"CUNY CSci235的软件设计与分析II课程讲义,由Stewart Weiss教授主讲,涵盖了编程与软件工程的关键概念,包括软件工程的定义、重要性和应用,以及对象导向问题解决的方法。" 在CUNY CSci235的“软件设计与分析II”课程中,主要探讨了编程与软件工程的核心概念。软件工程是一个多领域交叉的学科,它结合了计算机科学、项目管理和更多领域的技术和实践,以规范、设计、开发和维护软件应用程序。课程指出,没有一种万能的最佳方法来构建软件,也没有统一的理论指导如何进行,但软件工程师们在软件开发过程中确实共享了一些共同的理念。 首先,课程强调编码前缺乏解决方案设计会显著增加调试时间,因此在开始编码之前,团队需要有一个整体的计划。对于大型软件开发项目,团队需要组织结构、明确的沟通以及协调工作。这些元素确保项目的高效进行,避免因无序开发导致的问题和延误。 其次,软件工程并不仅限于大规模项目。它的原则同样适用于小型程序的开发。这些原则涵盖设计方法、测试策略、文档编写和开发过程的管理。课程可能会深入讨论如何将这些原则应用于不同规模的项目,以优化开发流程。 在对象导向问题解决方面,课程可能会介绍对象导向分析(OOA)和对象导向设计(OOD)。这是一种以对象为中心的方法,通过识别问题域中的实体(对象)和它们之间的关系来解决问题。OOA和OOD强调封装、继承和多态等核心概念,帮助开发者创建更灵活、可扩展和易于维护的代码。通过实例化和消息传递,对象可以相互交互,实现复杂的功能。 此外,课程可能还会涉及软件生命周期模型,如瀑布模型、敏捷开发、螺旋模型等,讨论它们各自的优点和适用场景。测试是软件开发中的关键环节,课程可能会讲解单元测试、集成测试和系统测试等不同层次的测试方法,以及自动化测试工具的使用。 最后,文档在整个软件开发过程中起着至关重要的作用。课程可能会涵盖需求规格说明书、设计文档、用户手册等不同类型文档的编写,强调清晰、准确的文档对软件质量和可维护性的影响。 CUNY CSci235的这门课程旨在通过理论与实践的结合,帮助学生掌握软件工程的全面知识,从而能够在实际项目中应用所学,提高软件开发的效率和质量。
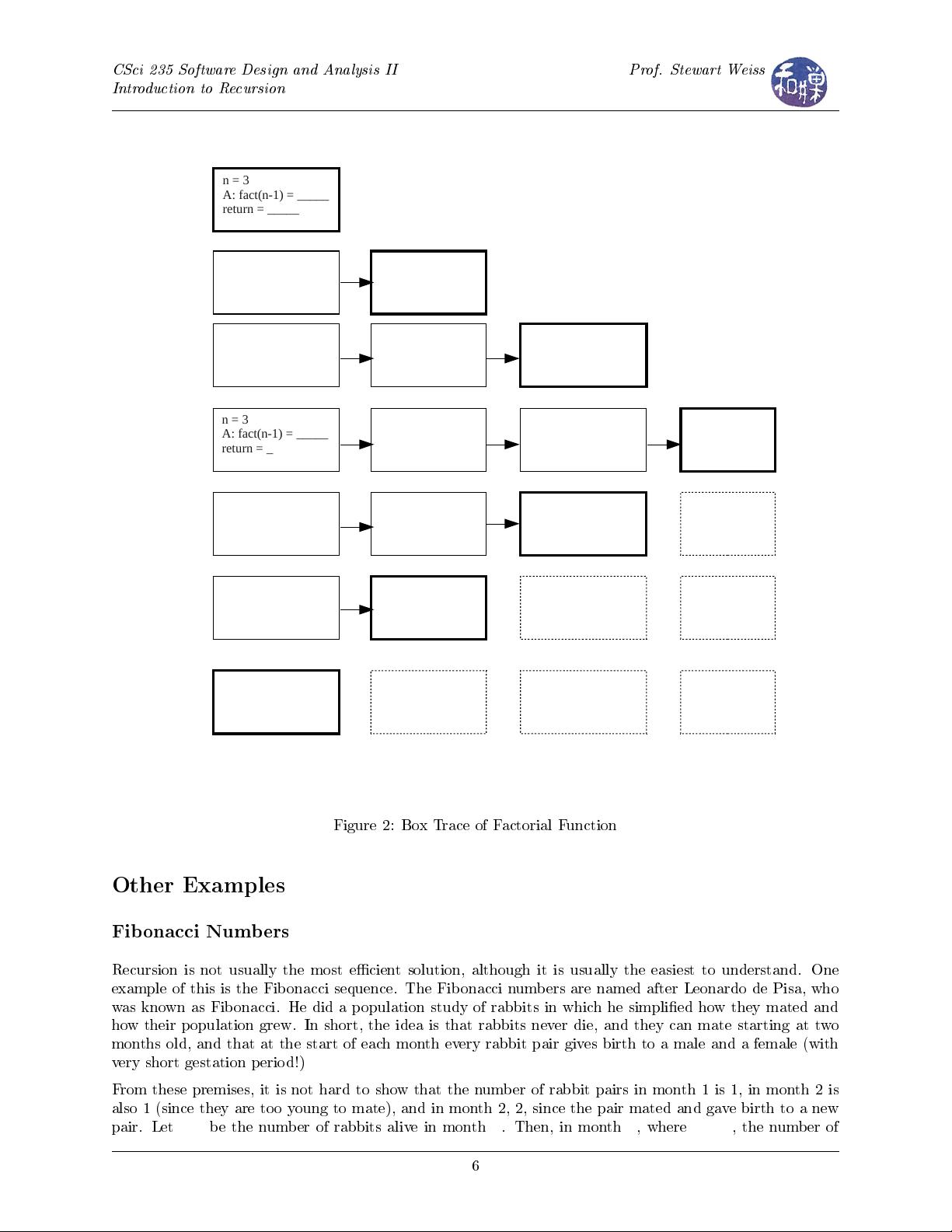
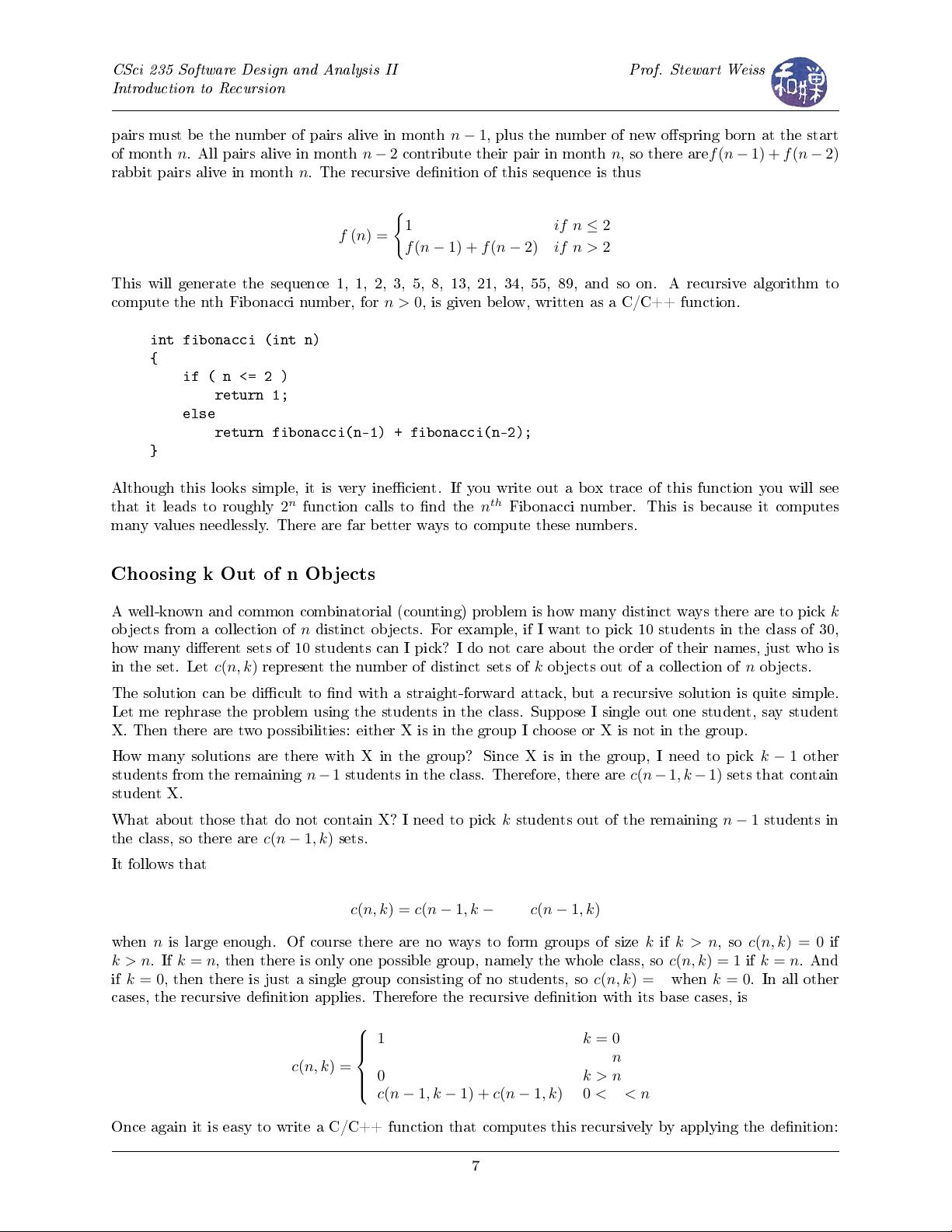
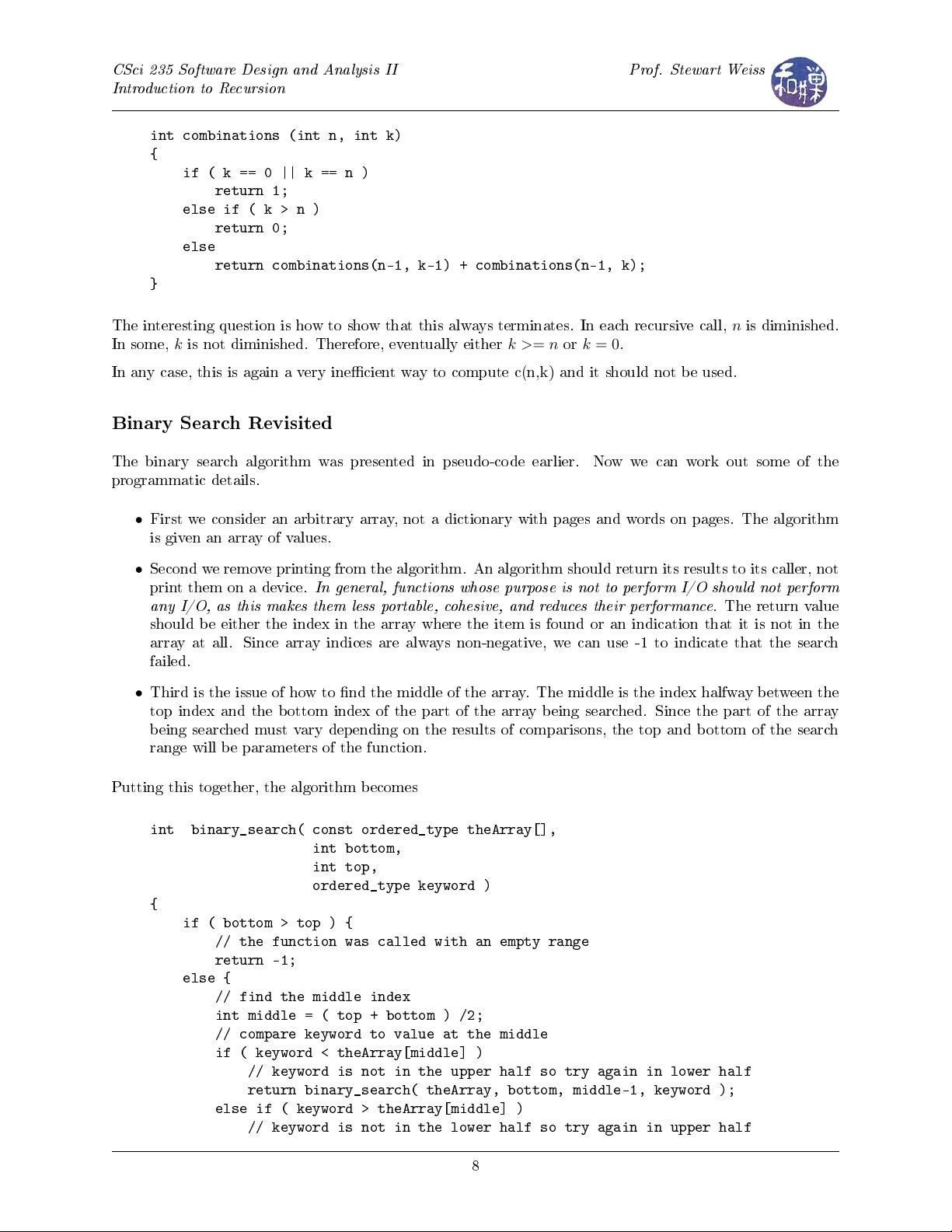
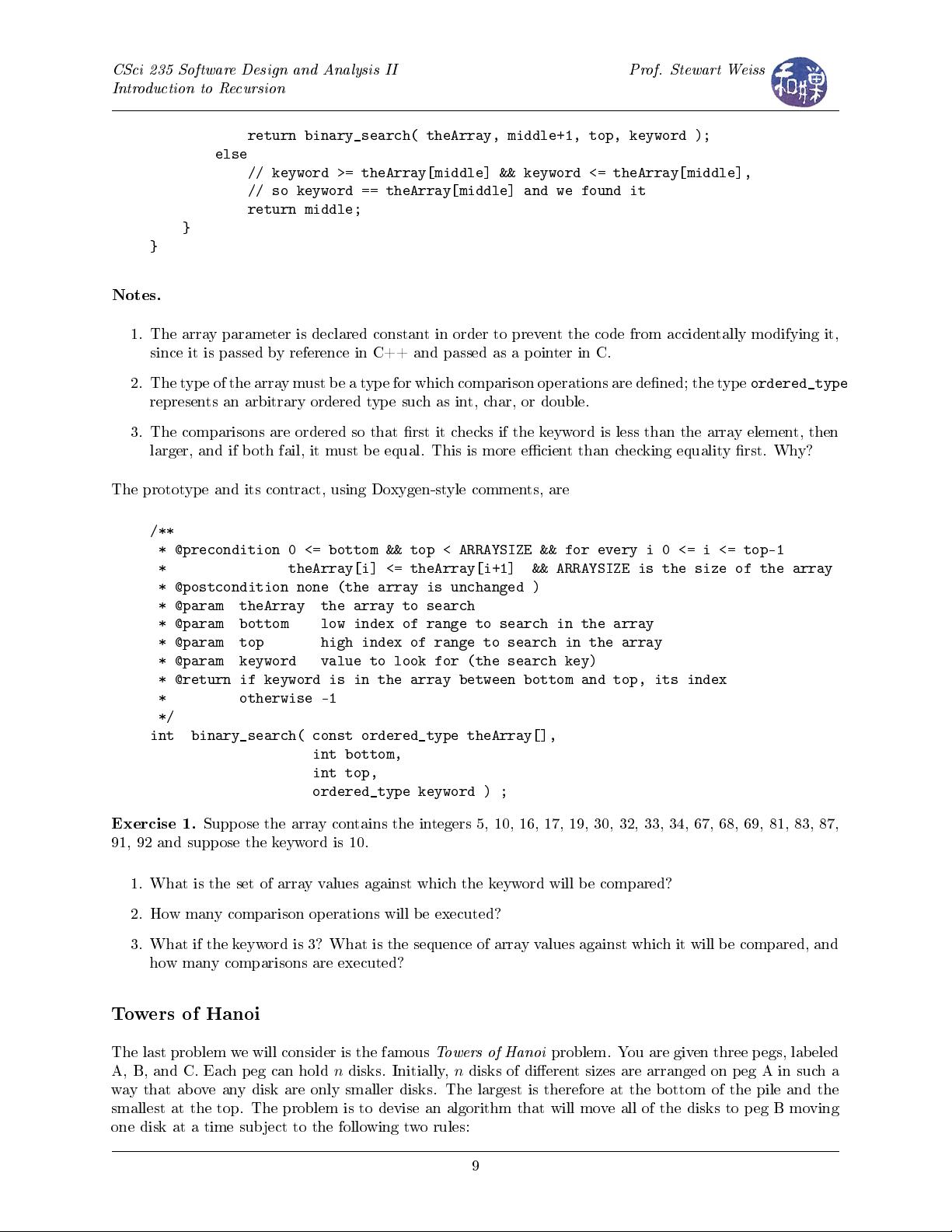
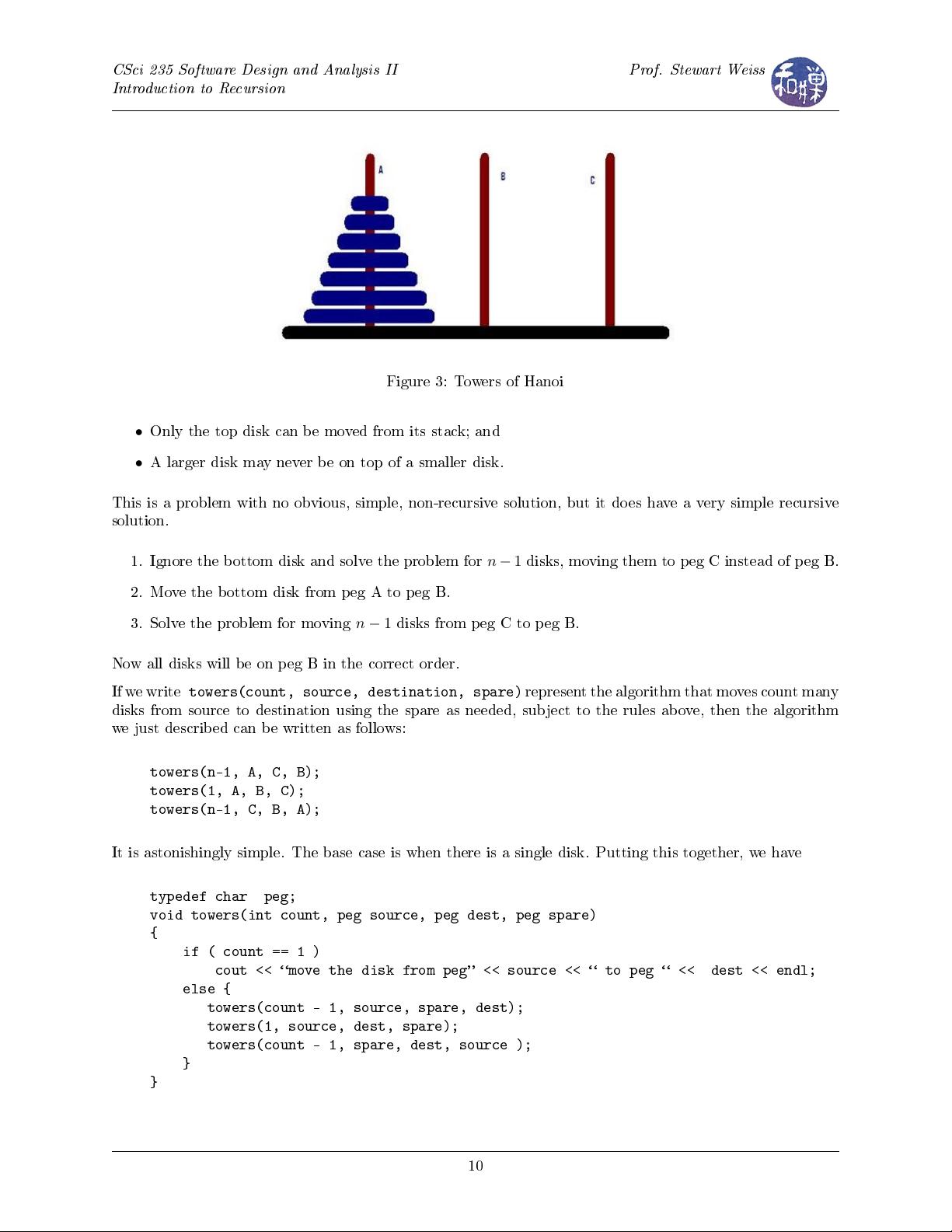
剩余162页未读,继续阅读
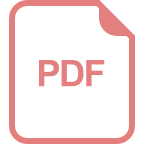



















- 粉丝: 3w+
- 资源: 1088
我的内容管理 展开
我的资源 快来上传第一个资源
我的收益
登录查看自己的收益我的积分 登录查看自己的积分
我的C币 登录后查看C币余额
我的收藏
我的下载
下载帮助

最新资源
- 构建Cadence PSpice仿真模型库教程
- VMware 10.0安装指南:步骤详解与网络、文件共享解决方案
- 中国互联网20周年必读:影响行业的100本经典书籍
- SQL Server 2000 Analysis Services的经典MDX查询示例
- VC6.0 MFC操作Excel教程:亲测Win7下的应用与保存技巧
- 使用Python NetworkX处理网络图
- 科技驱动:计算机控制技术的革新与应用
- MF-1型机器人硬件与robobasic编程详解
- ADC性能指标解析:超越位数、SNR和谐波
- 通用示波器改造为逻辑分析仪:0-1字符显示与电路设计
- C++实现TCP控制台客户端
- SOA架构下ESB在卷烟厂的信息整合与决策支持
- 三维人脸识别:技术进展与应用解析
- 单张人脸图像的眼镜边框自动去除方法
- C语言绘制图形:余弦曲线与正弦函数示例
- Matlab 文件操作入门:fopen、fclose、fprintf、fscanf 等函数使用详解

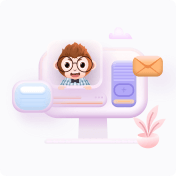
