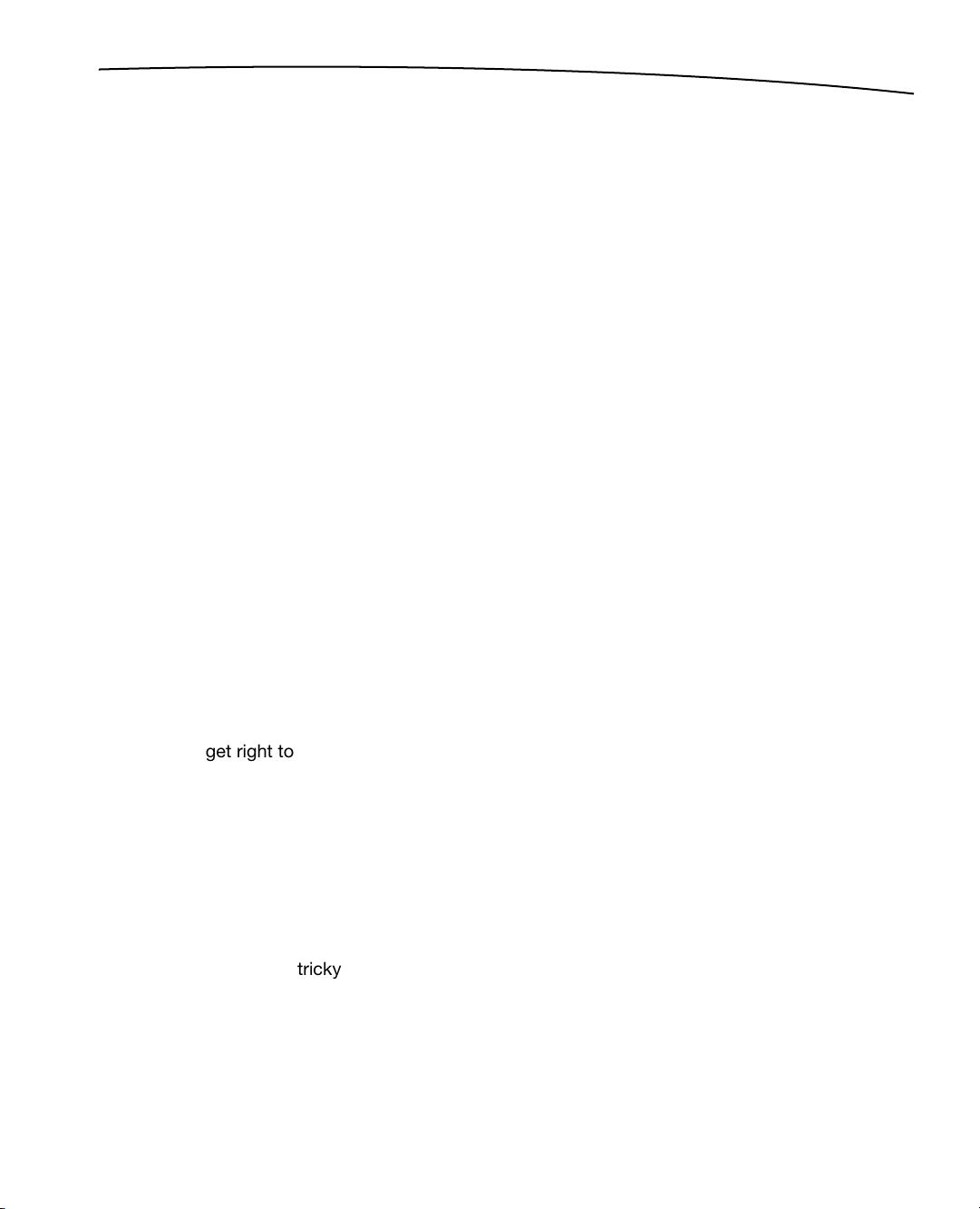
11CHAPTER 1: Hello World
Here is the bulk of the useful code. This is where everything that you saw happened when you ran
the game. The “Hello, World!” label, the debugging information, where the spaceships were spawned
from, and where the spinning actions were initiated—all of this happened when the user “touched”
the iPhone screen, and you clicked in the Simulator screen. That’s a lot going on for two short
methods, don’t you think?
Let’s examine it in more detail. First, take a look at the initWithSize method.
self.backgroundColor = [SKColor colorWithRed:0.15 green:0.15 blue:0.3 alpha:1.0];
Here, you set a background color using RGB (color) and alpha (transparency) values.
SKLabelNode *myLabel = [SKLabelNode labelNodeWithFontNamed:@"Chalkduster"];
myLabel.text = @"Hello, World!";
myLabel.fontSize = 30;
myLabel.position = CGPointMake(CGRectGetMidX(self.frame), CGRectGetMidY(self.frame));
Then you create an SKLabelNode object and set some important values such as its font, size,
and position, as well as the text itself. For the position calculation, you might notice that some
convenience methods (CGPointMake and GetMidX) are being used to determine the center of the
screen using the SKScene frame property.
[self addChild:myLabel];
Finally, you add the SKLabelNode that you created as a child of the SKScene. This is how sprites or
nodes are added to the screen: by using the addChild method of the SKScene object.
You may have noticed that all of this happened inside the scene initialization. In other words,
immediately after the app launch, since this scene will be instantiated when the storyboard is loaded.
This method then becomes the best place to add code to take care of whatever you want to have
happen when the user starts your game. You may want to go have some sort of splash screen, or
you may just get right to the point by presenting the user with several buttons from which to select:
a one- or two-player game perhaps.
Finally, you have the touchesBeganWithEvent method. As you will infer from its name, this method
will be called when the user places a finger on the screen. Not when they lift their finger off the
screen (that would be the touchesEndedWithEvent method), as is the common method employed
when using buttons, for instance. This allows the users to change their mind when making choices
between buttons. But that’s a different topic for a different book. When a user touches the screen in
this game, you don’t want to delay immediate action, so this is a better choice for method picking.
The for loop is used so that multiple simultaneous touches can be recognized and handled
accordingly. This is a bit tricky on the simulator. However, on an actual device, try pressing two
fingers down at the same time, and you’ll see what you would expect: two spaceships appear where
your fingers were placed.
CGPoint location = [touch locationInNode:self];
For each touch event, you first determine the position of the touch.
SKSpriteNode *sprite = [SKSpriteNode spriteNodeWithImageNamed:@"Spaceship"];
www.it-ebooks.info