没有合适的资源?快使用搜索试试~ 我知道了~
首页精通iOS 6开发:挑战极限
精通iOS 6开发:挑战极限
需积分: 10 2 下载量 148 浏览量
更新于2024-07-24
收藏 52.85MB PDF 举报
"iOS 6 Programming Pushing the Limits"
这本书,"iOS 6 Programming: Pushing the Limits" 是针对已经具备基础的iOS开发者的一本高级指南,旨在帮助他们深入理解和利用iOS 6平台的新特性和API,以开发出卓越的iPhone、iPad和iPod touch应用程序。随着iOS成为全球最热门的开发平台,iOS 6的发布带来了更多的可能性,书中涵盖了如何通过Apple的iPhone SDK 6.0充分利用这些新功能,包括针对新iPad的应用程序构建。
在本书中,读者将学习到以下关键知识点:
1. **安全性**:探讨如何在iOS平台上实现强大的安全措施,保护用户数据和应用程序的安全,这对于任何面向市场的应用都是至关重要的。
2. **多任务处理**:了解如何有效地控制和管理多任务,使应用能够在后台运行而不会消耗过多资源,同时保持用户体验的流畅。
3. **内购机制**:通过内购增加收入是移动应用常见的商业模式,书里会讲解如何集成并优化这一功能,以提高应用的盈利能力。
4. **Cocoa中的键值观察(Key-Value Observing, KVO)**:掌握KVO机制,用于监听对象属性变化,从而实现灵活的数据绑定和实时更新视图。
5. **跨平台兼容**:学习如何编写能够在不同iOS设备上运行的代码,适应iPhone、iPad和iPod touch的屏幕尺寸和性能差异。
6. **高级文本布局**:深入研究iOS中的文本处理,包括复杂的排版和格式化,以提供更高级的用户体验。
7. **块(Blocks)与函数式编程**:理解并运用Objective-C的块语言特性,以及如何利用它们实现函数式编程风格,简化代码并提高效率。
8. **Core Foundation**:构建基于Core Foundation的坚实基础,这是一套底层库,为许多其他Apple框架提供了基础服务。
9. **新API**:详细介绍iOS 6引入的主要新API,如地图服务、社交整合等,这些都是提升应用功能的关键。
通过这本书,开发者可以提升他们的技能,创作出更具吸引力、性能更优且盈利潜力更大的iOS应用。无论是对于个人开发者还是团队,这本书都提供了丰富的实践指导和深入的技术洞察。
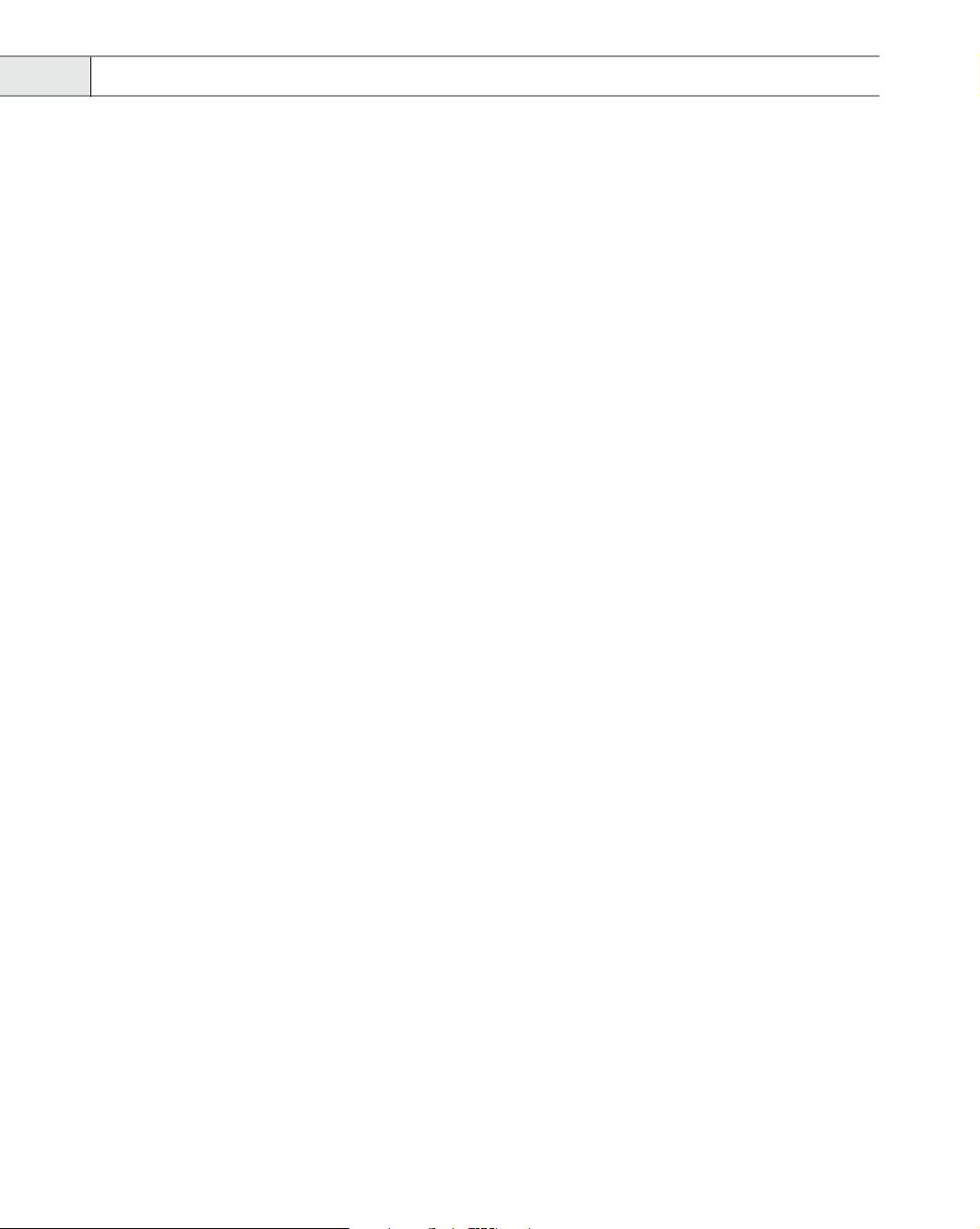
xiv Contents
Part III: The Right Tool for the Job ............................ 197
Chapter 12 Common UI Paradigms Using Table Views .................... 199
Pull-To-Refresh ................................................................199
Infinite Scrolling ..............................................................202
Inline Editing and Keyboard ...................................................204
Animating a UITableView ......................................................206
Practical Implementations of Table View Animations ...........................208
Summary .....................................................................211
Further Reading ...............................................................212
Chapter 13 Controlling Multitasking..................................... 213
Best Practices for Backgrounding: With Great Power
Comes Great Responsibility .................................................213
When We Left Our Heroes: State Restoration ...................................215
Introduction to Multitasking and Run Loops ...................................223
Developing Operation-Centric Multitasking .................................... 224
Multitasking with Grand Central Dispatch ...................................... 229
Summary .....................................................................235
Further Reading ...............................................................236
Chapter 14 REST for the Weary ........................................... 237
The REST Philosophy ..........................................................238
Choosing Your Data Exchange Format .........................................238
A Hypothetical Web Service ...................................................241
Important Reminders .........................................................242
RESTfulEngine Architecture (iHotelApp Sample Code) .......................... 243
Summary .....................................................................258
Further Reading ...............................................................258
Chapter 15 Batten the Hatches with Security Services .................... 261
Understanding the iOS Sandbox ............................................... 261
Securing Network Communications ...........................................263
Employing File Protection .....................................................270
Using Keychains ..............................................................272
Using Encryption ..............................................................274
Summary .....................................................................287
Further Reading ...............................................................287
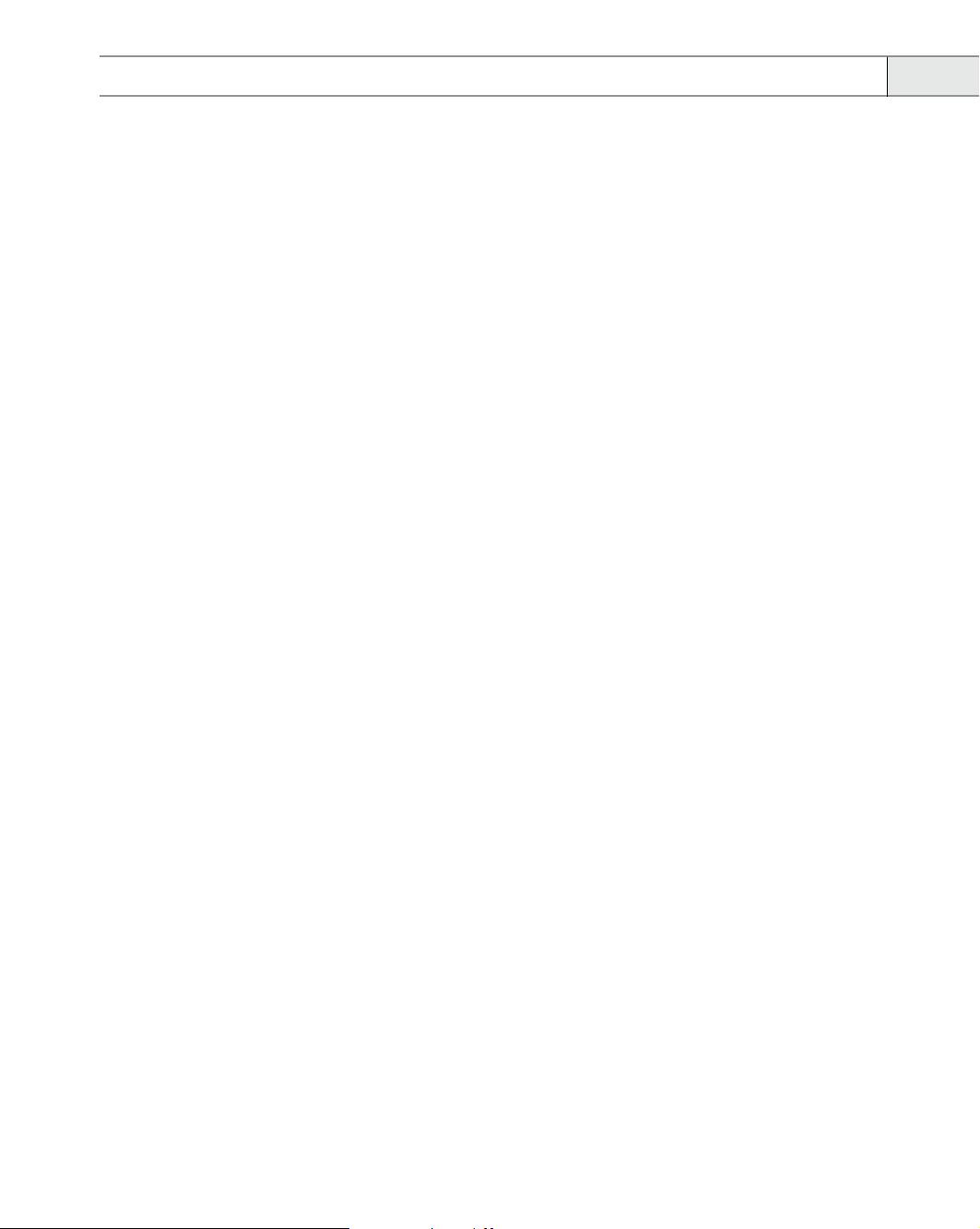
Contents xv
Chapter 16 Running on Multiple iPlatforms and iDevices ................. 289
Developing for Multiple Platforms .............................................290
Detecting Device Capabilities .................................................294
In App Email and SMS .........................................................300
Checking Multitasking Awareness .............................................300
Supporting the iPhone 5 ......................................................301
UIRequiredDeviceCapabilities .................................................303
Summary .....................................................................304
Further Reading ...............................................................304
Chapter 17 Internationalization and Localization......................... 305
What Is Localization? ..........................................................305
Localizing Strings .............................................................306
Auditing for Nonlocalized Strings .............................................. 307
Formatting Numbers and Dates ...............................................309
Nib Files and Base Internationalization ......................................... 312
Localizing Complex Strings .................................................... 312
Summary .....................................................................315
Further Reading ...............................................................315
Chapter 18 Selling Past the Sale with In App Purchases................... 317
Before You Start ...............................................................317
In App Purchase Products .....................................................317
Setting Up Products on iTunes Connect ........................................320
In App Purchase Implementation ..............................................326
Introduction to MKStoreKit ....................................................327
Making the Purchase ..........................................................331
Downloading Hosted Content ................................................. 332
Testing Your Code ............................................................332
Troubleshooting ..............................................................333
Summary .....................................................................334
Further Reading ...............................................................334
Chapter 19 Debugging.................................................. 335
LLDB. . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 335
Debugging with LLDB ......................................................... 335
Breakpoints ...................................................................338
Watchpoints ..................................................................343
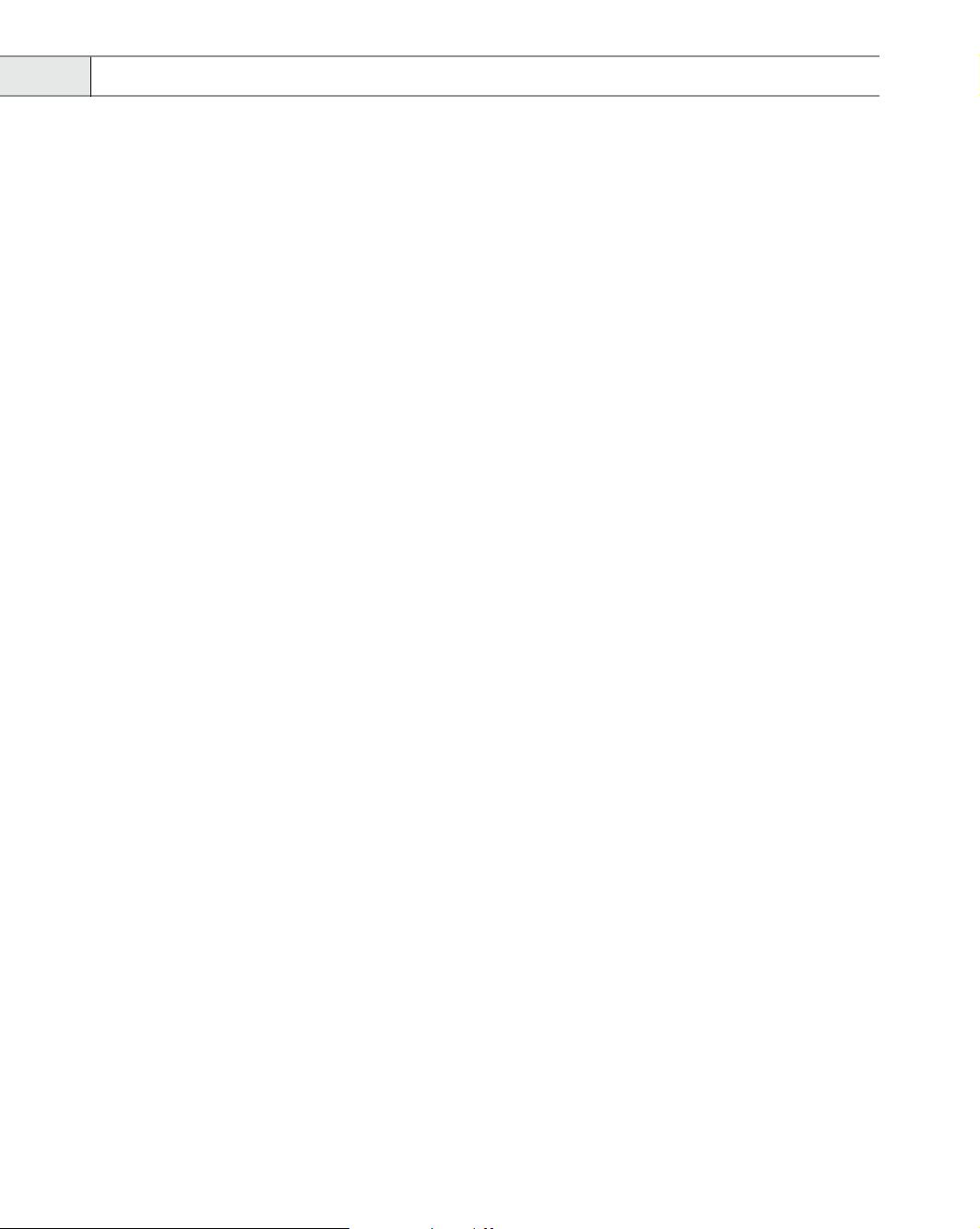
xvi Contents
The LLDB Console ............................................................. 344
NSZombieEnabled Flag .......................................................348
Different Types of Crashes ..................................................... 348
Collecting Crash Reports ......................................................352
Third-Party Crash Reporting Services. . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 353
Summary .....................................................................354
Further Reading ...............................................................354
Chapter 20 Performance Tuning Until It Flies ............................. 357
The Performance Mindset .....................................................357
Welcome to Instruments ...................................................... 358
Finding Memory Problems ....................................................360
Finding CPU Problems ........................................................364
Drawing Performance .........................................................368
Optimizing Disk and Network Access ..........................................371
Summary .....................................................................371
Further Reading ...............................................................372
Part IV: Pushing the Limits ................................... 373
Chapter 21 Storyboards and Custom Transitions ......................... 375
Getting Started with Storyboards ..............................................375
Custom Transitions ............................................................380
Customizing Your Views Using UIAppearance Protocol .........................382
Summary .....................................................................383
Further Reading ...............................................................383
Chapter 22 Cocoa’s Biggest Trick: Key-Value Coding and Observing ....... 385
Key-Value Coding .............................................................385
Key-Value Observing ..........................................................395
KVO Tradeoffs .................................................................399
Summary .....................................................................400
Further Reading ...............................................................401
Chapter 23 Think Different: Blocks and Functional Programming ......... 403
What Is a Block? ...............................................................403
Declaring a Block ..............................................................406
Implementing a Block .........................................................408
Blocks and Concurrency .......................................................410
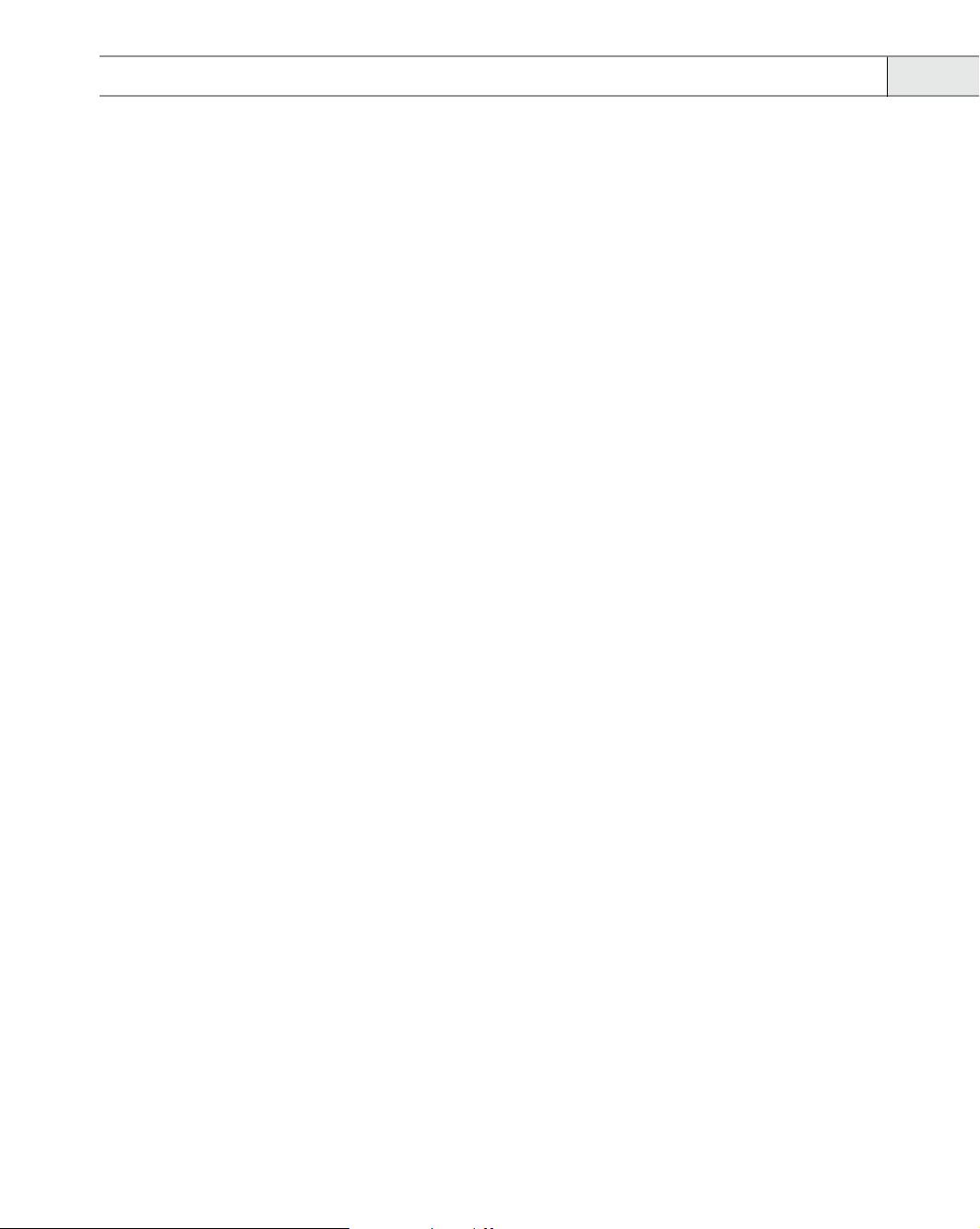
Contents xvii
The LLDB Console ............................................................. 344
NSZombieEnabled Flag .......................................................348
Different Types of Crashes ..................................................... 348
Collecting Crash Reports ......................................................352
Third-Party Crash Reporting Services. . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 353
Summary .....................................................................354
Further Reading ...............................................................354
Chapter 20 Performance Tuning Until It Flies ............................. 357
The Performance Mindset .....................................................357
Welcome to Instruments ...................................................... 358
Finding Memory Problems ....................................................360
Finding CPU Problems ........................................................364
Drawing Performance .........................................................368
Optimizing Disk and Network Access ..........................................371
Summary .....................................................................371
Further Reading ...............................................................372
Part IV: Pushing the Limits ................................... 373
Chapter 21 Storyboards and Custom Transitions ......................... 375
Getting Started with Storyboards ..............................................375
Custom Transitions ............................................................380
Customizing Your Views Using UIAppearance Protocol .........................382
Summary .....................................................................383
Further Reading ...............................................................383
Chapter 22 Cocoa’s Biggest Trick: Key-Value Coding and Observing ....... 385
Key-Value Coding .............................................................385
Key-Value Observing ..........................................................395
KVO Tradeoffs .................................................................399
Summary .....................................................................400
Further Reading ...............................................................401
Chapter 23 Think Different: Blocks and Functional Programming ......... 403
What Is a Block? ...............................................................403
Declaring a Block ..............................................................406
Implementing a Block .........................................................408
Blocks and Concurrency .......................................................410
Block-Based Cocoa Methods ...................................................412
Supported Platforms ..........................................................415
Summary .....................................................................415
Further Reading ...............................................................415
Chapter 24 Going Offline................................................ 417
Reasons for Going Offline .....................................................417
Strategies for Caching ......................................................... 418
Data Model Cache. . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 422
Cache Versioning .............................................................426
Creating an In-Memory Cache .................................................427
Creating a URL Cache .........................................................431
Summary .....................................................................433
Further Reading ...............................................................433
Chapter 25 Data in the Cloud............................................ 435
iCloud ........................................................................435
Third-Party Cloud Offerings. . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 437
Parse .........................................................................438
StackMob ..................................................................... 442
Disadvantages of Using a Backend as a Service ................................. 444
Summary .....................................................................444
Further Reading ...............................................................444
Chapter 26 Fancy Text Layout ........................................... 447
The Normal Stuff: Fields, Views, and Labels .....................................447
Rich Text in UIKit ..............................................................448
Web Views for Rich Text .......................................................453
Core Text .....................................................................454
Summary .....................................................................464
Further Reading ...............................................................464
Chapter 27 Building a (Core) Foundation ................................. 465
Core Foundation Types ........................................................465
Naming and Memory Management ............................................466
Allocators ..................................................................... 467
Introspection .................................................................468
Strings and Data .............................................................. 469
Collections ....................................................................474
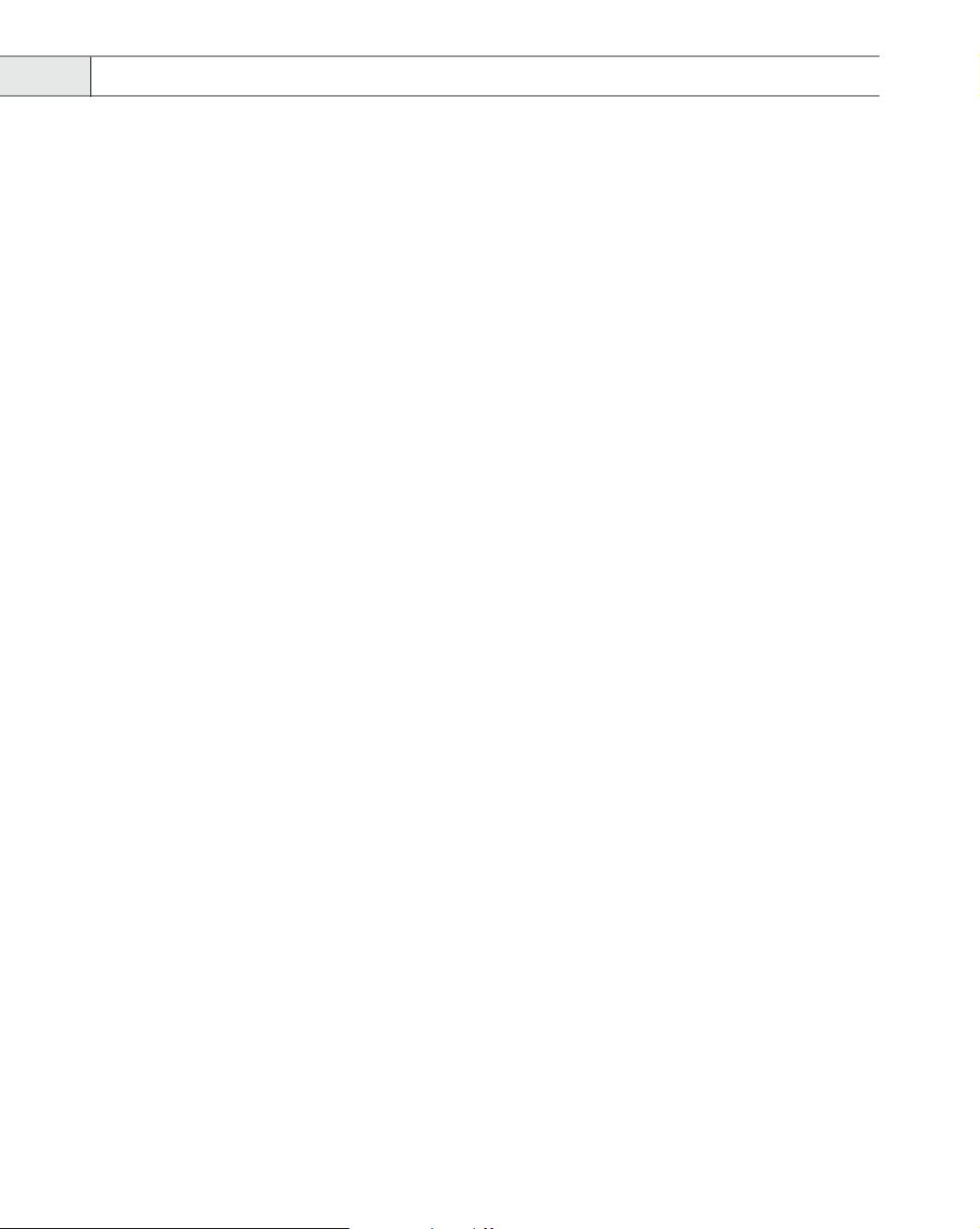
xviii Contents
Toll-Free Bridging .............................................................477
Summary .....................................................................480
Further Reading ...............................................................480
Chapter 28 Deep Objective-C ............................................ 481
Understanding Classes and Objects ............................................481
Working with Methods and Properties .........................................483
How Message Passing Really Works ............................................ 486
Method Swizzling .............................................................494
ISA Swizzling ..................................................................496
Method Swizzling Versus ISA Swizzling ........................................498
Summary .....................................................................498
Further Reading ...............................................................498
Index ..........................................................................501
剩余553页未读,继续阅读

csz0102
- 粉丝: 1
上传资源 快速赚钱
我的内容管理 展开
我的资源 快来上传第一个资源
我的收益
登录查看自己的收益我的积分 登录查看自己的积分
我的C币 登录后查看C币余额
我的收藏
我的下载
下载帮助

最新资源
- 网络电视压缩包内容解析
- Verilog实现贪吃蛇游戏的FPGA源码解析
- iOS PanCardView动画拖动效果实现教程
- Eclipse插件spket-1.6.23实现JS和JQuery代码提示功能
- Angular自定义组合框指令及模糊搜索功能介绍
- C#实现Textbox智能提示功能指南
- STM32MP157单通道ADC采集DMA读取HAL库驱动程序
- 将Woz的SWEET16 16位处理器移植至C64的Kick汇编程序
- MATLAB时频分析工具箱TFTB-0.2使用教程
- Netty实例5.0:全面解析IO通信框架及其应用
- 基于51单片机的16按键计算器设计与实现
- iOS开发中MBProgressHUD网络加载视图的应用
- STM32MP157 HAL库驱动PCF8563实时时钟程序教程
- 淘宝卖家不可或缺的钻展教程指南
- librender渲染器: C++实现的单对象渲染技术
- 安卓设备USB驱动安装与更新教程
安全验证
文档复制为VIP权益,开通VIP直接复制
