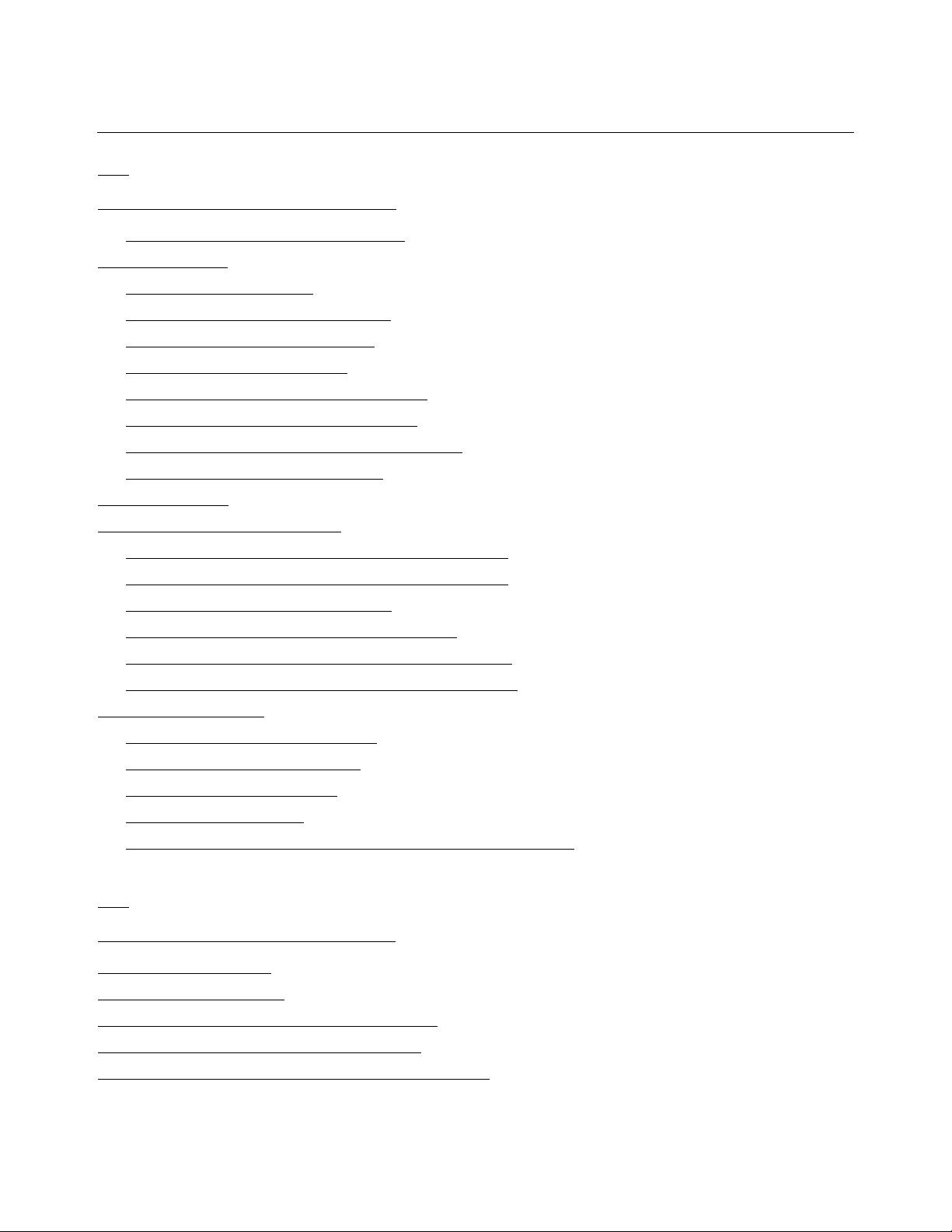
SKILL Language User Guide
March 2003 16 Product Version 06.10
16
SKILL++ Object System . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 331
The Common Lisp Object System . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 332
Basic Concepts . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 332
Classes and Instances . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 332
Generic Functions and Methods . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 333
Subclasses and Superclasses . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 333
Defining a Class (defclass) . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 333
Instantiating a Class (makeInstance) . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 335
Reading and Writing Instance Slots . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 335
Defining a Generic Function (defgeneric) . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 336
Defining a Method (defmethod) . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 337
Class Hierarchy . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 338
Browsing the Class Hierarchy . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 339
Getting the Class Object from the Class Name . . . . . . . . . . . . . . . . . . . . . . . . . . . . 340
Getting the Class Name from the Class Object . . . . . . . . . . . . . . . . . . . . . . . . . . . . 340
Getting the Class of an Instance . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 340
Getting the Superclasses of an Instance . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 340
Checking if an Object Is an Instance of a Class . . . . . . . . . . . . . . . . . . . . . . . . . . . . 340
Checking if One Class Is a Subclass of Another . . . . . . . . . . . . . . . . . . . . . . . . . . . 341
Advanced Concepts . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 341
Method Argument Restrictions . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 342
Applying a Generic Function . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 342
Incremental Development . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 344
Methods versus Slots . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 345
Sharing Private Functions and Data Between Methods . . . . . . . . . . . . . . . . . . . . . . 345
17
Programming Examples . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 347
Symbol Manipulation . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 348
Sorting a List of Points . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 349
Computing the Center of a Bounding Box . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 350
Computing the Area of a Bounding Box . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 351
Computing a Bounding Box Centered at a Point . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 351