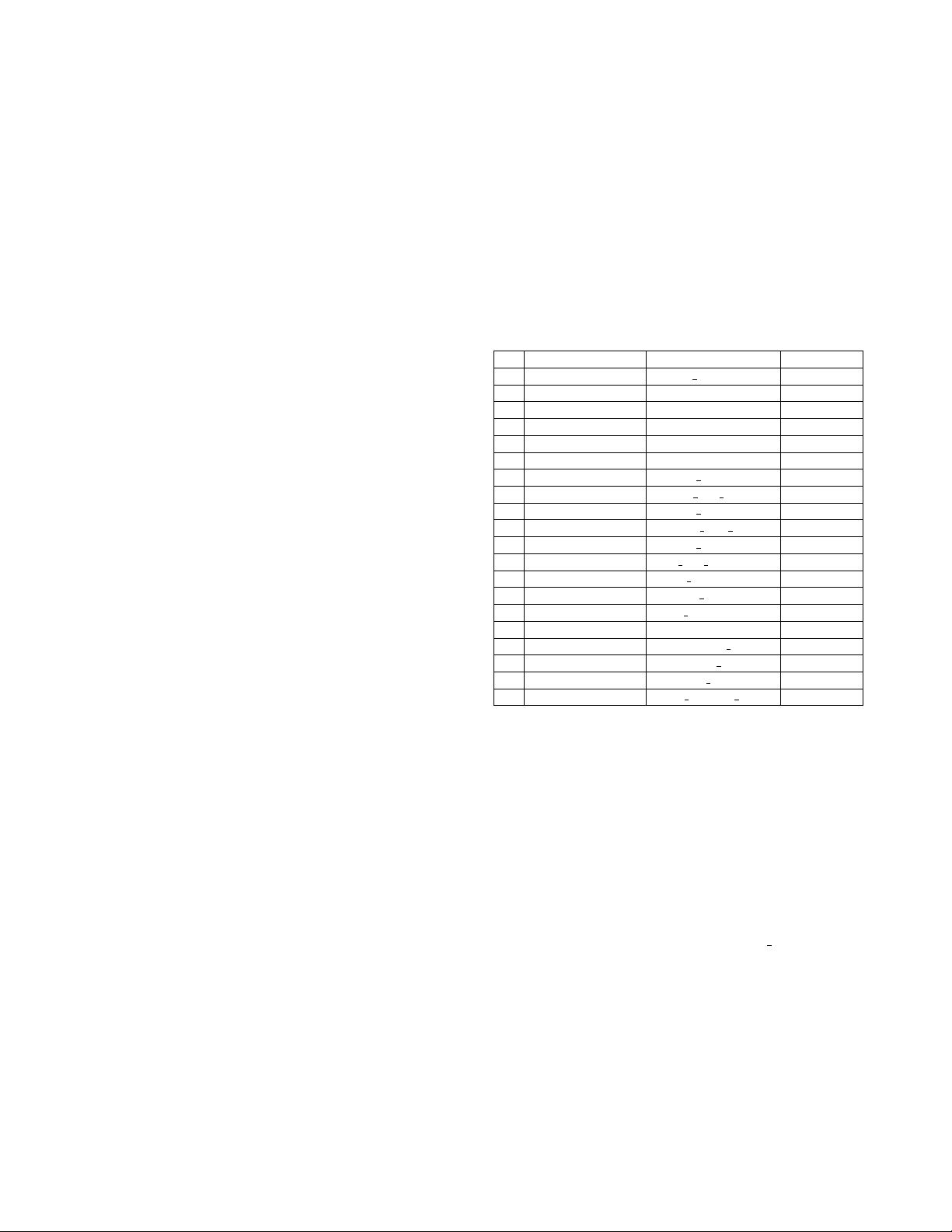
Linux Interrupts: The Basic Concepts
Mika J. Järvenpää
University of Helsinki
Department of Computer Science
mjjarven@cs.helsinki.fi
ABSTRACT
The idea of this document is to shed some light to the con-
cepts and main ideas of implementation of interrupts in
Linux kernel. Main focus is in Linux kernel version 2.4.18-
10.
1. LINUX INTERRUPTS
At any time one CPU in a Linux system can be:
- serving a hardware interrupt in kernel mode
- serving a softirq, tasklet or bottom half in kernel mode
- running a process in user mo de.
There is a strict order between these. Other than the last
category (user mode) can only be preempted by those above.
For example, while a softirq is running on a CPU, no other
softirqs will preempt it, but a hardware interrupt can.
In the next chapters different interrupt and exception types,
initialization, hardware handling and software handling of
interrupts, interrupt data structures and terms like IDT,
b ottom half, softirq and tasklet are explained in more detail.
2. INTERRUPTS AND EXCEPTIONS
An interrupt is an asynchronous event that is typically
generated by an I/O device not synchronized to processor
instruction execution.
An exception is a synchronous event that is generated when
the processor detects one or more predefined conditions
while executing an instruction.
Interrupts can be divided to maskable interrupts and
non-maskable interrupts.
Also exceptions can be divided to pro cessor-detected
exceptions ie. faults, traps, aborts and programmed
exceptions. Example of fault is Page fault. Traps are used
mainly for debugging purposes. Aborts inform about hard-
ware failures and invalid system tables and and abort han-
dler has no choice but to force affected process to terminate.
[1], [3], p. 4-9, 4-10.
Table 1: Signals sent by the exception handlers
# Exception Handler Signal
0 Divide error divide error() SIGFPE
1 Debug debug() SIGTRAP
2 NMI nmi() None
3 Breakp oint int3() SIGTRAP
4 Overflow overflow() SIGSEGV
5 Bounds check b ounds() SIGSEGV
6 Invalid opcode invalid op() SIGILL
7 Device not avail. device not avail.() SIGSEGV
8 Double fault double fault() SIGSEGV
9 Copro c.seg.ovr. copro c. seg. ovr.() SIGFPE
10 Invalid TSS invalid tss() SIGSEGV
11 Seg. not present seg. not present() SIGBUS
12 Stack seg. fault stack segment() SIGBUS
13 General protect. general prot.() SIGSEGV
14 Page fault page fault() SIGSEGV
15 Intel reserved None None
16 FP error copro cessor error() SIGFPE
17 Alignment check alignment check() SIGSEGV
18 Machine check machine check() None
19 SIMD coproc.err simd coproc. error Dep ends
2.1 Exception and Interrupt Vectors
Intel architecture identifies different interrupts and excep-
tions by a number ranging from 0 to 255 ([5], p. 4-11).
This number is called a vector. Linux uses vectors 0 to
31, which are for exceptions and non-maskable interrupts,
vectors 32 to 47, which are for maskable interrupts ie. in-
terrupts caused by interrupt requests (IRQs) and only one
vector (128) from the remaining vectors ranging from 48 to
255, which are meant for software interrupts.
Linux uses this vector 128 to implement a system call ie.
when an int 0x80 opcode ([6]) is executed by a process
running in user mode the CPU switches into kernel mode
and starts executing kernel function system call().
2.2 Hardware Interrupts
Hardware devices capable of issuing IRQs are connected to
Interrupt Controller. The Intel 8259A Programmable Inter-
rupt Controller (PIC) handles up to eight vectored prior-
ity interrupts for the CPU and PICs can be cascaded ([2]).
Typical configuration for 15 IRQs is cascade of two PICs.
PIC can remember one IRQ while IRQ is masked. Vector
of masked IRQ is sent to CPU after unmasking. Processing
of previous interrupt is finished by writing End Of Interrupt