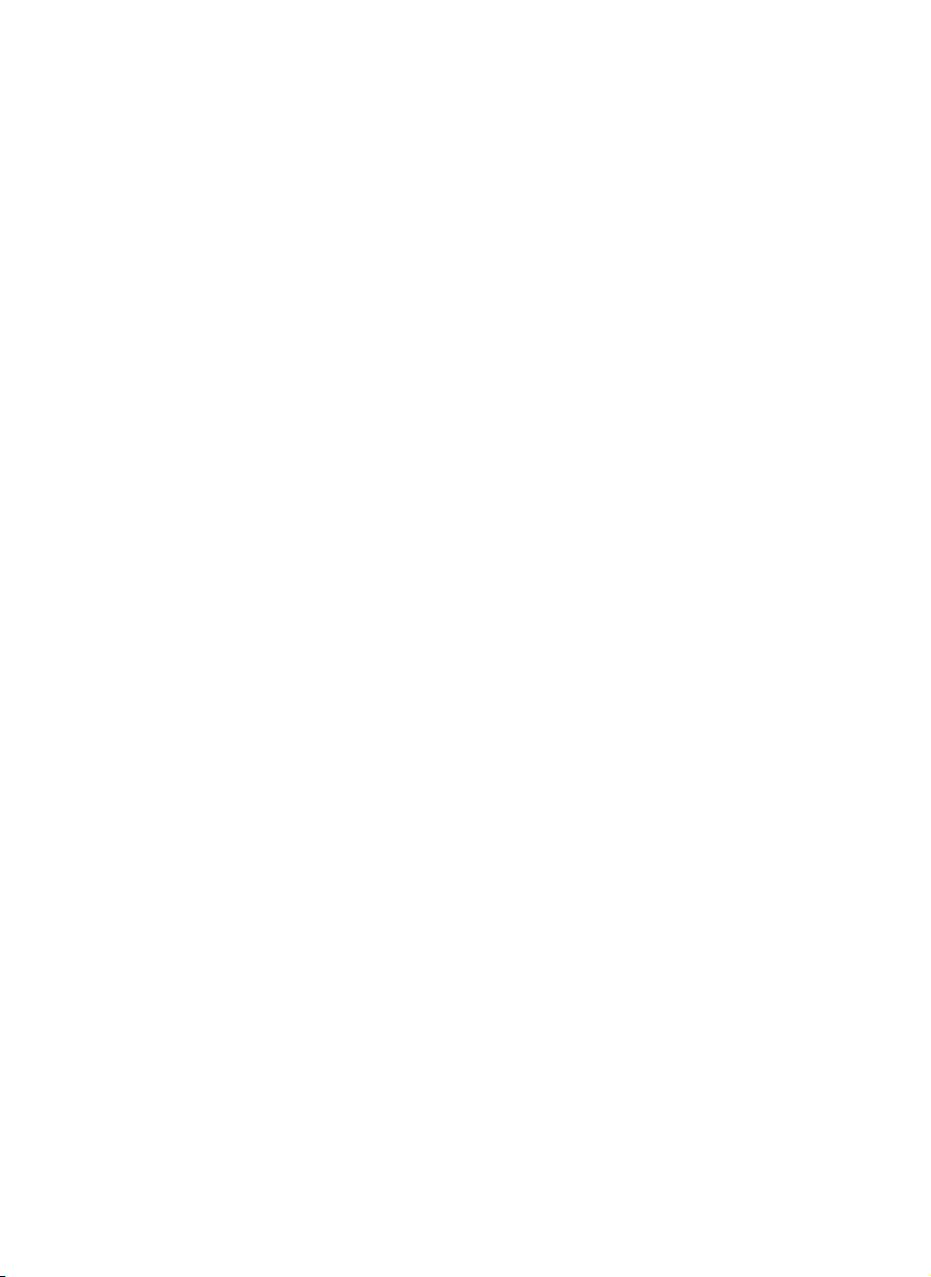
ptg7068951
4
Introduction
n
Chapter 7: Functions: C++’s Programming Modules—Functions are the basic
building blocks of C++ programming. Chapter 7 concentrates on features that
C++ functions share with C functions. In particular, you’ll review the general for-
mat of a function definition and examine how function prototypes increase the
reliability of programs. Also, you’ll investigate how to write functions to process
arrays, character strings, and structures. Next, you’ll learn about recursion, which is
when a function calls itself, and see how it can be used to implement a divide-and-
conquer strategy. Finally, you’ll meet pointers to functions, which enable you to use
a function argument to tell one function to use a second function.
n
Chapter 8: Adventures in Functions—Chapter 8 explores the new features C++
adds to functions.You’ll learn about inline functions, which can speed program exe-
cution at the cost of additional program size.You’ll work with reference variables,
which provide an alternative way to pass information to functions. Default argu-
ments let a function automatically supply values for function arguments that you
omit from a function call. Function overloading lets you create functions having the
same name but taking different argument lists.All these features have frequent use
in class design.Also you’ll learn about function templates, which allow you to spec-
ify the design of a family of related functions.
n
Chapter 9: Memory Models and Namespaces—Chapter 9 discusses putting
together multifile programs. It examines the choices in allocating memory, looking
at different methods of managing memory and at scope, linkage, and namespaces,
which determine what parts of a program know about a variable.
n
Chapter 10: Objects and Classes—A class is a user-defined type, and an object
(such as a variable) is an instance of a class. Chapter 10 introduces you to object-
oriented programming and to class design.A class declaration describes the infor-
mation stored in a class object and also the operations (class methods) allowed for
class objects. Some parts of an object are visible to the outside world (the public
portion), and some are hidden (the private portion). Special class methods (con-
structors and destructors) come into play when objects are created and destroyed.
Yo u w i l l l e a r n a b o u t a l l t h i s a n d o t h e r c l a s s d e t a i l s i n t h i s c h a p t e r , and you’ll see
how classes can be used to implement ADTs, such as a stack.
n
Chapter 11:Working with Classes—In Chapter 11 you’ll further your under-
standing of classes. First, you’ll learn about operator overloading, which lets you
define how operators such as + will work with class objects.You’ll learn about
friend functions, which can access class data that’s inaccessible to the world at large.
Yo u ’ l l s e e h o w c e r t a i n c o n s t r u c t o r s a n d o v e r l o a d e d o p e r a t o r m e m b e r f u n c t i o n s c a n
be used to manage conversion to and from class types.
n
Chapter 12: Classes and Dynamic Memory Allocation—Often it’s useful to
have a class member point to dynamically allocated memory. If you use new in a
class constructor to allocate dynamic memory, you incur the responsibilities of pro-
viding an appropriate destructor, of defining an explicit copy constructor, and of