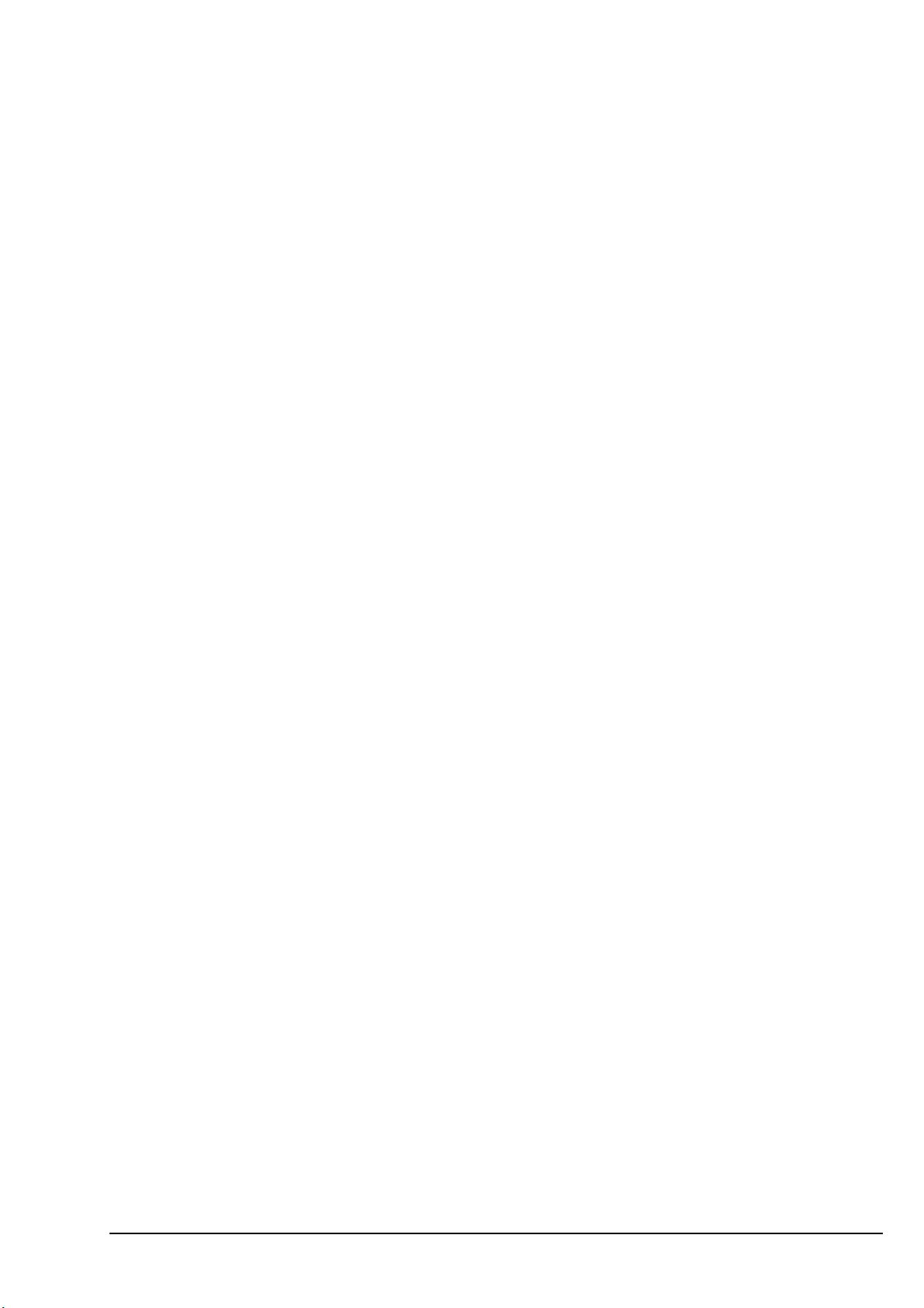
Computers and Programs C#
C# Programming © Rob Miles 2010 15
with it. Switching to unmanaged mode is analogous to removing the guard from your
new chainsaw because it gets in the way.
C# is a great language to start learning with as the managed parts will make it easier for
you to understand what has happened when your programs go wrong.
1.4.3 C# and Objects
The C# language is object oriented. Objects are an organisational mechanism which let
you break your program down into sensible chunks, each of which is in charge of part
of the overall system. Object Oriented Design makes large projects much easier to
design, test and extend. It also lets you create programs which can have a high degree
of reliability and stability.
I am very keen on object oriented programming, but I am not going to tell you much
about it just yet. This is not because I don't know much about it (honest) but because I
believe that there are some very fundamental programming issues which need to be
addressed before we make use of objects in our programs.
The use of objects is as much about design as programming, and we have to know how
to program before we can design larger systems.
1.4.4 Making C# Run
You actually write the
program using some form of
text editor - which may be
part of the compiling and
running system.
C# is a compiled programming language. The computer cannot understand the
language directly, so a program called a compiler converts the C# text into the low
level instructions which are much simpler. These low level instructions are in turn
converted into the actual commands to drive the hardware which runs your program.
We will look in more detail at this aspect of how C# programs work a little later, for
now the thing to remember is that you need to show your wonderful C# program to
the compiler before you get to actually run it.
A compiler is a very large program which knows how to decide if your program is
legal. The first thing it does is check for errors in the way that you have used the
language itself. Only if no errors are found by the compiler will it produce any output.
The compiler will also flag up warnings which occur when it notices that you have
done something which is not technically illegal, but may indicate that you have made a
mistake somewhere. An example of a warning situation is where you create something
but don't use it for anything. The compiler would tell you about this, in case you had
forgotten to add a bit of your program.
The C# language is supplied with a whole bunch of other stuff (to use a technical term)
which lets C# programs do things like read text from the keyboard, print on the screen,
set up network connections and the like. These extra features are available to your C#
program but you must explicitly ask for them. They are then located automatically
when your program runs. Later on we will look at how you can break a program of
your own down into a number of different chunks (perhaps so several different
programmers can work on it).
1.4.5 Creating C# Programs
Microsoft has made a tool called Visual Studio, which is a great place to write
programs. It comprises the compiler, along with an integrated editor, and debugger. It
is provided in a number of versions with different feature sets. There is a free version,
called Visual Studio Express edition, which is a great place to get started. Another free
resources is the Microsoft .NET Framework. This provides a bunch of command line
tools, i.e. things that you type to the command prompt, which can be used to compile
and run C# programs. How you create and run your programs is up to you.