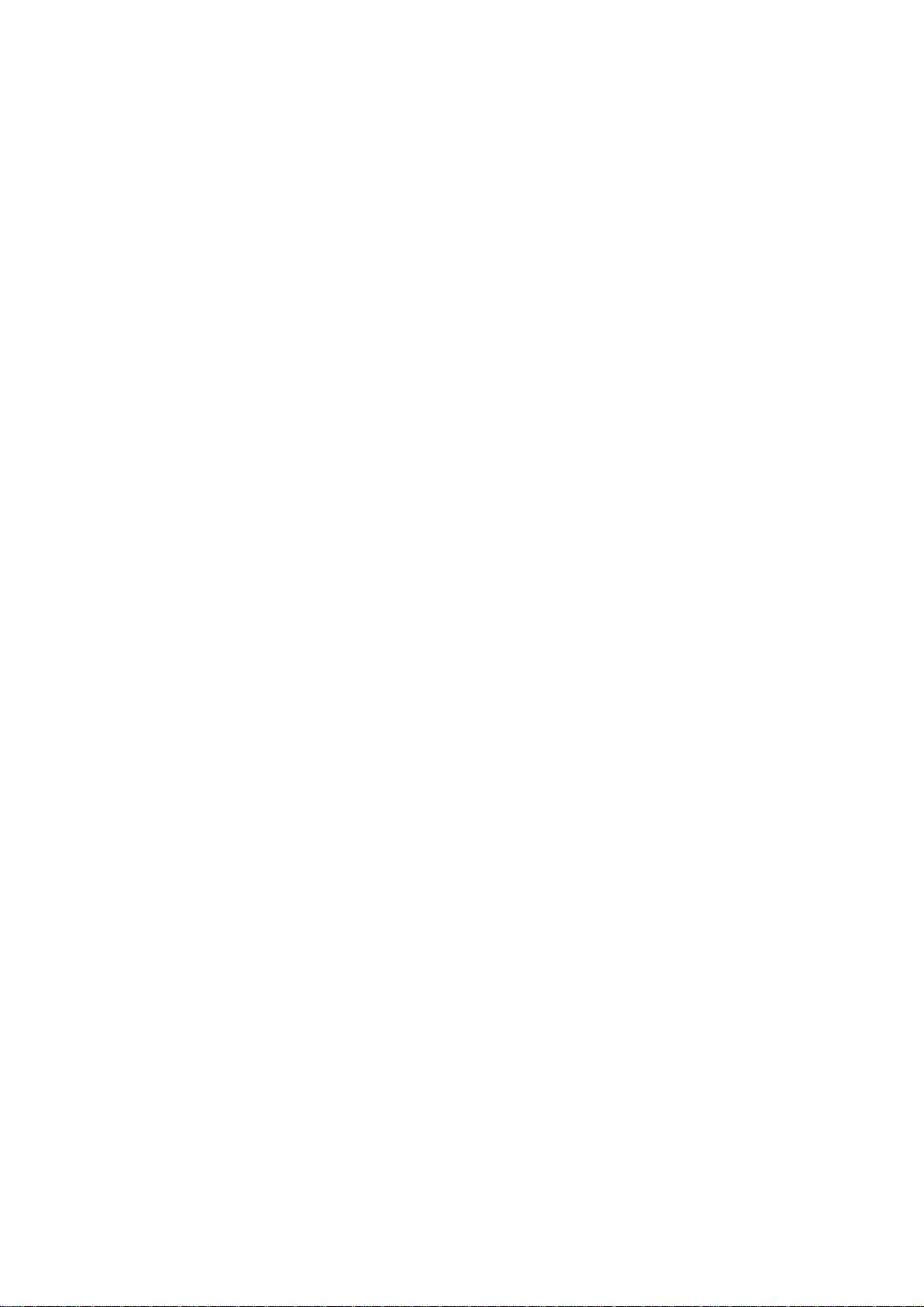
Programming Web Services with XML-RPC
- 17 -
An XML-RPC request is always followed by exactly one XML-RPC response; the response is
synchronous with the request. This happens because the response must occur on the same
HTTP connection as the request.
Furthermore, the client process blocks (waits) until it receives a response from the server.
This step has consequences for program design: your code should be written in such a way
that the potential blocking of a response for some time will not affect its operation, or else
your program should restrict itself to calling remote methods that execute in "reasonable" time.
"Reasonable" may vary, according to the needs of your program. Methods that are called
frequently in time-sensitive environments may be unreasonable if they take more than a
fraction of a second; methods that are called occasionally to return large volumes of
information may be perfectly reasonable even if they take a few minutes to return the full
collection of information requested.
It is possible to implement an asynchronous system, where the response to a request is
delivered at some point subsequent to the time of request. However, this implementation
would require both processes to be XML-RPC client and server enabled and to contain a
significant amount of code for handling such asynchronous responses. Systems that require
asynchronous responses can build such systems on the synchronous foundation of XML-
RPC using multiple request-response cycles. One simple way forward would be to have the
server process return a unique identifier and the calling process implement a special XML-
RPC method that allows the transmission of results corresponding with the request. If the
transactions are conducted over the Internet, this also means that both processes must be
accessible through any firewall that might be in place.
In general, synchronous requests are capable of fulfilling many processes' requirements, and
the overhead of creating an asynchronous system is probably prohibitive in most cases.
2.1.2 Stateless
HTTP is an inherently stateless technology. This means that no context is preserved from one
request to the next. XML-RPC inherits this feature. So, if your client program invokes one
method on the server and invokes it again, XML-RPC itself has no way to treat them as
anything other than two isolated, unconnected incidents. This avoids the sometimes massive
overhead involved in maintaining state across multiple systems.
But there are good reasons why you might want to preserve state. For instance, one request
might create a certain object inside the server, and subsequent requests modify that object.
Users of HTTP have gotten around its statelessness by storing state server-side and using
identifiers held in cookies, or in the URL of the page itself, to indicate to the server a
continuation of a session of requests. Although this is often necessary for projects like web-
based shopping carts, the program-to-program communication of XML-RPC doesn't need this
infrastructure for the simple processes it supports.
Although XML-RPC itself provides no support for preservation of state, you can implement a
stateful system on top of XML-RPC. For instance, you could make the first argument of all
your procedure calls be a session identifier. The procedures would need to have some kind of
state management system, perhaps a database, in the background. They could then keep
track of which calls came from where and perhaps use the history of previous calls to
determine responses to current calls.
2.2 Data Types
XML-RPC calls closely model function calls in programming languages. An invocation of a
remote method is accompanied by data in the form of parameters, and the response to that
method contains data as a return value. Although a request may contain multiple parameters,
the response must contain exactly one return value.