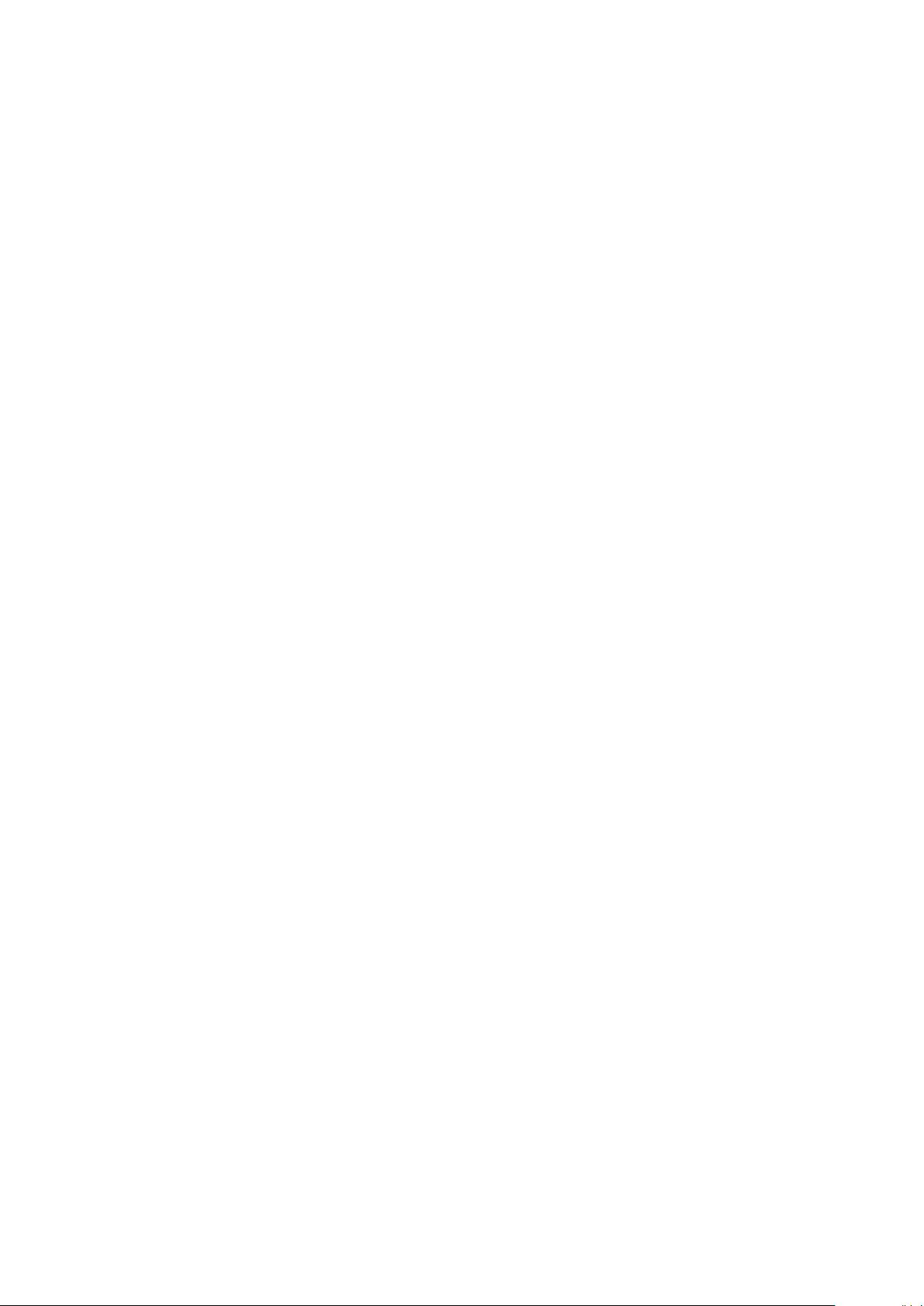
CHAPTER 2. SIMPLE APPLICATIVE PROGRAMMING 13
2.6.3 The Char structure
Operations on characters are found in the Char structure. These include the integer equiva-
lents of the word-to-character conversion functions, Char.chr and Char.ord. Also included
are successor and predecessor functions, Char.succ and Char.pred. Many functions perform
obvious tests on characters, such as Char.isAlpha, Char.isUpper, Char.isLower, Char.isDigit,
Char.isHexDigit and Char.isAlphaNum. Some exhibit slightly more complicated behaviour,
such as Char.isAscii which identifies seven-bit ASCII characters; and Char.isSpace which
identifies whitespace (spaces, tabulate characters, line break and page break characters);
Char.isPrint identifies printable characters and Char.isGraph identifies printable, non-
whitespace characters. The functions Char.toUpper and Char.toLower behave as expected,
leaving non-lowercase (respectively non-uppercase) characters unchanged. The pair of comple-
mentary functions Char.conta ins and Char.notContains may be used to determine the
presence or absence of a character in a string. Relational operators on characters are also
provided in the Char structure.
2.6.4 The Int structure
The Int structure contains many arithmetic operators. Some of these, such as Int.quot and
Int.rem, are subtle variants on more familiar operators such as div and mod . Quotient
and remainder differ from divisor and modulus in their behaviour with negative operands.
Other operations on integers include Int.abs and Int.min and Int.max, which behave as
expected. This structure also provides conversions to and from strings, named Int.toString
and Int.fromString, of course. An additional formatting function provides the ability to
represent integers in bases other than decimal. The chosen base is specified using a type
which is defined in another library structure, the string convertors structure, StringCvt. This
permits very convenient formatting of integers in binary, octal, decimal or hexadecimal, as
shown below.
Int.fmt StringCvt.BIN 1024 = "10000000000"
Int.fmt StringCvt.OCT 1024 = "2000"
Int.fmt StringCvt.DEC 1024 = "1024"
Int.fmt StringCvt.HEX 1024 = "400"
The Int structure may define largest and smallest integer values. This is not to say that
structures may arbitrarily shrink or grow in size, Int.maxInt either takes the value NONE
or SOME i, with i being an integer value. Int .minInt has the same type.
2.6.5 The Real structure
It would not be very misleading to say that the Real structure contains all of the corre-
sponding real equivalents of the integer functions from the Int structure. In addition to
these, it provides conversions to and from integers. For the former we have Real.trunc, which
truncates; Real.round, which rounds up; Real.floor which returns the greatest integer less
than its argument; and Real.ceil, which returns the least integer greater than its argument.
For the latter we have Real.fromInt, which we have already seen. One function which is