没有合适的资源?快使用搜索试试~ 我知道了~
首页Java核心技术第8版英文非扫描全彩版详解
Java核心技术第8版英文非扫描全彩版详解

《Java核心技术卷1 第8版英文版非扫描》是一本由Gary Cornell编著的专业级Java编程教材,本书是该系列的第一卷,重点关注Java语言的基础知识和核心概念。作为第8版,它反映了Java技术的最新发展,适合那些希望深入理解Java编程语言的读者。
本书由Cay Horstmann与Cornell共同编写,涵盖了Java语言的基础理论,包括类、对象、继承、封装、多态性等核心特性。作者们以清晰易懂的方式阐述了Java语言的设计哲学和API使用,帮助读者建立起坚实的Java编程基础。
书中内容广泛,不仅介绍了Java语言本身,还包含了与之相关的Sun Microsystems(现Oracle Corporation)的技术,如Java ME、Solaris操作系统、J2ME、Javadoc、NetBeans等,这些都是Java生态系统的重要组成部分。对于初学者而言,这本书是学习现代企业级开发不可或缺的参考书籍。
值得注意的是,由于版权和商标问题,书中的某些制造商名称和产品标识如Sun Microsystems、Java、UNIX等使用了特定的格式,比如首字母大写或全大写,以表明它们是受法律保护的品牌。书中可能涉及的一些专利权,包括美国和国际范围内的专利申请,体现了Sun Microsystems在Java技术领域的知识产权保护。
《Java核心技术卷1 第8版英文版非扫描》不仅适合在校学生和专业开发者用于系统学习,也是技术培训和企业内部培训的重要教材,能够帮助读者不断提升Java编程技能,并紧跟技术发展趋势。无论是在个人自学还是团队协作项目中,这本书都是一个值得信赖的知识宝库。
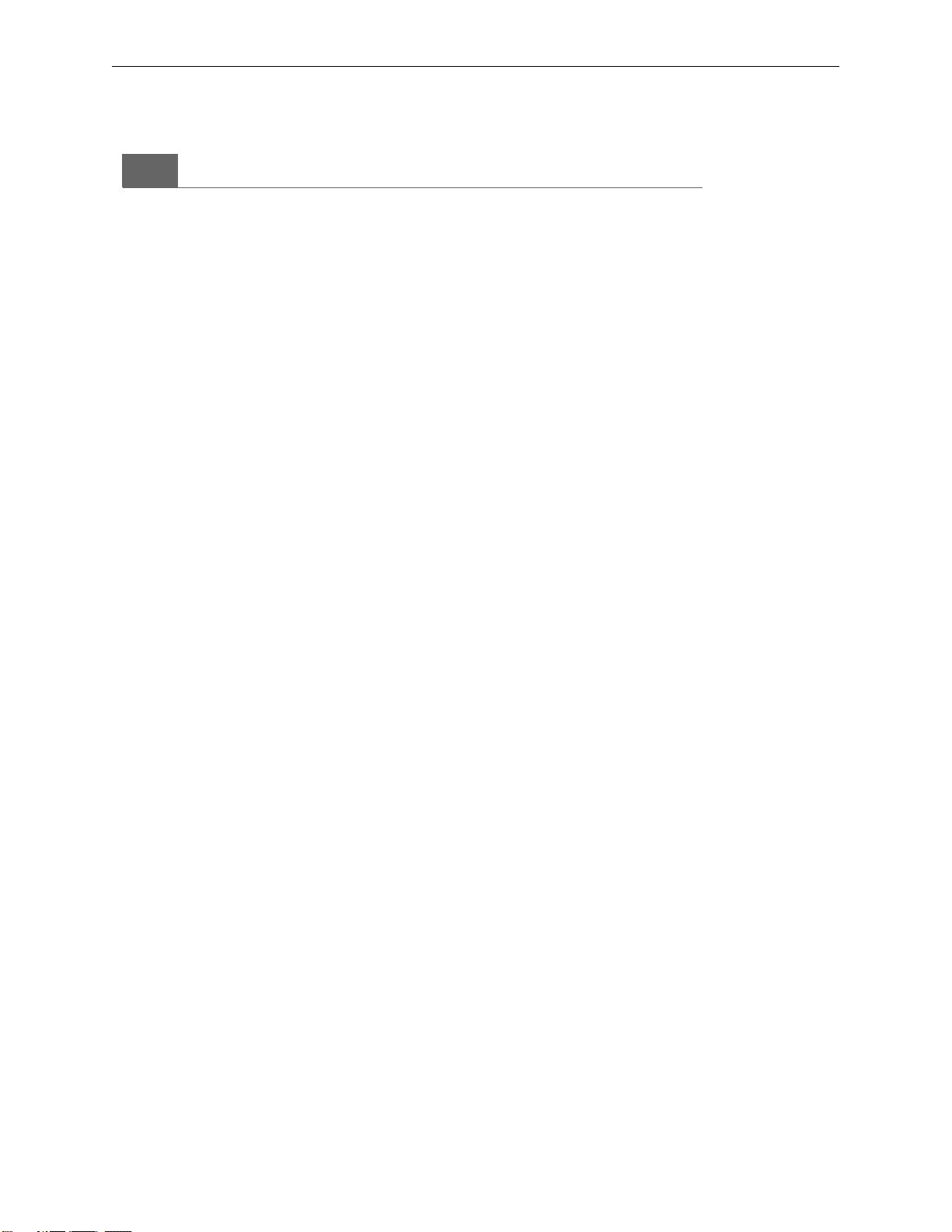
Chapter 1
■
An Introduction to Java
2
T
he first release of Java in 1996 generated an incredible amount of excitement, not
just in the computer press, but in mainstream media such as The New York Times, The
Washington Post, and Business Week. Java has the distinction of being the first and only
programming language that had a ten-minute story on National Public Radio. A
$100,000,000 venture capital fund was set up solely for products produced by use of a
specific computer language. It is rather amusing to revisit those heady times, and we
give you a brief history of Java in this chapter.
Java As a Programming Platform
In the first edition of this book, we had this to write about Java:
“As a computer language, Java’s hype is overdone: Java is certainly a good program-
ming language. There is no doubt that it is one of the better languages available to
serious programmers. We think it could potentially have been a great programming
language, but it is probably too late for that. Once a language is out in the field, the ugly
reality of compatibility with existing code sets in.”
Our editor got a lot of flack for this paragraph from someone very high up at Sun Micro-
systems who shall remain unnamed. But, in hindsight, our prognosis seems accurate.
Java has a lot of nice language features—we examine them in detail later in this chapter.
It has its share of warts, and newer additions to the language are not as elegant as the
original ones because of the ugly reality of compatibility.
But, as we already said in the first edition, Java was never just a language. There are lots
of programming languages out there, and few of them make much of a splash. Java is a
whole platform, with a huge library, containing lots of reusable code, and an execution
environment that provides services such as security, portability across operating sys-
tems, and automatic garbage collection.
As a programmer, you will want a language with a pleasant syntax and comprehensible
semantics (i.e., not C++). Java fits the bill, as do dozens of other fine languages. Some
languages give you portability, garbage collection, and the like, but they don’t have
much of a library, forcing you to roll your own if you want fancy graphics or network-
ing or database access. Well, Java has everything—a good language, a high-quality exe-
cution environment, and a vast library. That combination is what makes Java an
irresistible proposition to so many programmers.
The Java “White Paper” Buzzwords
The authors of Java have written an influential White Paper that explains their design
goals and accomplishments. They also published a shorter summary that is organized
along the following 11 buzzwords:
Simple Portable
Object Oriented Interpreted
Network-Savvy High Performance
Robust Multithreaded
Secure Dynamic
Architecture Neutral
Chapter 1. An Introduction to Java
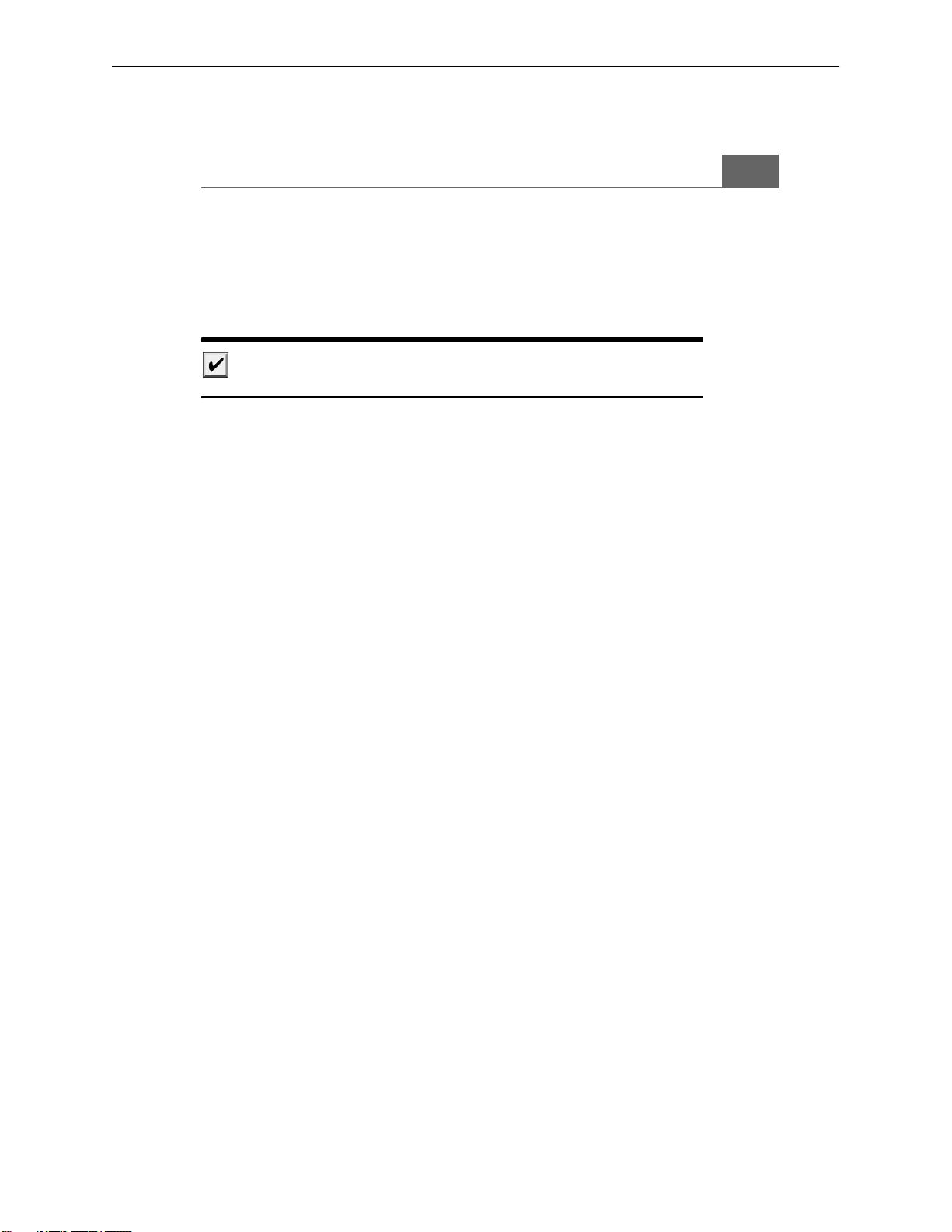
The Java “White Paper” Buzzwords
3
In this section, we will
• Summarize, with excerpts from the White Paper, what the Java designers say about
each buzzword; and
• Tell you what we think of each buzzword, based on our experiences with the cur-
rent version of Java.
NOTE: As we write this, the White Paper can be found at http://java.sun.com/docs/white/
langenv/. The summary with the 11 buzzwords is at http://java.sun.com/docs/overviews/java/
java-overview-1.html.
Simple
We wanted to build a system that could be programmed easily without a lot of eso-
teric training and which leveraged today’s standard practice. So even though we
found that C++ was unsuitable, we designed Java as closely to C++ as possible in
order to make the system more comprehensible. Java omits many rarely used, poorly
understood, confusing features of C++ that, in our experience, bring more grief
than benefit.
The syntax for Java is, indeed, a cleaned-up version of the syntax for C++. There is no
need for header files, pointer arithmetic (or even a pointer syntax), structures, unions,
operator overloading, virtual base classes, and so on. (See the C++ notes interspersed
throughout the text for more on the differences between Java and C++.) The designers
did not, however, attempt to fix all of the clumsy features of C++. For example, the syn-
tax of the
switch
statement is unchanged in Java. If you know C++, you will find the tran-
sition to the Java syntax easy.
If you are used to a visual programming environment (such as Visual Basic), you will
not find Java simple. There is much strange syntax (though it does not take long to get
the hang of it). More important, you must do a lot more programming in Java. The
beauty of Visual Basic is that its visual design environment almost automatically pro-
vides a lot of the infrastructure for an application. The equivalent functionality must be
programmed manually, usually with a fair bit of code, in Java. There are, however,
third-party development environments that provide “drag-and-drop”-style program
development.
Another aspect of being simple is being small. One of the goals of Java is to enable
the construction of software that can run stand-alone in small machines. The size of
the basic interpreter and class support is about 40K bytes; adding the basic stan-
dard libraries and thread support (essentially a self-contained microkernel) adds an
additional 175K.
This was a great achievement at the time. Of course, the library has since grown to huge
proportions. There is now a separate Java Micro Edition with a smaller library, suitable
for embedded devices.
Object Oriented
Simply stated, object-oriented design is a technique for programming that focuses
on the data (= objects) and on the interfaces to that object. To make an analogy with
carpentry, an “object-oriented” carpenter would be mostly concerned with the chair
Chapter 1. An Introduction to Java
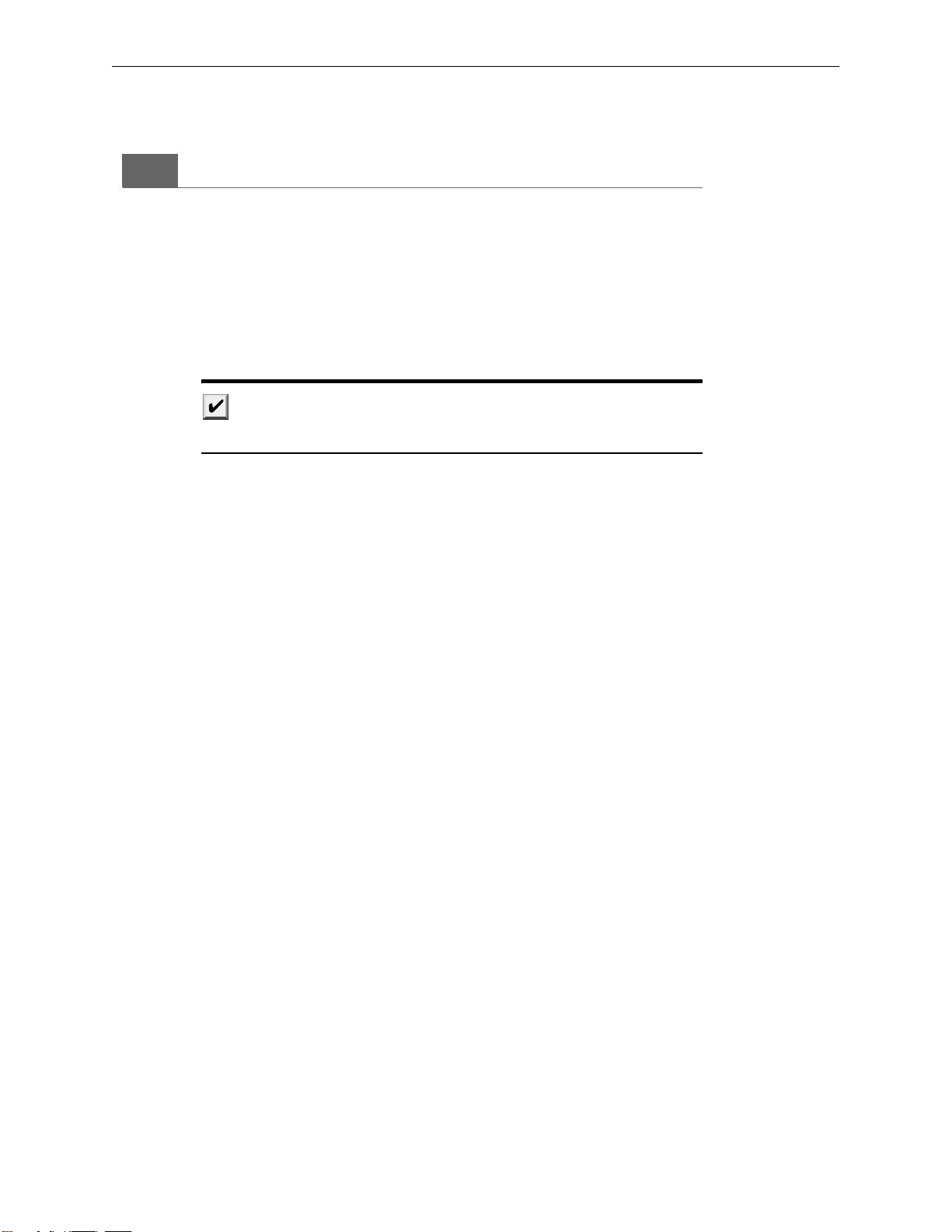
Chapter 1
■
An Introduction to Java
4
he was building, and secondarily with the tools used to make it; a “non-object-
oriented” carpenter would think primarily of his tools. The object-oriented facilities
of Java are essentially those of C++.
Object orientation has proven its worth in the last 30 years, and it is inconceivable that a
modern programming language would not use it. Indeed, the object-oriented features of
Java are comparable to those of C++. The major difference between Java and C++ lies in
multiple inheritance, which Java has replaced with the simpler concept of interfaces,
and in the Java metaclass model (which we discuss in Chapter 5).
NOTE: If you have no experience with object-oriented programming languages, you will
want to carefully read Chapters 4 through 6. These chapters explain what object-oriented
programming is and why it is more useful for programming sophisticated projects than are
traditional, procedure-oriented languages like C or Basic.
Network-Savvy
Java has an extensive library of routines for coping with TCP/IP protocols like
HTTP and FTP. Java applications can open and access objects across the Net via
URLs with the same ease as when accessing a local file system.
We have found the networking capabilities of Java to be both strong and easy to use.
Anyone who has tried to do Internet programming using another language will revel in
how simple Java makes onerous tasks like opening a socket connection. (We cover net-
working in Volume II of this book.) The remote method invocation mechanism enables
communication between distributed objects (also covered in Volume II).
Robust
Java is intended for writing programs that must be reliable in a variety of ways.
Java puts a lot of emphasis on early checking for possible problems, later dynamic
(runtime) checking, and eliminating situations that are error-prone. . . . The single
biggest difference between Java and C/C++ is that Java has a pointer model that elim-
inates the possibility of overwriting memory and corrupting data.
This feature is also very useful. The Java compiler detects many problems that, in other
languages, would show up only at runtime. As for the second point, anyone who has
spent hours chasing memory corruption caused by a pointer bug will be very happy
with this feature of Java.
If you are coming from a language like Visual Basic that doesn’t explicitly use pointers,
you are probably wondering why this is so important. C programmers are not so lucky.
They need pointers to access strings, arrays, objects, and even files. In Visual Basic, you
do not use pointers for any of these entities, nor do you need to worry about memory
allocation for them. On the other hand, many data structures are difficult to implement
in a pointerless language. Java gives you the best of both worlds. You do not need point-
ers for everyday constructs like strings and arrays. You have the power of pointers if
you need it, for example, for linked lists. And you always have complete safety, because
you can never access a bad pointer, make memory allocation errors, or have to protect
against memory leaking away.
Chapter 1. An Introduction to Java
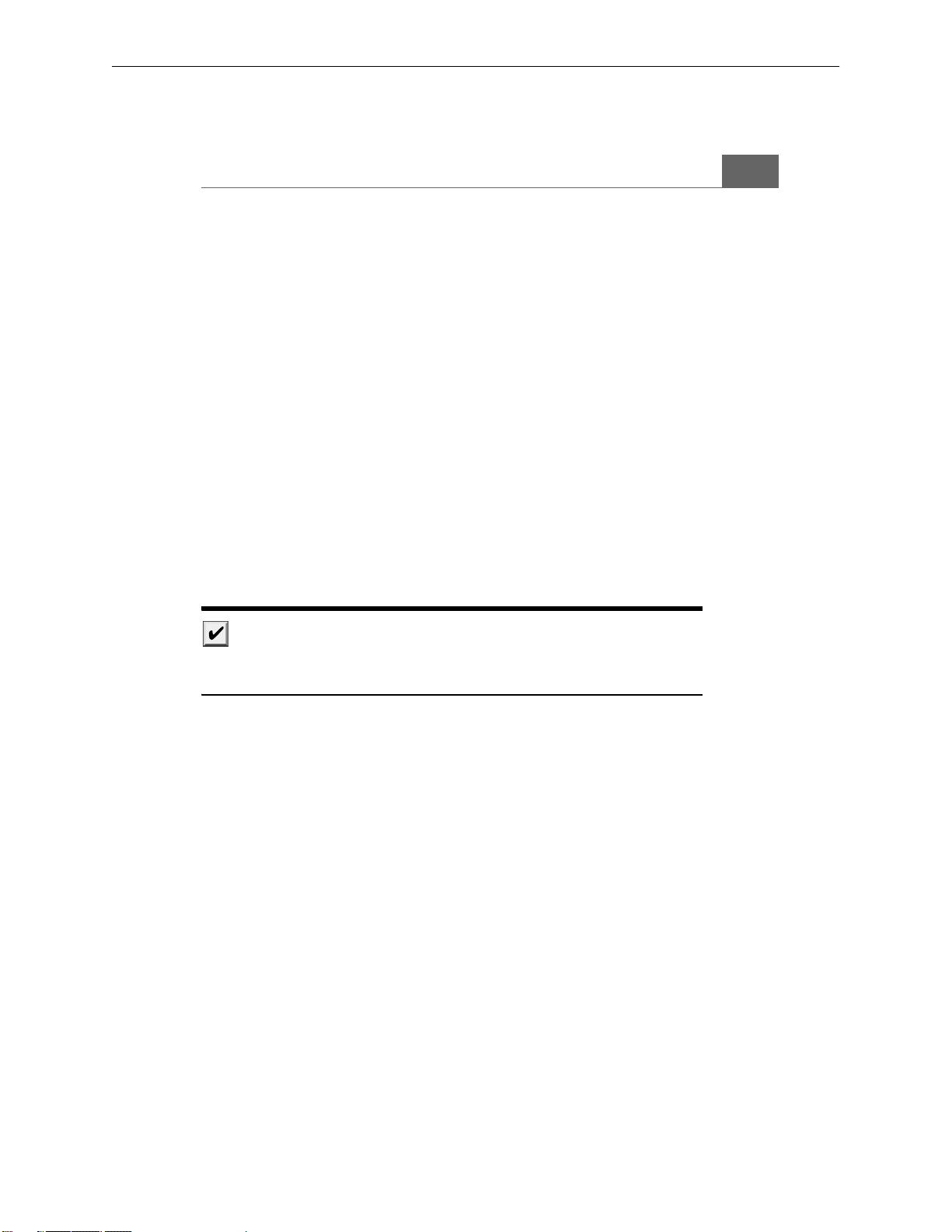
The Java “White Paper” Buzzwords
5
Secure
Java is intended to be used in networked/distributed environments. Toward that
end, a lot of emphasis has been placed on security. Java enables the construction of
virus-free, tamper-free systems.
In the first edition of Core Java we said: “Well, one should ‘never say never again,’” and
we turned out to be right. Not long after the first version of the Java Development Kit
was shipped, a group of security experts at Princeton University found subtle bugs in
the security features of Java 1.0. Sun Microsystems has encouraged research into Java
security, making publicly available the specification and implementation of the virtual
machine and the security libraries. They have fixed all known security bugs quickly. In
any case, Java makes it extremely difficult to outwit its security mechanisms. The bugs
found so far have been very technical and few in number.
From the beginning, Java was designed to make certain kinds of attacks impossible,
among them:
• Overrunning the runtime stack—a common attack of worms and viruses
• Corrupting memory outside its own process space
• Reading or writing files without permission
A number of security features have been added to Java over time. Since version 1.1, Java
has the notion of digitally signed classes (see Volume II). With a signed class, you can be
sure who wrote it. Any time you trust the author of the class, the class can be allowed
more privileges on your machine.
NOTE: A competing code delivery mechanism from Microsoft based on its ActiveX technol-
ogy relies on digital signatures alone for security. Clearly this is not sufficient—as any user
of Microsoft’s own products can confirm, programs from well-known vendors do crash and
create damage. Java has a far stronger security model than that of ActiveX because it con-
trols the application as it runs and stops it from wreaking havoc.
Architecture Neutral
The compiler generates an architecture-neutral object file format—the compiled
code is executable on many processors, given the presence of the Java runtime sys-
tem. The Java compiler does this by generating bytecode instructions which have
nothing to do with a particular computer architecture. Rather, they are designed to
be both easy to interpret on any machine and easily translated into native machine
code on the fly.
This is not a new idea. More than 30 years ago, both Niklaus Wirth’s original implemen-
tation of Pascal and the UCSD Pascal system used the same technique.
Of course, interpreting bytecodes is necessarily slower than running machine instruc-
tions at full speed, so it isn’t clear that this is even a good idea. However, virtual
machines have the option of translating the most frequently executed bytecode
sequences into machine code, a process called just-in-time compilation. This strategy
has proven so effective that even Microsoft’s .NET platform relies on a virtual machine.
Chapter 1. An Introduction to Java
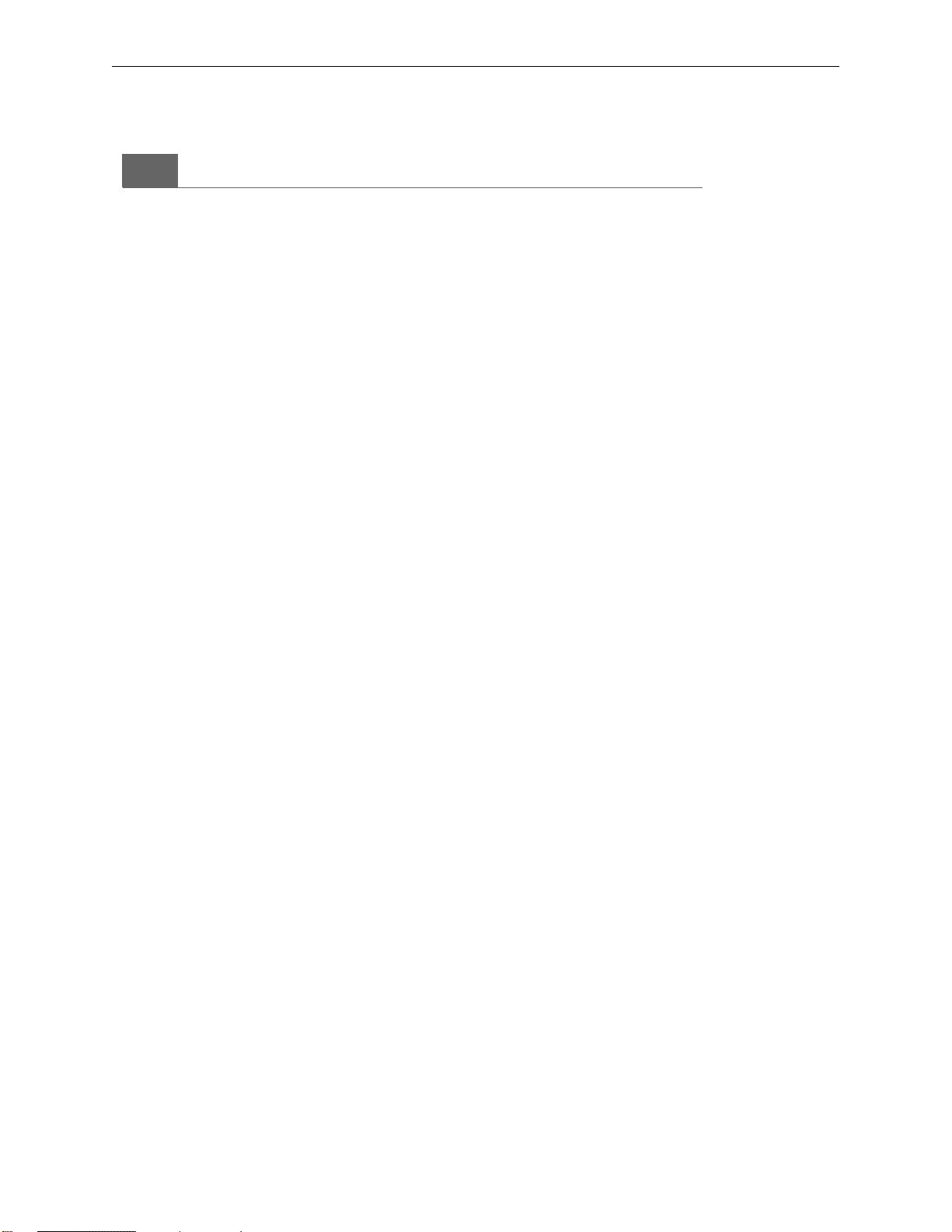
Chapter 1
■
An Introduction to Java
6
The virtual machine has other advantages. It increases security because the virtual
machine can check the behavior of instruction sequences. Some programs even produce
bytecodes on the fly, dynamically enhancing the capabilities of a running program.
Portable
Unlike C and C++, there are no “implementation-dependent” aspects of the specifi-
cation. The sizes of the primitive data types are specified, as is the behavior of arith-
metic on them.
For example, an
int
in Java is always a 32-bit integer. In C/C++,
int
can mean a 16-bit
integer, a 32-bit integer, or any other size that the compiler vendor likes. The only
restriction is that the
int
type must have at least as many bytes as a
short int
and cannot
have more bytes than a
long int
. Having a fixed size for number types eliminates a major
porting headache. Binary data is stored and transmitted in a fixed format, eliminating
confusion about byte ordering. Strings are saved in a standard Unicode format.
The libraries that are a part of the system define portable interfaces. For example,
there is an abstract Window class and implementations of it for UNIX, Windows,
and the Macintosh.
As anyone who has ever tried knows, it is an effort of heroic proportions to write a pro-
gram that looks good on Windows, the Macintosh, and ten flavors of UNIX. Java 1.0
made the heroic effort, delivering a simple toolkit that mapped common user interface
elements to a number of platforms. Unfortunately, the result was a library that, with a
lot of work, could give barely acceptable results on different systems. (And there were
often different bugs on the different platform graphics implementations.) But it was a
start. There are many applications in which portability is more important than user
interface slickness, and these applications did benefit from early versions of Java. By
now, the user interface toolkit has been completely rewritten so that it no longer relies
on the host user interface. The result is far more consistent and, we think, more attrac-
tive than in earlier versions of Java.
Interpreted
The Java interpreter can execute Java bytecodes directly on any machine to which
the interpreter has been ported. Since linking is a more incremental and lightweight
process, the development process can be much more rapid and exploratory.
Incremental linking has advantages, but its benefit for the development process is
clearly overstated. Early Java development tools were, in fact, quite slow. Today, the
bytecodes are translated into machine code by the just-in-time compiler.
High Performance
While the performance of interpreted bytecodes is usually more than adequate,
there are situations where higher performance is required. The bytecodes can be
translated on the fly (at runtime) into machine code for the particular CPU the
application is running on.
In the early years of Java, many users disagreed with the statement that the perfor-
mance was “more than adequate.” Today, however, the just-in-time compilers have
become so good that they are competitive with traditional compilers and, in some cases,
even outperform them because they have more information available. For example, a
just-in-time compiler can monitor which code is executed frequently and optimize just
Chapter 1. An Introduction to Java
剩余824页未读,继续阅读

Cap_zhou
- 粉丝: 0
- 资源: 9
上传资源 快速赚钱
我的内容管理 展开
我的资源 快来上传第一个资源
我的收益
登录查看自己的收益我的积分 登录查看自己的积分
我的C币 登录后查看C币余额
我的收藏
我的下载
下载帮助

最新资源
- 深入浅出:自定义 Grunt 任务的实践指南
- 网络物理突变工具的多点路径规划实现与分析
- multifeed: 实现多作者间的超核心共享与同步技术
- C++商品交易系统实习项目详细要求
- macOS系统Python模块whl包安装教程
- 掌握fullstackJS:构建React框架与快速开发应用
- React-Purify: 实现React组件纯净方法的工具介绍
- deck.js:构建现代HTML演示的JavaScript库
- nunn:现代C++17实现的机器学习库开源项目
- Python安装包 Acquisition-4.12-cp35-cp35m-win_amd64.whl.zip 使用说明
- Amaranthus-tuberculatus基因组分析脚本集
- Ubuntu 12.04下Realtek RTL8821AE驱动的向后移植指南
- 掌握Jest环境下的最新jsdom功能
- CAGI Toolkit:开源Asterisk PBX的AGI应用开发
- MyDropDemo: 体验QGraphicsView的拖放功能
- 远程FPGA平台上的Quartus II17.1 LCD色块闪烁现象解析
安全验证
文档复制为VIP权益,开通VIP直接复制
