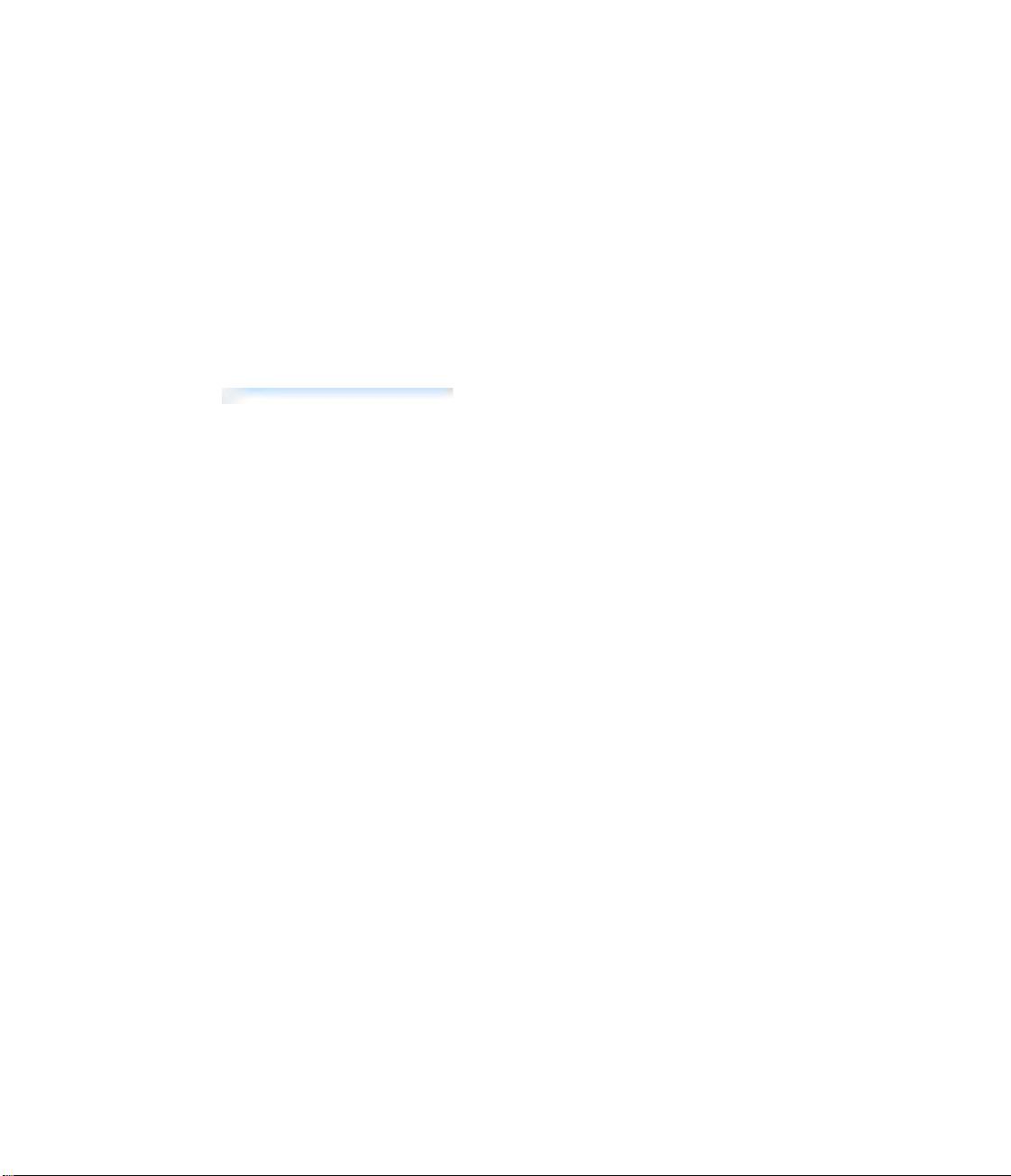
2 | Chapter 1: Software Engineering Principles
At this point in your computing career, you have completed at least one semester of
computer science course work. You can take a problem of medium complexity, write an
algorithm to solve the problem, code the algorithm in C++, and demonstrate the correct-
ness of your solution. At least, that’s what the syllabus for your introductory class said
you should be able to do when you complete the course. Now that you are starting your
second (or third?) semester, it is time to stop and review those principles that, if adhered
to, guarantee that you can indeed do what your previous syllabus claimed.
In this chapter, we review the software design process and the verification of soft-
ware correctness. In Chapter 2, we review data design and implementation.
1.1
The Software Process
When we consider computer programming, we immediately think of writing a program
for a computer to execute—the generation of code in some computer language. As a
beginning student of computer science, you wrote programs that solved relatively sim-
ple problems. Much of your initial effort went into learning the syntax of a program-
ming language such as C++: the language’s reserved words, its data types, its constructs
for selection (if-else and switch) and looping (while, do while, and for), and its
input/output mechanisms (cin and cout).
You may have learned a programming methodology that took you from the problem
description that your instructor handed out all the way through the delivery of a good
software solution. Programmers have created many design techniques, coding standards,
and testing methods to help develop high-quality software. But why bother with all that
methodology? Why not just sit down at a computer and write programs? Aren’t we
wasting a lot of time and effort, when we could just get started on the “real” job?
If the degree of our programming sophistication never had to rise above the level of
trivial programs (like summing a list of prices or averaging grades), we might get away
with such a code-first technique (or, rather, lack of technique). Some new programmers
work this way, hacking away at the code until the program works more or less cor-
rectly—usually less.
As your programs grow larger and more complex, however, you must pay attention
to other software issues in addition to coding. If you become a software professional,
someday you may work as part of a team that develops a system containing tens of
thousands, or even millions, of lines of code. The activities involved in such a software
project’s whole “life cycle” clearly go beyond just sitting down at a computer and writ-
ing programs. These activities include
• Problem analysis Understanding the nature of the problem to be solved
• Requirements elicitation Determining exactly what the program must do
• Requirements definition Specifying what the program must do (functional
requirements) and any constraints on the solution approach (nonfunctional
requirements such as what language to use)
• High- and low-level design Recording how the program meets the requirements,
from the “big picture” overview to the detailed design