C语言字符串处理函数详解

"这篇文档汇总了C语言中的字符串处理函数,包括stpcpy、strcat、strchr和strcmp等常用函数,提供了相应的示例代码,帮助读者理解和掌握这些函数的用法。" C语言字符串函数在编程中起着至关重要的作用,能够帮助我们有效地操作和处理字符串数据。以下是对这些函数的详细解释: 1. stpcpy 函数 - 功能:stpcpy函数用于将源字符串(source)的内容复制到目标字符串(destin)的末尾,并返回指向目标字符串结束符的指针。 - 用法:`char* stpcpy(char* destin, char* source);` - 示例: ```c #include<stdio.h> #include<string.h> int main(void) { char string[10]; char* str1 = "abcdefghi"; stpcpy(string, str1); printf("%s\n", string); return 0; } ``` - 在这个例子中,"abcdefghi"被复制到了string数组中,输出即为"abcdefghi"。 2. strcat 函数 - 功能:strcat函数用于连接两个字符串。它将源字符串(source)追加到目标字符串(destin)的末尾。 - 用法:`char* strcat(char* destin, char* source);` - 示例: ```c #include<string.h> #include<stdio.h> int main(void) { char destination[25]; char* blank = "", * c = "C++", * Borland = "Borland"; strcpy(destination, Borland); strcat(destination, blank); strcat(destination, c); printf("%s\n", destination); return 0; } ``` - 结果会输出"BorlandC++",因为"Borland"先被复制到destination,然后添加了空字符串和"C++"。 3. strchr 函数 - 功能:strchr函数用于在一个字符串(str)中查找指定字符(c)的第一个出现位置。 - 用法:`char* strchr(char* str, char c);` - 示例: ```c #include<string.h> #include<stdio.h> int main(void) { char string[15]; char* ptr, c = 'r'; strcpy(string, "Thisisastring"); ptr = strchr(string, c); if (ptr) printf("The character %c is at position: %d\n", c, ptr - string); else printf("The character was not found\n"); return 0; } ``` - 这个例子会输出"The character r is at position: 7",因为在字符串"Thisisastring"中,字符'r'的位置是第7个。 4. strcmp 函数 - 功能:strcmp函数比较两个字符串(str1和str2),根据ASCII码值判断它们的顺序。如果str1>str2,返回值>0;如果两串相等,返回0;如果str1<str2,返回值<0。 - 用法:`int strcmp(char* str1, char* str2);` - 示例代码未给出,但基本用法是这样的: ```c #include<string.h> #include<stdio.h> int main(void) { char str1[] = "hello"; char str2[] = "world"; int result = strcmp(str1, str2); if (result > 0) printf("%s is greater than %s\n", str1, str2); else if (result < 0) printf("%s is less than %s\n", str1, str2); else printf("%s and %s are equal\n", str1, str2); return 0; } ``` - 这段代码会输出"hello is less than world",因为'h'的ASCII码小于'w'。 了解并熟练掌握这些C语言字符串函数,对于编写涉及字符串操作的C程序非常有帮助。在实际编程中,可以根据需要选择合适的函数进行字符串的拷贝、连接、查找和比较。
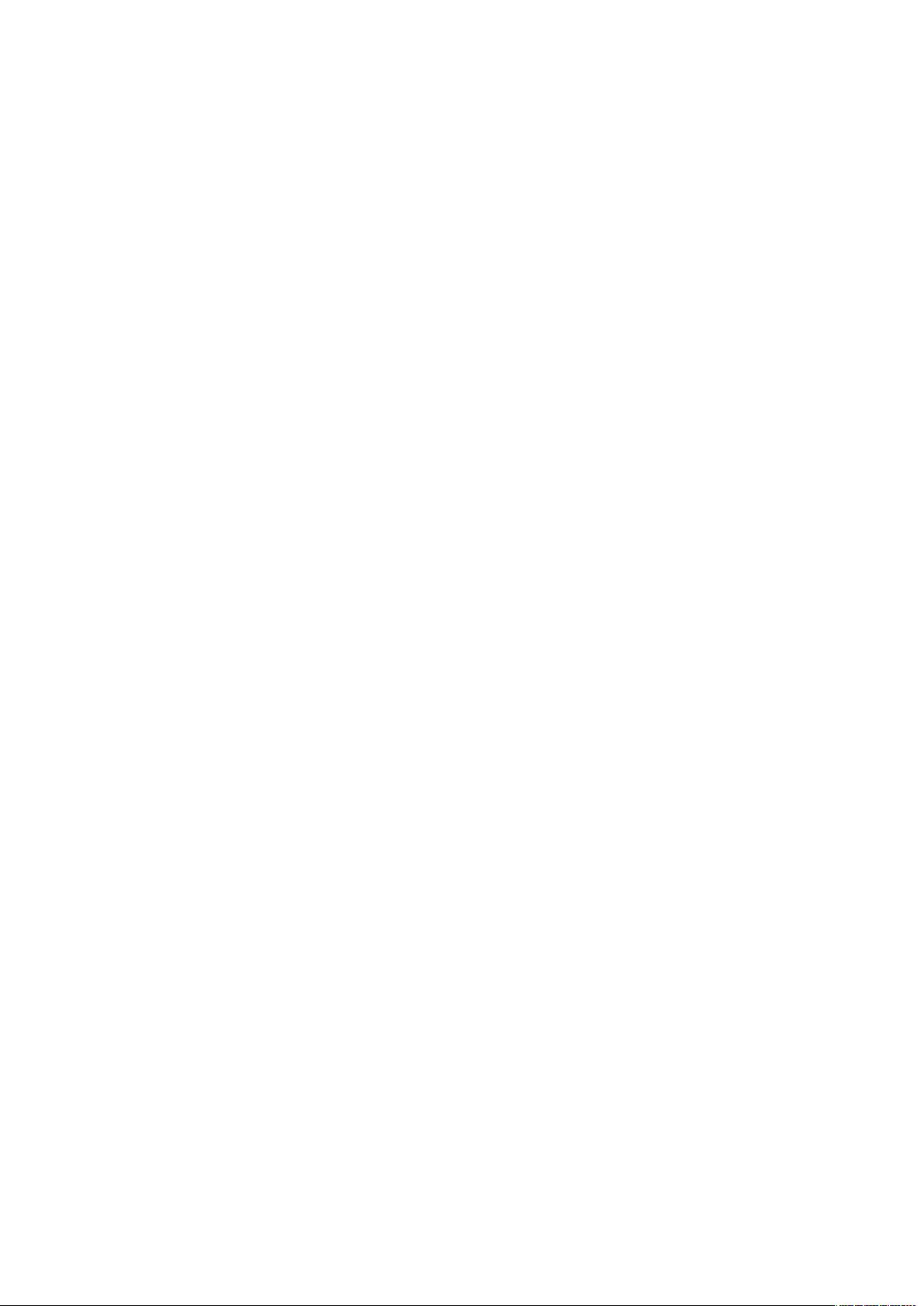
剩余10页未读,继续阅读
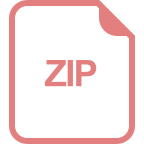


















- 粉丝: 0
- 资源: 6
我的内容管理 展开
我的资源 快来上传第一个资源
我的收益
登录查看自己的收益我的积分 登录查看自己的积分
我的C币 登录后查看C币余额
我的收藏
我的下载
下载帮助

最新资源
- 李兴华Java基础教程:从入门到精通
- U盘与硬盘启动安装教程:从菜鸟到专家
- C++面试宝典:动态内存管理与继承解析
- C++ STL源码深度解析:专家级剖析与关键技术
- C/C++调用DOS命令实战指南
- 神经网络补偿的多传感器航迹融合技术
- GIS中的大地坐标系与椭球体解析
- 海思Hi3515 H.264编解码处理器用户手册
- Oracle基础练习题与解答
- 谷歌地球3D建筑筛选新流程详解
- CFO与CIO携手:数据管理与企业增值的战略
- Eclipse IDE基础教程:从入门到精通
- Shell脚本专家宝典:全面学习与资源指南
- Tomcat安装指南:附带JDK配置步骤
- NA3003A电子水准仪数据格式解析与转换研究
- 自动化专业英语词汇精华:必备术语集锦

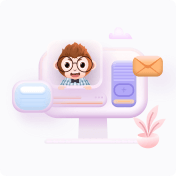
