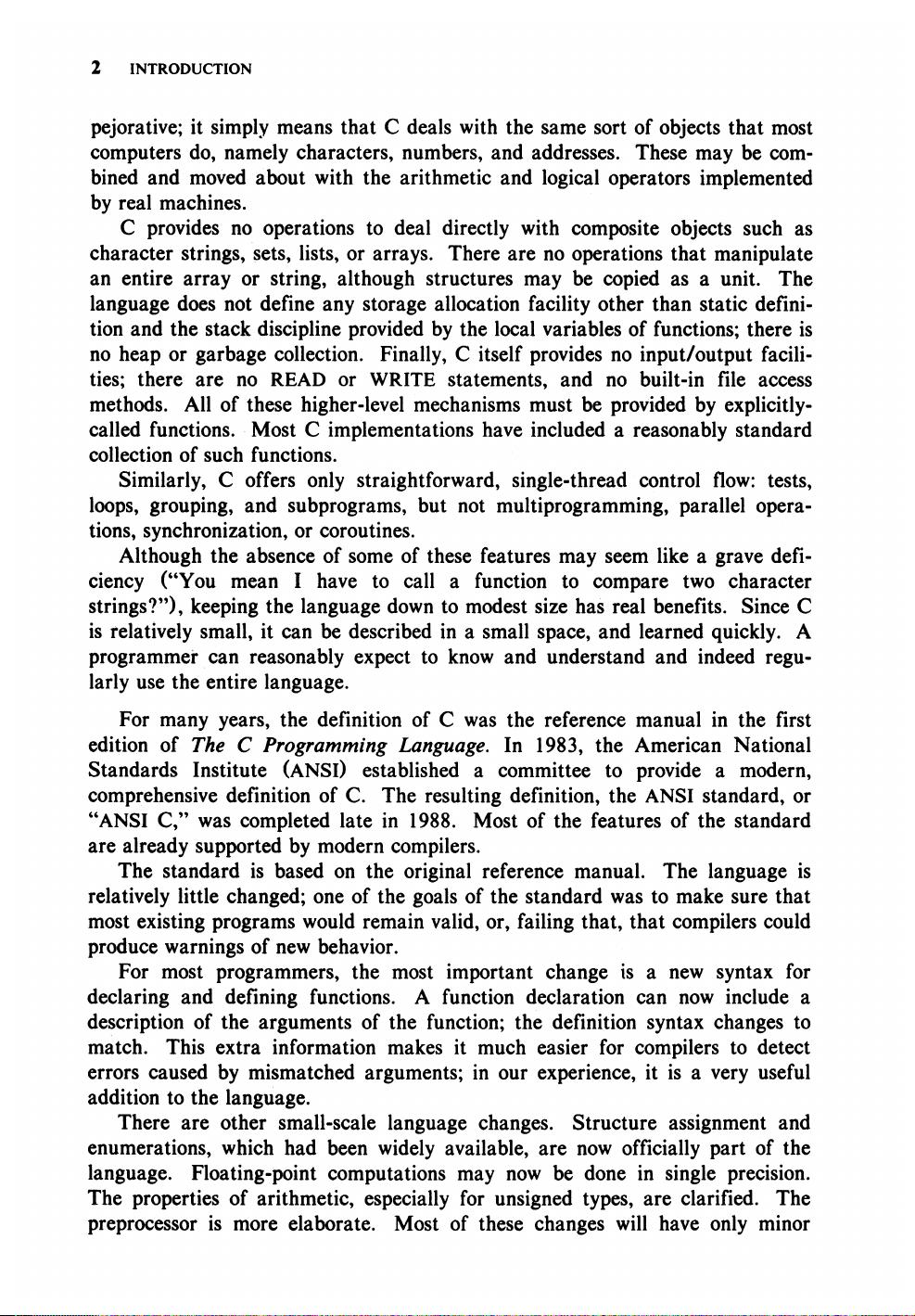
pejorative; it simply means that C deals with the same sort of objects that most
computers do, namely characters, numbers, and addresses. These may be com-
bined and moved about with the arithmetic and logical operators implemented
by real machines.
C provides no operations to deal directly with composite objects such as
character strings, sets, lists, or arrays. There are no operations that manipulate
an entire array or string, although structures may be copied as a unit. The
language does not define any storage allocation facility other than static defini-
tion and the stack discipline provided by the local variables of functions; there is
no heap or garbage collection. Finally, C itself provides no input/output facili-
ties; there are no READ or WRITE statements, and no built-in file access
methods. All of these higher-level mechanisms must be provided by explicitly-
called functions. Most C implementations have included a reasonably standard
collectionof such functions.
Similarly, C offers only straightforward, single-thread control flow: tests,
loops, grouping, and subprograms, but not multiprogramming, parallel opera-
tions, synchronization,or coroutines.
Although the absence of some of these features may seem like a grave defi-
ciency ("You mean I have to call a function to compare two character
strings?"), keeping the language down to modest size has real benefits. Since C
is relatively small, it can be described in a small space, and learned quickly. A
programmer can reasonably expect to know and understand and indeed regu-
larly use the entire language.
For many years, the definition of C was the reference manual in the first
edition of The C Programming Language. In 1983, the American National
Standards Institute
(ANSI)
established a committee to provide a modern,
comprehensive definition of C. The resulting definition, the
ANSI
standard, or
"ANSI
C," was completed late in 1988. Most of the features of the standard
are already supported by modern compilers.
The standard is based on the original reference manual. The language is
relatively little changed; one of the goals of the standard was to make sure that
most existing programs would remain valid, or, failing that, that compilers could
produce warnings of new behavior.
For most programmers, the most important change is a new syntax for
declaring and defining functions. A function declaration can now include a
description of the arguments of the function; the definition syntax changes to
match. This extra information makes it much easier for compilers to detect
errors caused by mismatched arguments; in our experience, it is a very useful
addition to the language.
There are other small-scale language changes. Structure assignment and
enumerations, which had been widely available, are now officially part of the
language. Floating-point computations may now be done in single precision.
The properties of arithmetic, especially for unsigned types, are clarified. The
preprocessor is more elaborate. Most of these changes will have only minor
1
INTRODUCTION