C++标准库参考指南
"C++ Standard Library Reference.pdf 是一份由微软提供的关于C++标准库的参考文档,方便查询标准库中的头文件、类和其他相关组件。它详细介绍了C++标准库的各种功能,包括容器、算法、分配器、原子操作、位集等,并提供了相关函数、操作符和类的详细说明。" 在C++编程中,标准库是至关重要的组成部分,它提供了一系列预先定义好的工具,可以帮助开发者高效地编写代码。这份文档详细涵盖了以下几个主要方面: 1. **头文件**:例如`<algorithm>`、`<array>`和`<atomic>`等,这些头文件包含了许多实用的功能,如排序、查找、内存管理等。`<algorithm>`包含了一整套用于处理容器中元素的算法,如排序(sort)、查找(find)和拷贝(copy)等;`<array>`提供了固定大小数组的封装,类似于C语言的数组但带有更多安全特性;`<atomic>`则提供了线程安全的原子操作,用于多线程环境。 2. **类和结构体**:如`allocator_base`、`bitset`、`atomic`和`chrono::duration`等。`allocator`系列类是C++中用于内存分配的模板类,可以自定义内存管理策略;`bitset`类提供了位运算的功能,可以用来存储和操作二进制数据;`atomic`结构体支持原子操作,防止并发访问时的数据竞争;`chrono::duration`则表示时间间隔,是`chrono`库的一部分,用于处理时间点和时间间隔。 3. **函数和操作符**:如`<algorithm>`中的函数、`<atomic>`的操作符等。这些函数和操作符提供了各种操作,如比较、赋值、算术运算等,使得开发者能够有效地使用标准库。 4. **枚举和常量**:例如 `<atomic>` 中的枚举,用于定义不同的原子操作类型。这些枚举常用于指定特定操作的行为或限制。 5. **时间相关**:如`<chrono>`库中的`chrono_literals`、`system_clock`和`time_point`等,它们提供了处理时间和日期的标准方法,支持高精度时间计算。 6. **其他组件**:如`<cassert>`用于断言,确保程序满足某些条件;`<ccomplex>`支持复数运算;`<cctype>`提供字符分类和转换函数;`<cerrno>`包含了错误码;`<cfenv>`处理浮点环境;`<cfloat>`提供了浮点数相关的常量和宏;`<chrono>`涉及时间日期操作;`<cfenv>`处理浮点环境;`<cfloat>`包含了浮点数的特性;`<iostream>`则涉及输入输出流。 通过这份参考文档,开发者能够快速查找并理解C++标准库中的各类组件,从而更有效地利用这些工具来编写高效、可靠的代码。对于C++程序员来说,这是一份非常有价值的参考资料。
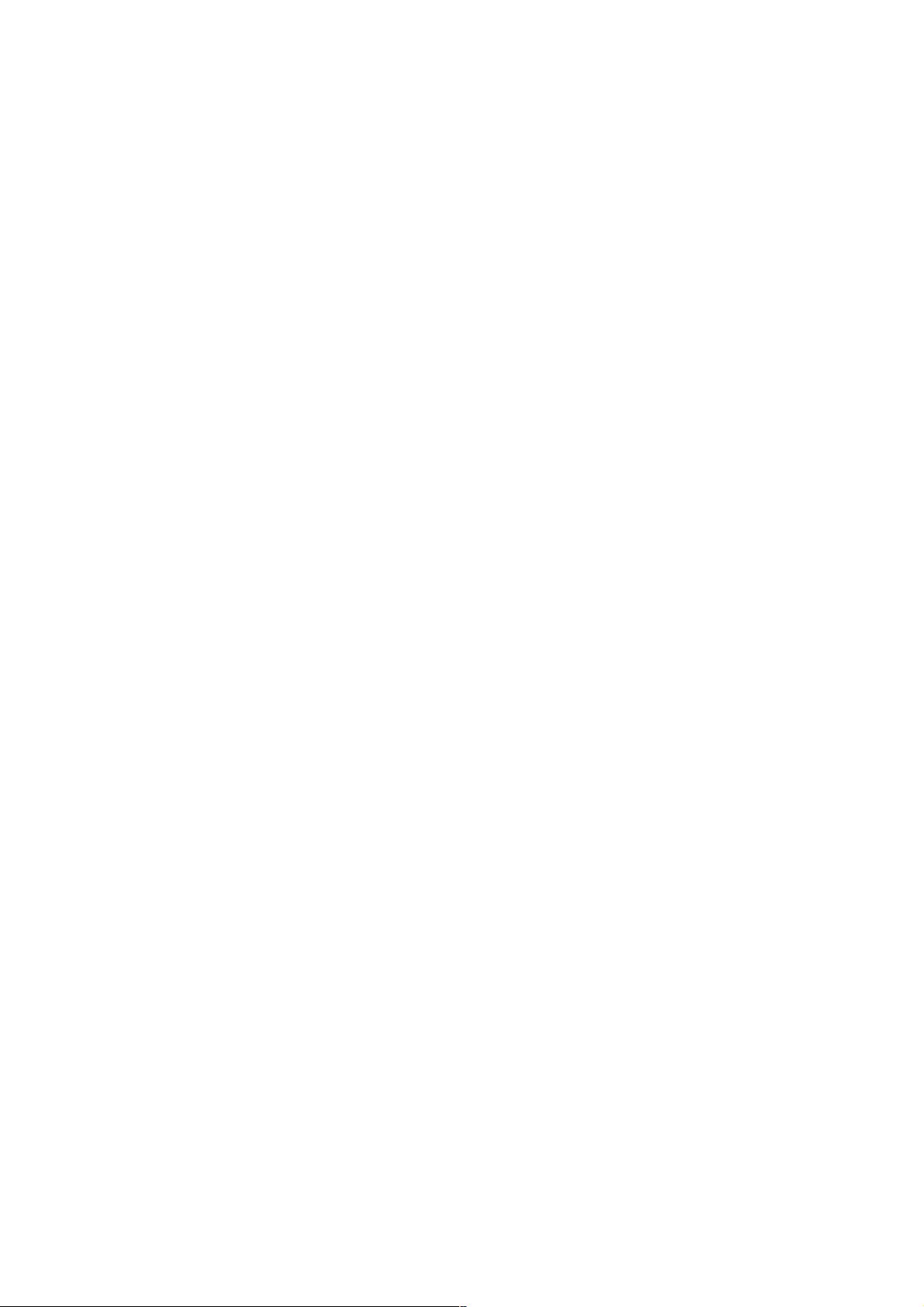
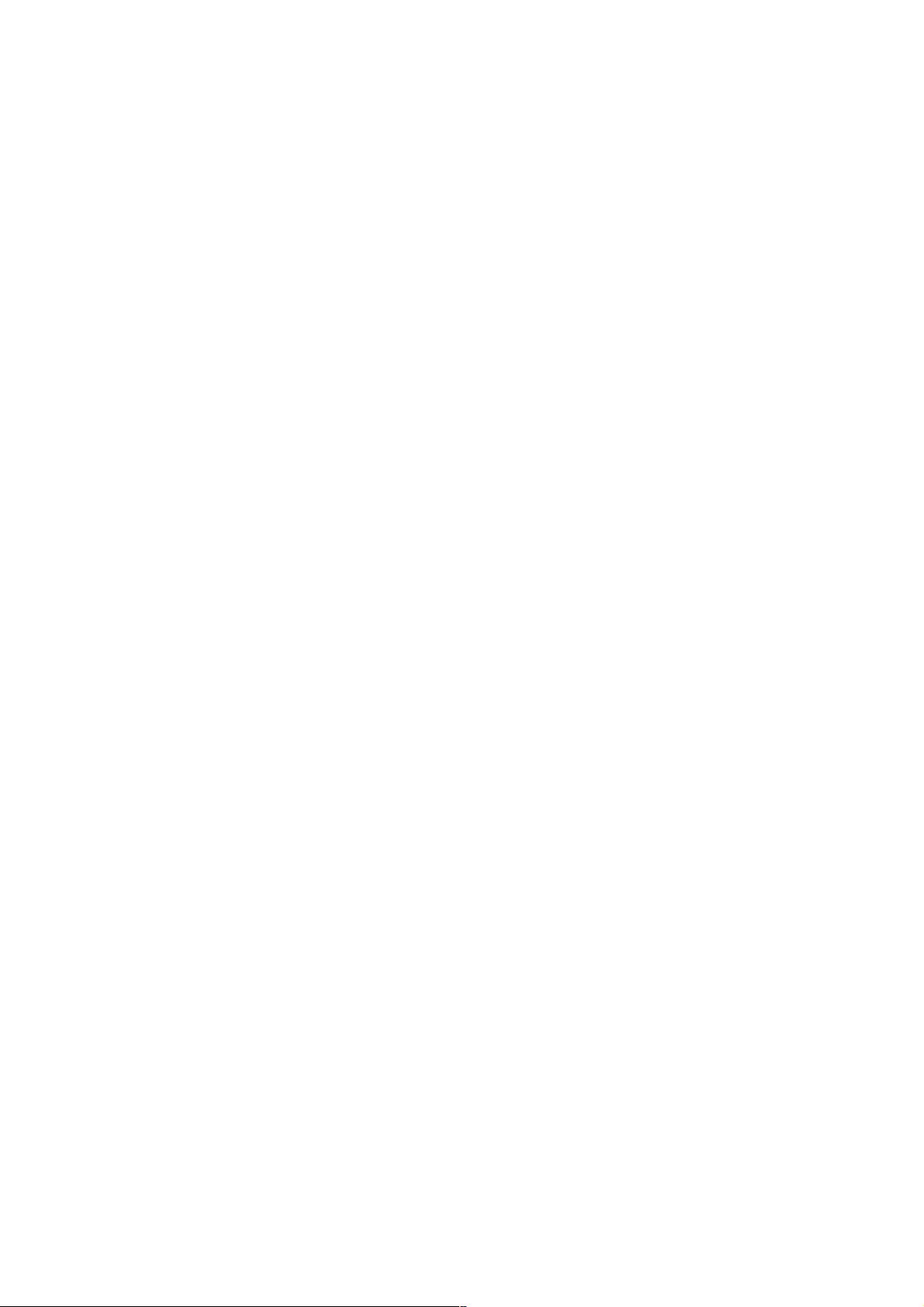
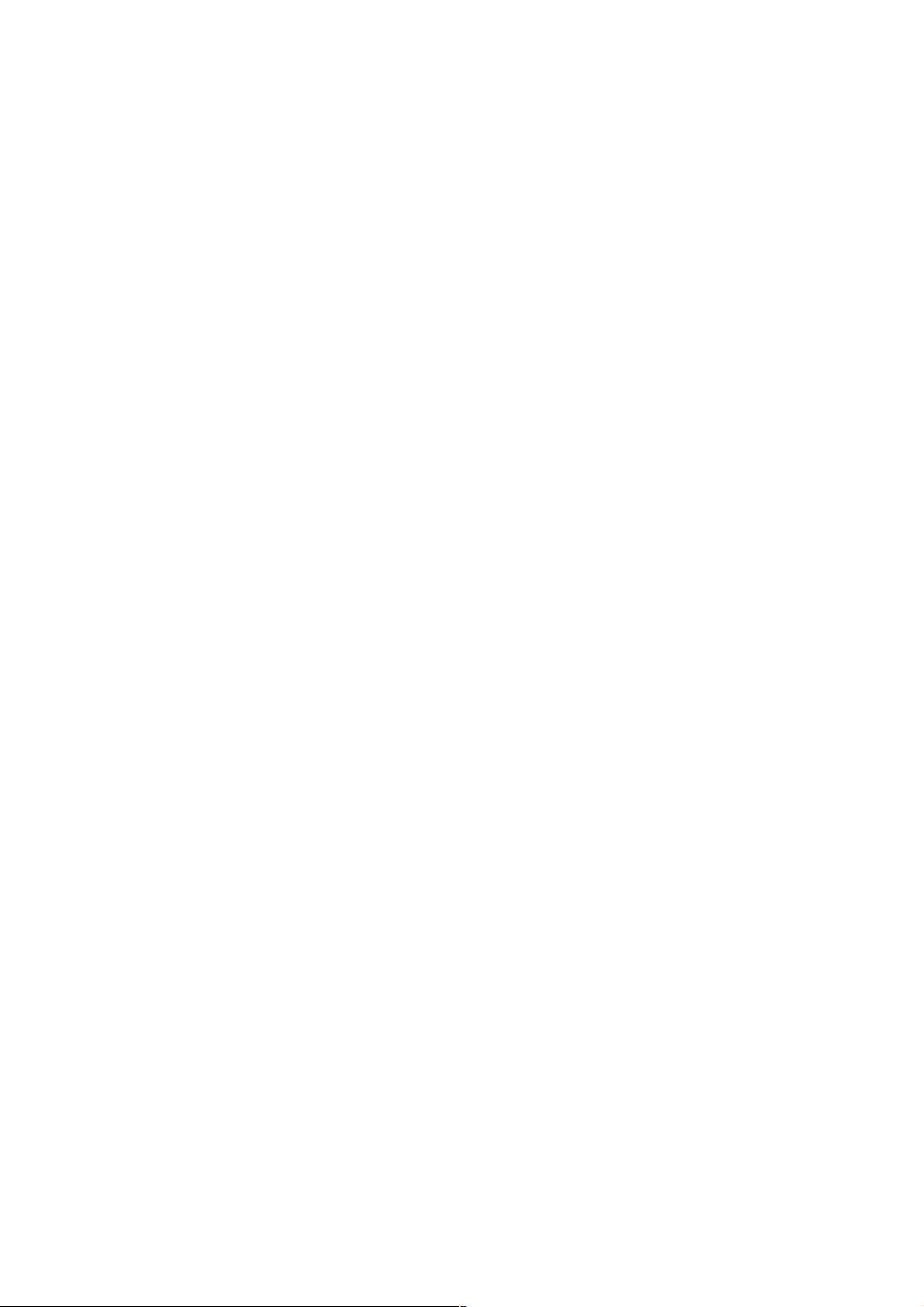
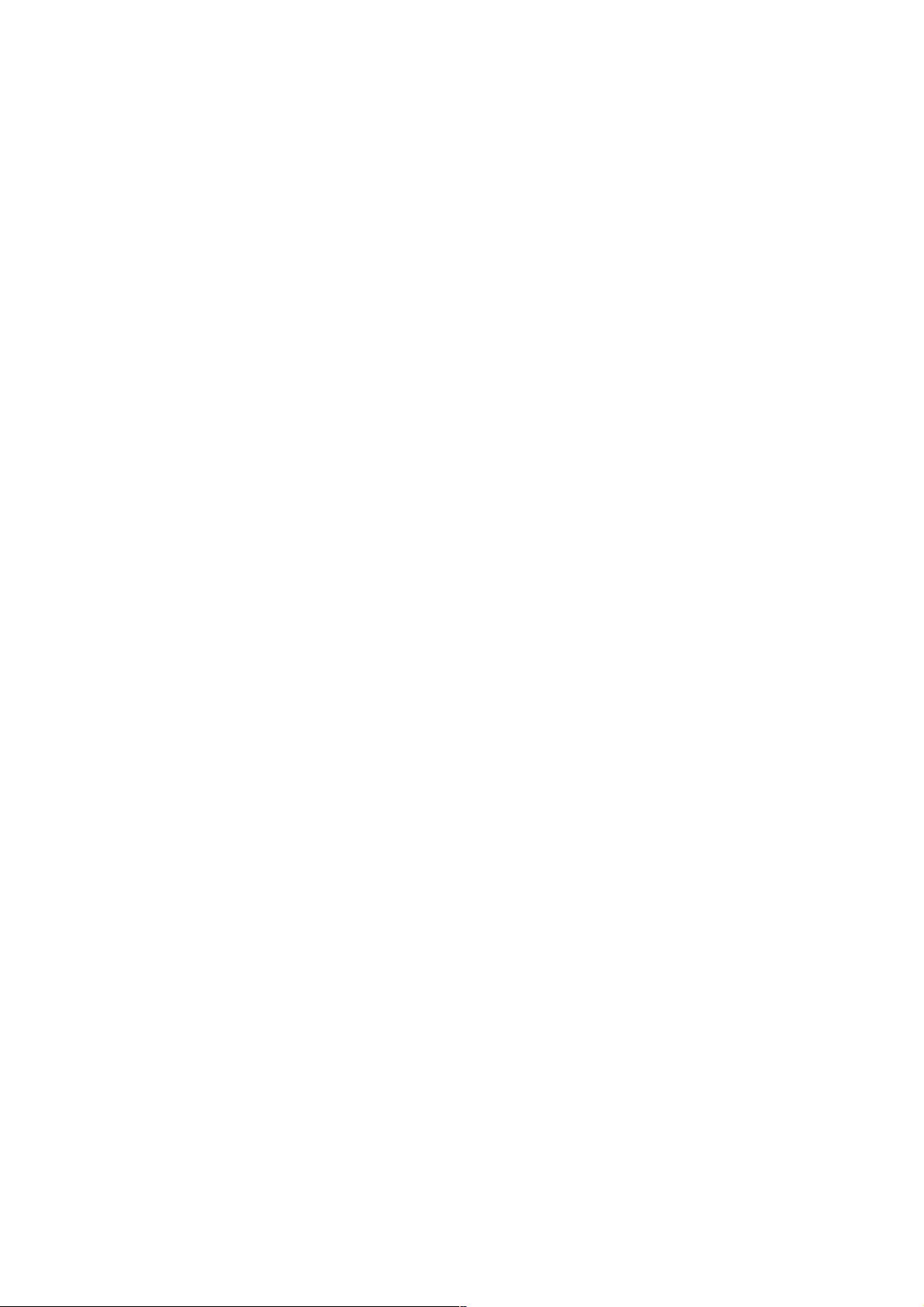
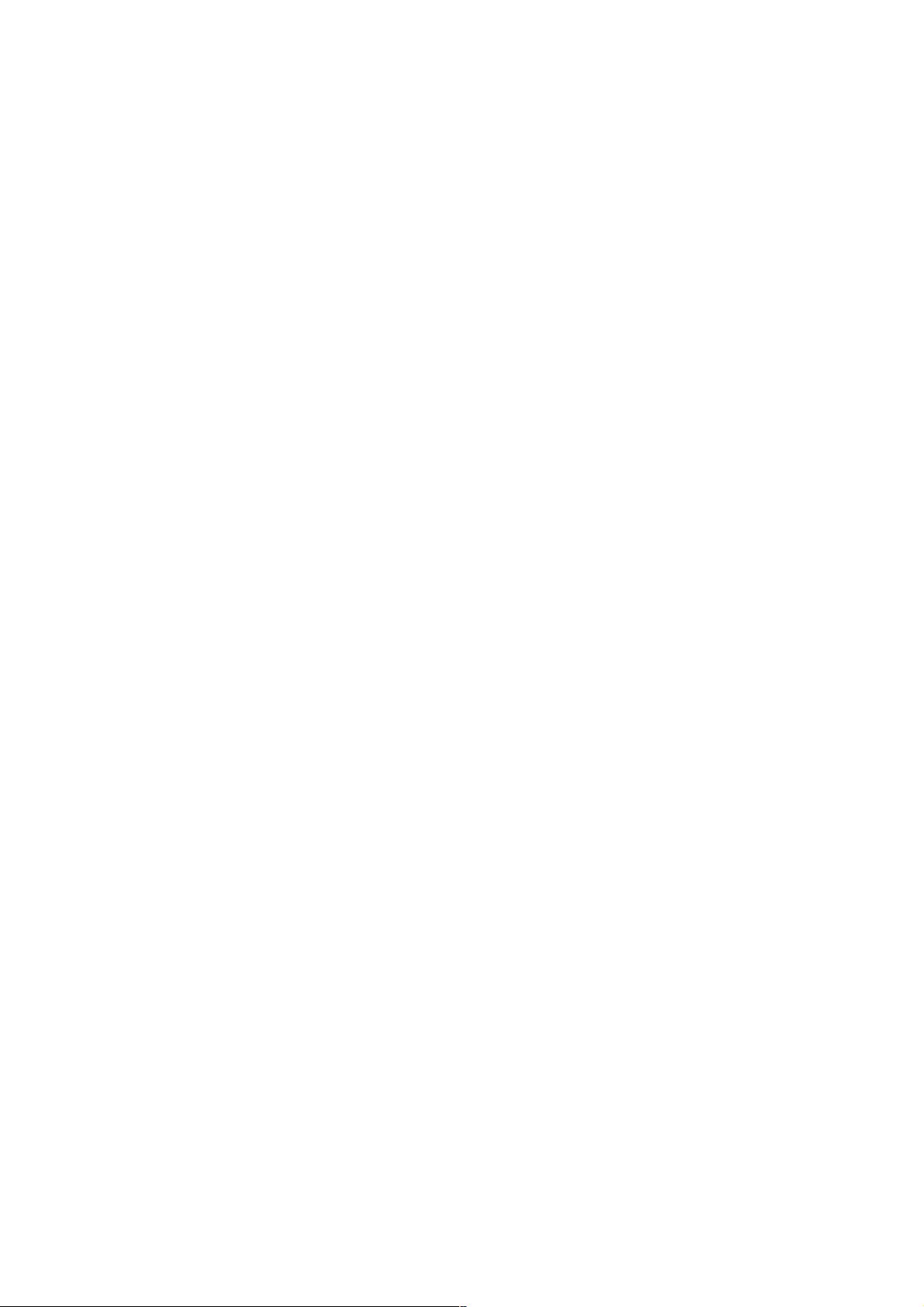
剩余3200页未读,继续阅读
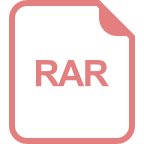
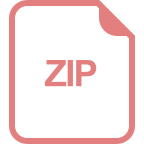

















- 粉丝: 19
- 资源: 21
我的内容管理 展开
我的资源 快来上传第一个资源
我的收益
登录查看自己的收益我的积分 登录查看自己的积分
我的C币 登录后查看C币余额
我的收藏
我的下载
下载帮助

最新资源
- 前端面试必问:真实项目经验大揭秘
- 永磁同步电机二阶自抗扰神经网络控制技术与实践
- 基于HAL库的LoRa通讯与SHT30温湿度测量项目
- avaWeb-mast推荐系统开发实战指南
- 慧鱼SolidWorks零件模型库:设计与创新的强大工具
- MATLAB实现稀疏傅里叶变换(SFFT)代码及测试
- ChatGPT联网模式亮相,体验智能压缩技术.zip
- 掌握进程保护的HOOK API技术
- 基于.Net的日用品网站开发:设计、实现与分析
- MyBatis-Spring 1.3.2版本下载指南
- 开源全能媒体播放器:小戴媒体播放器2 5.1-3
- 华为eNSP参考文档:DHCP与VRP操作指南
- SpringMyBatis实现疫苗接种预约系统
- VHDL实现倒车雷达系统源码免费提供
- 掌握软件测评师考试要点:历年真题解析
- 轻松下载微信视频号内容的新工具介绍

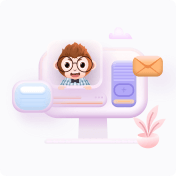
