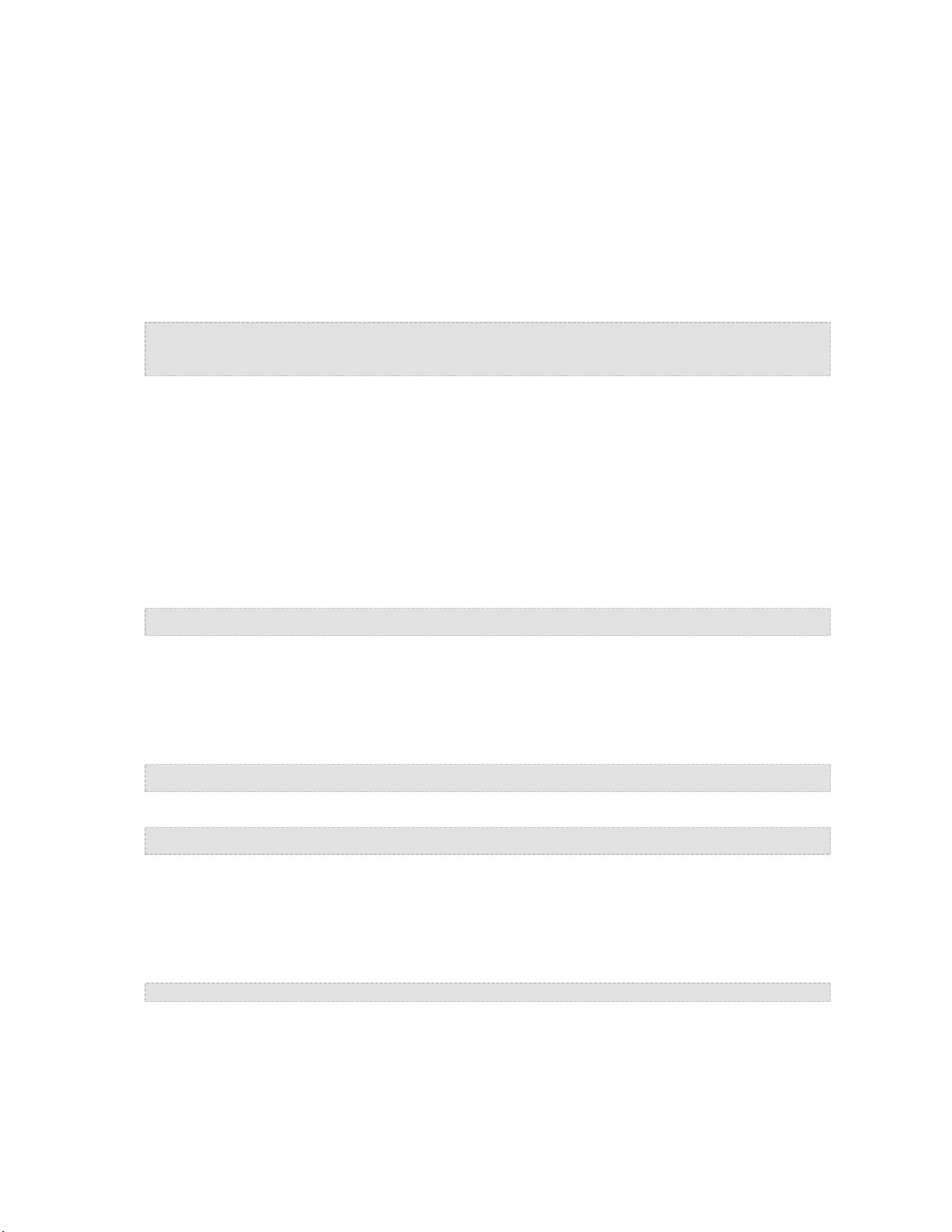
1. In the Android project view window, under app, click res > layout, then double-click activity_main.xml.
This opens the Preview view of the central content for the main activity layout. Note that depending on the exact Android Studio
version you are using and the initial activity template you chose, the project may contain multiple layout XML files—for example if
you chose the Basic Activity template, you may need to instead open the content_main.xml layout.
2. At the lower left of the window, click Text to show the XML view of the layout if it's not already shown.
By default, there are two tags in the layout XML file.
• The first is an Android ConstraintLayout. This is a view that can show other views inside itself. It arranges its children
views relative to each other. For more information, see the Constraint Layout and User Interface documentation from
Google.
• The second is a TextView, a child of the constraint layout, displaying the text "Hello world!".
3. Select the entire TextView XML element and replace it with a MapView element as follows:
<com.esri.arcgisruntime.mapping.view.MapView
android:id="@+id/mapView"
android:layout_width="match_parent"
android:layout_height="match_parent" >
</com.esri.arcgisruntime.mapping.view.MapView>
For a more comprehensive understanding of the declarative approach to user interface (UI) design, see Declaring Layout. Additionally,
there are different types of layout constraint attributes that are commonly used with the ConstraintLayout; refer to the Constraint
Layout documentation for more information.
Set a map on the MapView
By default, a MapView does not display anything, so the next step is to define a map to be displayed. You will specify that the map shows
a worldwide topographic basemap from ArcGIS Online. As it's often more useful for an app to initially display a specific region rather than
its entire extent, you'll also set the map to zoom in to a specific center point—showing the Esri campus in Redlands, California.
1. In the Android project view window, under app, click java > [package name], and double-click MainActivity.
This opens the Kotlin code defining the default activity of your app. (Kotlin code is still contained in a subfolder of a java folder).
2. Add the following code to the onCreate method, after the existing call to setContentView:
// create a map with the BasemapType topographic
val map = ArcGISMap(Basemap.Type.TOPOGRAPHIC, 34.056295, -117.195800, 16)
This code creates an ArcGISMap with a specific predefined Basemap.Type displaying a general-use topographic map,
centered at a specific set of coordinates, and zoomed to a specific level of detail.
3. Android Studio will highlight in red the ArcGISMap and Basemap classes, which must be imported into the activity class. Place
the pointer on the ArcGISMap text highlighted in red and press Alt+Enter to resolve the symbol. Choose import. Repeat this
step to import Basemap.
You should now have the following import statements at the top of your class.
import com.esri.arcgisruntime.mapping.ArcGISMap
import com.esri.arcgisruntime.mapping.Basemap
4. Add the following code to the onCreate method, after the previous line you added:
// set the map to be displayed in the layout's MapView
mapView.map = map
This code sets the ArcGISMap you created into the MapView you defined in the layout.
5. Android Studio will highlight mapView. Place the pointer on mapView and press Alt+Enter to resolve the symbol. This time you
should see more than one option to import. Choose kotlinx.android.synthetic.main.activity_main.mapView.
The kotlin android extensions plugin (part of your project by default when you add Kotlin support) generates synthetic properties,
so you can access views in the layout XML as if they were properties of the class. Android Studio will import all of the named
views in the layout by adding a general import.
import kotlinx.android.synthetic.main.activity_main.*
6. Add the following methods to the MainActivity class, to override the onPause and onResume methods inherited from the
parent activity class, and pause and resume the MapView when those methods are called:
ArcGIS Runtime SDK for Android
Copyright © 1995-2018 Esri. All rights reserved.
16