没有合适的资源?快使用搜索试试~ 我知道了~
首页精通汇编艺术:从基础到高级
"The Art of Assembly Language"
这本书是关于汇编语言的经典教程,旨在深入解析这种低级编程语言的精髓。作者通过丰富的实例和详细的解释,让读者理解汇编语言的各个方面,而非简单地列举规则和指令。以下是书中的主要知识点:
1. **数据表示** (Chapter One: Data Representation): 这一章介绍了计算机如何存储和处理不同类型的数据,包括整数、浮点数以及字符。读者将学习到二进制、八进制、十六进制以及它们与十进制之间的转换,还有位操作的概念。
2. **布尔代数** (Chapter Two: Boolean Algebra): 基础的逻辑运算(AND, OR, NOT, XOR)在汇编语言中至关重要。本章讲解了这些运算的原理及其在电路设计和编程中的应用。
3. **系统组织** (Chapter Three: System Organization): 这部分涵盖了计算机硬件的基础知识,如CPU架构、内存层次结构以及I/O设备的工作原理,这些都是理解汇编语言与硬件交互的关键。
4. **内存布局与访问** (Chapter Four: Memory Layout and Access): 详细讨论了内存的组织方式,包括地址空间、栈和堆的管理,以及如何通过指令直接访问内存。
5. **变量与数据结构** (Chapter Five: Variables and Data Structures): 介绍如何在汇编中声明和操作变量,以及如何构建和操作基本的数据结构,如数组和链表。
6. **80x86指令集** (Chapter Six: The 80x86 Instruction Set): 对Intel 80x86系列处理器的指令进行了详细介绍,包括数据移动、算术运算、逻辑运算、控制流程指令等。
7. **UCR标准库** (Chapter Seven: The UCR Standard Library): 提供了一套实用的汇编语言库函数,用于简化常见任务,如输入输出、数学运算等。
8. **MASM指令与伪指令** (Chapter Eight: MASM: Directives & Pseudo-Opcodes): 讲解了MASM汇编器的使用,包括如何使用指令和伪指令进行程序编写。
9. **算术与逻辑运算** (Chapter Nine: Arithmetic and Logical Operations): 深入探讨了不同类型的运算操作,包括加减乘除、移位操作以及位运算。
10. **控制结构** (Chapter Ten: Control Structures): 包括条件分支、循环和无条件跳转等,这是编写任何程序的基础。
11. **过程与函数** (Chapter Eleven: Procedures and Functions): 介绍了如何定义和调用过程和函数,以及参数传递和局部变量的管理。
12. **高级过程主题** (Chapter Twelve: Procedures: Advanced Topics): 探讨更复杂的函数调用机制,如递归和堆栈操作。
13. **DOS、BIOS和文件I/O** (Chapter Thirteen: MS-DOS, PC-BIOS, and File I/O): 教导读者如何在DOS环境下进行磁盘操作和文件读写。
14. **浮点数运算** (Chapter Fourteen: Floating Point Arithmetic): 解释了浮点数在汇编语言中的表示和计算方法。
15. **字符串与字符集** (Chapter Fifteen: Strings and Character Sets): 讨论了如何处理字符串数据,以及ASCII和其他字符编码系统。
16. **模式匹配** (Chapter Sixteen: Pattern Matching): 介绍了如何在汇编语言中实现文本模式匹配算法。
17. **中断、陷阱与异常** (Chapter Seventeen: Interrupts, Traps, and Exceptions): 揭示了如何处理硬件和软件中断,以及异常处理机制。
18. **驻留程序** (Chapter Eighteen: Resident Programs): 讨论了程序如何在内存中永久驻留并与其他程序交互。
19. **进程、协程与并发** (Chapter Nineteen: Processes, Coroutines, and Concurrency): 阐述了多任务环境下的程序设计,包括简单的并发模型。
20. **PC键盘** (Chapter Twenty: The PC Keyboard): 介绍了如何直接与键盘硬件交互,读取用户输入。
21. **PC并行端口** (Chapter Twenty-One: The PC Parallel Ports): 解释了如何利用并行端口进行数据传输和硬件控制。
22. **PC串行端口** (Chapter Twenty-Two: The PC Serial Ports): 讨论了串行通信协议,如RS-232,并展示了如何编程控制串行端口。
这本书全面覆盖了汇编语言的各个方面,适合希望深入了解底层编程和计算机工作原理的读者。无论是初学者还是经验丰富的程序员,都能从中获得宝贵的见解和技能。
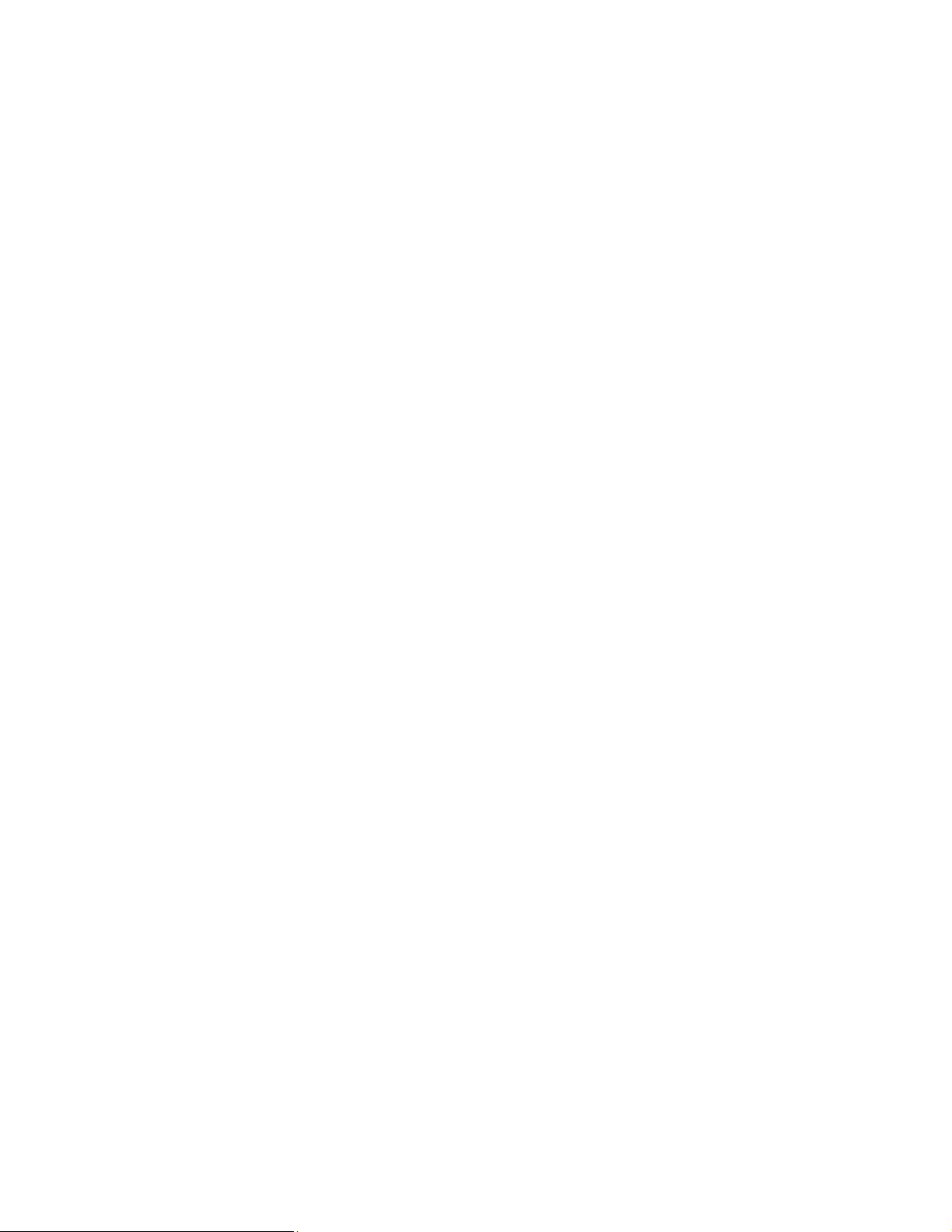
Page xvi
13.4.8 Fwrite ....................................................................................................................753
13.4.9 Redirecting I/O Through the StdLib File I/O Routines ........................................753
13.4.10 A File I/O Example .............................................................................................755
13.5 Sample Program ..............................................................................................................758
13.6 Laboratory Exercises .......................................................................................................763
13.7 Programming Projects .....................................................................................................768
13.8 Summary ..........................................................................................................................768
13.9 Questions .........................................................................................................................770
Chapter 14 Floating Point Arithmetic .......................................................................... 771
14.0 Chapter Overview ...........................................................................................................771
14.1 The Mathematics of Floating Point Arithmetic ...............................................................771
14.2 IEEE Floating Point Formats ............................................................................................774
14.3 The UCR Standard Library Floating Point Routines ........................................................777
14.3.1 Load and Store Routines .......................................................................................778
14.3.2 Integer/Floating Point Conversion .......................................................................779
14.3.3 Floating Point Arithmetic ......................................................................................780
14.3.4 Float/Text Conversion and Printff ........................................................................780
14.4 The 80x87 Floating Point Coprocessors .........................................................................781
14.4.1 FPU Registers ........................................................................................................781
14.4.1.1 The FPU Data Registers .............................................................................. 782
14.4.1.2 The FPU Control Register ........................................................................... 782
14.4.1.3 The FPU Status Register .............................................................................. 785
14.4.2 FPU Data Types ....................................................................................................788
14.4.3 The FPU Instruction Set ........................................................................................789
14.4.4 FPU Data Movement Instructions ........................................................................789
14.4.4.1 The FLD Instruction .................................................................................... 789
14.4.4.2 The FST and FSTP Instructions ................................................................... 790
14.4.4.3 The FXCH Instruction ................................................................................. 790
14.4.5 Conversions ..........................................................................................................791
14.4.5.1 The FILD Instruction ................................................................................... 791
14.4.5.2 The FIST and FISTP Instructions ................................................................ 791
14.4.5.3 The FBLD and FBSTP Instructions ............................................................. 792
14.4.6 Arithmetic Instructions .........................................................................................792
14.4.6.1 The FADD and FADDP Instructions .......................................................... 792
14.4.6.2 The FSUB, FSUBP, FSUBR, and FSUBRP Instructions ............................... 793
14.4.6.3 The FMUL and FMULP Instructions ............................................................ 794
14.4.6.4 The FDIV, FDIVP, FDIVR, and FDIVRP Instructions ................................. 794
14.4.6.5 The FSQRT Instruction ............................................................................... 795
14.4.6.6 The FSCALE Instruction .............................................................................. 795
14.4.6.7 The FPREM and FPREM1 Instructions ........................................................ 795
14.4.6.8 The FRNDINT Instruction ........................................................................... 796
14.4.6.9 The FXTRACT Instruction ........................................................................... 796
14.4.6.10 The FABS Instruction ................................................................................ 796
14.4.6.11 The FCHS Instruction ................................................................................ 797
14.4.7 Comparison Instructions ......................................................................................797
14.4.7.1 The FCOM, FCOMP, and FCOMPP Instructions ........................................ 797
14.4.7.2 The FUCOM, FUCOMP, and FUCOMPP Instructions ................................ 798
14.4.7.3 The FTST Instruction .................................................................................. 798
14.4.7.4 The FXAM Instruction ................................................................................. 798
14.4.8 Constant Instructions ............................................................................................798
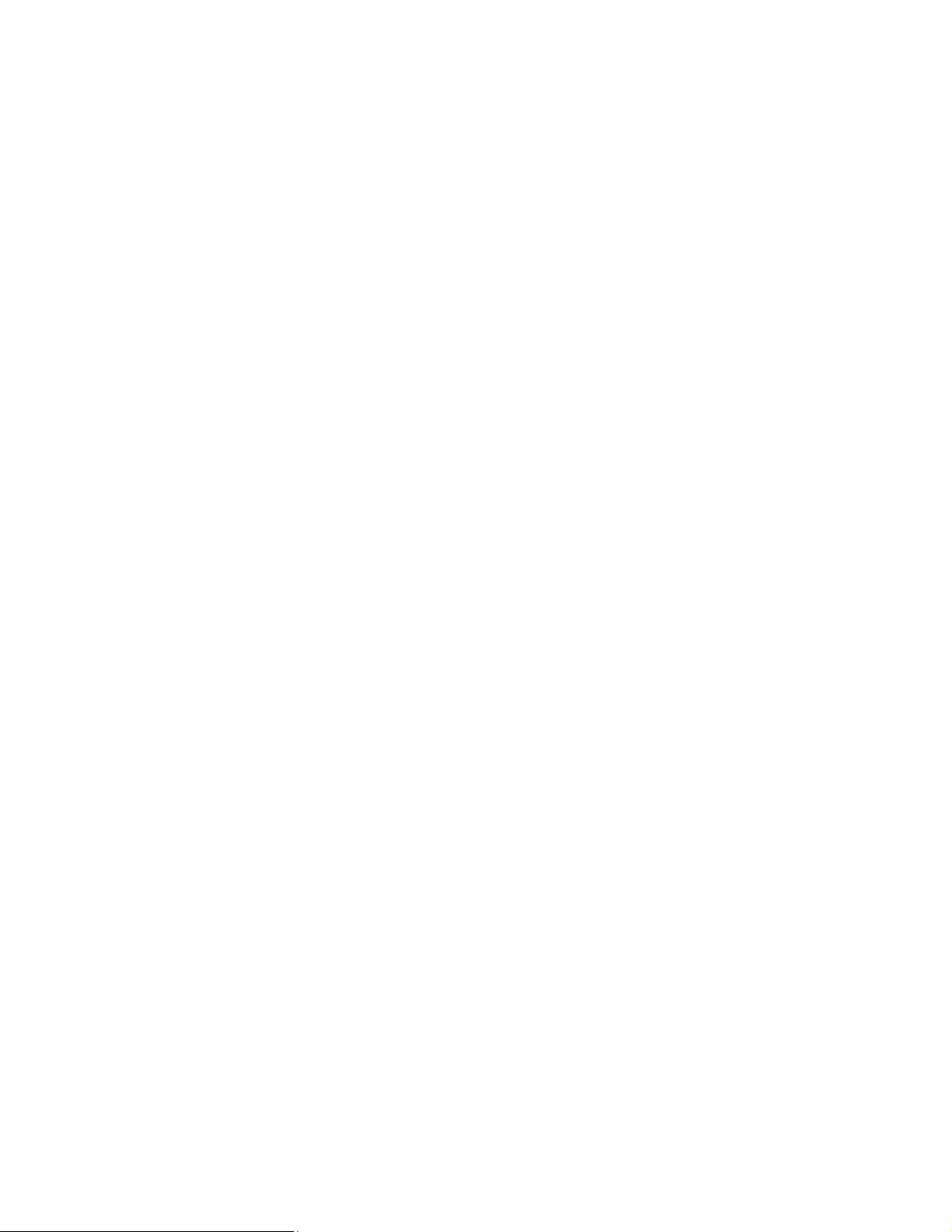
The Art of Assembly Language
Page xvii
14.4.9 Transcendental Instructions ................................................................................799
14.4.9.1 The F2XM1 Instruction ............................................................................... 799
14.4.9.2 The FSIN, FCOS, and FSINCOS Instructions ............................................. 799
14.4.9.3 The FPTAN Instruction .............................................................................. 799
14.4.9.4 The FPATAN Instruction ............................................................................ 800
14.4.9.5 The FYL2X and FYL2XP1 Instructions ....................................................... 800
14.4.10 Miscellaneous instructions ................................................................................. 800
14.4.10.1 The FINIT and FNINIT Instructions ......................................................... 800
14.4.10.2 The FWAIT Instruction ............................................................................. 801
14.4.10.3 The FLDCW and FSTCW Instructions ...................................................... 801
14.4.10.4 The FCLEX and FNCLEX Instructions ...................................................... 801
14.4.10.5 The FLDENV, FSTENV, and FNSTENV Instructions ................................ 801
14.4.10.6 The FSAVE, FNSAVE, and FRSTOR Instructions ..................................... 802
14.4.10.7 The FSTSW and FNSTSW Instructions ..................................................... 803
14.4.10.8 The FINCSTP and FDECSTP Instructions ................................................ 803
14.4.10.9 The FNOP Instruction .............................................................................. 803
14.4.10.10 The FFREE Instruction ............................................................................ 803
14.4.11 Integer Operations ............................................................................................. 803
14.5 Sample Program: Additional Trigonometric Functions ................................................. 804
14.6 Laboratory Exercises .......................................................................................................810
14.6.1 FPU vs StdLib Accuracy ....................................................................................... 811
14.7 Programming Projects ....................................................................................................814
14.8 Summary ......................................................................................................................... 814
14.9 Questions ........................................................................................................................ 817
Chapter 15 Strings and Character Sets ......................................................................... 819
15.0 Chapter Overview ........................................................................................................... 819
15.1 The 80x86 String Instructions ......................................................................................... 819
15.1.1 How the String Instructions Operate ...................................................................819
15.1.2 The REP/REPE/REPZ and REPNZ/REPNE Prefixes ............................................. 820
15.1.3 The Direction Flag ............................................................................................... 821
15.1.4 The MOVS Instruction ......................................................................................... 822
15.1.5 The CMPS Instruction .......................................................................................... 826
15.1.6 The SCAS Instruction ........................................................................................... 828
15.1.7 The STOS Instruction ........................................................................................... 828
15.1.8 The LODS Instruction .......................................................................................... 829
15.1.9 Building Complex String Functions from LODS and STOS ................................ 830
15.1.10 Prefixes and the String Instructions ...................................................................830
15.2 Character Strings ............................................................................................................. 831
15.2.1 Types of Strings .................................................................................................... 831
15.2.2 String Assignment ................................................................................................ 832
15.2.3 String Comparison ............................................................................................... 834
15.3 Character String Functions .............................................................................................835
15.3.1 Substr .................................................................................................................... 835
15.3.2 Index ................................................................................................................... 838
15.3.3 Repeat .................................................................................................................840
15.3.4 Insert ...................................................................................................................841
15.3.5 Delete ................................................................................................................... 843
15.3.6 Concatenation ...................................................................................................... 844
15.4 String Functions in the UCR Standard Library ................................................................845
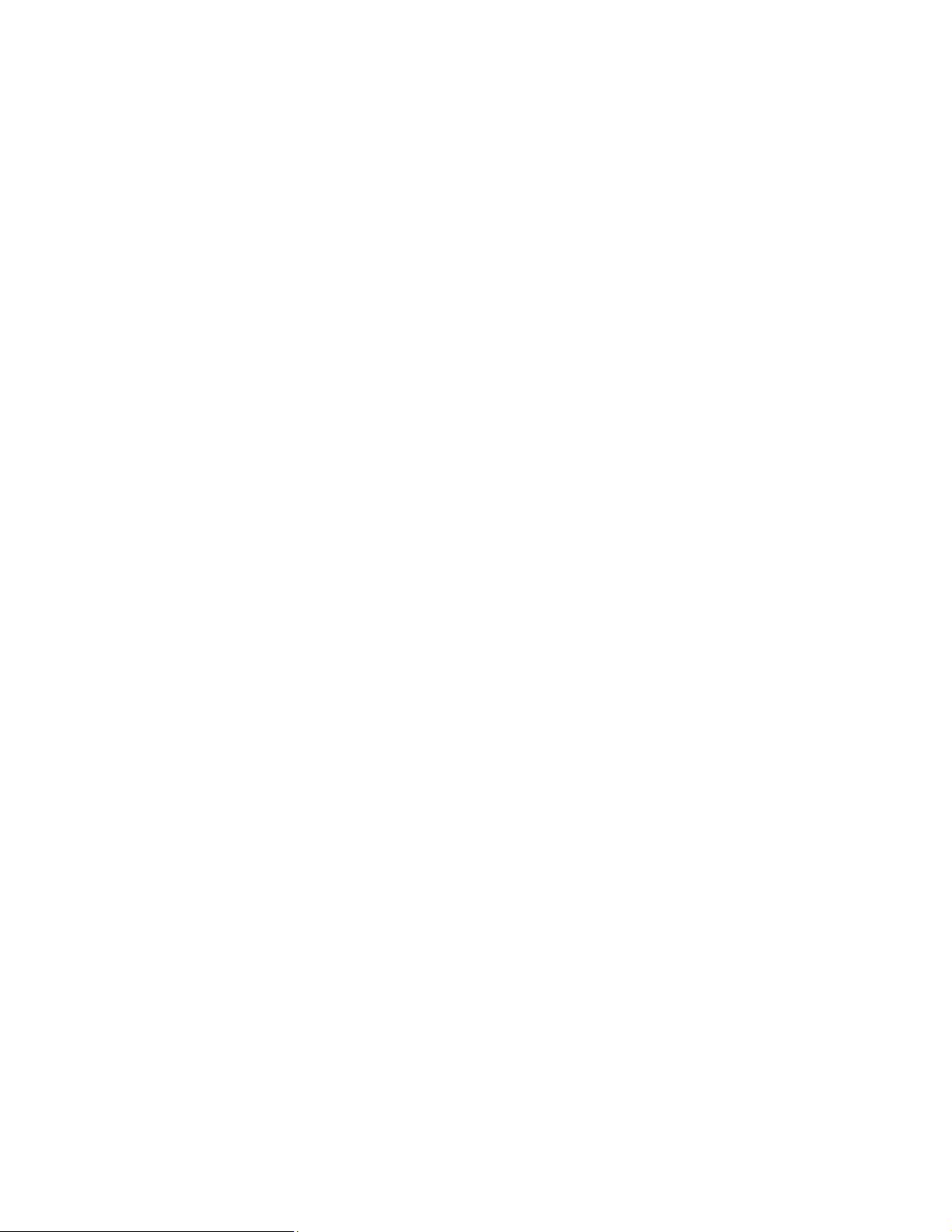
Page xviii
15.4.1 StrBDel, StrBDelm ................................................................................................846
15.4.2 Strcat, Strcatl, Strcatm, Strcatml ............................................................................847
15.4.3 Strchr .....................................................................................................................848
15.4.4 Strcmp, Strcmpl, Stricmp, Stricmpl .......................................................................848
15.4.5 Strcpy, Strcpyl, Strdup, Strdupl .............................................................................849
15.4.6 Strdel, Strdelm .......................................................................................................850
15.4.7 Strins, Strinsl, Strinsm, Strinsml ............................................................................851
15.4.8 Strlen .....................................................................................................................852
15.4.9 Strlwr, Strlwrm, Strupr, Struprm ...........................................................................852
15.4.10 Strrev, Strrevm .....................................................................................................853
15.4.11 Strset, Strsetm ......................................................................................................853
15.4.12 Strspan, Strspanl, Strcspan, Strcspanl .................................................................854
15.4.13 Strstr, Strstrl .........................................................................................................855
15.4.14 Strtrim, Strtrimm ..................................................................................................855
15.4.15 Other String Routines in the UCR Standard Library ...........................................856
15.5 The Character Set Routines in the UCR Standard Library ...............................................856
15.6 Using the String Instructions on Other Data Types ........................................................859
15.6.1 Multi-precision Integer Strings .............................................................................859
15.6.2 Dealing with Whole Arrays and Records .............................................................860
15.7 Sample Programs .............................................................................................................860
15.7.1 Find.asm ................................................................................................................860
15.7.2 StrDemo.asm .........................................................................................................862
15.7.3 Fcmp.asm ..............................................................................................................865
15.8 Laboratory Exercises .......................................................................................................868
15.8.1 MOVS Performance Exercise #1 ...........................................................................868
15.8.2 MOVS Performance Exercise #2 ...........................................................................870
15.8.3 Memory Performance Exercise ............................................................................872
15.8.4 The Performance of Length-Prefixed vs. Zero-Terminated Strings .....................874
15.9 Programming Projects .....................................................................................................878
15.10 Summary ........................................................................................................................878
15.11 Questions .......................................................................................................................881
Chapter 16 Pattern Matching ....................................................................................... 883
16.1 An Introduction to Formal Language (Automata) Theory .............................................883
16.1.1 Machines vs. Languages .......................................................................................883
16.1.2 Regular Languages ................................................................................................884
16.1.2.1 Regular Expressions .................................................................................... 885
16.1.2.2 Nondeterministic Finite State Automata (NFAs) ........................................ 887
16.1.2.3 Converting Regular Expressions to NFAs ................................................... 888
16.1.2.4 Converting an NFA to Assembly Language ................................................ 890
16.1.2.5 Deterministic Finite State Automata (DFAs) .............................................. 893
16.1.2.6 Converting a DFA to Assembly Language .................................................. 895
16.1.3 Context Free Languages .......................................................................................900
16.1.4 Eliminating Left Recursion and Left Factoring CFGs ...........................................903
16.1.5 Converting REs to CFGs .......................................................................................905
16.1.6 Converting CFGs to Assembly Language .............................................................905
16.1.7 Some Final Comments on CFGs ...........................................................................912
16.1.8 Beyond Context Free Languages .........................................................................912
16.2 The UCR Standard Library Pattern Matching Routines ...................................................913
16.3 The Standard Library Pattern Matching Functions .........................................................914
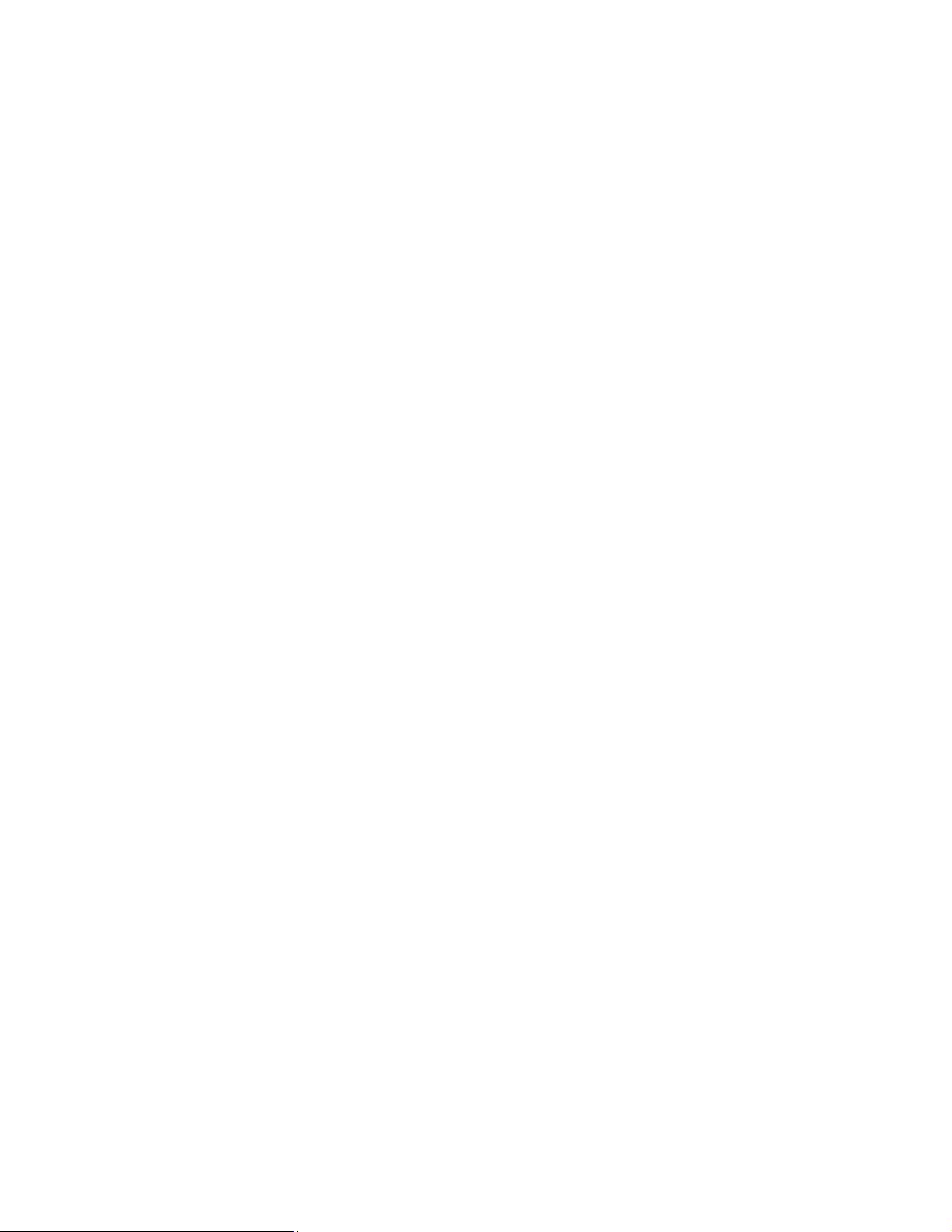
The Art of Assembly Language
Page xix
16.3.1 Spancset ............................................................................................................... 914
16.3.2 Brkcset .................................................................................................................. 915
16.3.3 Anycset ................................................................................................................. 915
16.3.4 Notanycset ........................................................................................................... 916
16.3.5 MatchStr ................................................................................................................ 916
16.3.6 MatchiStr ............................................................................................................... 916
16.3.7 MatchToStr ........................................................................................................... 917
16.3.8 MatchChar ............................................................................................................ 917
16.3.9 MatchToChar ........................................................................................................918
16.3.10 MatchChars ......................................................................................................... 918
16.3.11 MatchToPat ........................................................................................................ 918
16.3.12 EOS ..................................................................................................................... 919
16.3.13 ARB ..................................................................................................................... 919
16.3.14 ARBNUM ............................................................................................................ 920
16.3.15 Skip ..................................................................................................................... 920
16.3.16 Pos ...................................................................................................................... 921
16.3.17 RPos ....................................................................................................................921
16.3.18 GotoPos ..............................................................................................................921
16.3.19 RGotoPos ...........................................................................................................922
16.3.20 SL_Match2 .......................................................................................................... 922
16.4 Designing Your Own Pattern Matching Routines ..........................................................922
16.5 Extracting Substrings from Matched Patterns ................................................................925
16.6 Semantic Rules and Actions ............................................................................................ 929
16.7 Constructing Patterns for the MATCH Routine .............................................................. 933
16.8 Some Sample Pattern Matching Applications ................................................................ 935
16.8.1 Converting Written Numbers to Integers ............................................................935
16.8.2 Processing Dates .................................................................................................. 941
16.8.3 Evaluating Arithmetic Expressions ...................................................................... 948
16.8.4 A Tiny Assembler ................................................................................................. 953
16.8.5 The “MADVENTURE” Game ................................................................................963
16.9 Laboratory Exercises .......................................................................................................979
16.9.1 Checking for Stack Overflow (Infinite Loops) .................................................... 979
16.9.2 Printing Diagnostic Messages from a Pattern ......................................................984
16.10 Programming Projects ................................................................................................... 988
16.11 Summary .......................................................................................................................988
16.12 Questions ...................................................................................................................... 991
Section Four: ............................................................................................................... 993
Advanced Assembly Language Programming ...................................................................... 993
Chapter 17 Interrupts, Traps, and Exceptions ............................................................ 995
17.1 80x86 Interrupt Structure and Interrupt Service Routines (ISRs) ..................................996
17.2 Traps ............................................................................................................................... 999
17.3 Exceptions ......................................................................................................................1000
17.3.1 Divide Error Exception (INT 0) ........................................................................... 1000
17.3.2 Single Step (Trace) Exception (INT 1) ................................................................1000
17.3.3 Breakpoint Exception (INT 3) ............................................................................. 1001
17.3.4 Overflow Exception (INT 4/INTO) .....................................................................1001
17.3.5 Bounds Exception (INT 5/BOUND) ...................................................................1001
17.3.6 Invalid Opcode Exception (INT 6) ...................................................................... 1004
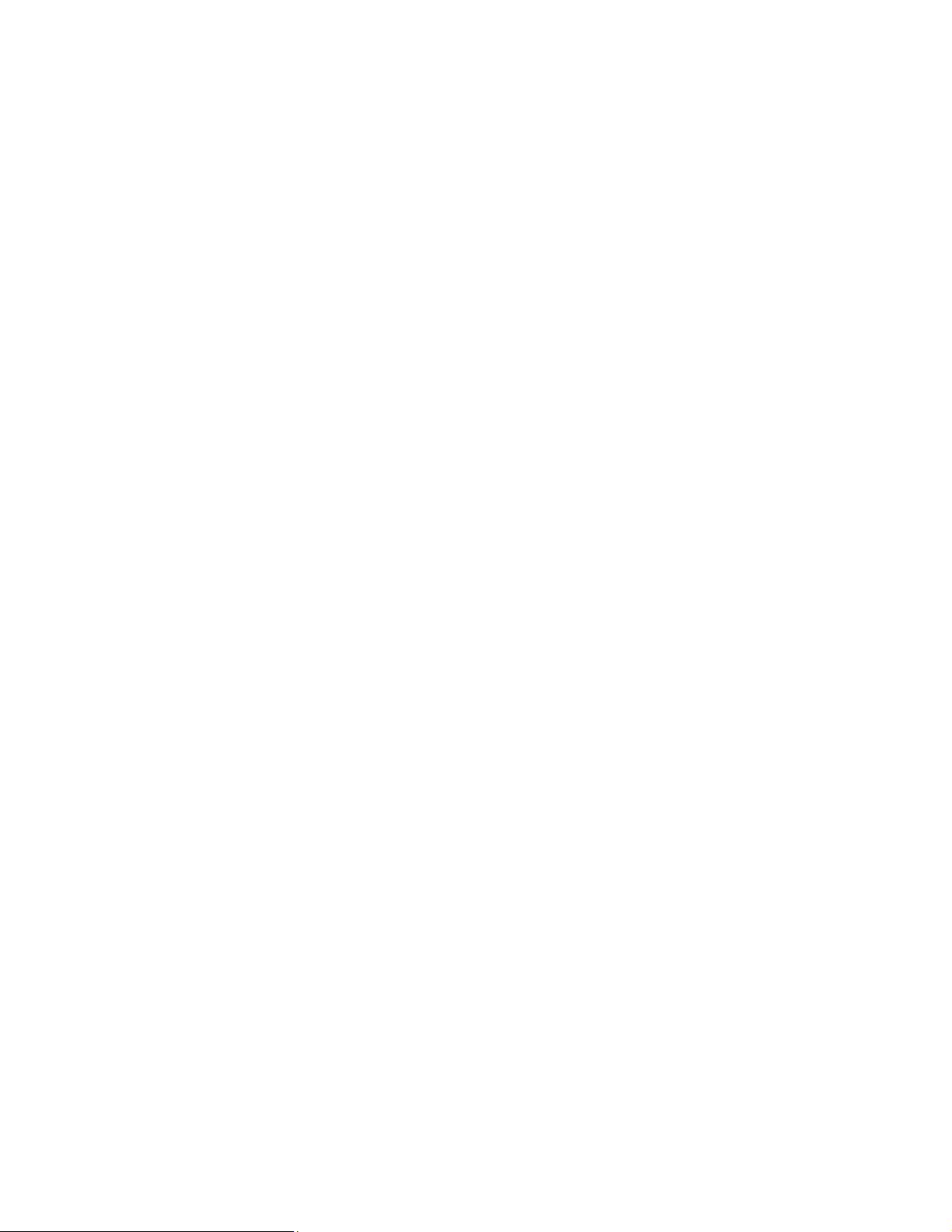
Page xx
17.3.7 Coprocessor Not Available (INT 7) ......................................................................1004
17.4 Hardware Interrupts ........................................................................................................1004
17.4.1 The 8259A Programmable Interrupt Controller (PIC) .........................................1005
17.4.2 The Timer Interrupt (INT 8) .................................................................................1007
17.4.3 The Keyboard Interrupt (INT 9) ...........................................................................1008
17.4.4 The Serial Port Interrupts (INT 0Bh and INT 0Ch) ..............................................1008
17.4.5 The Parallel Port Interrupts (INT 0Dh and INT 0Fh) ...........................................1008
17.4.6 The Diskette and Hard Drive Interrupts (INT 0Eh and INT 76h) ........................1009
17.4.7 The Real-Time Clock Interrupt (INT 70h) ............................................................1009
17.4.8 The FPU Interrupt (INT 75h) ................................................................................1009
17.4.9 Nonmaskable Interrupts (INT 2) ..........................................................................1009
17.4.10 Other Interrupts ..................................................................................................1009
17.5 Chaining Interrupt Service Routines ...............................................................................1010
17.6 Reentrancy Problems ......................................................................................................1012
17.7 The Efficiency of an Interrupt Driven System ................................................................1014
17.7.1 Interrupt Driven I/O vs. Polling ...........................................................................1014
17.7.2 Interrupt Service Time ..........................................................................................1015
17.7.3 Interrupt Latency ..................................................................................................1016
17.7.4 Prioritized Interrupts .............................................................................................1020
17.8 Debugging ISRs ...............................................................................................................1020
17.9 Summary ..........................................................................................................................1021
Chapter 18 Resident Programs ................................................................................... 1025
18.1 DOS Memory Usage and TSRs ........................................................................................1025
18.2 Active vs. Passive TSRs ....................................................................................................1029
18.3 Reentrancy .......................................................................................................................1032
18.3.1 Reentrancy Problems with DOS ...........................................................................1032
18.3.2 Reentrancy Problems with BIOS ..........................................................................1033
18.3.3 Reentrancy Problems with Other Code ...............................................................1034
18.4 The Multiplex Interrupt (INT 2Fh) ..................................................................................1034
18.5 Installing a TSR ................................................................................................................1035
18.6 Removing a TSR ...............................................................................................................1037
18.7 Other DOS Related Issues ...............................................................................................1039
18.8 A Keyboard Monitor TSR ................................................................................................1041
18.9 Semiresident Programs ....................................................................................................1055
18.10 Summary ........................................................................................................................1064
Chapter 19 Processes, Coroutines, and Concurrency ............................................... 1065
19.1 DOS Processes .................................................................................................................1065
19.1.1 Child Processes in DOS ........................................................................................1065
19.1.1.1 Load and Execute ....................................................................................... 1066
19.1.1.2 Load Program .............................................................................................. 1068
19.1.1.3 Loading Overlays ........................................................................................ 1069
19.1.1.4 Terminating a Process ................................................................................. 1069
19.1.1.5 Obtaining the Child Process Return Code ................................................. 1070
19.1.2 Exception Handling in DOS: The Break Handler ................................................1070
19.1.3 Exception Handling in DOS: The Critical Error Handler .....................................1071
19.1.4 Exception Handling in DOS: Traps ......................................................................1075
19.1.5 Redirection of I/O for Child Processes ................................................................1075
剩余1426页未读,继续阅读
2017-09-21 上传
2014-03-13 上传
2008-03-01 上传
2009-05-05 上传
2010-10-29 上传
2019-02-24 上传
2024-11-08 上传

demornov
- 粉丝: 0
- 资源: 8
上传资源 快速赚钱
我的内容管理 展开
我的资源 快来上传第一个资源
我的收益
登录查看自己的收益我的积分 登录查看自己的积分
我的C币 登录后查看C币余额
我的收藏
我的下载
下载帮助

最新资源
- 构建基于Django和Stripe的SaaS应用教程
- Symfony2框架打造的RESTful问答系统icare-server
- 蓝桥杯Python试题解析与答案题库
- Go语言实现NWA到WAV文件格式转换工具
- 基于Django的医患管理系统应用
- Jenkins工作流插件开发指南:支持Workflow Python模块
- Java红酒网站项目源码解析与系统开源介绍
- Underworld Exporter资产定义文件详解
- Java版Crash Bandicoot资源库:逆向工程与源码分享
- Spring Boot Starter 自动IP计数功能实现指南
- 我的世界牛顿物理学模组深入解析
- STM32单片机工程创建详解与模板应用
- GDG堪萨斯城代码实验室:离子与火力基地示例应用
- Android Capstone项目:实现Potlatch服务器与OAuth2.0认证
- Cbit类:简化计算封装与异步任务处理
- Java8兼容的FullContact API Java客户端库介绍
安全验证
文档复制为VIP权益,开通VIP直接复制
