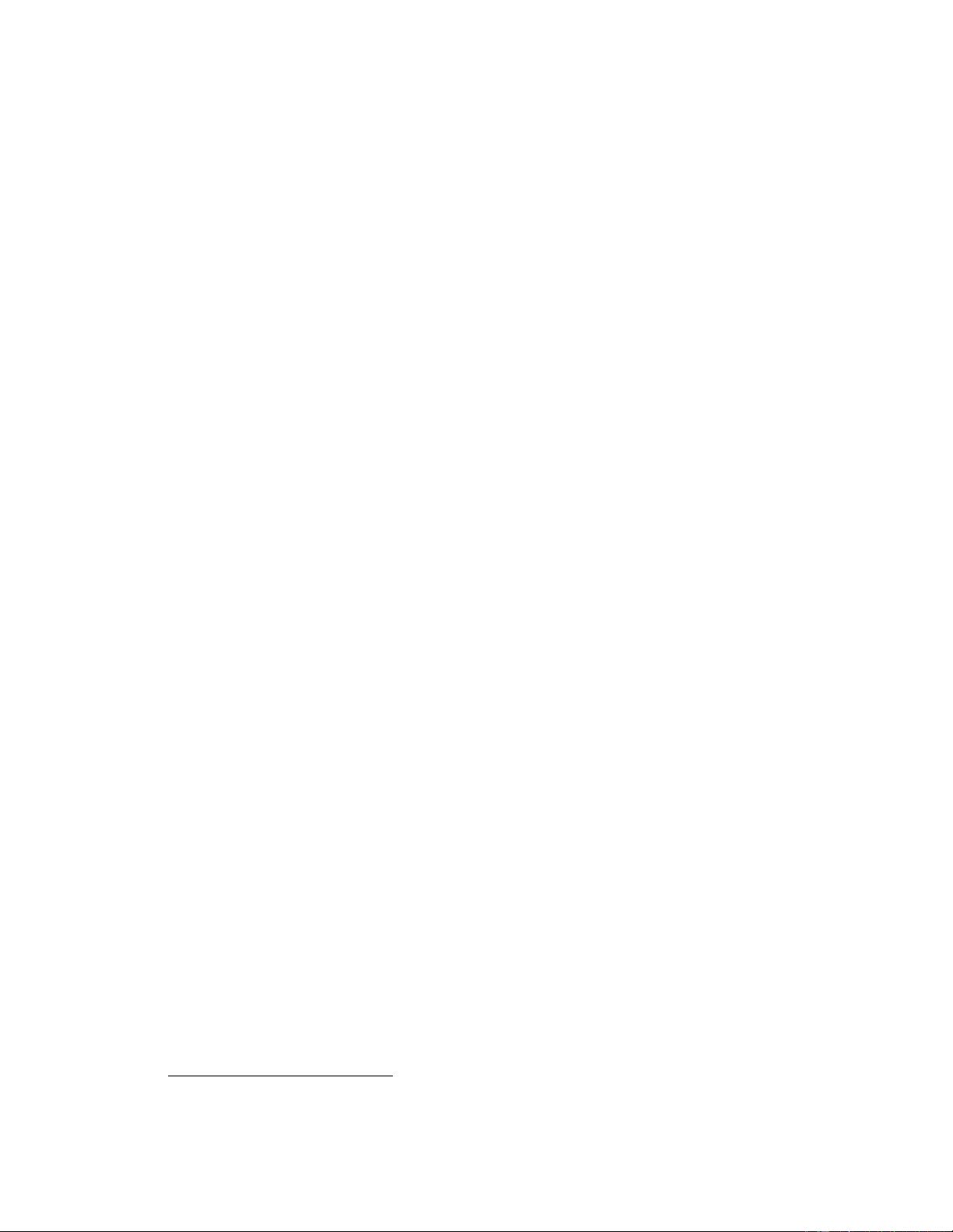
19
• Array Basics
5. Array Basics
This chapter introduces some of the basic functions which will be used throughout the text.
Basics
Before we explore the world of image manipulation as a case-study in array manipulation, we should first de-
fine a few terms which we’ll use over and over again. Discussions of arrays and matrices and vectors can get
confusing due to disagreements on the nomenclature. Here is a brief definition of the terms used in this tutorial,
and more or less consistently in the error messages of NumPy.
The python objects under discussion are formally called “multiarray” objects, but informally we’ll just call
them “array” objects or just “arrays.” These are different from the array objects defined in the standard array
module (which is designed at processing one-dimensional data such as sound files).
These array objects are arrays in the computer science meaning of homogeneous block of elements, i.e. their el-
ements all have the same “kind” (usually, a specific kind of number, such as a 64-bit integer). This is quite dif-
ferent from most Python container objects, which can contain heterogeneous collections. Imposing this
restriction was a necessary step for a fast implementation, and in practice, it is not a cumbersome restriction in
many cases
1
.
Any given array object has a rank, which is the number of “dimensions” or “axes” it has. For example, a point
in 3D space [1, 2, 1] is an array of rank 1 – it has one dimension. That dimension has a
length
of 3.
As another example, the array
1.0 0.0 0.0
0.0 1.0 2.0
is an array of rank 2 (it is 2-dimensional). The first dimension has a length of 2, the second dimension has a
length of 3. Because the word “dimension” has many different meanings to different folks, in general the word
“axis” will be used instead. Axes are numbered just like Python list indices: they start at 0, and can also be
counted from the end, so that axis -1 is the last axis of an array, axis -2 is the penultimate axis, etc.
There are two important and potentially unintuitive behaviors of NumPy arrays which take some getting used
to. The first is that by default, operations on arrays are performed element-wise. This means that when adding
two arrays, the resulting array has as elements the pairwise sums of the two operand arrays. This is true for all
operations, including multiplication. Thus, array multiplication using the * operator will default to element-
wise multiplication, not matrix multiplication as used in linear algebra. Many people will want to use arrays as
linear algebra-type matrices (including their rank-1 versions, vectors). For those users, the Matrix class pro-
vides a more intuitive interface. We defer discussion of the Matrix class until later. The second behavior which
will catch many users by surprise is that functions which return arrays which are simply different views at the
same data will in fact
share
their data. This will be discussed at length when we have more concrete examples
of what exactly this means.
Now that all of these definitions and warnings are laid out, let's see what we can do with these arrays.
1. As we’ll also see, one valid “object kind” is a Python reference – in other words, these arrays can be
made heterogeneous at the cost of speed.