C#编程:使用GET方法获取网页源代码
本文将介绍如何在C#中使用GET方法获取网页的源代码,主要涉及.NET框架中的WebRequest、WebResponse和WebClient类。 在C#编程中,获取网页源码通常用于网络爬虫或者需要解析网页内容的场景。以下是两种常用的方法: 1. 使用WebRequest和WebResponse: ```csharp using System; using System.Collections.Generic; using System.Text; using System.Net; using System.IO; namespace Search { class GetHttp { public static string GetHttpCountent(string url) { string html = null; WebRequest req = WebRequest.Create(url); WebResponse res = req.GetResponse(); Stream receiveStream = res.GetResponseStream(); // 需要确定网页编码,这里以"gb2312"为例 Encoding encode = Encoding.GetEncoding("gb2312"); StreamReader sr = new StreamReader(receiveStream, encode); char[] readBuffer = new char[256]; int n = sr.Read(readBuffer, 0, 256); while (n > 0) { string str = new string(readBuffer, 0, n); html += str; n = sr.Read(readBuffer, 0, 256); } return html; } } } ``` 在这个示例中,首先创建一个WebRequest对象,然后调用`Create`方法初始化,传入目标URL。接着,通过`GetResponse`获取响应,然后读取响应流。由于网页可能有不同的编码,这里假设网页编码为“gb2312”,并使用`Encoding.GetEncoding`获取相应的编码器。最后,使用StreamReader读取流内容并拼接成字符串。 2. 使用WebClient: ```csharp public static string GetHttpContent(string url) { string strWebData = null; try { WebClient myWebClient = new WebClient(); myWebClient.Credentials = CredentialCache.DefaultCredentials; // 下载网页数据为字节数组 byte[] myDataBuffer = myWebClient.DownloadData(url); // 将字节数组转换为字符串 strWebData = Encoding.Default.GetString(myDataBuffer); // 此处可以添加处理网页编码的逻辑,例如查找meta标签中的charset属性 Match charSetMatch = Regex.Match(strWebData, @"<meta([^<]*)charset=([^<]*?)\s*?/", RegexOptions.IgnoreCase | RegexOptions.Multiline); string webChar = charSetMatch.Groups[2].Value; // 如果找到charset,可以根据找到的编码值重新解码 } catch (Exception ex) { // 处理异常情况 } return strWebData; } ``` WebClient是.NET提供的一个简单易用的类,可以直接下载网页内容为字节数组。这里同样需要处理网页编码,可以通过正则表达式匹配meta标签中的charset属性来确定正确的编码。如果找到charset,可以使用`Encoding.GetEncoding`创建新的编码器,并用它来解码字节数组得到字符串。 总结,C#中获取网页源码的方法主要包括使用WebRequest/WebResponse的组合,以及使用WebClient。这两种方法都需要考虑网页的编码问题,确保正确地解析和处理获取到的数据。在实际应用中,可能还需要处理网络异常、超时等错误情况,以提高程序的健壮性。
using System.Collections.Generic;
using System.Text;
using System.Net;
using System.IO;
// 读取网页源码类
namespace Search
{
class GetHttp
{
public static string GetHttpCountent(string url)
{
string html = null;
WebRequest req = WebRequest.Create(url);
WebResponse res = req.GetResponse();
Stream receiveStream = res.GetResponseStream();
Encoding encode = Encoding.GetEncoding("gb2312");
StreamReader sr = new StreamReader(receiveStream, encode);
char[] readbuffer = new char[256];
int n = sr.Read(readbuffer, 0, 256);
while (n > 0)
{
string str = new string(readbuffer, 0, n);
html += str;
n = sr.Read(readbuffer, 0, 256);
}
return html;
}
//取网页源码
public static string GetHttpContent(string url)
{
string strWebData = null;
try
{
WebClient myWebClient = new WebClient();
myWebClient.Credentials = CredentialCache.DefaultCredentials;
byte[] myDataBuffer = myWebClient.DownloadData(url);
strWebData = Encoding.Default.GetString(myDataBuffer);
Match charSetMatch = Regex.Match(strWebData, "<meta([^<]*)charset=([^<]*?)\"", RegexOptions.IgnoreCase | RegexOptions.Multiline);
string webCharSet = charSetMatch.Groups[2].Value;
if (webCharSet != null && webCharSet != "" && Encoding.GetEncoding(webCharSet) != Encoding.Default)
strWebData = Encoding.GetEncoding(webCharSet).GetString(myDataBuffer);
}
catch
{
}
return strWebData;
}
/**/
/// <summary>
/// 获取指定网页的源码,支持编码自动识别
/// </summary>
/// <param name="url"></param>
/// <returns></returns>
剩余5页未读,继续阅读
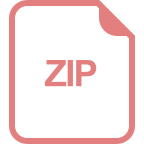
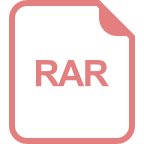
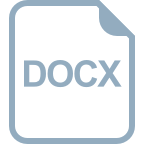

















- 粉丝: 2
- 资源: 3
我的内容管理 展开
我的资源 快来上传第一个资源
我的收益
登录查看自己的收益我的积分 登录查看自己的积分
我的C币 登录后查看C币余额
我的收藏
我的下载
下载帮助

最新资源
- 李兴华Java基础教程:从入门到精通
- U盘与硬盘启动安装教程:从菜鸟到专家
- C++面试宝典:动态内存管理与继承解析
- C++ STL源码深度解析:专家级剖析与关键技术
- C/C++调用DOS命令实战指南
- 神经网络补偿的多传感器航迹融合技术
- GIS中的大地坐标系与椭球体解析
- 海思Hi3515 H.264编解码处理器用户手册
- Oracle基础练习题与解答
- 谷歌地球3D建筑筛选新流程详解
- CFO与CIO携手:数据管理与企业增值的战略
- Eclipse IDE基础教程:从入门到精通
- Shell脚本专家宝典:全面学习与资源指南
- Tomcat安装指南:附带JDK配置步骤
- NA3003A电子水准仪数据格式解析与转换研究
- 自动化专业英语词汇精华:必备术语集锦

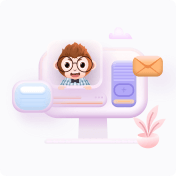
