深入理解Java:思维之旅
"《Think IN java》是一本深入探讨Java编程思想的书籍,作者Bruce Eckel是MindView, Inc.的总裁。这本书旨在帮助读者理解Java的强大之处,尤其是在解决复杂问题时的优势。书中强调了Java不仅仅是一系列特性的集合,而是需要在设计层面去理解和应用。通过构建一个知识结构模型,读者可以更深入地掌握Java语言,并能够运用它来解决问题。书中的章节围绕特定类型的编程问题展开,提供了挑战性的练习,并特别提到了关于Collections的精彩内容。这本书不仅适合初学者全面学习,也是经验丰富的开发者的重要参考书籍。读者反馈中,有人提到它对于通过Sun Certified Java Programmer考试有很大帮助,同时也称赞其深入浅出的示例和解释,认为它是学习Java的理想教材。" 《Think IN java》第四版深入介绍了Java编程的核心概念,鼓励读者不仅仅是从语法层面去学习Java,而是要理解其设计理念。书中提到,Java的强大在于其在面对复杂问题时的表达能力和灵活性。作者强调,不应孤立地看待Java的特性,而应将它们放在整体的设计框架下去理解。为了帮助读者更好地掌握这些概念,书中的每一章都会围绕一个特定的编程问题进行讲解,通过实例来展示Java如何解决这些问题。 此外,书中还强调建立一个“知识结构”模型的重要性。这个模型可以帮助读者在遇到困难时,将问题放入相应的位置,通过自我演绎找到答案。这种学习方法强调了主动思考和自我解决问题的能力,使学习过程更加高效。 书中的练习部分是提升技能的关键,它们设计得富有挑战性,旨在让读者在实践中深化理解。特别提到的“Collections”章节,可能涵盖了Java集合框架的深入探讨,包括List、Set、Map等接口及其实现类的使用,以及相关算法和数据结构的应用。 读者的评价反映了《Think IN java》的高质量和实用性,无论对于初学者还是有经验的开发者,这本书都提供了极高的价值。它被赞誉为编程教程的典范,以其完整的内容、精准的例子和严谨的解释赢得了高度认可,被认为是学习Java的必备读物。
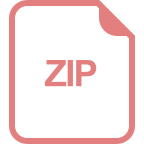
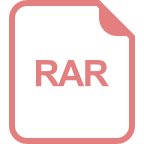
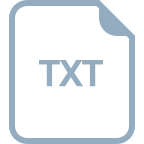
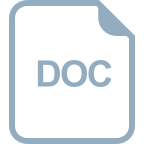
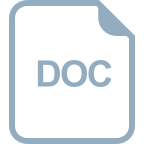
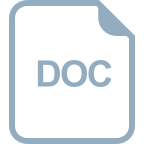
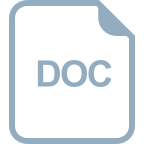
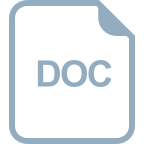












- 粉丝: 0
- 资源: 2
我的内容管理 展开
我的资源 快来上传第一个资源
我的收益
登录查看自己的收益我的积分 登录查看自己的积分
我的C币 登录后查看C币余额
我的收藏
我的下载
下载帮助

最新资源
- AirKiss技术详解:无线传递信息与智能家居连接
- Hibernate主键生成策略详解
- 操作系统实验:位示图法管理磁盘空闲空间
- JSON详解:数据交换的主流格式
- Win7安装Ubuntu双系统详细指南
- FPGA内部结构与工作原理探索
- 信用评分模型解析:WOE、IV与ROC
- 使用LVS+Keepalived构建高可用负载均衡集群
- 微信小程序驱动餐饮与服装业创新转型:便捷管理与低成本优势
- 机器学习入门指南:从基础到进阶
- 解决Win7 IIS配置错误500.22与0x80070032
- SQL-DFS:优化HDFS小文件存储的解决方案
- Hadoop、Hbase、Spark环境部署与主机配置详解
- Kisso:加密会话Cookie实现的单点登录SSO
- OpenCV读取与拼接多幅图像教程
- QT实战:轻松生成与解析JSON数据

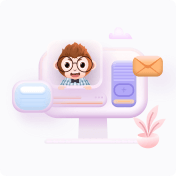
