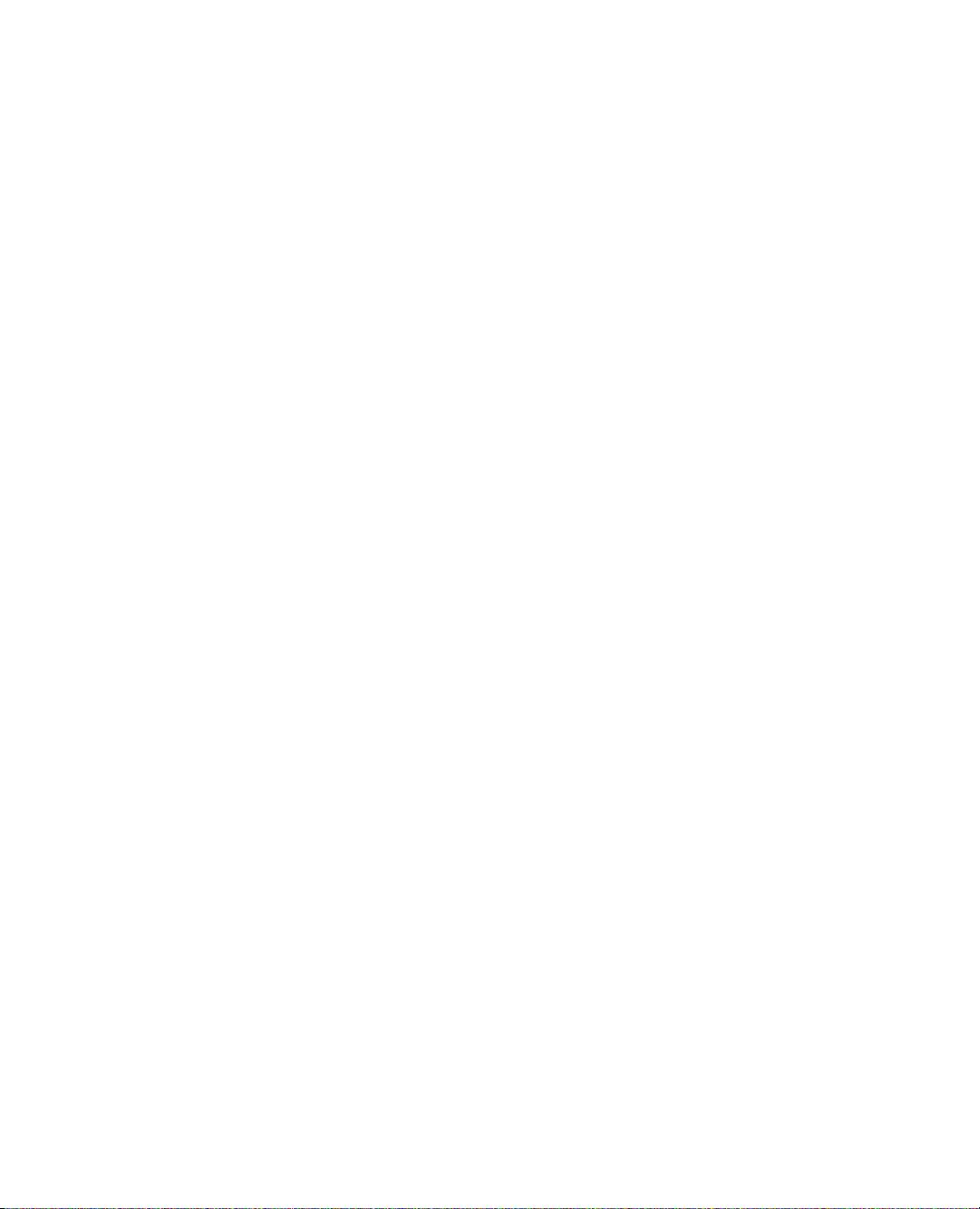
CHAPTER 2 ■ DJANGO IS PYTHON
15
This declarative syntax has become an identifying feature of Django code, so many third-party applications that
supply additional frameworks are written to use a syntax similar to that of Django itself. This helps developers easily
understand and utilize new code by making it all feel more cohesive. Once you understand how to create a class using
declarative syntax, you’ll easily be able to create classes using many Django features, both official and community-provided.
Looking at declarative syntax on its own will demonstrate how easy it is to create an entirely new framework for Django
that fits with this pattern. Using declarative syntax in your own code will help you and your colleagues more easily adapt to
the code, ensuring greater productivity. After all, developer efficiency is a primary goal of Django and of Python itself.
While the next few sections describe declarative syntax in general, the examples shown are for Django’s
object-relational mapper (ORM), detailed in Chapter 3.
Centralized Access
Typically, a package will supply a single module from which applications can access all the necessary utilities. This
module may pull the individual classes and functions from elsewhere in its tree, so they can still use maintainable
namespaces, but they will all be collected into one central location.
from django.db import models
Once imported, this module provides at least one class intended as the base class for subclasses based on the
framework. Additional classes are provided to be used as attributes of the new subclass. Together, these objects will
combine to control how the new class will work.
The Base Class
Each feature starts with at least one base class. There may be more, depending on the needs of the framework, but at
least one will always be required in order to make this syntax possible. Without it, every class you ask your users to define
will have to include a metaclass explicitly, which is an implementation detail most users shouldn’t need to know about.
class Contact(models.Model):
In addition to inspecting the defined attributes, this base class will provide a set of methods and attributes that
the subclass will automatically inherit. Like any other class, it can be as simple or complex as necessary to provide
whatever features the framework requires.
Attribute Classes
The module supplying the base class will also provide a set of classes to be instantiated, often with optional arguments
to customize their behavior and assigned as attributes of a new class.
class Contact(models.Model):
name = models.CharField(max_length=255)
email = models.EmailField()
The features these objects provide will vary greatly across frameworks, and some may behave quite differently
from a standard attribute. Often they will combine with the metaclass to provide some additional, behind-the-scenes
functionality beyond simply assigning an attribute. Options to these attribute classes are usually read by the metaclass
when creating this extra functionality.
www.allitebooks.com